Navigating Deprecated Features in JDK 9 Enhancements
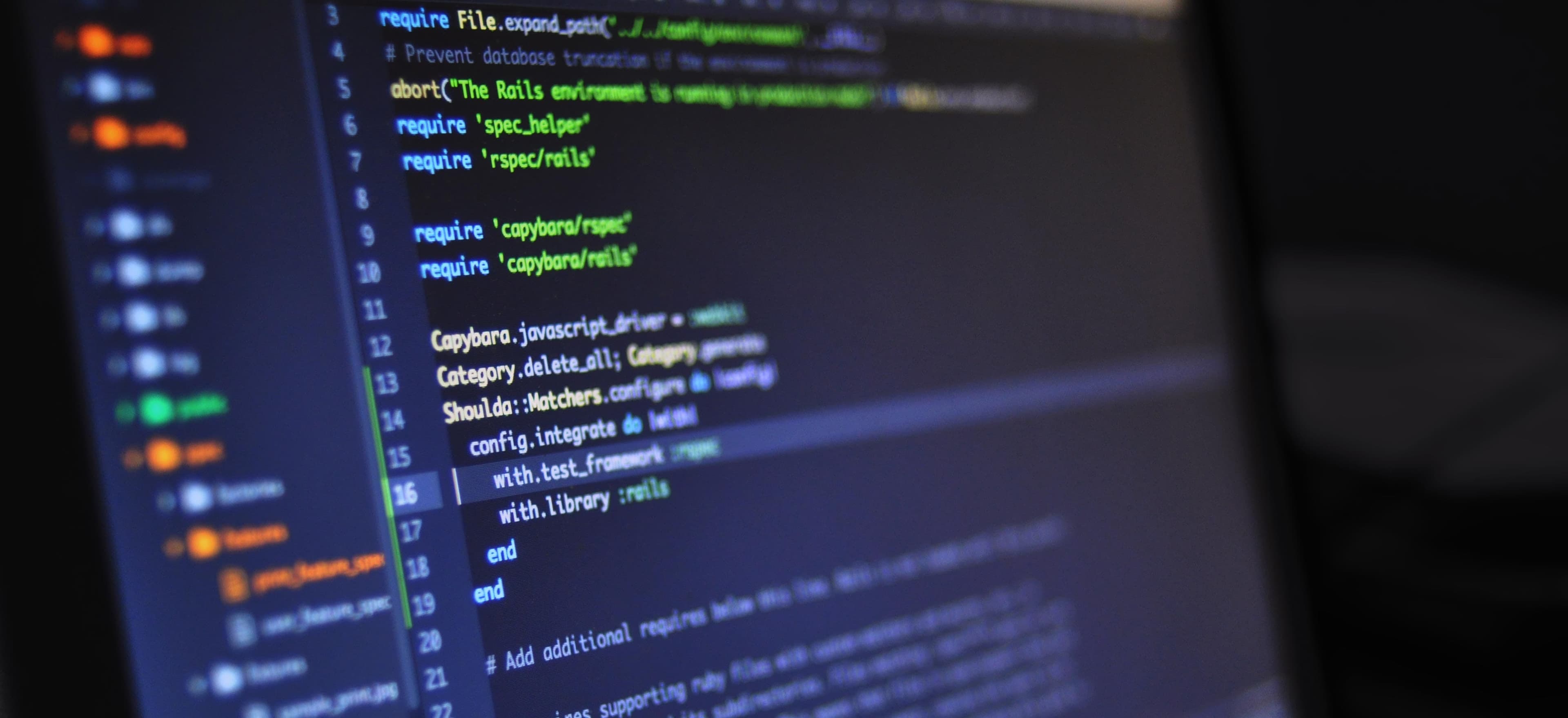
- Published on
Navigating Deprecated Features in JDK 9 Enhancements
Java has long been a staple in the world of programming, celebrated for its versatility and robustness. As we navigate deeper into JDK 9 enhancements, a noteworthy aspect to consider is the deprecation of certain features that can impact your coding journey. Understanding these deprecated features is crucial for ensuring compatibility and harnessing the full potential of the new JDK iterations.
In this blog post, we'll explore the deprecated features in JDK 9, their implications, and how to adapt your code while leveraging the new enhancements. Let’s dive in!
What Does 'Deprecated' Mean?
Before we delve into the specifics of JDK 9, it's essential to clarify what "deprecated" means in the Java world. A deprecated feature is one that is no longer recommended for use and is slated for removal in future releases. However, it may still function currently, albeit with warnings.
Deprecation often arises from several reasons, including:
- Improvements in performance: Newer alternatives may offer better efficiency.
- Code maintainability: Redundant or complex code can be simplified.
- Security: Outdated features might pose security risks.
By recognizing deprecated features early, developers can avoid future issues and ensure their applications remain functional and secure.
Key Deprecated Features in JDK 9
Now that we have a clear understanding of what deprecation implies, let's take a closer look at some significant deprecated features introduced in JDK 9.
1. java.util.Vector
and java.util.Stack
Why It Was Deprecated
While the usability and appeal of java.util.Vector
and java.util.Stack
have been noteworthy through the years, they have largely been supplanted by more modern alternatives like ArrayList
and Deque
. The design of these classes is considered less flexible and more prone to errors in concurrent situations.
Alternatives
The recommendation is to transition to the use of ArrayList
for list manipulation and Deque
for stack behavior.
Here’s a quick example illustrating the transition:
import java.util.ArrayList;
import java.util.Deque;
import java.util.LinkedList;
public class ListStackExample {
public static void main(String[] args) {
// Original Vector usage (deprecated)
// Vector<Integer> vector = new Vector<>();
ArrayList<Integer> list = new ArrayList<>();
list.add(1);
list.add(2);
// Using Deque as a Stack (recommended)
Deque<Integer> stack = new LinkedList<>();
stack.push(1);
stack.push(2);
System.out.println("List: " + list);
System.out.println("Stack: " + stack);
}
}
The above snippet illustrates how to adapt code away from deprecated Vector and Stack towards more preferred alternatives.
2. java.util.legacy
Packages
Why They Were Deprecated
The legacy packages, such as java.util.legacy
, have been deprecated as developers increasingly adopted the Java Collections Framework and other modern libraries. They provide older class implementations that lack some of the newer functionalities and optimizations.
Alternatives
Moving to the java.util
or java.util.concurrent
packages is advised. This switch offers access to enhanced collections, better performance, and new features.
Example code demonstrating the use of modern collections:
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class LegacyPackageExample {
public static void main(String[] args) {
// Original use of legacy packages
// Vector<String> legacyVector = new Vector<>();
List<String> list = new ArrayList<>();
list.add("Example");
// Original use of HashTable (deprecated)
// Hashtable<String, String> legacyMap = new Hashtable<>();
Map<String, String> map = new HashMap<>();
map.put("key", "value");
System.out.println("List: " + list);
System.out.println("Map: " + map);
}
}
This snippet showcases how to use modern alternatives over deprecated legacy features.
3. java.security.acl
Package
Why It Was Deprecated
The java.security.acl
package has been earmarked for deprecation due to its convoluted model for permissions. In modern Java development practices, a more straightforward and effective approach has emerged in the form of the Java Security framework.
Alternatives
Java's modern security package provides improved methods for handling permissions without the need for the acl
package.
Handling Warnings for Deprecated Features
If you still need to use a deprecated feature, you might see warnings during compilation. To manage these warnings effectively, you can suppress them using the @SuppressWarnings
annotation as shown below:
@SuppressWarnings("deprecation")
public void legacyMethod() {
// Call to a deprecated method
deprecatedMethod();
}
public void deprecatedMethod() {
// This is a deprecated method.
}
Best Practices to Follow
-
Stay Informed: Always keep an eye on the official JDK documentation for updates on deprecated features.
-
Test Your Code: Before transitioning to newer Java versions, test your application thoroughly. Pay close attention to deprecated features.
-
Leverage IDE Tools: Use Integrated Development Environments (IDEs) that highlight deprecated APIs and suggest alternatives.
-
Continuous Refactoring: Regularly revisit your codebase. Refactor as necessary to align with current best practices.
To Wrap Things Up
Navigating the deprecation of features in JDK 9 may seem daunting, but it is an essential part of modernizing your Java applications. By understanding the rationale behind deprecation and the available alternatives, developers can ensure that their code remains efficient and secure.
Transitioning away from deprecated features not only improves code maintainability but also embraces all that the new enhancements have to offer. By following the best practices outlined in this post, you can safeguard your applications against future compatibility issues.
For more information on Java best practices, check out Oracle's Java Documentation.
Happy coding!