Common Pitfalls When Using MongoDB with Java
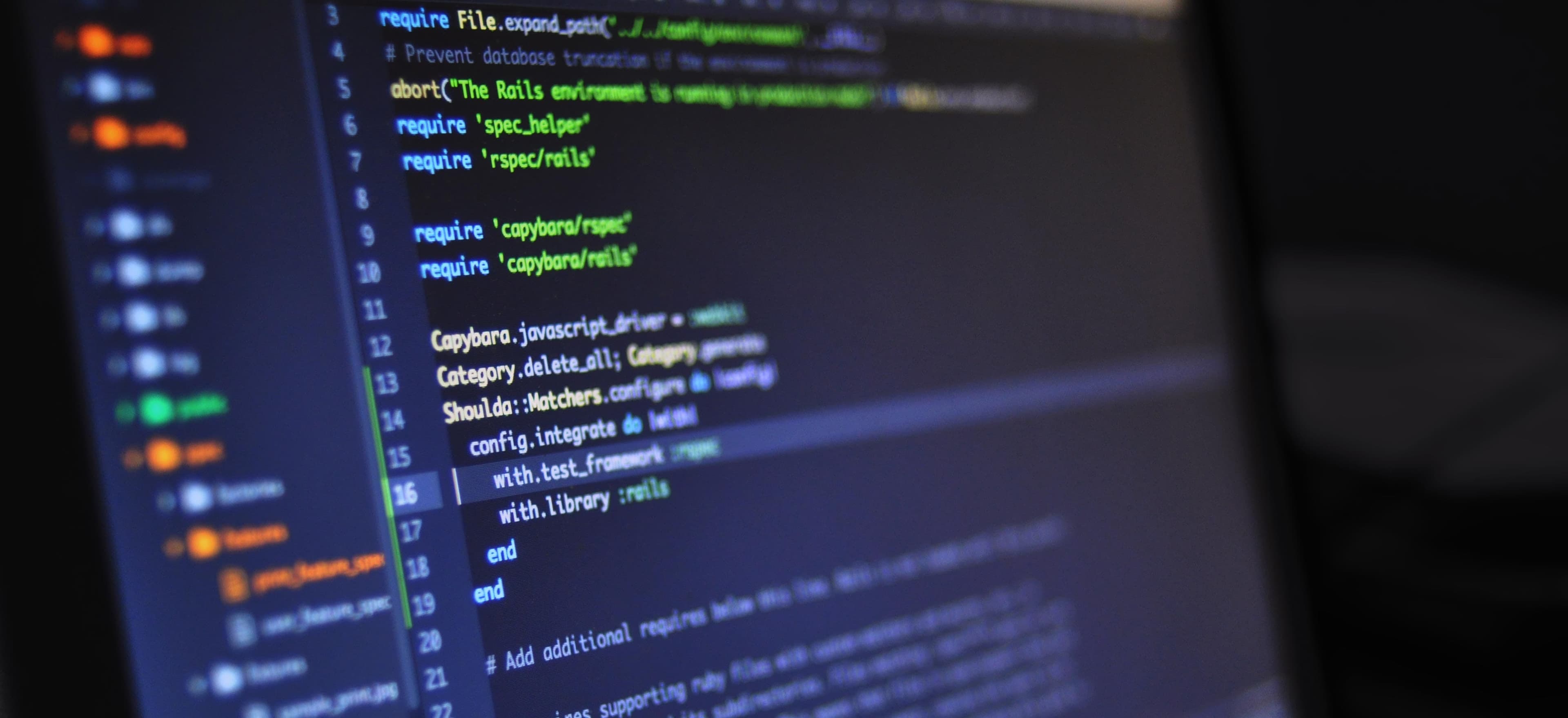
- Published on
Common Pitfalls When Using MongoDB with Java
MongoDB, a leading NoSQL database, is increasingly popular among developers for its flexibility and scalability. Java, one of the most widely used programming languages, pairs well with MongoDB, allowing for robust application development. However, using MongoDB with Java is not without its challenges. In this post, we will explore some common pitfalls developers encounter when working with MongoDB in Java, how to avoid them, and best practices to follow.
Establishing the Context to MongoDB and Java
Java offers native support for MongoDB through the official MongoDB Java Driver. This driver provides a comprehensive interface for performing database operations effectively. However, while it simplifies the implementation of database interactions, developers often run into issues that can hinder performance, lead to data inconsistency, or even cause application failures. Understanding these pitfalls is crucial in developing a robust application.
Pitfall 1: Poor Schema Design
Description
MongoDB's flexible schema design can be a double-edged sword. Developers may be tempted to store data in a way that appears convenient at first but becomes unmanageable as the application scales.
Solution
Define a clear schema strategy based on your application requirements. Utilize MongoDB's capabilities for embedding or referencing documents wisely.
Example: Embedding vs. Referencing
Consider a User
document where each user can have multiple Orders
.
Embedding Example:
public class User {
private String id;
private String name;
private List<Order> orders; // Embedding orders within user
}
public class Order {
private String orderId;
private double amount;
}
This design works well when users have a manageable number of orders. However, if some users have hundreds of orders, it may lead to performance issues.
Referencing Example:
public class User {
private String id;
private String name;
// Removing orders from the user document
}
public class Order {
private String orderId;
private double amount;
private String userId; // Referencing user with userId
}
In this case, using references provides better performance for users with many orders.
Pitfall 2: Ignoring Proper Indexing
Description
One of the main advantages of using MongoDB is its ability to handle large amounts of data. However, without proper indexing, query performance can degrade significantly.
Solution
Create indexes on fields that you frequently query, sort, or join. MongoDB allows various types of indexes, including single-field, compound, and geospatial indexes.
Example: Creating an Index
import com.mongodb.client.MongoCollection;
import org.bson.Document;
MongoCollection<Document> collection = database.getCollection("users");
// Creating an index on the "name" field
collection.createIndex(Indexes.ascending("name"));
Failing to index appropriately can lead to slow queries and a poor user experience.
Pitfall 3: Not Using Connection Pooling
Description
Every time a MongoDB connection is established, it consumes resources. New developers may overlook configuring connection pooling, leading to performance bottlenecks.
Solution
Utilize connection pooling to manage connections efficiently. Connection pools allow multiple operations to reuse existing connections instead of creating new ones.
Example: Configuring Connection Pooling
MongoClientOptions options = MongoClientOptions.builder()
.connectionsPerHost(10)
.build();
MongoClient mongoClient = new MongoClient(new ServerAddress("localhost", 27017), options);
This example sets up a connection pool that allows 10 concurrent connections, optimizing resource usage.
Pitfall 4: Data Redundancy and Inconsistency
Description
In NoSQL databases like MongoDB, data redundancy can occur when multiple copies of the same data are stored in different documents. This can lead to data inconsistency, especially in applications requiring frequent updates.
Solution
Ensure consistency by selecting the appropriate data modeling strategy. Employ a mix of embedding and referencing, depending on the frequency of updates.
Example: Updating Embedded Documents
If you're embedding documents, ensure you consider the impact of updates.
// Updating an embedded order
collection.updateOne(Filters.eq("userId", userId),
Updates.set("orders.$[elem].amount", newAmount),
new UpdateOptions().arrayFilters(Arrays.asList(Filters.eq("elem.orderId", orderId))));
Using array filters can help locate embedded documents for an efficient update.
Pitfall 5: Lack of Proper Error Handling
Description
When interacting with MongoDB, not handling exceptions correctly can lead to silent failures or unhandled scenarios in the application.
Solution
Implement robust error handling mechanisms through try-catch blocks.
Example: Handling Exceptions
try {
collection.insertOne(newUser);
} catch (MongoWriteException e) {
// Log the error
System.err.println("Write error occurred: " + e.getMessage());
}
This approach ensures that developers are informed of issues, allowing for timely troubleshooting.
Pitfall 6: Overlooking Data Validation
Description
MongoDB's schema-less nature can lead developers to skip data validation steps, resulting in corrupted or inconsistent data.
Solution
Implement data validation directly within the application or use MongoDB's built-in validation features.
Example: Using Validators
db.createCollection("users", {
validator: { $jsonSchema: {
bsonType: "object",
required: [ "name", "email" ],
properties: {
name: {
bsonType: "string",
description: "must be a string and is required"
},
email: {
bsonType: "string",
pattern: "^.+@.+..+$",
description: "must be a valid email and is required"
}
}
} }
})
This creates a validator for the users
collection, ensuring that all documents adhere to specified rules.
Pitfall 7: Not Setting Up Backups
Description
Failing to implement a proper backup and recovery solution can lead to irretrievable data losses.
Solution
Establish a backup strategy using MongoDB's tools like mongodump and mongorestore.
Example: Basic Backup Command
mongodump --db your_database_name --out /path_to_backup/
Regular backups enhance data safety, allowing teams to restore systems quickly when necessary.
Final Considerations
Working with MongoDB in Java offers numerous advantages, but it also comes with unique challenges. By being aware of common pitfalls and implementing best practices, such as proper schema design, effective indexing, connection pooling, and robust error handling, developers can maximize the performance and reliability of their applications.
If you want to dive deeper into MongoDB's capabilities or explore advanced features, check out the official MongoDB documentation or the MongoDB Java Driver documentation.
By maintaining vigilance and applying the outlined strategies, you can confidently navigate the complexities of MongoDB in your Java applications and build robust, scalable solutions.
Checkout our other articles