Common Pitfalls When Creating MongoDB Capped Collections in Java
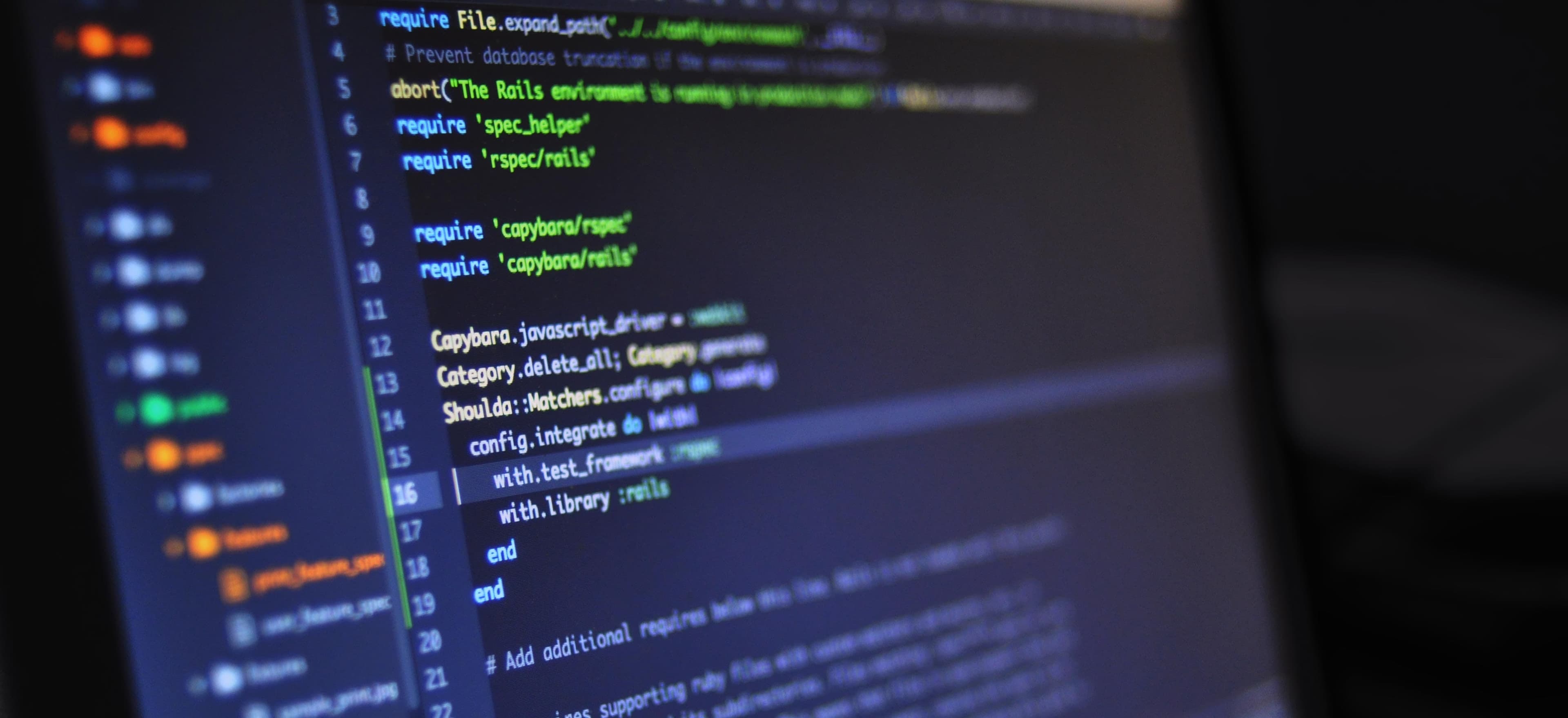
- Published on
Common Pitfalls When Creating MongoDB Capped Collections in Java
MongoDB is a popular NoSQL database known for its flexibility and scalability. One of its unique features is capped collections. These are fixed-size collections that maintain the insertion order and automatically remove the oldest documents when the specified size limit is reached. While capped collections can be beneficial for logging or caching systems, there are several common pitfalls developers encounter, particularly when using Java. This post will explore those pitfalls, provide code snippets to illustrate key points, and suggest best practices for avoiding these issues.
What is a Capped Collection?
Before diving into the pitfalls, let's briefly cover what capped collections are and their use cases. A capped collection has a maximum size limit, either in bytes or document count. When a document is added to a capped collection and the limit has been reached, MongoDB automatically removes the oldest document.
Use cases for capped collections include:
- Logging systems where only the most recent logs are relevant.
- Storing temporary data that doesn't need to persist beyond a certain limit.
- Circular buffers for application results.
To create a capped collection in MongoDB using Java, you can use the createCollection
method from the MongoCollection
class, as shown below:
MongoCollection<Document> collection = database.getCollection("logs");
CreateCollectionOptions options = new CreateCollectionOptions()
.capped(true)
.sizeInBytes(1048576); // 1 MB
database.createCollection("logs", options);
Common Pitfalls
1. Not Understanding Size Limitations
The first pitfall developers often make is misunderstanding how size limitations work with capped collections. It’s not just about the total size; it’s also about the size of individual documents.
Why Does It Matter?
A capped collection might have a total size limit of 1 MB, but if the individual document sizes are large, you might run out of space quickly. In contrast, smaller documents allow for more entries, optimizing both space and performance.
Code Snippet:
Here’s how you might check the size of the documents before insertion:
Document newLog = new Document("message", "This is a log entry.")
.append("timestamp", System.currentTimeMillis());
if (newLog.toJson().getBytes().length < (1048576 - collection.countDocuments())) {
collection.insertOne(newLog);
} else {
// Handle the scenario when the log exceeds the capped collection limit
System.out.println("Cannot insert; will exceed capped collection size.");
}
2. Forgetting About the Default Behavior
When a capped collection reaches its size limit, it automatically removes the oldest documents. For applications that depend on certain data remaining present, this can lead to surprises.
Why Does It Matter?
When designing your application, understand that capped collections do not support document removal by ID or query. You cannot selectively delete documents, which may lead to unexpected data loss.
Code Snippet:
Here’s an example of how you might update your application logic to account for this behavior:
if (collection.countDocuments() < maxDocuments) {
collection.insertOne(newLog);
} else {
// Log already full; consider alternatives
System.out.println("Capped collection is full; cannot add more logs.");
}
3. Misusing Capped Collections with Unique Indexes
Another common issue is trying to enforce unique indexes on capped collections. MongoDB treats capped collections differently and doesn't allow certain types of indexes.
Why Does It Matter?
This restriction can frustrate developers who expect their collections to enforce uniqueness. If your capped collection relies on unique identifiers, be prepared to handle this limitation.
Code Snippet:
To create a capped collection with a unique index (which will fail), you might write:
try {
collection.createIndex(Indexes.ascending("uniqueField"), new IndexOptions().unique(true));
} catch (MongoCommandException e) {
System.out.println("Cannot create unique index on capped collection: " + e.getMessage());
}
4. Ignoring Oplog-like Behavior
Since capped collections maintain insertion order, if your application is not designed to handle the chronological nature of data, you may face challenges.
Why Does It Matter?
If your application relies on the order of operations or timestamps, capped collections can inadvertently create anomalies if not handled properly.
Code Snippet:
Here's a simple way to read the last entries while respecting insertion order:
FindIterable<Document> lastEntries = collection.find()
.sort(Sorts.ascending("timestamp"))
.limit(10);
for (Document doc : lastEntries) {
System.out.println(doc.toJson());
}
5. Failing to Handle Exceptions
In Java, there are numerous exceptions that can arise when interacting with MongoDB. Failing to account for these can lead to application crashes.
Why Does It Matter?
Proper error handling ensures that your application can gracefully recover or notify the user, rather than crashing unexpectedly.
Code Snippet:
Here’s an example of handling MongoWriteException
when inserting logs:
try {
collection.insertOne(newLog);
} catch (MongoWriteException e) {
System.out.println("Write failed: " + e.getMessage());
}
Best Practices
To prevent the pitfalls mentioned above, consider the following best practices:
-
Monitor Collection Sizes: Implement monitoring to keep track of the size and document count in your capped collections.
-
Design for Uniqueness: If unique identifiers are essential, consider alternate data structures like normal collections or composite keys.
-
Implement Error Handling: Use Java's exception handling to catch potential errors during database interactions.
-
Document Dependencies: Clearly document where capped collections are being used to avoid misuse by other developers.
-
Test Extensively: Conduct tests specifically around the behavior of capped collections, focusing on edge cases like size limits and deletion behaviors.
The Last Word
Capped collections in MongoDB can provide significant benefits for specific use cases but come with their own set of challenges, particularly when implemented in Java. Understanding the limitations and common pitfalls will help you leverage this powerful feature effectively.
For more in-depth coverage on MongoDB features and best practices, you can visit the official MongoDB documentation.
By being aware of these pitfalls and implementing the suggested best practices, you can optimize your use of capped collections and avoid potential headaches down the line. Happy coding!