Mastering Android: Avoiding NetworkOnMainThread Exception
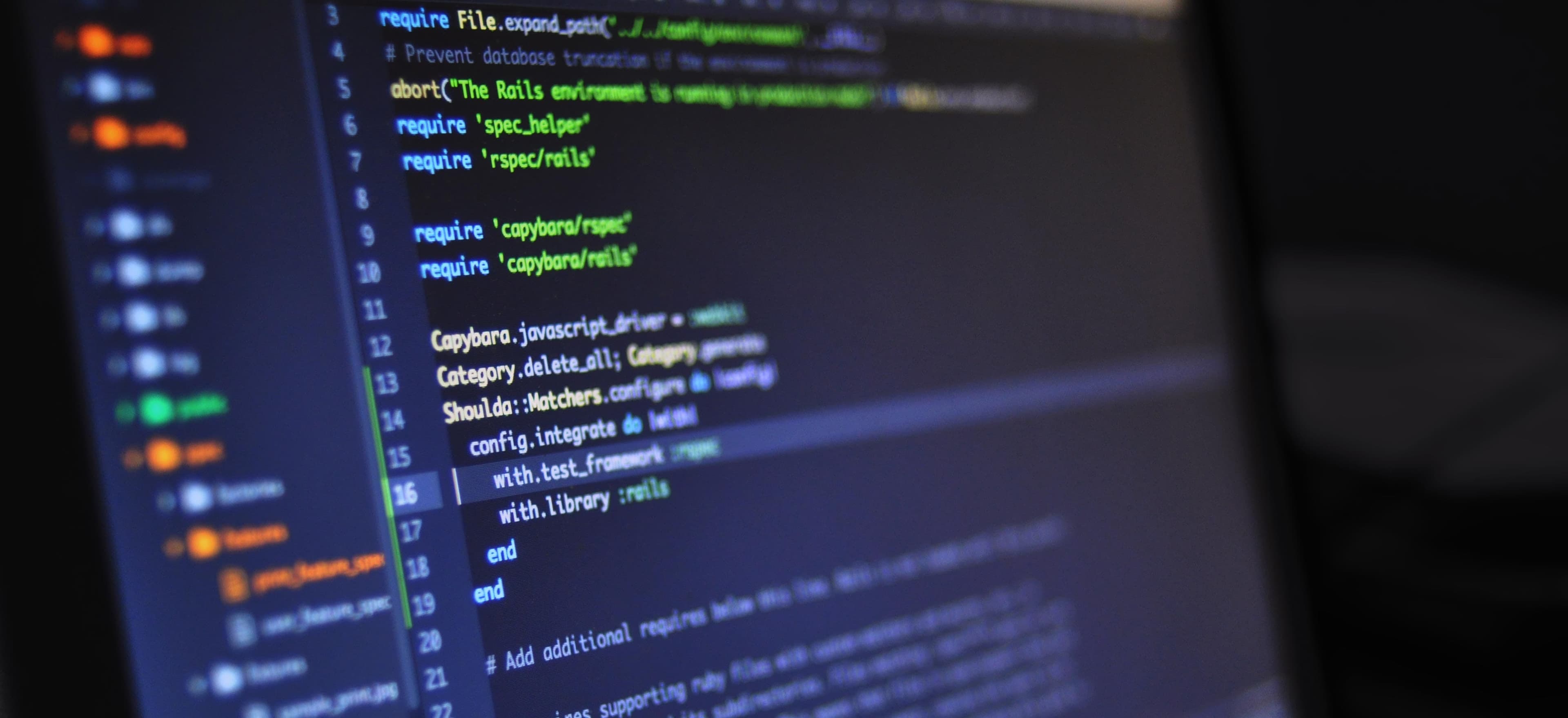
- Published on
Mastering Android: Avoiding NetworkOnMainThread Exception
When developing Android applications, one essential principle every developer must embrace is the concept of threading. The Android operating system has a strict rule: long-running operations, especially network calls, should never occur on the main (UI) thread. Doing so leads to a NetworkOnMainThreadException
. In this blog post, we will explore the reasons behind this exception, pitfalls to avoid, and best practices for performing network operations effectively.
Understanding NetworkOnMainThreadException
The NetworkOnMainThreadException
is thrown when a network operation is executed on the main thread. The main thread is responsible for updating the user interface and processing user interactions. If you block this thread with a network call, your application becomes unresponsive, leading to a poor user experience.
Why Do You Get This Exception?
- Responsiveness: Users expect apps to be responsive. A blocking network call can freeze the UI, leading to a frustrating experience.
- Performance: Android frameworks are designed to provide smooth interaction. Heavy operations on the UI thread can degrade device performance.
- Best Practices: Following Android’s guidelines ensures that your code is efficient and robust.
The exception is a built-in mechanism to enforce these best practices.
The Right Approach: Using Async Tasks
The primary way to avoid a NetworkOnMainThreadException
is to move your network calls off the main thread. One of the simplest ways to do this is by using an AsyncTask
. However, note that AsyncTask
has been deprecated in recent Android versions. You should consider using other methods like Kotlin coroutines or the Android Architecture Components.
Example Using AsyncTask
Here’s a basic illustration of how to use AsyncTask
to perform a network operation:
import android.os.AsyncTask;
import java.net.HttpURLConnection;
import java.net.URL;
public class MyNetworkTask extends AsyncTask<String, Void, String> {
@Override
protected String doInBackground(String... params) {
String response = "";
try {
URL url = new URL(params[0]);
HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection();
try {
// Read the response from the input stream
response = readStream(urlConnection.getInputStream());
} finally {
urlConnection.disconnect();
}
} catch (Exception e) {
e.printStackTrace();
}
return response;
}
@Override
protected void onPostExecute(String result) {
// Update UI with the result
super.onPostExecute(result);
}
private String readStream(InputStream inputStream) {
// Implement your logic to read from the InputStream
}
}
Why Use AsyncTask?
AsyncTask
allows you to perform background operations and publish results on the UI thread without needing to manipulate threads and handlers directly. The doInBackground()
method executes on a background thread, while the onPostExecute()
method runs on the main thread, facilitating easy UI updates.
Transitioning from AsyncTask
While AsyncTask
is a straightforward solution, it can cause memory leaks if not handled correctly. As it is deprecated, ensure to adapt your codebase to more modern approaches, such as Kotlin coroutines, which we will explore now.
Mastering Kotlin Coroutines
Kotlin coroutines provide a more powerful and flexible approach to handling asynchronous operations in a structured way. They are lightweight, making it easier to manage tasks without risking memory leaks or complications associated with legacy threading models.
Setting Up Coroutines in Your Project
To use Kotlin coroutines in your Android project, ensure you have the following dependencies in your build.gradle file:
implementation 'org.jetbrains.kotlinx:kotlinx-coroutines-core:1.5.2'
implementation 'org.jetbrains.kotlinx:kotlinx-coroutines-android:1.5.2'
Example Using Coroutines
Here’s how to execute a network call using Kotlin coroutines:
import kotlinx.coroutines.CoroutineScope
import kotlinx.coroutines.Dispatchers
import kotlinx.coroutines.launch
import kotlinx.coroutines.withContext
import java.net.HttpURLConnection
import java.net.URL
fun fetchNetworkData(url: String) {
CoroutineScope(Dispatchers.IO).launch {
val response = makeNetworkCall(url)
withContext(Dispatchers.Main) {
// Update UI with the response
}
}
}
suspend fun makeNetworkCall(urlString: String): String {
var response = ""
val url = URL(urlString)
(url.openConnection() as HttpURLConnection).apply {
requestMethod = "GET"
response = inputStream.bufferedReader().readText()
disconnect()
}
return response
}
Why Use Coroutines?
Coroutines allow you to write asynchronous code in a sequential manner, which makes it cleaner and easier to read. They are also highly efficient and do not block threads, allowing you to manage complex flows of data effortlessly.
Recommendations for Best Practices
-
Always Use Background Threads: Avoid any network calls in your UI thread. Use coroutines, RxJava, or other concurrency tools.
-
Error Handling: Implement robust error handling to manage timeouts, connectivity issues, and other exceptions. For example:
try { val response = makeNetworkCall(url) } catch (e: Exception) { // Handle the exception accordingly }
-
Cancellation of Tasks: Design your application to handle cancellation gracefully. When users navigate away from a screen, ensure incomplete tasks are canceled to avoid unnecessary computation.
-
Testing Asynchronous Code: Use testing libraries designed for coroutines, such as
kotlinx-coroutines-test
, to simplify unit testing. -
Leverage ViewModel: When using Jetpack components, always manage your network requests within a
ViewModel
to ensure proper lifecycle handling.
In Conclusion, Here is What Matters
Avoiding NetworkOnMainThreadException
is crucial for every Android developer. Understanding the implications of background vs. UI thread operations is the first step in proficient Android programming. By using methods like AsyncTask
(legacy) or Kotlin coroutines (modern), you can ensure your app remains responsive and performant.
As you continue your Android development journey, mastering asynchronous programming will significantly enhance your app's structure and user experience. To dive deeper into coroutines, refer to the Kotlin official documentation on coroutines.
Additional Resources
By keeping these practices in mind, you'll not only avoid frustrating exceptions but also elevate your skills as an Android developer. Happy coding!