Common Pitfalls When Testing Dockerized SQL Databases
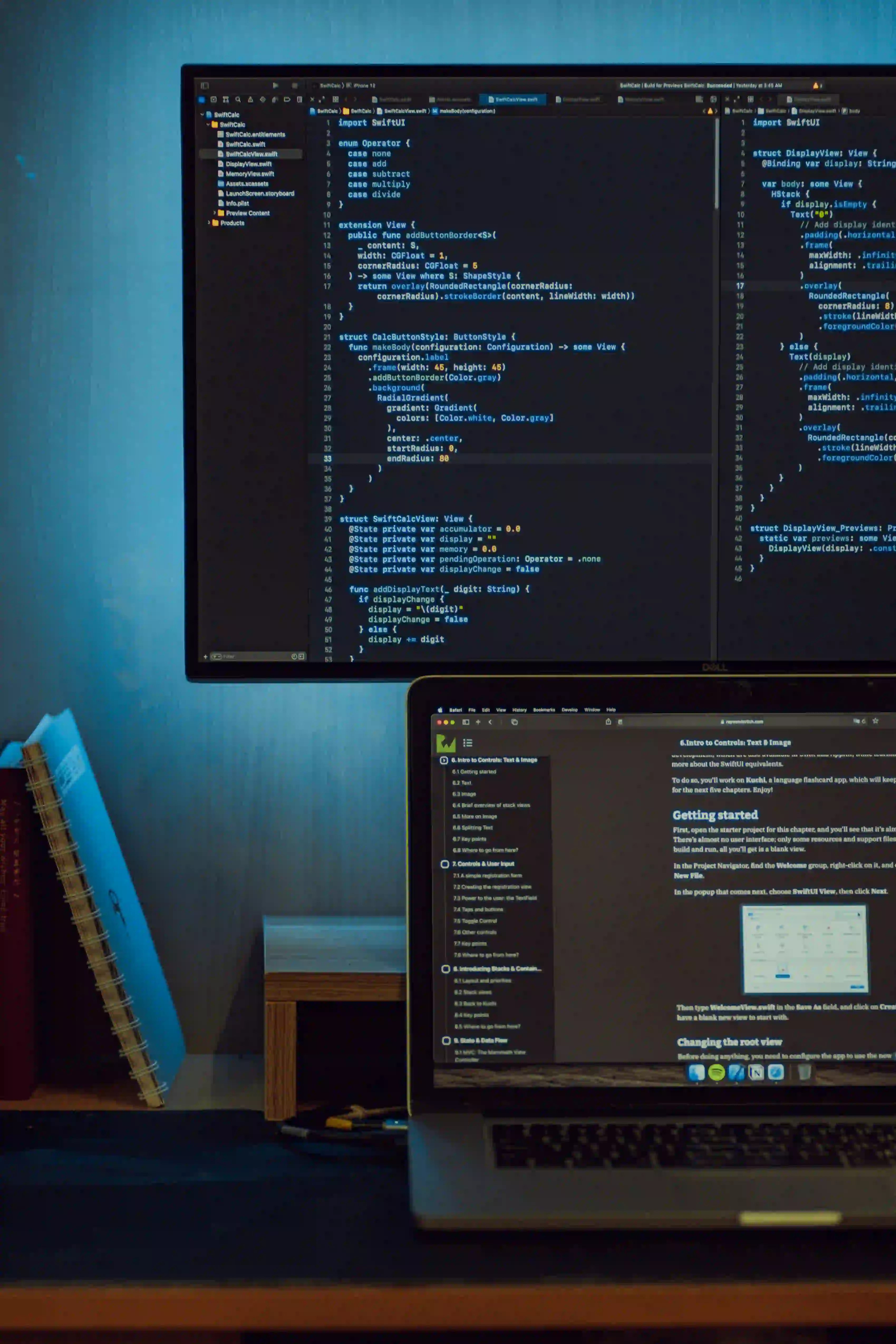
Common Pitfalls When Testing Dockerized SQL Databases
As the devops landscape continues to evolve, the use of containers for deploying applications has gained immense popularity. Docker, in particular, has become a staple in many development workflows. However, when it comes to database management, especially SQL databases, there are several common pitfalls that developers encounter. In this blog post, we will explore the intricacies of testing Dockerized SQL databases and highlight key considerations to keep in mind to avoid potential issues.
Understanding the Dockerized SQL Environment
Before diving into the pitfalls, it's important to grasp the concept of Dockerizing a SQL database. Docker allows you to create, deploy, and run applications within containers, which are lightweight, portable, and consistent across different environments.
Dockerfile for SQL Database
Creating a Dockerized SQL database begins with a Dockerfile. Below is a simple example of how to set up a MySQL environment in a Docker container.
# Start from the official MySQL image
FROM mysql:5.7
# Set environment variables
ENV MYSQL_ROOT_PASSWORD=rootpassword
ENV MYSQL_DATABASE=testdb
# Copy the initialization script
COPY ./init.sql /docker-entrypoint-initdb.d/
Why Use a Dockerfile?
Using a Dockerfile allows you to define your environment in a clear and reusable way. You can control the database version, configuration settings, and even seed data with ease. Being explicit is critical to managing a reliable test environment.
Pitfall #1: Not Using Persistent Data Volumes
One of the most significant mistakes developers make when testing with Dockerized SQL databases is failing to use persistent data volumes. By default, Docker containers are ephemeral. This means that whenever a container is stopped or removed, the data within it is lost.
Solution: Configure Persistent Volumes
To avoid losing crucial data during testing, you should configure Docker volumes. Here's an example of using a named volume with your SQL container:
docker run --name my-mysql -e MYSQL_ROOT_PASSWORD=rootpassword -e MYSQL_DATABASE=testdb -v mysql_data:/var/lib/mysql -d mysql:5.7
Why Use Named Volumes?
Named volumes ensure that the data persists independently from the container lifecycle. This practice is essential for testing scenarios where you might want to preserve the state of the database between different test runs.
Pitfall #2: Overlooking Network Isolation
Another common error is not considering the security and network isolation aspects of Docker containers. When running multiple containers, especially in a microservices architecture, you must manage how they communicate with each other.
Solution: Use Docker Networks
Leverage Docker's built-in networking features to isolate your SQL container from others unless necessary.
docker network create my-network
docker run --network my-network --name my-mysql -e MYSQL_ROOT_PASSWORD=rootpassword -d mysql:5.7
Why Is Network Isolation Important?
Network isolation aids in preventing unwanted access and potential security vulnerabilities. It also simplifies the debugging process by clearly defining which containers can communicate with each other.
Pitfall #3: Ignoring Database Initialization
When testing SQL databases, developers often overlook the initialization phase. This is critical for ensuring that the database is set up correctly before executing tests.
Solution: Use Initialization Scripts
In your Dockerfile, you can include an initialization script to create tables or insert seed data.
Consider the following SQL script (init.sql
):
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(50) NOT NULL,
email VARCHAR(100) NOT NULL
);
INSERT INTO users (username, email) VALUES
('testuser1', 'test1@example.com'),
('testuser2', 'test2@example.com');
Why Include Initialization Scripts?
By including an initialization script, you ensure your database starts in a known state. This is particularly beneficial for automated tests, where you need repeatable and consistent setups.
Pitfall #4: Relying on Hardcoded Values
Another common pitfall is the reliance on hardcoded connection values in your application code. This can make testing more cumbersome and error-prone.
Solution: Use Environment Variables
Utilize environment variables for managing database configurations. Adjust your database connection settings according to the active environment.
String dbUrl = System.getenv("DB_URL");
String username = System.getenv("DB_USER");
String password = System.getenv("DB_PASS");
Why Use Environment Variables?
Environment variables enhance flexibility and security. They allow you to modify configurations without changing the code base, making it easier to switch between development, testing, and production environments.
Pitfall #5: Neglecting Clean Up
After running tests, many developers do not properly clean up their Docker resources, leading to orphaned containers and volumes.
Solution: Implement a Cleanup Strategy
After test execution, ensure resources are removed. Use commands like:
docker rm -f my-mysql
docker volume rm mysql_data
Why Is Cleanup Necessary?
Neglecting to clean up can lead to resource exhaustion on your machine, ultimately impacting performance. Additionally, cleaning up prevents conflicts in future test runs.
Lessons Learned
Testing Dockerized SQL databases can vastly improve your application’s development process, but it does come with its set of challenges. By being vigilant about certain pitfalls—such as not using persistent volumes, overlooking network isolation, ignoring initialization, relying on hardcoded values, and neglecting cleanup—you can ensure a smoother and more efficient testing process.
Resources for Further Reading
- For more details on setting up Docker networks, Docker Networking Overview provides comprehensive guidance.
- If you want to dive deeper into Docker volumes, Docker Volumes Documentation is an excellent resource.
By incorporating the strategies discussed above, you'll equip yourself to handle the complexities of Dockerized SQL databases effectively. Happy coding!