Overcoming Challenges in Polyglot Persistence Integration
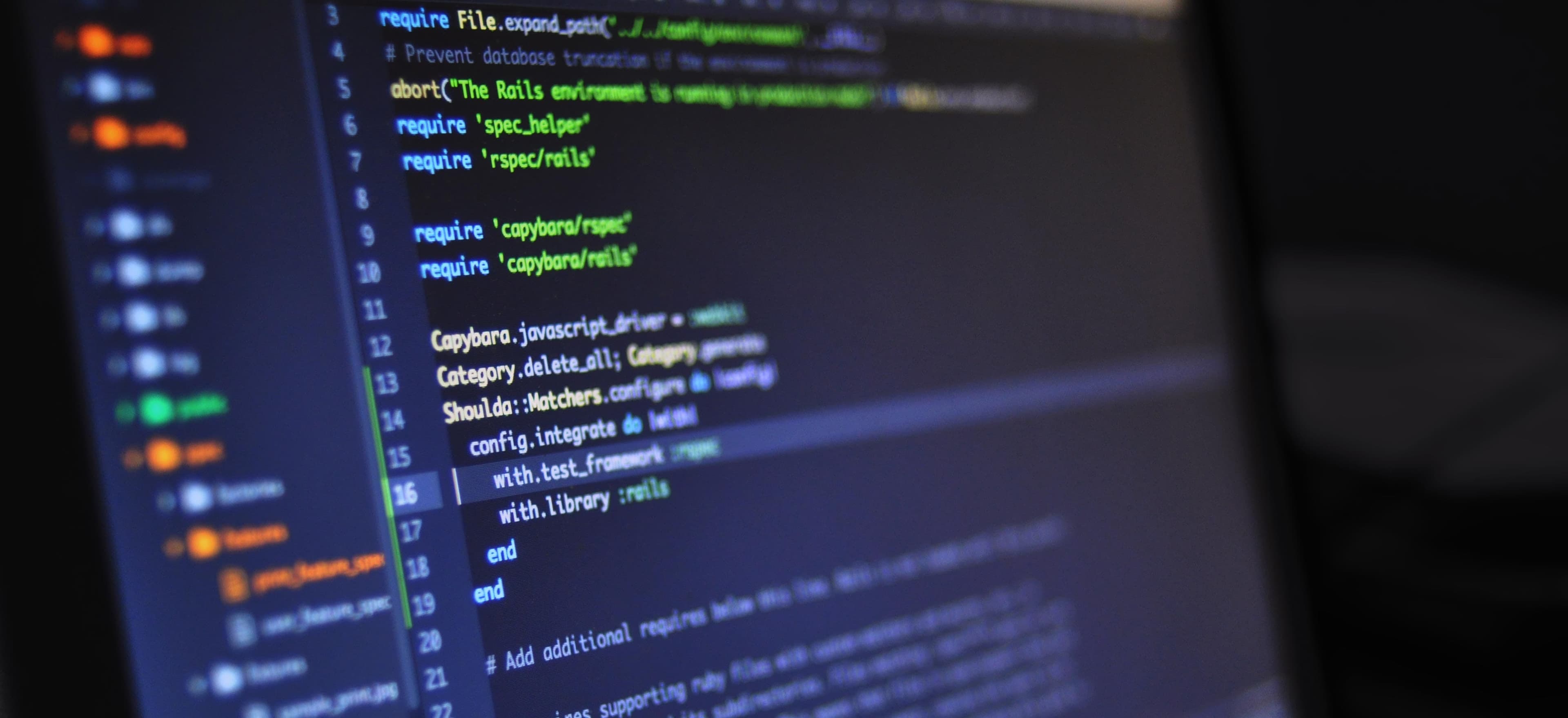
- Published on
Overcoming Challenges in Polyglot Persistence Integration
In the world of software development, the term "polyglot persistence" describes the ability to use multiple data storage technologies to meet various data storage needs. As applications become more complex, developers are increasingly adopting this strategy. However, integrating diverse data systems poses significant challenges. In this blog post, we’ll dive into the fundamental challenges in polyglot persistence integration and how you can effectively overcome them.
Understanding Polyglot Persistence
Before we explore the challenges, let’s clarify what polyglot persistence is. In simple terms, it allows developers to use different data storage technologies based on specific use cases. For instance:
- Relational databases may handle structured data.
- NoSQL databases could manage unstructured data.
- In-memory databases may be utilized for real-time applications.
This flexibility is immensely beneficial but not without its hurdles.
Common Challenges in Polyglot Persistence Integration
1. Data Consistency
Challenge: Managing data consistency across different databases can be tricky. Each database has its consistent models and strategies, making it challenging to ensure that all data remains accurate and synchronized.
Solution: The use of distributed transactions or eventual consistency models can help. When implementing distributed transactions, additional mechanisms, such as the two-phase commit protocol, can maintain integrity across databases.
// Pseudo-code example of a distributed transaction
public void performTransaction() {
try {
DatabaseA.beginTransaction();
DatabaseB.beginTransaction();
// Perform operations on Database A
DatabaseA.insert(recordA);
// Perform operations on Database B
DatabaseB.update(recordB);
DatabaseA.commit();
DatabaseB.commit();
} catch (Exception e) {
DatabaseA.rollback();
DatabaseB.rollback();
System.out.println("Transaction rolled back due to: " + e.getMessage());
}
}
2. Complexity of Queries
Challenge: Writing queries that span multiple data stores introduces significant complexity. Each database has different querying mechanisms.
Solution: To bridge the gap, consider adopting APIs to abstract away the complexities. Using graph databases in combination with other databases can also simplify traversing relationships across different data stores.
// Example of using APIs to abstract multiple database queries
public List<User> fetchUsersAndOrders() {
List<User> users = userApi.fetchAllUsers();
for (User user : users) {
List<Order> orders = orderApi.fetchOrdersByUserId(user.getId());
user.setOrders(orders);
}
return users;
}
3. Data Modeling
Challenge: Each database has its data modeling techniques, from relational schemas to document-based models. Establishing a coherent and effective data model across different systems is daunting.
Solution: Implement a common domain model across databases. This model should focus on the business requirements rather than the database technologies used.
// Unified domain model example
public class User {
private int id;
private String name;
private List<Order> orders; // Represents a relationship between user and orders
}
Why this Matters: This model ensures a level of abstraction and eases the mapping of objects to various database systems.
4. Transaction Management
Challenge: In a polyglot environment, managing transactions manually can lead to deadlocks and data inconsistencies.
Solution: Use orchestrated transaction management via integration frameworks like Spring Cloud Data Flow. These frameworks provide tools to manage complex transactions across multiple data stores.
@SpringBootApplication
public class ExampleApplication {
public static void main(String[] args) {
SpringApplication.run(ExampleApplication.class, args);
}
@Bean
public TransactionManager transactionManager(EntityManagerFactory emf) {
return new JpaTransactionManager(emf);
}
}
5. Performance Overhead
Challenge: Polyglot persistence can introduce performance overhead due to the increased complexity in handling multiple systems and their respective APIs.
Solution: Optimize data retrieval and storage mechanisms. Use caching strategies, such as Redis, to reduce the load on databases.
// Example of caching with Redis
public class UserService {
private final RedisTemplate<String, User> redisTemplate;
public UserService(RedisTemplate<String, User> redisTemplate) {
this.redisTemplate = redisTemplate;
}
public User getUser(String userId) {
User user = redisTemplate.opsForValue().get(userId);
if (user == null) {
user = userRepository.findById(userId);
redisTemplate.opsForValue().set(userId, user); // Cache it for future requests
}
return user;
}
}
6. Skills and Learning Curve
Challenge: A polyglot persistence architecture can be overwhelming for teams. Different databases mean mastering various technologies, leading to a steep learning curve.
Solution: Continuous learning and training must be prioritized. Regular workshops or collaborative projects can help teams familiarize themselves with new technologies.
Best Practices for Successfully Implementing Polyglot Persistence
-
Understand the Requirements: Start with a thorough understanding of the business needs and choose storage technologies wisely based on those requirements.
-
Design for Interoperability: Ensure that all systems can communicate effectively, using REST APIs or message brokers for smooth integration.
-
Monitor Performance: Employ monitoring tools to gain insights into data access patterns and address performance bottlenecks proactively.
-
Incremental Implementation: Start integrating polyglot persistence gradually. Begin with one or two databases, then expand as the team becomes comfortable.
-
Documentation and Standards: Maintain comprehensive documentation of your data architecture, integration points, and design standards for easier onboarding of team members.
The Last Word
Polyglot persistence offers developers tremendous flexibility and efficiency for handling diverse data needs. Nevertheless, the complexities surrounding integration present challenges that demand careful strategy and robust solutions.
To effectively manage these hurdles, embrace best practices such as incremental implementation, continuous learning, and robust monitoring. By understanding both the what and the why of your design choices, your team can harness the power of polyglot persistence, reaping its benefits without falling prey to its pitfalls.
For further reading, consider visiting Martin Fowler's article on Polyglot Persistence or exploring the nuances of databases and modeling representations in Domain-Driven Design.
Remember, the key to mastering polyglot persistence lies in your team’s adaptability, ongoing education, and a thoughtful approach to system architecture.
Checkout our other articles