Common Pitfalls of Overusing @Autowired in Spring Framework
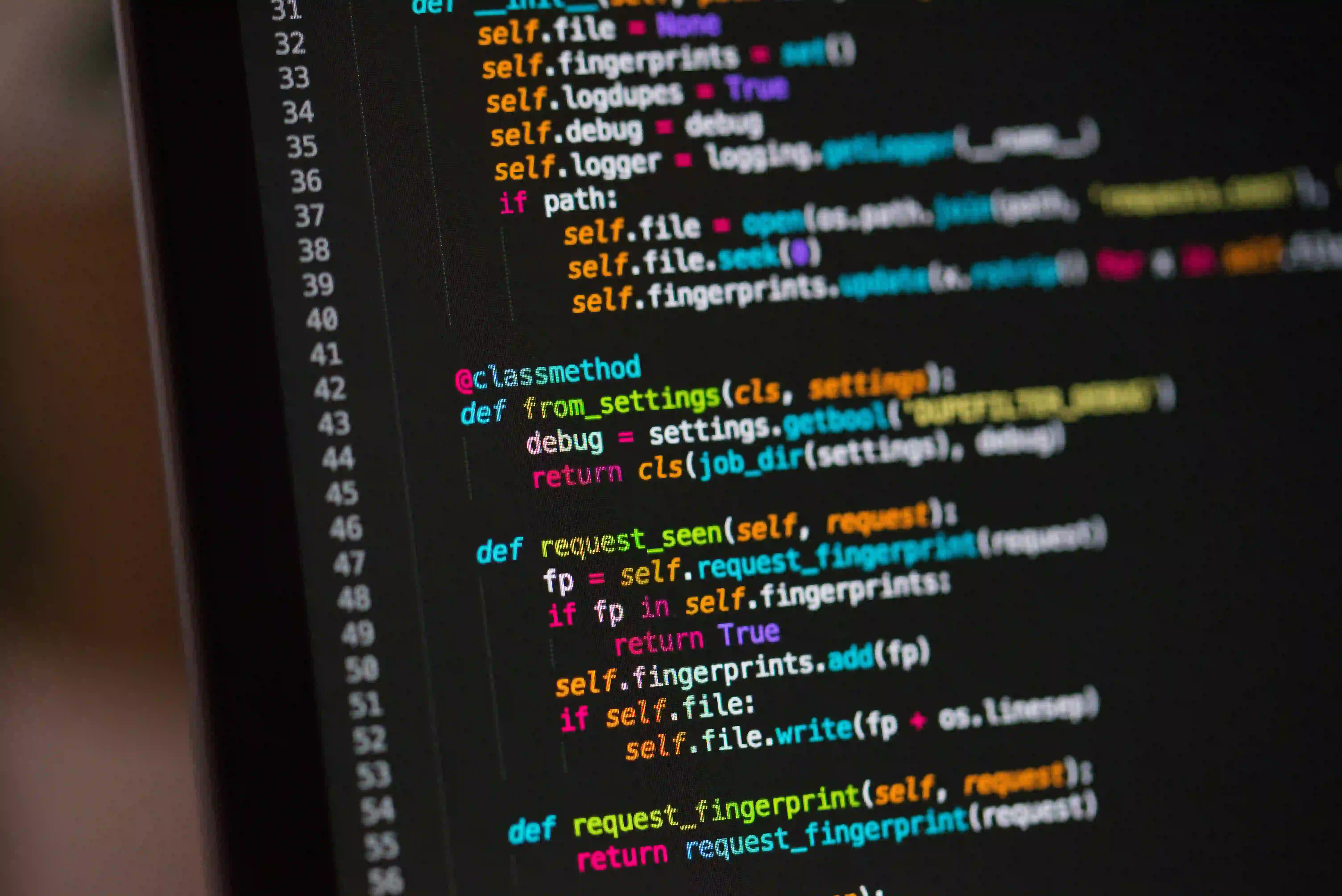
Common Pitfalls of Overusing @Autowired in Spring Framework
The Spring Framework provides a powerful set of tools for dependency injection, with the @Autowired
annotation serving as a key mechanism for injecting beans into your application. While it offers convenience, overusing @Autowired
can lead to code that is difficult to maintain, test, and understand. In this blog post, we will explore common pitfalls associated with the overuse of @Autowired
, along with practical recommendations to avoid these issues.
Understanding Dependency Injection
Before we delve into the pitfalls, it’s important to understand what dependency injection (DI) is. At its core, DI is a design pattern that allows a program to achieve inversion of control (IoC) between classes and their dependencies. In Spring, @Autowired
automatically resolves and injects beans into your components.
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
// Business logic methods here
}
In this example, UserService
gets its dependency on UserRepository
automatically injected. While this is clean and readable, we need to be cautious not to overuse @Autowired
.
Pitfall 1: Hidden Dependencies
One of the most significant downsides of overusing @Autowired
is that it hides dependencies. When dependencies are injected directly, it becomes increasingly challenging to understand what a class needs to function properly.
Example:
@Component
public class OrderService {
@Autowired
private UserService userService;
@Autowired
private PaymentService paymentService;
// Other methods...
}
Why This is an Issue:
In this setup, to understand OrderService
, you must check its injected dependencies. This can lead to confusion and complicate code comprehension, especially for new team members or for future maintenance.
Solution:
Instead, consider using constructor injection. It makes dependencies explicit and is particularly well-suited for unit testing.
@Component
public class OrderService {
private final UserService userService;
private final PaymentService paymentService;
@Autowired
public OrderService(UserService userService, PaymentService paymentService) {
this.userService = userService;
this.paymentService = paymentService;
}
// Other methods...
}
With constructor injection, anyone reading OrderService
can immediately see its dependencies.
Pitfall 2: Difficulties in Testing
While @Autowired
simplifies bean management, it can complicate unit testing. Tests become tightly coupled to the Spring context, resulting in slow test execution and masking possible design issues.
Example:
@RunWith(SpringJUnit4ClassRunner.class)
@SpringBootTest
public class OrderServiceTest {
@Autowired
private OrderService orderService;
@Test
public void testProcessOrder() {
// Test logic
}
}
Why This is an Issue:
The above test relies heavily on the Spring container, making it more difficult to isolate the unit being tested. It also incurs the overhead of starting up the application context.
Solution:
To make your tests more unit-test friendly, you can use Mockito to mock dependencies.
@RunWith(MockitoJUnitRunner.class)
public class OrderServiceTest {
@InjectMocks
private OrderService orderService;
@Mock
private UserService userService;
@Mock
private PaymentService paymentService;
@Test
public void testProcessOrder() {
// Test logic
}
}
By isolating the class using Mockito mocks, your tests run faster and focus on the OrderService
logic.
Pitfall 3: Over-Complex Configurations
While Spring Configuration classes can use @Autowired
, excessive usage can lead to overly complex configurations that detract from the benefits of dependency injection.
Example:
@Configuration
public class AppConfig {
@Autowired
private DataSource dataSource;
@Autowired
private UserRepository userRepository;
// Other bean definitions...
}
Why This is an Issue:
When multiple dependencies are injected directly into configuration classes, it spreads configuration concerns throughout your application.
Solution:
Use Java Configuration with method-level annotations to define dependencies, which enhances clarity.
@Configuration
public class AppConfig {
@Bean
public UserService userService(UserRepository userRepository) {
return new UserService(userRepository);
}
// Other bean definitions...
}
In this example, dependencies are handled directly within method parameters, resulting in clearer and more concise configuration.
Pitfall 4: Circular Dependencies
Another problem that can arise from overusing @Autowired
is the potential for circular dependencies. When two classes depend on each other via constructor injection, it leads to runtime exceptions.
Example:
@Component
public class A {
@Autowired
private B b;
}
@Component
public class B {
@Autowired
private A a;
}
Why This is an Issue:
When attempting to create instances of A
and B
, Spring cannot resolve the dependencies, resulting in a circular reference error.
Solution:
Consider refactoring your design. You can introduce an event-driven approach or extract the dependency into another supporting class to break the cycle.
@Component
public class A {
private final B b;
@Autowired
public A(B b) {
this.b = b;
}
}
@Component
public class B {
// No direct dependency on A
}
My Closing Thoughts on the Matter
Overusing @Autowired
in the Spring Framework can lead to hidden dependencies, testing difficulties, over-complex configurations, and circular dependencies. Embracing constructor injection, utilizing mocking frameworks for testing, simplifying configurations, and carefully analyzing dependencies can significantly enhance the maintainability and readability of your Spring applications.
If you want to dive deeper into dependency injection and avoid common pitfalls, check out Baeldung's Spring Dependency Injection Guide and Spring.io's official documentation.
Remember, the goal is not just to make code work, but to craft solutions that are elegant, easy to maintain, and facilitate collaboration within your development team. Happy coding!