Understanding Hibernate's Read-Only Cache Concurrency Confusion
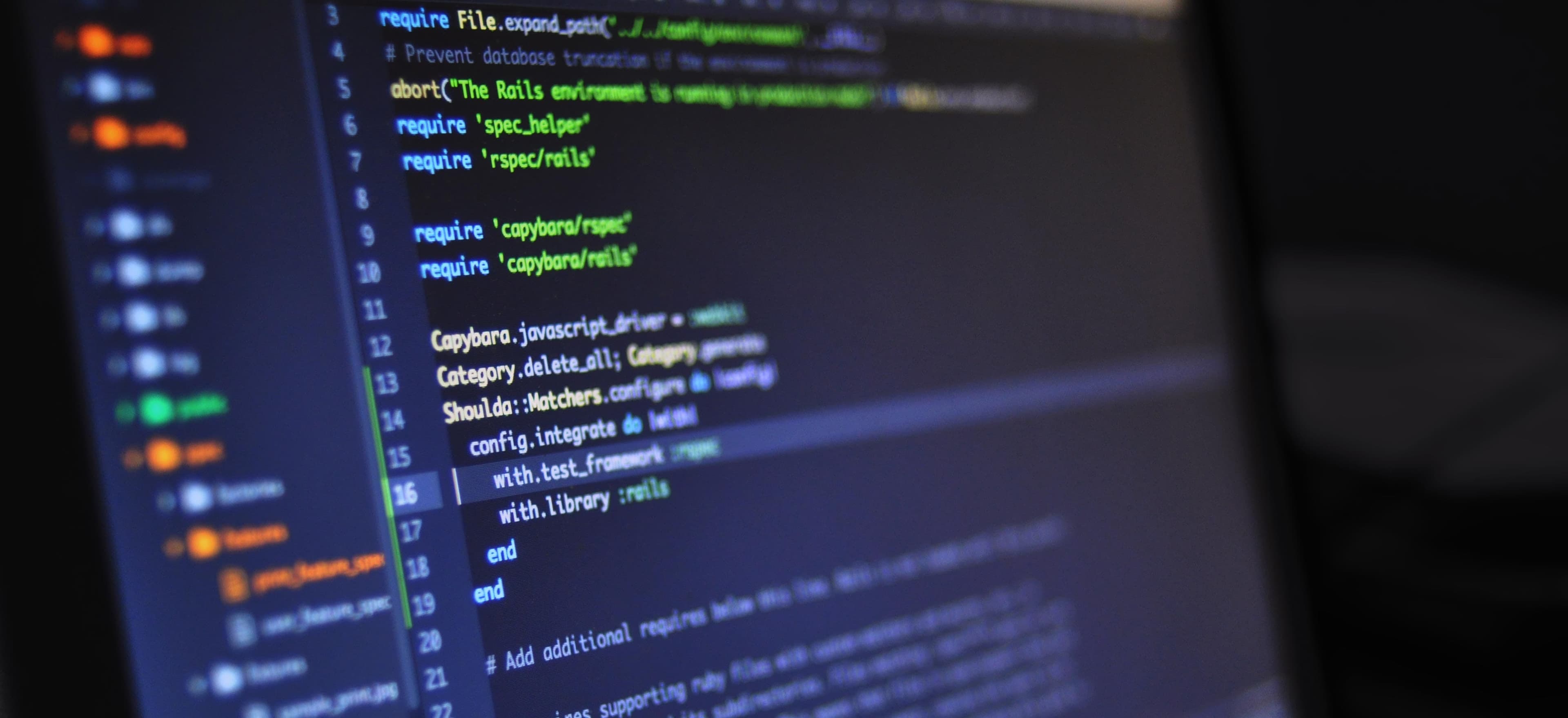
- Published on
Understanding Hibernate's Read-Only Cache Concurrency Confusion
The world of data persistence is complex, especially when it comes to managing cache concurrency in frameworks like Hibernate. When building applications, developers often grapple with the balance between performance and data consistency. This is where understanding Hibernate's read-only cache becomes crucial. In this blog post, we will dissect Hibernate's read-only cache and clarify any confusion surrounding its concurrency model.
What is Hibernate?
Hibernate is a popular Object-Relational Mapping (ORM) framework for Java. It simplifies database interactions by allowing developers to work with objects instead of rows and columns. By encapsulating the database access logic, Hibernate enhances productivity and maintains cleaner code.
The Cache System in Hibernate
Hibernate employs a multi-level caching strategy to optimize performance. The cache is divided into two main levels:
-
First-Level Cache: This cache is tied to the session and is always active. Any data fetched during that session remains available until the session is closed or cleared.
-
Second-Level Cache: This cache spans across sessions. It's shared and can be used in multiple transactions. Hibernate's second-level cache can be configured to support various caching strategies, including read-only.
Benefits of Using Cache
Caching leads to:
- Improved Performance: By storing frequently accessed data, cache reduces database hits.
- Reduced Latency: As data retrieval becomes faster, users experience less waiting time.
Read-Only Cache in Hibernate
A read-only cache is designed to store data that doesn’t change across transactions. This is ideal for static data or configurations. When working with a read-only cache, the goal is to maximize read performance while maintaining the integrity of the data.
Why Use a Read-Only Cache?
Using a read-only cache offers several benefits:
- No Overhead for Locking: Since data cannot change, there's no need for overhead related to locking mechanisms.
- Efficiency in Memory Usage: The read-only cache can compress data, further enhancing efficiency.
- Faster Reads: For applications focused on reading data, a read-only cache can dramatically improve performance.
Configuration Example
To configure a read-only cache in Hibernate, you can annotate your entity class with @Cache
and specify the cache type. Below is an example:
import org.hibernate.annotations.Cache;
import org.hibernate.annotations.CacheConcurrencyStrategy;
@Entity
@Table(name = "BOOK")
@Cacheable
@Cache(usage = CacheConcurrencyStrategy.READ_ONLY)
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "title")
private String title;
// Getters and Setters
}
In this example:
- We annotate the class
Book
with@Cache
and setusage
toCacheConcurrencyStrategy.READ_ONLY
. This informs Hibernate to treat all instances ofBook
as read-only, improving performance during read operations.
Concurrency Issues in the Read-Only Cache
Despite its advantages, confusion often arises when dealing with concurrency in Hibernate's read-only cache. Let's explore some common concerns and potential pitfalls.
Concern #1: Stale Data
One of the primary issues with using a read-only cache is the potential for stale data. When an application retrieves data that has been cached, there is no guarantee that it reflects the most current state in the database. This can lead to misleading data being presented to the user.
Example:
Imagine an e-commerce platform where product prices change frequently. If you cache the product data as read-only, and the price is updated in the database, the cached data may still return the old price until the cache is refreshed.
Mitigating Stale Data
To mitigate stale data concerns, you can consider the following approaches:
-
Cache Expiration: Set a time-to-live (TTL) on the cache. By doing so, the data will be invalidated after a certain period.
@Cache(usage = CacheConcurrencyStrategy.READ_ONLY, region="bookCache") @CacheConcurrencyStrategy.READ_WRITE @Cacheable(value = "bookCache", key = "#id", unless = "#result == null", sync = true) public Book findBookById(Long id) { return entityManager.find(Book.class, id); }
-
Manual Cache Eviction: When you know that a value in the database has changed, manually evict the cached entry.
// Evict the cache for the specific entity sessionFactory.getCache().evictEntityRegion(Book.class);
Concern #2: Performance Bottlenecks
When using a read-only cache, developers often assume they will achieve a performance boost merely by adding it. However, if not implemented wisely, the cache can become a performance bottleneck. For example, if the cache contains a large amount of data but is rarely accessed, the unnecessary memory usage can slow down your application.
Avoiding Performance Bottlenecks
-
Cache Size Management: Keep track of the objects stored in the cache. Ensure you are only caching what is necessary to avoid memory overflow. Use region settings in your cache provider to limit the cache's size.
-
Profiling and Monitoring: Regularly profile your application to identify any bottlenecks caused by the caching mechanism. Tools like YourKit or VisualVM can help measure the application's performance.
Best Practices for Read-Only Caching
-
Understand Your Data's Nature: Before opting for a read-only cache, ensure that the data being cached is indeed read-only.
-
Use Appropriate Keywords: Clearly mark your entities as cacheable and specify how they should be cached.
-
Monitor and Tune: Use monitoring tools to observe cache performance and tweak parameters as needed.
-
Combine Caching Strategies: Mix read-only and read-write caching when dealing with partially mutable data to optimize performance while maintaining data integrity.
-
Test Thoroughly: Always run tests in a staging environment before moving to production to see the effects of your caching configuration.
Bringing It All Together
Hibernate's read-only cache can significantly enhance application performance. However, it comes with its own set of complexities, especially concerning concurrency. By understanding these aspects, developers can better architect their applications to avoid pitfalls like stale data and performance bottlenecks.
Ultimately, while Hibernate automates much of the database interaction, caching remains a nuanced subject that requires careful consideration and understanding. Striking a balance between cache utilization and data consistency is key to building robust applications.
For further reading, check out the Hibernate documentation for deeper insights into caching strategies and best practices.
Happy coding, and may your caching efforts lead to smoother and more efficient applications!