Why Naming Your Thread Pools Can Save Your Code from Chaos
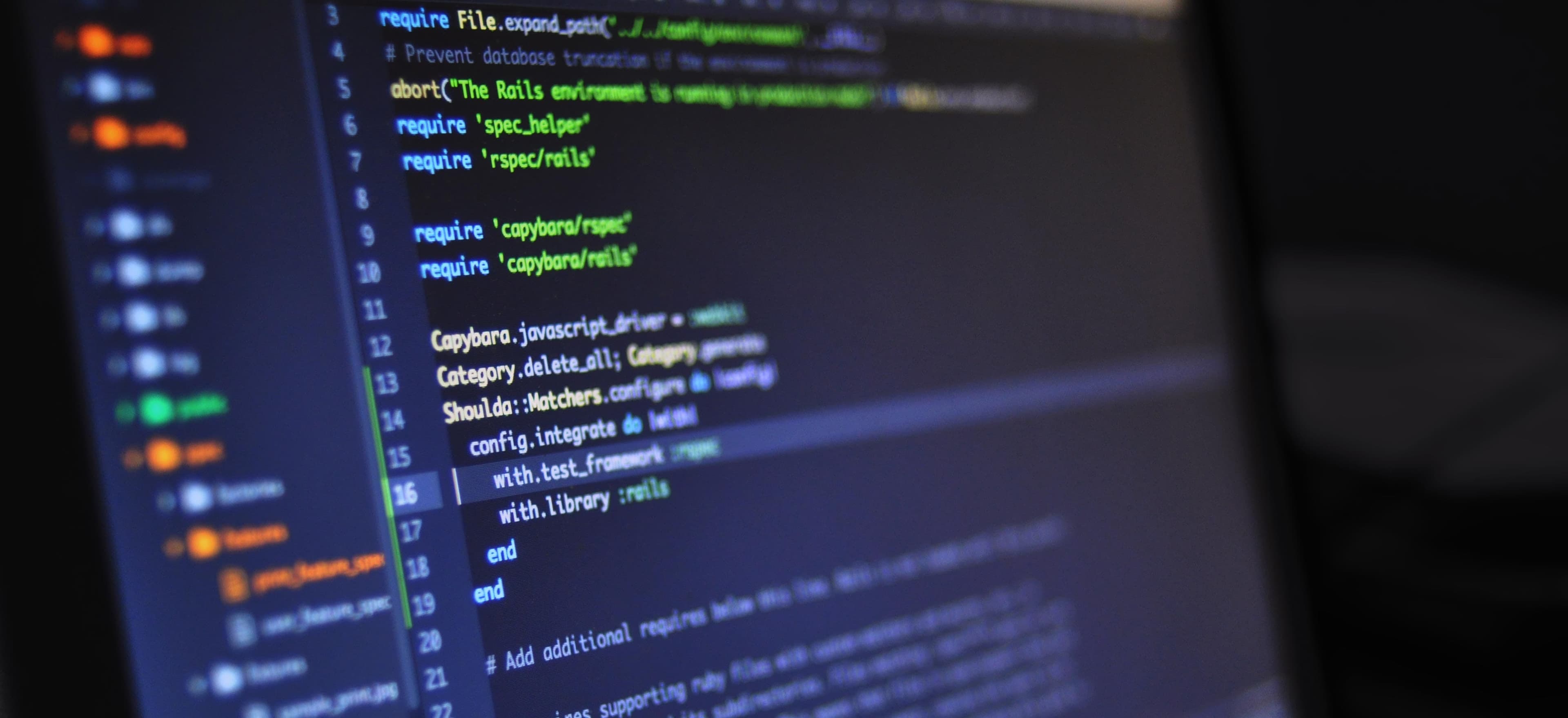
- Published on
Why Naming Your Thread Pools Can Save Your Code from Chaos
In modern software development, especially in Java, creating efficient and manageable applications often requires the use of concurrency through thread pools. However, many developers overlook an essential aspect of thread management: naming your thread pools. This simple practice can lead to clearer code, easier debugging, and better overall application performance. In this post, we will delve into the "why" and "how" of naming thread pools, supported by practical examples.
Understanding Thread Pools
Before discussing naming conventions, let’s briefly review what thread pools are. Simply put, a thread pool is a collection of pre-initialized threads that can be reused to execute tasks.
Advantages of Using Thread Pools
- Resource Management: They help in managing system resources by limiting the number of concurrent threads, preventing resource exhaustion.
- Performance Improvements: Reusing threads can reduce the overhead of thread creation and destruction, enhancing performance.
- Control Over Concurrency: Thread pools allow for fine-tuning of concurrency levels, which can lead to improved application responsiveness.
However, as you scale your application, the complexity can increase rapidly. This is where coherent naming strategies can help.
The Importance of Naming Thread Pools
-
Clarity and Context
When developing complex business logic, having clearly named thread pools can immediately convey the purpose or context of the thread being executed. For example, simply noting that a thread is responsible for "Order Processing" can help both developers and maintainers understand that part of the system at a glance. Contrast this with a generic name like "ThreadPool-1", which provides little to no information about its function.
ExecutorService orderProcessingThreadPool = Executors.newFixedThreadPool(10);
In the above example, naming the thread pool orderProcessingThreadPool
informs anyone reading the code what the pool is used for.
-
Easier Debugging
When an issue arises, being able to quickly identify the relevant thread pool can shave hours off debugging time. If a thread pool for database operations fails and it’s labeled as databaseThreadPool
, you can easily search logs or even stack traces without sifting through irrelevant information.
Consider the following example:
ExecutorService databaseThreadPool = Executors.newCachedThreadPool();
During debugging, you would be able to trace back errors to the specific database thread pool, streamlining the process of investigation.
-
Monitoring and Metrics
Many Java applications run in environments where performance monitoring tools are used. When you name your threads appropriately, it not only allows for easier monitoring but also aids in generating accurate metrics.
For example, if you're using a monitoring tool like Prometheus, clear thread names will allow you to tailor metrics specific to your applications, such as response times or execution counts.
ExecutorService apiCallThreadPool = Executors.newScheduledThreadPool(5);
Using the apiCallThreadPool
name helps identify usage patterns for API-related tasks. This can lead to more informed architectural decisions and performance tuning.
Guidelines for Naming Thread Pools
Establishing a consistent naming convention can be just as valuable as the names themselves. Below are some best practices for naming thread pools in Java:
-
Use Descriptive Names: The name should reflect the purpose of the thread pool clearly and accurately.
-
Include the Type of Work: If the thread pool serves a specific function, include that in the name—like
imageProcessingThreadPool
ornotificationThreadPool
. -
Indicate the Number of Threads: If applicable, include the maximum number of threads in the name, such as
imageProcessingThreadPool-5
. -
Avoid Generic Names: Stay away from names like
threadPool1
ormyThreadPool
. These offer no context. -
Maintain a Consistent Format: Whether you use camelCase, snake_case, or any other style, keep it consistent throughout your codebase.
Examples of Naming Thread Pools
Let's look at several practical examples comparing poor and good naming practices:
Example 1: File Upload Processing
ExecutorService genericPool = Executors.newCachedThreadPool(); // Poor naming
Versus
ExecutorService fileUploadProcessingThreadPool = Executors.newCachedThreadPool(); // Good naming
In this instance, the good naming version immediately informs the reader of the pool's purpose.
Example 2: Background Tasks
ExecutorService bgTaskPool = Executors.newFixedThreadPool(3); // Mediocre
Versus
ExecutorService backgroundDataAnalysisThreadPool = Executors.newFixedThreadPool(3); // Excellent
The second name tells you exactly what kind of background tasks this pool is engaged in, making it much clearer what to expect.
Closing the Chapter
When it comes to developing high-performance, scalable applications in Java, every detail counts—including the lesser-thought-of aspects of naming thread pools. Descriptive names can provide clarity in your code, simplify debugging, and enhance maintenance.
Having a systematic approach to naming your thread pools can save you from future chaos, especially as your codebase scales. Proper naming might seem like a trivial detail, but it can lead to significant efficiencies in understanding and managing code.
For further reading, you can check out the following resources: Java Concurrency in Practice for a deep dive into concurrency models in Java, and Effective Java for best practices in Java programming.
Embrace the habit of naming your thread pools appropriately and watch your code transform from a chaotic jumble into a structured masterpiece.
Checkout our other articles