Mastering Thread.sleep in Selenium: Avoiding Common Pitfalls
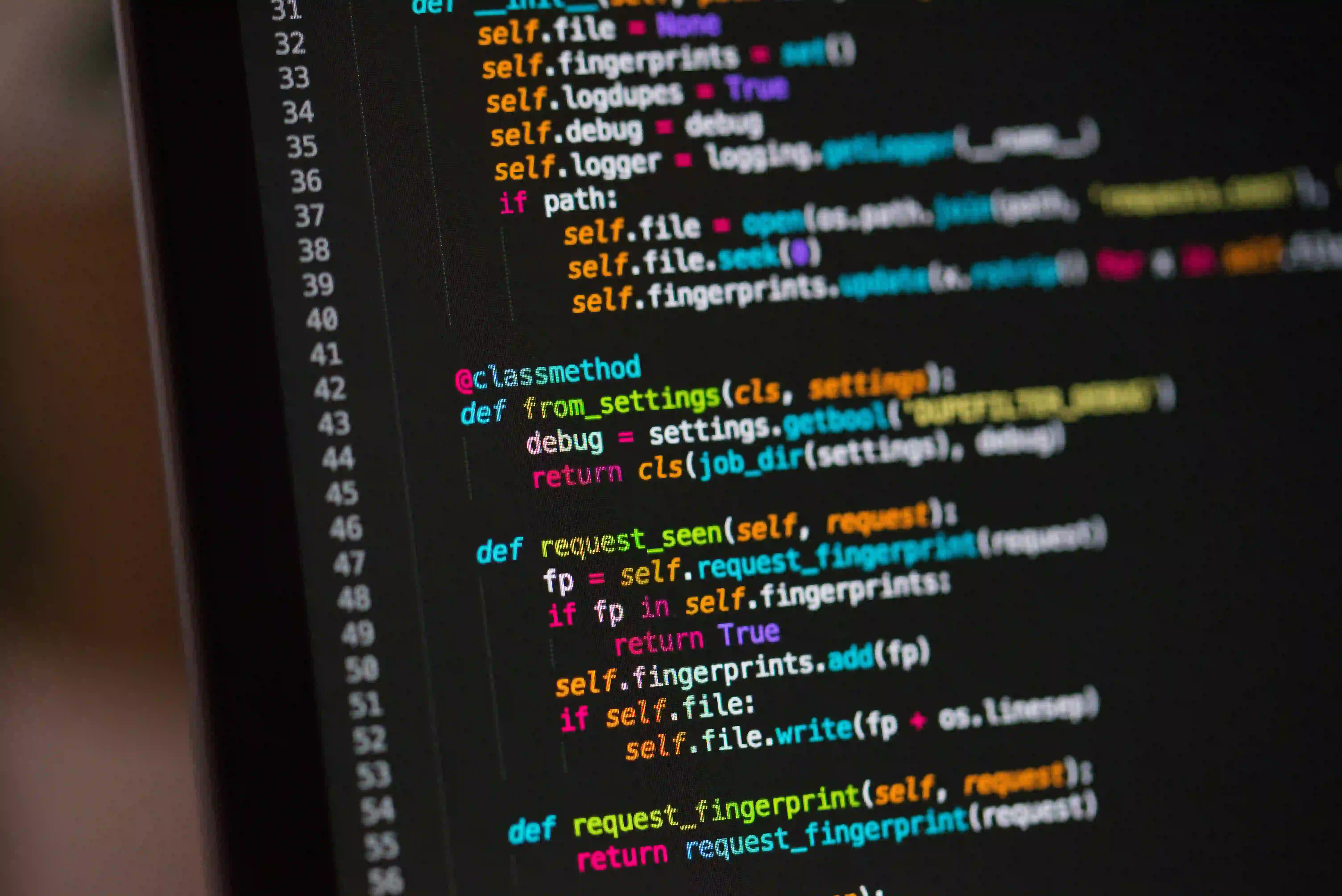
Mastering Thread.sleep in Selenium: Avoiding Common Pitfalls
When it comes to automated testing using Selenium, understanding how to manage time is critical. One common method is using Thread.sleep()
. While it might seem like a simple solution, it can lead to unexpected issues if used improperly. In this article, we will explore Thread.sleep()
, discuss its pros and cons, and present best practices to avoid common pitfalls.
What is Thread.sleep() in Selenium?
Thread.sleep()
is a method in Java that tells the executing thread to pause for a specified amount of time. In Selenium, it's often used to give web elements time to load or become interactive.
Here is a brief example:
// Pausing the execution for 5 seconds
try {
Thread.sleep(5000); // Time is in milliseconds
} catch (InterruptedException e) {
e.printStackTrace();
}
Why use Thread.sleep()?
Using Thread.sleep()
might be tempting because it’s easy to implement. It's straightforward and doesn't involve complex logic, making it accessible even for those new to Selenium.
The Drawbacks of Thread.sleep()
Despite its simplicity, this method can introduce several problems:
-
Unnecessary Waiting: If you set a static wait time, your tests may wait even if the element is already loaded, leading to longer execution times.
-
Concurrency Issues: In a multi-threaded environment, a sudden
sleep()
can block threads that might need to execute, affecting performance. -
Not Dynamic: Different pages may load at different speeds. A fixed sleep time won't accommodate varying load times, which can lead to test failures.
-
Hard to Debug: When tests fail due to timing issues, it can be difficult to identify if the failure was due to a broken test or an incorrect wait time.
Best Practices: Avoid Thread.sleep()
Given the disadvantages, it's generally advisable to avoid Thread.sleep()
. Instead, consider these alternatives:
1. Implicit Waits
Implicit waits tell Selenium to poll the DOM for a certain amount of time when trying to find an element. Here's how to set it up:
// Set implicit wait
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
Why Use Implicit Waits?
- They dynamically wait for an element to appear, making your tests more resilient to load times.
- They apply to all elements in the session, simplifying the code.
2. Explicit Waits
Explicit waits allow you to wait for a specific condition to occur before proceeding. For example, you might wait for an element to be clickable:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.elementToBeClickable(By.id("submit")));
Why Use Explicit Waits?
- They are specific to particular elements or conditions, making them more flexible.
- You can wait for multiple conditions, such as visibility, clickability, etc.
Example: A Comparative Approach
Let’s compare Thread.sleep()
with using explicit waits in a practical Selenium test scenario.
Using Thread.sleep()
driver.get("http://example.com/login");
Thread.sleep(5000); // Poor practice
driver.findElement(By.id("username")).sendKeys("testuser");
Using Explicit Wait
driver.get("http://example.com/login");
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement usernameField = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("username")));
usernameField.sendKeys("testuser");
This code illustrates how explicit waits can improve test accuracy and performance.
When to Use Thread.sleep()
There are rare use cases where Thread.sleep()
might be justified. For example:
- Debugging: If you are troubleshooting flaky tests and need to pause execution to observe behavior.
- Synchronization Issues: In environments where you can’t control element timing but are forced to wait.
However, these scenarios are exceptions rather than the rule.
Combining Wait Types
Sometimes you might want to mix implicit and explicit waits for improved control, but this should be approached cautiously. Here’s how:
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("message")));
Key Takeaways
- Avoid relying on Thread.sleep(): This method can lead to unnecessary wait times and flaky tests.
- Leverage implicit and explicit waits: These are built-in tools that make element interactions more predictable.
- Prioritize clarity and maintainability: Your tests should be straightforward and robust.
Bringing It All Together
While Thread.sleep()
may provide an easy workaround for timing issues in Selenium, its use can lead to a variety of problems. By exploring implicit and explicit waits, you can create tests that are not only faster but also more reliable and easier to maintain.
For a deeper understanding of WebDriver waits, you can check Selenium Official Documentation or read about test design strategies on Software Testing Help.
By adopting these best practices, you will greatly enrich your testing framework and avoid common pitfalls that come with improper wait handling. Happy testing!