Tackling Latency Issues in Real-Time Updates with Storm
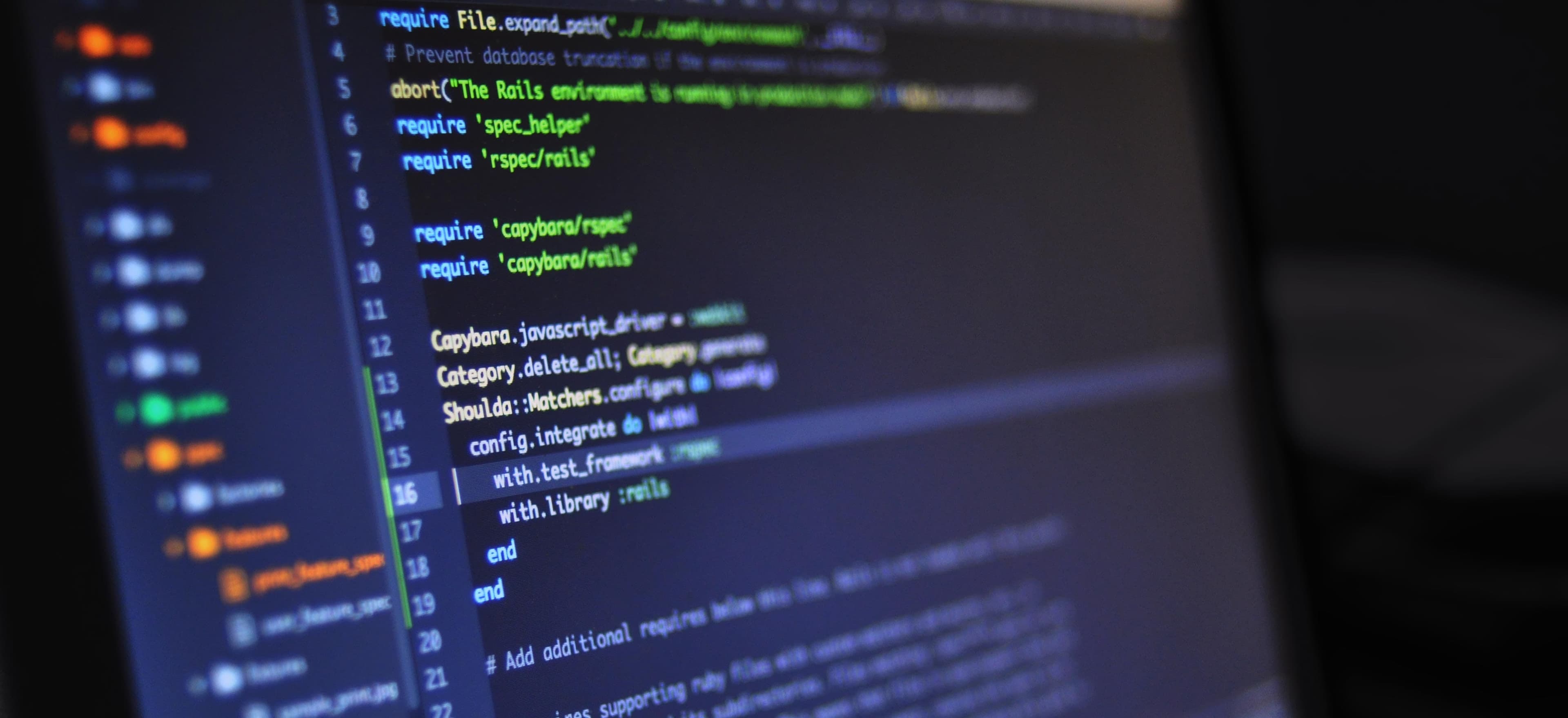
- Published on
Tackling Latency Issues in Real-Time Updates with Apache Storm
In today's fast-paced digital landscape, real-time data processing has become a critical necessity for businesses looking to stay ahead of the competition. Apache Storm, an open-source distributed real-time computation system, is a powerful tool for building complex data pipelines that can process vast amounts of data efficiently. However, latency issues can arise when implementing real-time updates. In this blog post, we'll dive deep into how to tackle these latency challenges with Apache Storm, providing code snippets and explanations to illuminate the solutions.
Understanding Latency in Real-Time Data Processing
Latency refers to the delay before data processing begins and affects the overall performance of your application. In the context of Apache Storm, several factors can contribute to increased latency:
- Network Delays: Communication between nodes can introduce significant delays.
- Processing Bottlenecks: Slow processing components can hold up the entire pipeline.
- Backpressure: When data production exceeds the rate at which it can be processed, it causes an accumulation of unprocessed data.
To understand how to mitigate these issues, let’s explore common strategies to optimize Apache Storm and ultimately reduce latency.
Optimizing Apache Storm for Low Latency
1. Topology Design
The way you design your topology can greatly influence latency. A topology in Storm is a graph of computation, where you have spouts (data sources) and bolts (processing units). Keeping a minimal number of processing steps can reduce latency.
Here's an example of a simple topology setup in Java:
import org.apache.storm.Config;
import org.apache.storm.LocalCluster;
import org.apache.storm.StormSubmitter;
import org.apache.storm.topology.TopologyBuilder;
public class SimpleTopology {
public static void main(String[] args) throws Exception {
TopologyBuilder builder = new TopologyBuilder();
// Define your spout
builder.setSpout("my-spout", new MySpout(), 1);
// Define your bolt
builder.setBolt("my-bolt", new MyBolt(), 1)
.shuffleGrouping("my-spout");
// Configuration for topology
Config conf = new Config();
conf.setDebug(true);
// Submit topology
if (args != null && args.length > 0) {
StormSubmitter.submitTopology(args[0], conf, builder.createTopology());
} else {
LocalCluster cluster = new LocalCluster();
cluster.submitTopology("simple-topology", conf, builder.createTopology());
Thread.sleep(10000);
cluster.shutdown();
}
}
}
Why Is This Important?
The setup above adheres to a straightforward design. By limiting the number of bolts in your topology and having a single spout and bolt, you reduce passing delays between components which is pivotal for minimizing latency.
2. Parallelism Tuning
Adjusting the degree of parallelism for your bolts and spouts is crucial for maximizing throughput. Each bolt can process data in parallel, thus spreading the workload.
builder.setBolt("my-bolt", new MyBolt(), 4) // Adjusted for higher parallelism
.shuffleGrouping("my-spout");
Why Parallelism Matters
Increasing the parallelism of your bolts allows Storm to handle more tuples per second, reducing the risk of backpressure and processing delays. However, finding the right balance is vital as too much parallelism can lead to excessive context switching and resource contention.
3. Use of State Management
Maintaining application state is sometimes necessary, but it can introduce latency. Use frameworks like Apache Kafka or Redis to store state externally, which can reduce overhead in the Storm framework.
import org.apache.storm.task.OutputCollector;
public class MyBolt extends BaseRichBolt {
private OutputCollector collector;
@Override
public void prepare(Map conf, TopologyContext context, OutputCollector collector) {
this.collector = collector;
// Optionally connect to an external state management system here
}
@Override
public void execute(Tuple tuple) {
// Process tuple and possibly store state externally
collector.ack(tuple);
}
}
Why Use External State?
External state management offloads state responsibility from your topology, enabling faster processing cycles and reducing the risk of slowdowns.
Monitoring for Latency Issues
Using monitoring tools to gain insights into your topology’s performance is paramount. Popular tools such as:
- Apache Storm UI: Offers a real-time view of your topology and its performance.
- Prometheus and Grafana: Perfect for custom metrics collection.
Why Monitoring Is Key
By keeping track of latency metrics, you can quickly identify bottlenecks and optimize specific parts of your Storm topology, improving your system’s responsiveness.
Handling Backpressure
Backpressure occurs when a bolt is overwhelmed with data. To handle this, Storm allows you to enable backpressure by setting the topology.backpressure.enabled
configuration to true.
conf.setBoolean(Config.TOPOLOGY_BACKPRESSURE_ENABLED, true);
Why Backpressure Handling Is Important
When backpressure is enabled, Storm automatically signals spouts to slow down and enables a smoother flow of data processing.
Final Thoughts
By focusing on efficient topology design, tweaking parallelism, implementing external state management, and utilizing effective monitoring tools, developers can significantly mitigate latency issues in real-time data processing with Apache Storm.
Further Learning Resources
- Apache Storm Documentation
- Prometheus Monitoring
- Click here to explore state management solutions like Redis
In summary, real-time data processing demands optimizations and constant monitoring to achieve low latency. With the right strategies in place, you can enhance your application's performance and deliver timely, relevant data to your users.
Let me know in the comments if you have any questions, or share your experiences in dealing with latency in Apache Storm!