Mastering Java: Avoiding Pitfalls When Splitting Strings
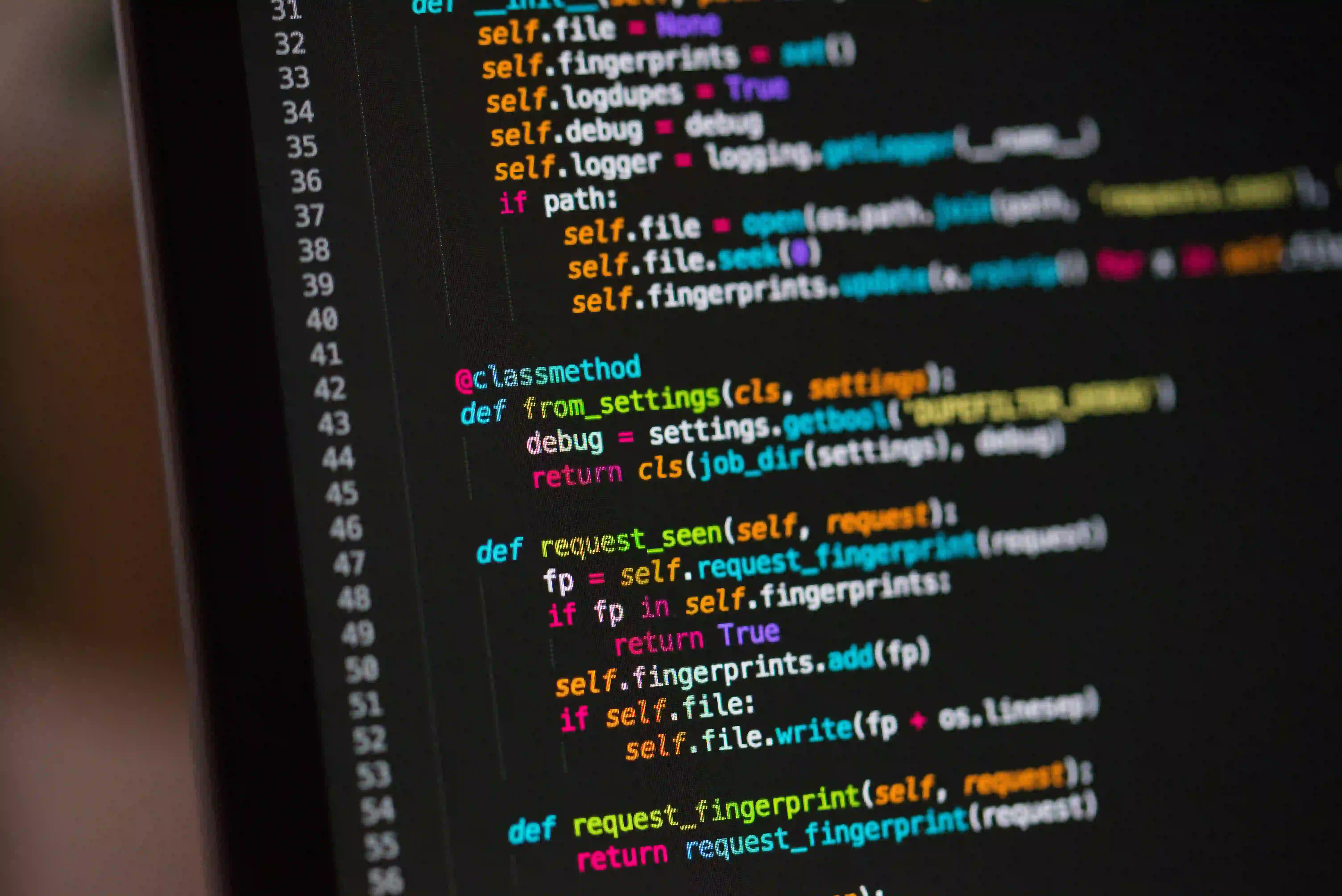
Mastering Java: Avoiding Pitfalls When Splitting Strings
Java is a powerful programming language that is widely used in various fields, including web development, app development, and enterprise solutions. One common task developers encounter is working with strings. Specifically, splitting strings into substrings is a frequent requirement. However, pitfalls may arise during this process if one is not careful. In this blog post, we will explore common pitfalls, best practices, and example code snippets to help you master splitting strings in Java.
Why Split Strings?
Before we dive into the intricacies of splitting strings, let's briefly discuss why we need to do this. In many programming scenarios, you may receive data in a single string format that needs to be broken down for easier manipulation or processing. This can include anything from parsing CSV files to handling user input. The primary method for splitting strings in Java is the split()
method provided by the String
class.
Basic Usage of split()
The split()
method takes a regular expression as an argument and splits the string based on the given delimiter. Here's a simple use case:
String text = "apple,banana,cherry";
String[] fruits = text.split(",");
// Output each fruit
for (String fruit : fruits) {
System.out.println(fruit);
}
Output:
apple
banana
cherry
In this example, we split a string of fruits by the comma delimiter. The result is an array of substrings, and it's straightforward to iterate through and print each element.
Common Pitfalls When Splitting Strings
Splitting strings may seem simple, but there are several common pitfalls to be aware of:
1. Misunderstanding Regular Expressions
The split()
method uses regular expressions, and misunderstanding them can lead to unexpected results. For example, consider splitting a string by a period:
String sentence = "Hello. How are you? I hope you're well.";
String[] parts = sentence.split(". ");
Issue: This code will not work as expected. The dot .
is a special character in regular expressions that matches any character. Therefore, the split will occur on every character.
Solution: To escape the dot, use a double backslash:
String[] parts = sentence.split("\\. "); // Correct way to split
2. Losing Trailing Empty Strings
If your string ends with the delimiter and you need to retain trailing empty strings in the resulting array, you might face challenges since split()
excludes them by default.
String csv = "apple,,banana,";
String[] items = csv.split(",");
// Length of the array is 3, not 4
System.out.println(items.length); // Outputs: 3
Solution: Use the overloaded version of split()
that takes a second argument indicating the limit:
String[] items = csv.split(",", -1); // -1 retains trailing empty strings
System.out.println(items.length); // Outputs: 4
3. Performance Issues with Large Strings
When working with large strings, repeated calls to split()
can lead to performance issues due to the overhead of regular expression processing. Consider alternatives like the StringTokenizer
class or manual splitting using indexOf()
and substring()
.
StringTokenizer tokenizer = new StringTokenizer(bigString, ",");
while (tokenizer.hasMoreTokens()) {
System.out.println(tokenizer.nextToken());
}
While StringTokenizer
is less flexible compared to regex, it can provide better performance in specific use cases.
4. Splitting Multi-Dimensional Data
When dealing with more complex data structures such as multidimensional strings, you might encounter challenges if you try to apply a single delimiter split.
String data = "apple;banana;orange|grape;melon|peach";
String[] rows = data.split("\\|"); // Split rows
Now, if you want to split the items within each row:
for (String row : rows) {
String[] fruits = row.split(";"); // Split columns in each row
for (String fruit : fruits) {
System.out.println(fruit);
}
}
Here, the first split creates an array of rows, and the second one processes each row to yield individual items.
5. Handling Input from Different Sources
Input strings can come from different sources, each with potential inconsistencies in formatting. When your application receives inputs from user forms or CSV uploads, you may encounter leading/trailing spaces or unexpected characters.
String input = " apple, banana , cherry ";
String[] fruits = input.trim().split("\\s*,\\s*"); // Trim spaces around delimiters
for (String fruit : fruits) {
System.out.println(fruit);
}
This trick uses regex to trim spaces before and after the delimiter, enhancing the robustness of your string-splitting logic.
Best Practices for Splitting Strings in Java
To ensure efficient string splitting and avoid common pitfalls, you can follow these best practices:
-
Understand Regular Expressions: Familiarize yourself with regular expression syntax. This knowledge can save you from many potential issues.
-
Use Limits Wisely: When calling
split()
, use the limit parameter to control how many times the string should be split. -
Consider Performance: For performance-critical applications, consider the alternatives like
StringTokenizer
or manual processing instead of using regex. -
Enhance Input Handling: Implement input sanitization techniques to handle unexpected spaces or characters effectively.
-
Test Extensively: Create test cases that cover a wide range of input scenarios, particularly corner cases like empty strings, strings ending with delimiters, and strings with inconsistent spacing.
My Closing Thoughts on the Matter
String splitting is a critical skill for any Java programmer. By understanding the common pitfalls and best practices discussed in this post, you can improve the robustness and performance of your string manipulation code. Always remember to validate your inputs and choose the right tools for the job.
For further learning about string handling in Java, you can refer to the official Java documentation and insightful tutorials on using regular expressions in Java.
Happy coding, and may you navigate strings in Java like a pro!