Apache Commons vs JDK: Choosing the Right Utility Library
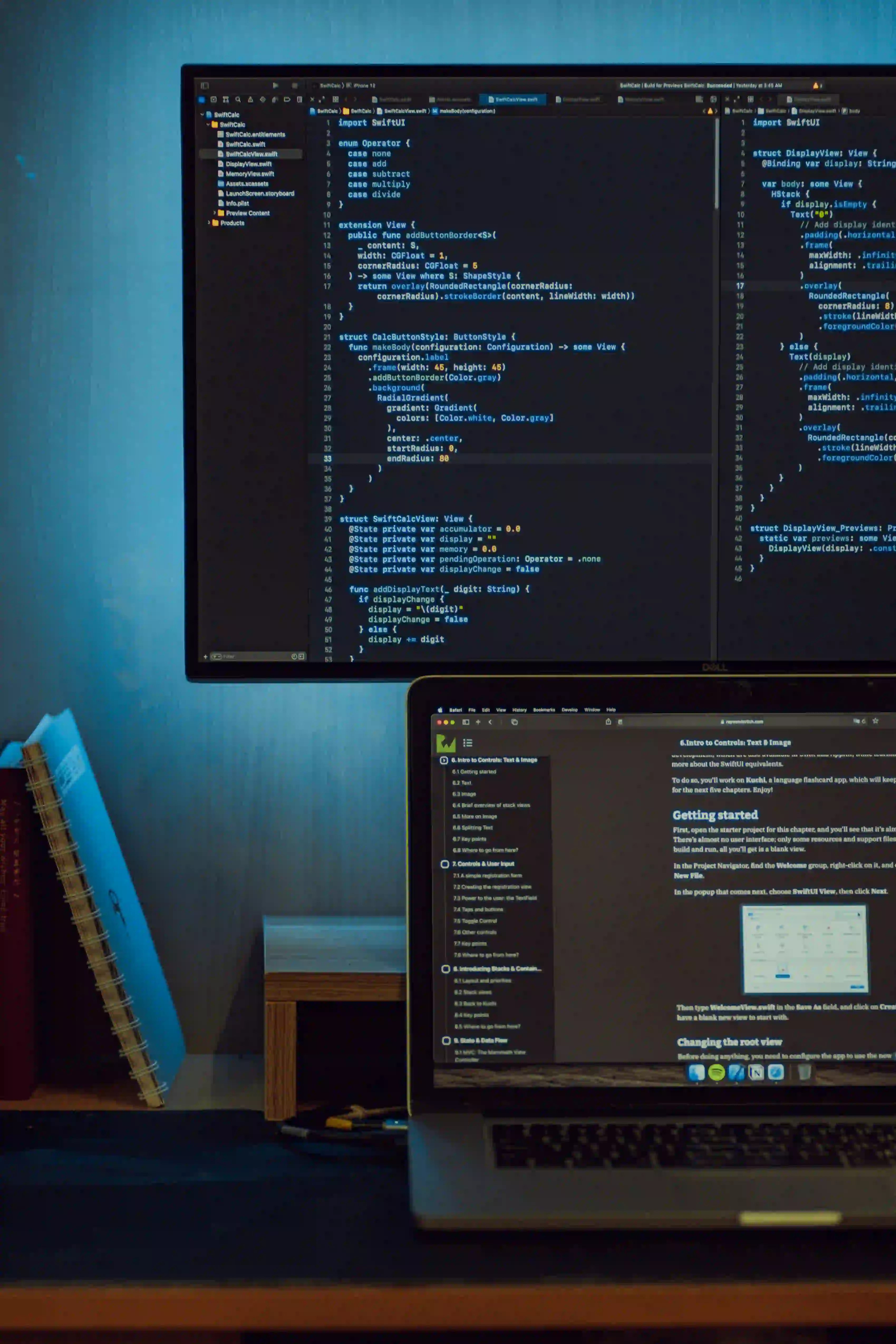
Apache Commons vs JDK: Choosing the Right Utility Library
When developing Java applications, the decision between using standard Java Development Kit (JDK) utilities or third-party libraries like Apache Commons can impact your code's efficiency, maintainability, and overall performance. In this blog post, we'll delve into the strengths and weaknesses of both options, and provide insights on how to choose the right utility library for your project.
Understanding the JDK Utilities
The JDK offers a rich set of utility classes that are designed to simplify common programming tasks. For example, the java.util
package includes classes like ArrayList
, HashMap
, and Collections
, which provide powerful functionality out of the box.
Example: Using Java Collections
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class JdkUtilsExample {
public static void main(String[] args) {
List<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
names.add("Charlie");
Collections.sort(names);
System.out.println("Sorted names: " + names);
}
}
In the above code, we create an ArrayList
of names, add a few entries, sort them using the Collections.sort()
method, and print the sorted list. This highlights the utility of JDK's built-in libraries.
Pros of JDK Utilities
- Built-in Availability: No need for additional dependencies. You can start coding right away.
- Performance: Being native to Java, JDK utilities are generally optimized and offer good performance.
- Stability: JDK classes are well-tested and provide a consistent interface across Java versions.
Cons of JDK Utilities
- Limited Functionality: JDK utilities may not cover all use cases, especially for more complex tasks.
- Redundancy: Different classes often perform similar functions, leading to potential confusion.
Stepping into the Topic to Apache Commons
Apache Commons is a popular set of reusable Java components that aims to simplify common programming tasks. Its modular design allows developers to pick and choose the utilities they need, making it a flexible alternative to JDK libraries.
Example: Using Apache Commons Lang
import org.apache.commons.lang3.StringUtils;
public class CommonsUtilsExample {
public static void main(String[] args) {
String str = " Hello World! ";
// Trim leading and trailing spaces
String trimmed = StringUtils.trim(str);
System.out.println("Trimmed String: '" + trimmed + "'");
// Check for empty or null
boolean isEmpty = StringUtils.isEmpty(trimmed);
System.out.println("Is the string empty? " + isEmpty);
}
}
In this example, we leverage Apache Commons Lang for string manipulation. The StringUtils.trim()
method simplifies whitespace removal, while StringUtils.isEmpty()
more cleanly checks for empty or null strings compared to manual checks.
Pros of Apache Commons
- Extended Functionality: Apache Commons provides a broad range of utilities that extend Java's capabilities.
- Ease of Use: Its methods often streamline tasks, making the code cleaner and more readable.
- Specialized Libraries: Different Commons modules (such as
Commons Lang
,Commons IO
) cater to specific areas, allowing for targeted use.
Cons of Apache Commons
- Additional Dependency: Incorporating Apache Commons means adding extra libraries to your project.
- Learning Curve: New users must familiarize themselves with the various modules, methods, and conventions.
Performance Comparison
When it comes to performance, JDK utilities often have the edge due to their inherent optimizations. However, Apache Commons is lightweight and typically performs well. The right choice will depend on the specific tasks you need to accomplish and how critical performance is for those tasks.
Performance Example: String Concatenation
// Using JDK
String result = "Hello" + " " + "World";
// Using Apache Commons
String resultWithCommons = StringUtils.join(new String[]{"Hello", "World"}, " ");
In scenarios where string manipulation is frequent, Apache Commons provides methods that can reduce overhead and enhance readability. However, for simple tasks such as concatenation, JDK's built-in capabilities are often sufficient and efficient.
Choosing the Right Utility Library
Assess Your Needs
The first step in your decision-making process is to thoroughly assess your project's requirements. Ask yourself the following questions:
- What kinds of tasks are you performing?
- Do you need extensive utility functions beyond what the JDK provides?
- Is maintaining simplicity and minimizing dependencies a priority?
Understand Team Familiarity
Another important consideration is your team's familiarity with either library. If your development team already has experience with Apache Commons, it might increase productivity compared to sticking solely with JDK utilities. Conversely, if your team is well-versed in the JDK, leveraging its utilities might be more beneficial.
Weigh Long-term Maintenance
Lastly, consider the long-term implications of your choice. The JDK's built-in utilities are routinely updated and maintained, while third-party libraries must be managed separately. Ensure that whichever library you choose aligns with your project's sustainability and maintainability goals.
The Bottom Line
In summary, both JDK utilities and Apache Commons have their place in Java development. JDK utilities offer performance, stability, and simplicity, making them ideal for straightforward programming tasks. However, Apache Commons shines when you need extended functionality and specialized utilities, enhancing your code's readability and maintainability.
By critically assessing your project's needs, team experience, and long-term goals, you can make an informed choice that balances functionality, performance, and simplicity.
For further reading on Apache Commons, you can visit their official documentation. If you're looking for more information on JDK utilities, check out the Java SE Documentation.
Now that you're equipped with the insights to choose wisely, happy coding!