Navigating Latency Issues in Distributed Quasar Actors
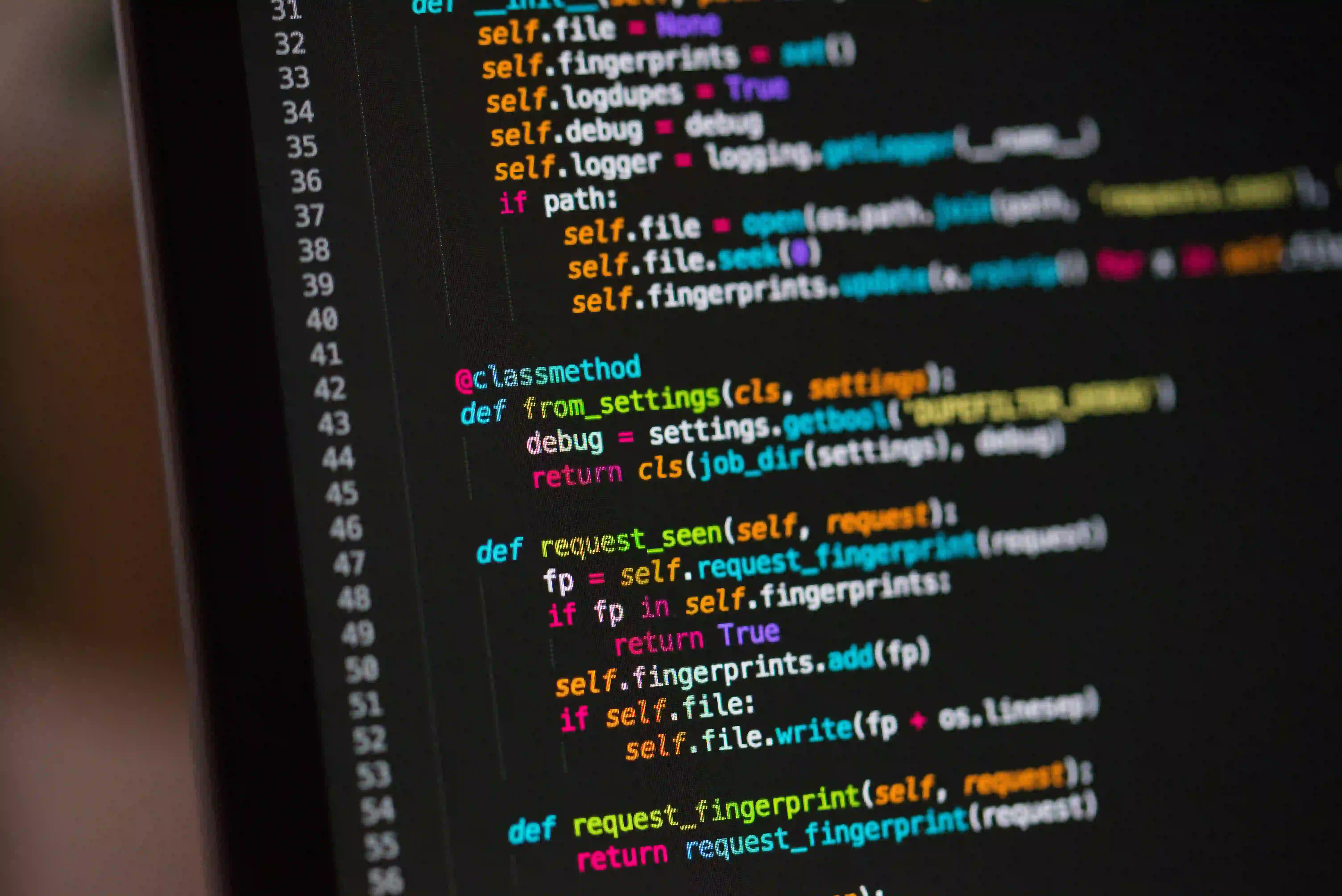
Navigating Latency Issues in Distributed Quasar Actors
As the need for high-performance, scalable applications continues to rise, developers frequently turn to asynchronous programming models. One such model gaining traction is the Actor model, which excels in building robust, concurrent systems. Quasar, a library for JVM that provides lightweight threading and actors, enhances the Actor model to improve the performance of Java applications. However, even the most sophisticated systems face latency issues. In this blog post, we will explore how to navigate these latency issues effectively using Quasar Actors.
Understanding Quasar Actors
Quasar provides a way to create and manage lightweight threads and actors in Java applications. Actors are fundamental building blocks that encapsulate state and behavior. They communicate with each other via asynchronous message passing, making them an ideal choice for distributed systems where components operate independently.
Here’s a simple example of creating an actor using Quasar:
import co.paralleluniverse.actors.Actor;
import co.paralleluniverse.actors.ActorRef;
public class HelloActor extends Actor<String> {
@Override
public void luzrun() {
while (true) {
String message = receive();
System.out.println("Hello, " + message + "!");
}
}
}
// In your main method
ActorRef<String> helloActor = spawn(new HelloActor());
helloActor.send("World");
Why Use Quasar Actors?
-
Lightweight Concurrency: Quasar actors use fibers, which are a lightweight alternative to traditional threads. This enables high throughput with considerably less memory overhead.
-
Transparent Asynchrony: Sending messages between actors is non-blocking, which keeps your system responsive.
-
Easier Maintenance: The encapsulated state in actors allows for a cleaner separation of concerns, making the system easier to manage.
However, despite these advantages, developers may face latency problems. Understanding where latency originates and how to mitigate it is crucial for maximizing the potential of Quasar Actors.
Identifying Latency Sources
Latency in distributed actor systems can stem from multiple factors:
-
Network Latency: The most apparent source of latency is the time taken to send messages over the network.
-
Processing Latency: Each actor has its own processing time based on the complexity of the task it performs.
-
Contention: When multiple actors try to access shared resources or invoke each other, contention can lead to delays.
-
Serialization Overhead: Messaging involves serialization and deserialization of data, which can introduce additional latency.
Identifying and Measuring Latency
To address latency effectively, it is crucial first to measure and identify its sources. You can use tools like Java Flight Recorder or VisualVM to monitor performance and diagnose where your system is lagging.
Strategies to Reduce Latency
1. Optimize Message Passing
Effective message passing is fundamental to mitigating latency. Here’s an example of an actor that processes messages in batches:
import co.paralleluniverse.actors.Actor;
import co.paralleluniverse.actors.ActorRef;
public class BatchingActor extends Actor<String[]> {
@Override
public void luzrun() {
String[] messages = new String[10];
int index = 0;
while (true) {
// Receive batch of messages
messages[index] = receive();
index++;
// Process batch when filled
if (index == messages.length) {
processMessages(messages);
index = 0; // Reset index
}
}
}
private void processMessages(String[] messages) {
for (String message : messages) {
System.out.println("Batch Processing: " + message);
}
}
}
// In your main method
ActorRef<String[]> batchingActor = spawn(new BatchingActor());
for (int i = 0; i < 25; i++) {
batchingActor.send("Message " + i);
}
Why Batching?
Batching reduces the number of send and process calls, thereby minimizing communication overhead. This technique can significantly cut down on the latency involved in frequent messaging.
2. Use Non-blocking Data Structures
Choosing the right data structure can have a marked impact on performance and latency. Non-blocking data structures can help alleviate contention, allowing multiple actors to access resources more smoothly.
For example, using a ConcurrentHashMap
instead of a synchronized HashMap
can improve throughput in an actor system.
3. Reduce Serialization Costs
Serialization can be expensive, especially if your messages are complex. You can use libraries such as Kryo or JSON libraries like Jackson to serialize data more effectively.
Here’s a simple serialization demo using Kryo:
import com.esotericsoftware.kryo.Kryo;
public class SerializationExample {
private static final Kryo kryo = new Kryo();
public static byte[] serialize(Object object) {
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
Output output = new Output(outputStream);
kryo.writeObject(output, object);
output.close();
return outputStream.toByteArray();
}
public static Object deserialize(byte[] bytes) {
Input input = new Input(bytes);
return kryo.readObject(input, YourObject.class);
}
}
Why Optimize Serialization?
Optimizing serialization can reduce the time taken to convert data to and from the byte stream, lowering the overall latency of network calls.
4. Horizontal Scaling
Finally, don’t underestimate the straightforward approach of scaling horizontally. By distributing actors across multiple instances, you reduce the load on individual actors, potentially decreasing the processing time per message.
Consider employing a load balancer to distribute incoming messages to actors efficiently, which can relieve bottlenecks arising from actor contention.
Closing Remarks
Navigating latency issues in distributed Quasar actor systems requires a multi-faceted approach. By understanding the factors that contribute to latency and employing necessary optimizations, developers can ensure their applications remain responsive and efficient.
As a best practice, always monitor your application’s performance and tweak your architecture accordingly. For further reading, check out the official Quasar documentation and Actor Model on Wikipedia for a more in-depth understanding of actors and concurrency.
By embracing such strategies, you can fully leverage the capabilities of Quasar Actors and deliver performant, high-throughput applications in a distributed environment.