Overcoming Common Pitfalls in Java Microservices Development
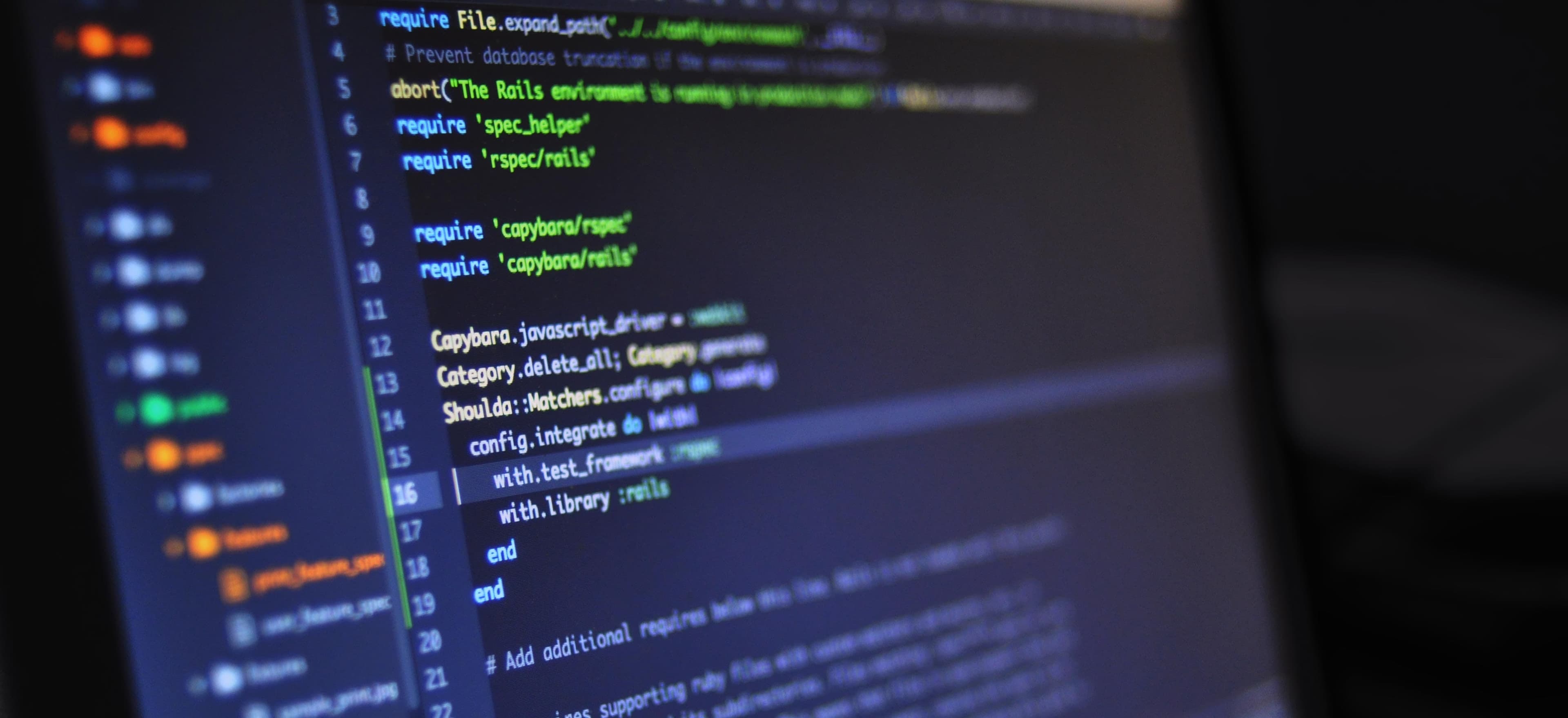
- Published on
Overcoming Common Pitfalls in Java Microservices Development
The rise of microservices architecture has transformed how developers create and deploy applications. Java, with its robust ecosystem and portability, is a popular choice for building microservices. However, as with any architectural style, it comes with its own set of challenges. In this blog post, we will explore some common pitfalls in Java microservices development and how to overcome them, while ensuring that your microservices are scalable, maintainable, and resilient.
Understanding Microservices Architecture
Microservices architecture divides an application into smaller, independent services that communicate explicitly with each other over well-defined APIs. This decentralization offers numerous benefits, including easier scaling, independent deployments, and enhanced fault isolation.
Yet, the move to microservices isn't without its hurdles. Below, we’ll look at common pitfalls and effective strategies to address them.
1. Poorly Defined Service Boundaries
One of the most common pitfalls in microservices development is having poorly defined service boundaries. When services are not clearly delineated, it can lead to tightly coupled systems, negating the benefits of microservices architecture.
Solution: Start with Domain-Driven Design (DDD)
Using DDD helps to identify natural boundaries within your domain. It emphasizes understanding the business requirements and how they can be encapsulated in microservices.
Example Code Snippet
// Sample DDD structure showcasing bounded context
public class OrderService {
private final OrderRepository orderRepository;
public OrderService(OrderRepository orderRepository) {
this.orderRepository = orderRepository;
}
public Order createOrder(Order order) {
// business logic for creating an order
return orderRepository.save(order);
}
}
// Explains why: The OrderService represents a clear boundary.
// Order-related functionality stays encapsulated within this service.
2. Synchronous Communication Between Services
Many developers new to microservices default to synchronous communication, such as HTTP or REST calls. While this may seem straightforward, it can lead to cascading failures when one service is down, resulting in a poor user experience.
Solution: Implement Asynchronous Communication
Asynchronous communication can help decouple services and improve resilience. Using message brokers like RabbitMQ or Kafka allows services to communicate without depending on the availability of one another.
Example Code Snippet
// Example of sending a message to a queue using Spring AMQP
@Autowired
private RabbitTemplate rabbitTemplate;
public void sendOrderConfirmation(OrderConfirmation confirmation) {
rabbitTemplate.convertAndSend("order.confirmation.queue", confirmation);
}
// Why? This sends the confirmation message independently, allowing the sending service to move on without waiting for the receiver's success response.
3. Underestimating Data Management Complexity
When transitioning from a monolithic to a microservices architecture, many teams overlook the complexity of data management. Transitioning to multiple databases can confuse data consistency and transaction management.
Solution: Use the Database per Service Pattern
Each microservice should manage its own database. This ensures that the services are truly independent. Use event sourcing or CQRS (Command Query Responsibility Segregation) for better data handling.
Example Code Snippet
// Sample code employing a separate database for a customer microservice
@Entity
public class Customer {
@Id
private Long id;
private String name;
// Getters and Setters
}
@Repository
public interface CustomerRepository extends JpaRepository<Customer, Long> {}
// Why? This isolates the customer data and ensures that changes do not affect other microservices directly.
4. Ignoring Logging and Monitoring
In a microservices architecture, managing and debugging can become a nightmare if logging and monitoring are not adequately planned. It can be challenging to trace requests through multiple services without a cohesive logging strategy.
Solution: Centralized Logging and Distributed Tracing
Implement centralized logging with tools like ELK Stack (Elasticsearch, Logstash, and Kibana) or Splunk to track logs from all services in one place. For distributed tracing, consider using OpenTelemetry or Zipkin to visualize the flow of requests and identify bottlenecks.
5. Security Concerns
Microservices often introduce new security challenges, especially when they communicate over a network. Ensuring data protection and securing communication channels is pivotal.
Solution: Apply Security Best Practices
Implement security measures like OAuth2 for authentication and HTTPS for secure communication. Each service should authenticate requests independently to maintain security integrity.
Example Code Snippet
// Configure security in Spring Boot
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/public/**").permitAll()
.anyRequest().authenticated()
.and().oauth2Login();
}
}
// Why? This enables secured endpoints that require authentication for access, thereby improving overall application security.
6. Lack of API Gateway
Not using an API Gateway can lead to the exposure of multiple endpoints for consumers, making maintenance tricky. It can also expose the services directly, risking security and version handling.
Solution: Implement an API Gateway
An API Gateway can streamline requests, handle authentication, and route traffic to the appropriate services. Tools like Spring Cloud Gateway or Zuul are great choices.
Example Code Snippet
// Basic setup of an API Gateway using Spring Cloud Gateway
@EnableGateway
public class GatewayConfig {
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("order-service", r -> r.path("/orders/**")
.uri("lb://order-service"))
.build();
}
}
// Why? Using an API Gateway simplifies access for clients and promotes easier service version management.
In Conclusion, Here is What Matters
Java microservices development presents exciting opportunities but also many challenges. By recognizing and addressing these common pitfalls, you can create a more resilient and effective microservices architecture.
Utilizing tools specific to the cloud-native ecosystem, focusing on best practices like DDD and asynchronous communication, enforcing security protocols, and implementing proper logging and monitoring will ensure that your microservices run smoothly.
For more insights into microservices design patterns, check out Microservices.io or explore the resources from Spring Cloud.
In the continuously evolving landscape of software development, staying equipped with the right knowledge and tools will help you harness the power of Java microservices effectively.
Checkout our other articles