Mastering Unit Testing for ExecutorService Without Thread Sleep
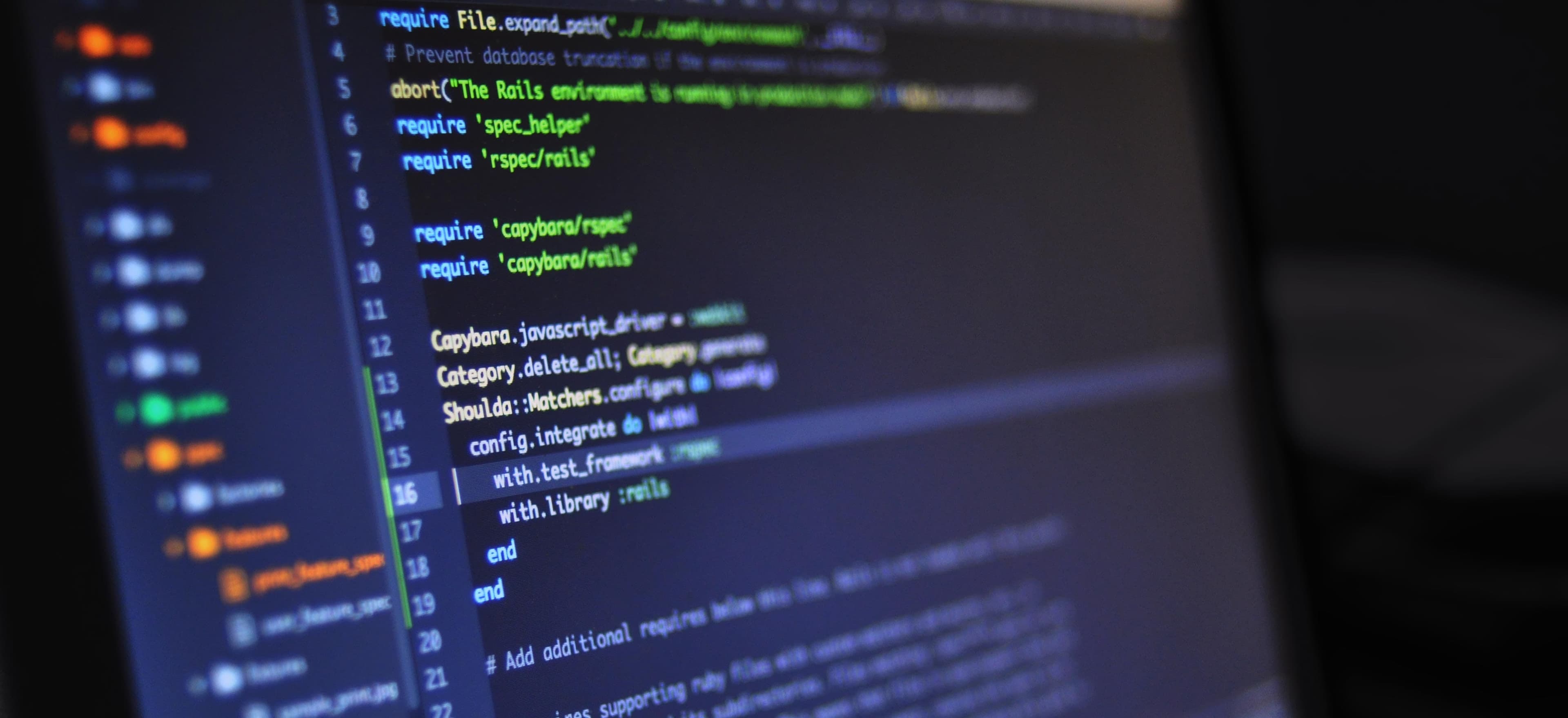
- Published on
Mastering Unit Testing for ExecutorService Without Thread Sleep
Unit testing is a vital part of the development process that ensures your code behaves as expected. In Java, ExecutorService
is a core component of the concurrency framework, enabling efficient execution of asynchronously running tasks. However, testing multi-threaded code can be tricky, especially when dealing with timing and dependencies. In this post, we'll explore how to effectively unit test ExecutorService
without resorting to Thread.sleep
, which can lead to flaky tests.
Why Avoid Thread.sleep in Unit Tests?
Using Thread.sleep
in unit tests can cause several issues:
- Flaky Tests: Tests may succeed or fail based on the timing and state of the system, leading to unreliable results.
- Buried Failures: If a test fails due to timing issues, it may be difficult to diagnose the real cause.
- Longer Execution Time: Adding artificial delays can slow down the test suite considerably.
Instead, we can adopt strategies that rely on synchronization and immediate feedback from the execution of our tasks.
The ExecutorService Overview
Before diving into unit tests, let's summarize ExecutorService
. It is part of the Java concurrency package and provides a high-level way to manage asynchronous task execution. You'll often use it for executing tasks in the background, allowing the main application to remain responsive.
Here's a simple example of using ExecutorService
:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class SimpleExecutorExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(2);
for (int i = 0; i < 5; i++) {
final int taskId = i;
executor.submit(() -> {
System.out.println("Task " + taskId + " is running.");
});
}
executor.shutdown();
}
}
In this example, we create a fixed thread pool and submit five tasks to it. Each task prints its ID, and we shut down the executor after submission.
Setting Up the Unit Test Environment
To unit test ExecutorService
, we need proper dependencies in your project. If you're using Maven, add the following to your pom.xml
:
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter</artifactId>
<version>5.8.2</version>
<scope>test</scope>
</dependency>
Testing with Mocking
Mocking libraries such as Mockito can be extremely helpful when it comes to unit testing ExecutorService
. Here's a simple example of using Mockito to test a method that utilizes an ExecutorService
.
Sample Service Class
First, we create a simple service class that uses ExecutorService
to perform some work:
import java.util.concurrent.ExecutorService;
public class TaskService {
private final ExecutorService executor;
public TaskService(ExecutorService executor) {
this.executor = executor;
}
public void executeTask(Runnable task) {
executor.submit(task);
}
}
Unit Test Class
Next, let's write a unit test for the TaskService
.
import static org.mockito.ArgumentMatchers.any;
import static org.mockito.Mockito.*;
import java.util.concurrent.ExecutorService;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
public class TaskServiceTest {
private ExecutorService executorService;
private TaskService taskService;
@BeforeEach
public void setUp() {
executorService = mock(ExecutorService.class);
taskService = new TaskService(executorService);
}
@Test
public void testExecuteTask() {
Runnable mockTask = mock(Runnable.class);
taskService.executeTask(mockTask);
// Verify that the task is submitted to the executorService
verify(executorService).submit(mockTask);
}
}
Commentary on the Code
- Mocking the ExecutorService: We mock
ExecutorService
using Mockito to isolate our tests. This enables us to focus on testing the behavior ofTaskService
without having to deal with actual threading. - Verifying Behavior: By validating that the
submit
method is called, we ensure that our service correctly submits the task. - Immediate Feedback: We don't introduce any sleep timers. Instead, the outcome is verified immediately.
Advanced Scenarios: Testing Completion of Tasks
While the simple test checks if tasks are submitted, it's essential also to test scenarios where tasks have completed. For this, we can use a combination of CountDownLatch and mocking.
Enhanced Service Class
Let's modify our service class to include a method to return the result of a task.
import java.util.concurrent.*;
public class TaskService {
private final ExecutorService executor;
public TaskService(ExecutorService executor) {
this.executor = executor;
}
public Future<Integer> executeCallable(Callable<Integer> task) {
return executor.submit(task);
}
}
Advanced Unit Test
Now, let's write a unit test to confirm that Callable
tasks execute correctly and return results.
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import java.util.concurrent.*;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class TaskServiceAdvancedTest {
private ExecutorService executorService;
private TaskService taskService;
@BeforeEach
public void setUp() {
executorService = mock(ExecutorService.class);
taskService = new TaskService(executorService);
}
@Test
public void testExecuteCallable() throws Exception {
Callable<Integer> mockTask = () -> 42;
Future<Integer> future = taskService.executeCallable(mockTask);
// Simulating the behavior of the executorService's submit method
when(executorService.submit(any(Callable.class))).thenReturn(mock(Task.class));
// Checking the result
assertEquals(42, future.get());
}
}
Commentary on the Enhanced Test
- Callable Tasks: We create a
Callable
that returns a fixed value and test if it executes successfully. - Using Futures: We leverage
Future.get()
to simulate retrieving the result of the callable task. - Mockito's Behavior Control: We use
when(...).thenReturn(...)
to control behaviors of our mockedExecutorService
.
Closing the Chapter
Unit testing for ExecutorService
in Java without Thread.sleep
is an achievable goal. By using mocking techniques with libraries such as Mockito, along with solid design practices, we can create reliable, fast-running tests for our concurrent code.
For those delving deeper into concurrency and asynchronous programming in Java, consider reviewing the Java Concurrency in Practice book for comprehensive insights.
By applying these principles and methodologies, you will greatly improve your unit testing practices for multi-threaded environments. Remember, the goal is clarity and reliability in your tests; with the diminished reliance on timing-based assertions, you will be well on your way to achieving just that!
Checkout our other articles