Troubleshooting Automated Integration Test Failures
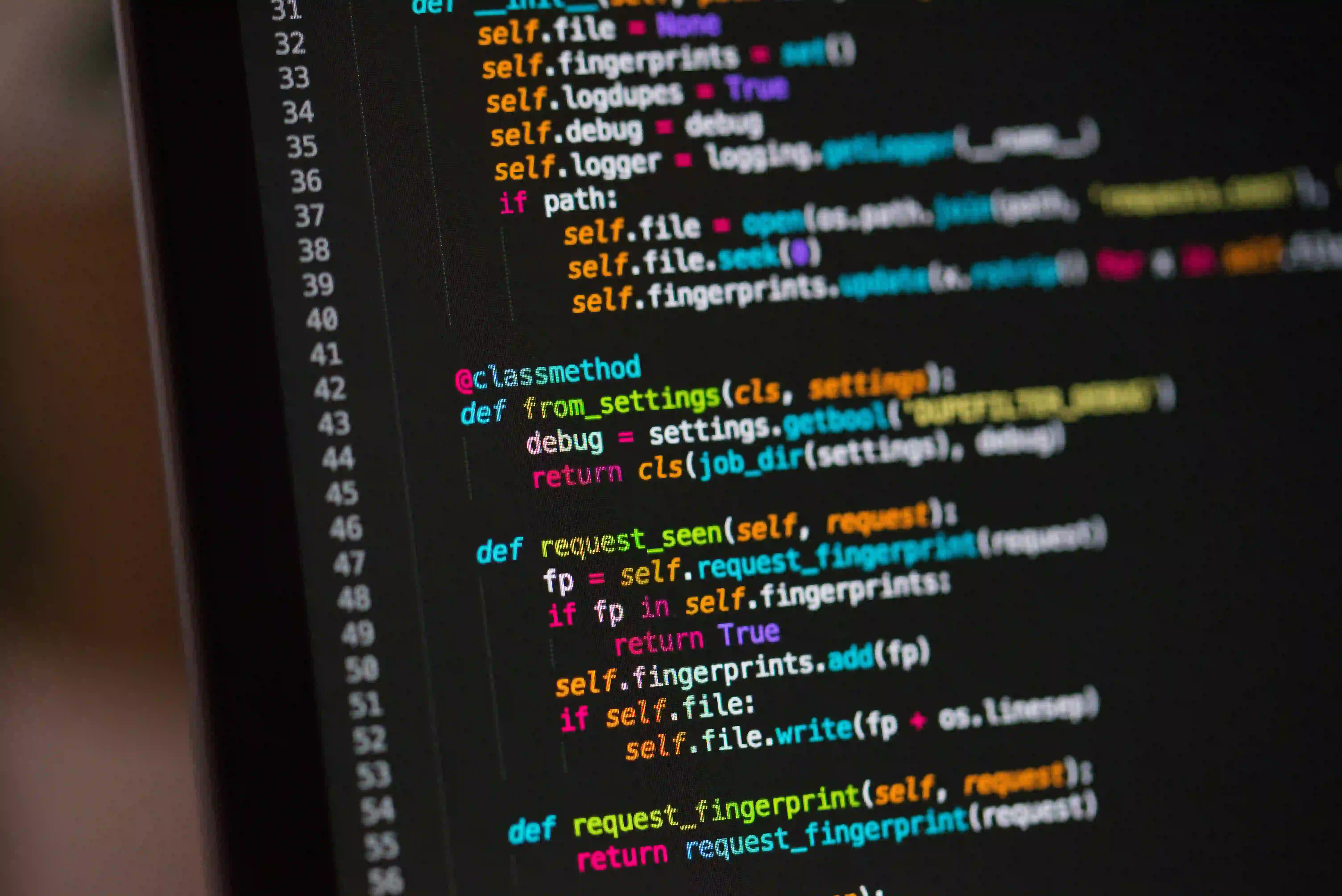
Troubleshooting Automated Integration Test Failures
Automated integration testing is a crucial part of modern software development. It ensures that various components of your application work together as intended. However, integration tests can fail for various reasons, leading to confusion and frustration. In this blog post, we’ll explore common reasons for integration test failures, effective troubleshooting strategies, and best practices to enhance your testing process.
Understanding Integration Tests
Before we dive into troubleshooting, let’s clarify what integration tests are. Unlike unit tests, which focus on individual components, integration tests assess how multiple components of an application interact with each other. This is vital in ensuring that changes in one part of your applicative structure do not adversely affect other components.
Why Integration Tests Matter
Integration tests can identify issues that unit tests may not catch. These issues might arise due to:
- Miscommunication between components
- Dependency issues
- Data format discrepancies
Recognizing problems at this stage can save time, cost, and potential headaches later in the development cycle.
Common Reasons for Integration Test Failures
-
Environment Issues
Integration tests often run in environments different from development or production, leading to discrepancies. Configuration settings or environment variables might not align with what the tests expect.
-
Flaky Tests
Tests that intermittently pass or fail due to timing issues, data race conditions, or external dependencies can cause a lack of confidence in your test suite.
-
Dependency Changes
Changes in external libraries or services can introduce breaking changes that lead to test failures. It's crucial to monitor and manage dependencies carefully.
-
Incorrect Test Data
Using stale or incorrect test data can render tests irrelevant or incorrect. Test data should be representative of real-world use cases.
-
Code Changes
Changes in the codebase, whether intentional or accidental, can break integration tests. Refactoring might also introduce bugs that go unnoticed in unit tests.
Step-by-Step Troubleshooting Guide
Step 1: Identify the Failure
Start by analyzing the output of the test run. Look for error messages and stack traces. They often provide initial clues regarding what went wrong.
@Test
public void testIntegration() {
// This is where we set up our test
IntegrationService service = new IntegrationService();
Throwable exception = assertThrows(SomeExpectedException.class, () -> {
service.performIntegration();
});
assertEquals("Expected exception message", exception.getMessage());
}
In this example, we’re using assertions to validate the expected exception message. The test will fail if the message does not match, allowing us to pinpoint where the problem may originate.
Step 2: Replicate the Issue
Run the test in isolation to ensure that the failure is not related to other tests or external factors. This can also help uncover dependencies that may not be immediately apparent.
Step 3: Check Configuration
Verify that the configuration settings, environment variables, and any external resources are correctly set up. If you're using tools like Docker for your test environments, ensure that your container configurations are accurate and match what's intended.
Step 4: Validate Test Data
Examine your test data to ensure that it is up-to-date and accurately represents the scenarios your integration tests are meant to cover. Test data should be reset between test runs to avoid cross-contamination.
Step 5: Monitor Dependencies
In your build.gradle or pom.xml, ensure that dependencies are correctly defined and have compatible versions. Use tools like Maven Dependency Plugin to analyze existing dependencies.
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>30.1-jre</version>
</dependency>
Ensure that version numbers are locked to prevent disruptive upgrades from breaking your tests unexpectedly.
Step 6: Fix Flaky Tests
If tests are flaky, introduce necessary wait times or implement retries. Look into using more robust synchronization methods when interacting with async behavior. Here’s an example using Java’s CompletableFuture:
CompletableFuture<Response> future = CompletableFuture.supplyAsync(() -> {
try {
// Simulate a long-running task
Thread.sleep(1000);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
return new Response("Success");
});
Response response = future.orTimeout(2, TimeUnit.SECONDS).join();
assertEquals("Success", response.getMessage());
By introducing timeout handling, we can ensure that our application doesn’t hang indefinitely.
Step 7: Review Code Changes
Check if any recently made changes might have inadvertently caused the issues. Look for code that interacts with the integration points of your application.
Best Practices for Preventing Failures
-
Continuous Monitoring: Utilize CI/CD pipelines to run integration tests regularly. This enables early detection of failures.
-
Consistent Test Data: Use tools like DBUnit or automated scripts to ensure test data is consistent and reproducible.
-
Clear Documentation: Maintain thorough documentation of your tests and their dependencies. This aids in understanding what configuration is necessary for successful runs.
-
Use of Mocks and Stubs: Consider using mocks or stubs for external services. While this may not test the real integrations, it allows for controlled testing conditions.
-
Regular Review and Refactoring: Keep your test cases clean and maintainable. Regularly refactor tests to avoid growing complexity over time.
Lessons Learned
Troubleshooting automated integration test failures can be a time-consuming yet crucial task in maintaining a healthy codebase. By understanding potential causes, adhering to structured troubleshooting guidelines, and implementing best practices, your testing process can become significantly more reliable.
Integration tests are not just a safety net; they're a gateway to ensuring that your application remains robust and user-friendly as it scales. Let's invest time in them, so they serve their purpose effectively. Happy coding!
For more insights on testing strategies, check out Testing Strategies for Java Applications and Best Practices for Automated Testing for enhancing your test coverage.