Common Tidy Codebase Mistakes You Must Avoid
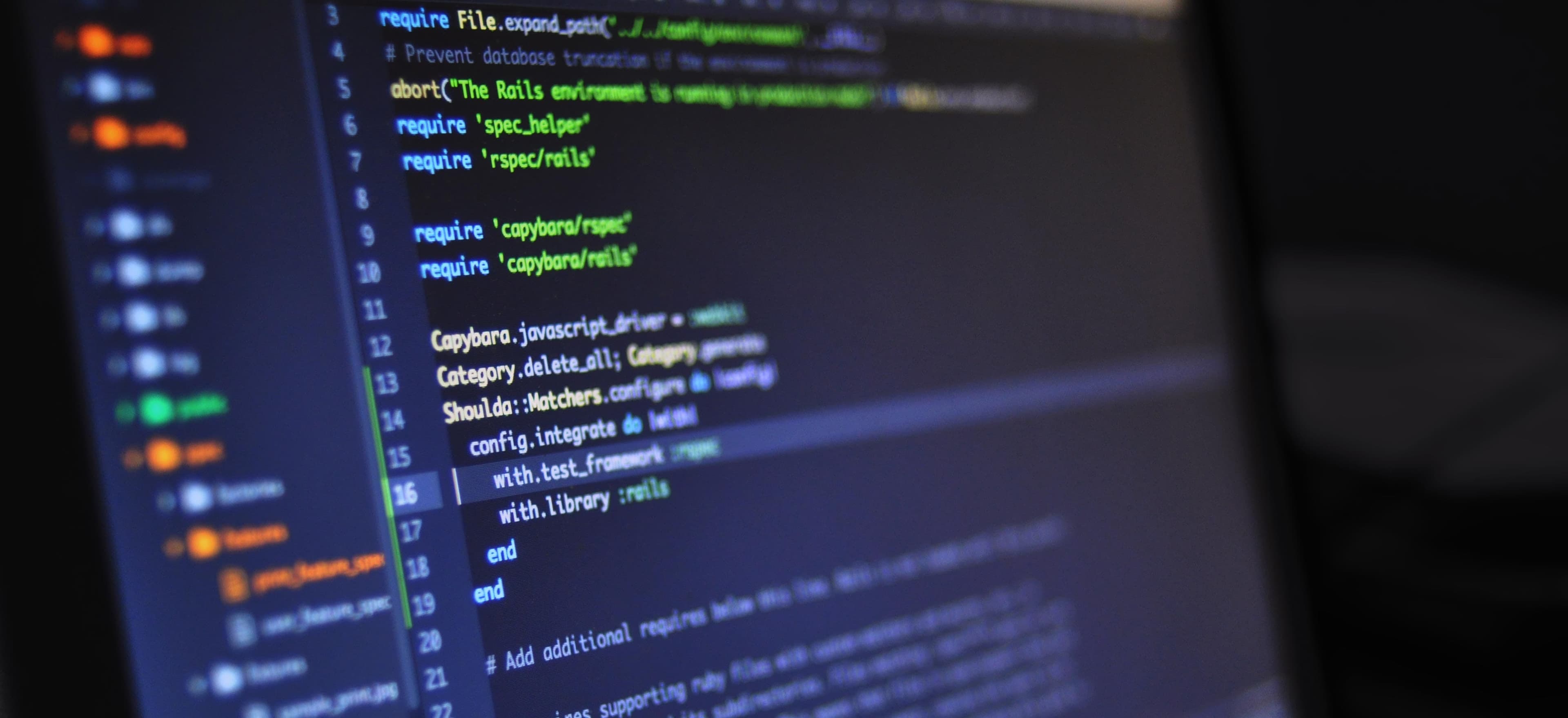
- Published on
Common Tidy Codebase Mistakes You Must Avoid
In the dynamic world of software development, maintaining a tidy and organized codebase is not just a best practice—it's a necessity. A clean codebase contributes to enhanced readability, easier debugging, and better collaboration among developers. However, many developers, especially those new to Java, can fall into a few common traps that can compromise the quality of their code. In this blog post, we will explore some of these mistakes, provide insights on how to avoid them, and illustrate with practical Java code snippets.
1. Ignoring Naming Conventions
The Importance of Naming
In Java, adhering to naming conventions is crucial for code readability. Not following these conventions can make your code look unprofessional, and it can even mislead other developers.
Good Practice:
- Use CamelCase for classes and interfaces.
- Use lowerCamelCase for methods and variables.
- Use ALL_CAPS for constants.
Example Code Snippet:
Here is a comparison between a poor naming convention and a cleaner approach:
// Bad Practice
class myclass {
int numberTwo;
void UpdateValue(int newvalue) {
numberTwo = newvalue;
}
}
// Good Practice
class MyClass {
private int numberTwo;
void updateValue(int newValue) {
this.numberTwo = newValue;
}
}
Why It Matters
The revised example not only follows naming conventions but also improves readability, making it easier for you and your team to understand the code at a glance. Following naming conventions builds a standardized language within your codebase.
2. Lack of Comments
Code Comments Are Key
Comments are essential for documenting your code, especially when the logic gets complex. A common mistake is writing code without enough comments, leaving others (or yourself, at a later time) confused about your logic.
Example Code Snippet:
// Bad Practice
public void calculateSpeciesPopulation() {
int population = 5;
population++;
// population updated
}
// Good Practice
/**
* Calculates the new population of the species.
* Increments the current population by one.
*/
public void calculateSpeciesPopulation() {
int population = 5; // Initial population
population++; // Increment population to account for a new member
}
Why It Matters
Well-placed comments explain the "why" behind your code. They make it easier for anyone reviewing the code to comprehend its functionality without having to dissect every line.
3. Overusing Comments
Finding the Balance
While comments are beneficial, over-commenting can lead to clutter and confusion. Avoid stating the obvious; rather, focus on explaining complex logic.
Example Code Snippet:
// Bad Practice
int number = 5; // Set number to 5
number++; // Increment number by 1
// Good Practice
int number = 5; // Starting population
number++; // Move to next generation
Why It Matters
Balance is key. While it’s essential to include comments, they should enhance code readability rather than detract from it. Aim for clarity in your code to minimize the need for comments.
4. Writing Long Methods
Keep It Simple
One common issue developers grapple with is writing lengthy methods that perform multiple tasks. This can complicate debugging and testing.
Example Code Snippet:
// Bad Practice
public void processUserData(String userData) {
// Validate userData
// Parse userData
// Store in database
// Notify user
}
// Good Practice
public void processUserData(String userData) {
if (!isValid(userData)) {
return; // Early exit for invalid data
}
storeInDatabase(parse(userData));
notifyUser();
}
private boolean isValid(String userData) {
// Logic for validation
}
private String parse(String userData) {
// Parsing logic
}
private void storeInDatabase(String data) {
// Database storage logic
}
private void notifyUser() {
// Notification logic
}
Why It Matters
By breaking the process into smaller methods, you enhance readability and maintainability. Each method now has a single responsibility, making it easier to test and debug.
5. Failing to Refactor
The Art of Refactoring
Failing to refactor can lead to technical debt and spaghetti code, wherein parts of your codebase become unnecessarily intertwined and complex over time.
How to Refactor Effectively:
- Identify code that can be improved.
- Make small, incremental changes.
- Test after each significant change.
Example:
// Un-refactored
public void handleEverything(User user) {
validateUser(user);
// Processing logic
// More processing logic...
}
// Refactored
public void handleUser(User user) {
if (isValidUser(user)) {
processUser(user);
}
}
private boolean isValidUser(User user) {
// Validation logic here
}
private void processUser(User user) {
// Processing logic here
}
Why It Matters
Refactoring allows you to improve the structure of existing code without changing its external behavior. This helps in keeping the codebase clean, organized, and maintainable in the long run.
6. Not Utilizing Version Control Properly
A Developer's Best Friend
Version control systems (like Git) allow you to manage changes to your code efficiently. One common mistake is to make extensive changes without committing regularly.
Best Practices:
- Commit often with clear messages.
- Use branches for new features or bug fixes.
- Regularly pull updates from others.
Why It Matters
Proper use of version control helps in keeping a history of changes, which can be invaluable when you need to debug or track issues. For more details on leveraging Git effectively, see this Git tutorial.
7. Ignoring Error Handling
Bumpiness on the Road
Many developers overlook error handling, assuming things will work perfectly. However, unexpected bugs can occur, and proper handling can save your application from crashing.
Example Code Snippet:
// Poor error handling
public void connectToDatabase() {
// Attempt to connect
}
// Improved error handling
public void connectToDatabase() {
try {
// Attempt to connect
} catch (SQLException e) {
System.err.println("Database connection failed: " + e.getMessage());
// Additional recovery or logging
}
}
Why It Matters
Robust error handling enhances the user experience and helps maintain application stability. It creates a safety net for unexpected scenarios.
Bringing It All Together
Navigating the world of Java development can be challenging, especially for newcomers. By avoiding these common tidy codebase mistakes—like poor naming conventions, lack of comments, long methods, and inadequate error handling—you can set yourself up for greater success and collaboration.
Remember, writing clean code is a skill that improves with practice. Make it a habit to review and refactor your code regularly.
For further reading on best coding practices, check out this comprehensive guide.
By making these changes and committing to a tidier codebase, you will enhance both your programming skills and your team's ability to deliver quality software.
Happy coding!
Checkout our other articles