Avoiding ClassCastException in Java 8 Streams
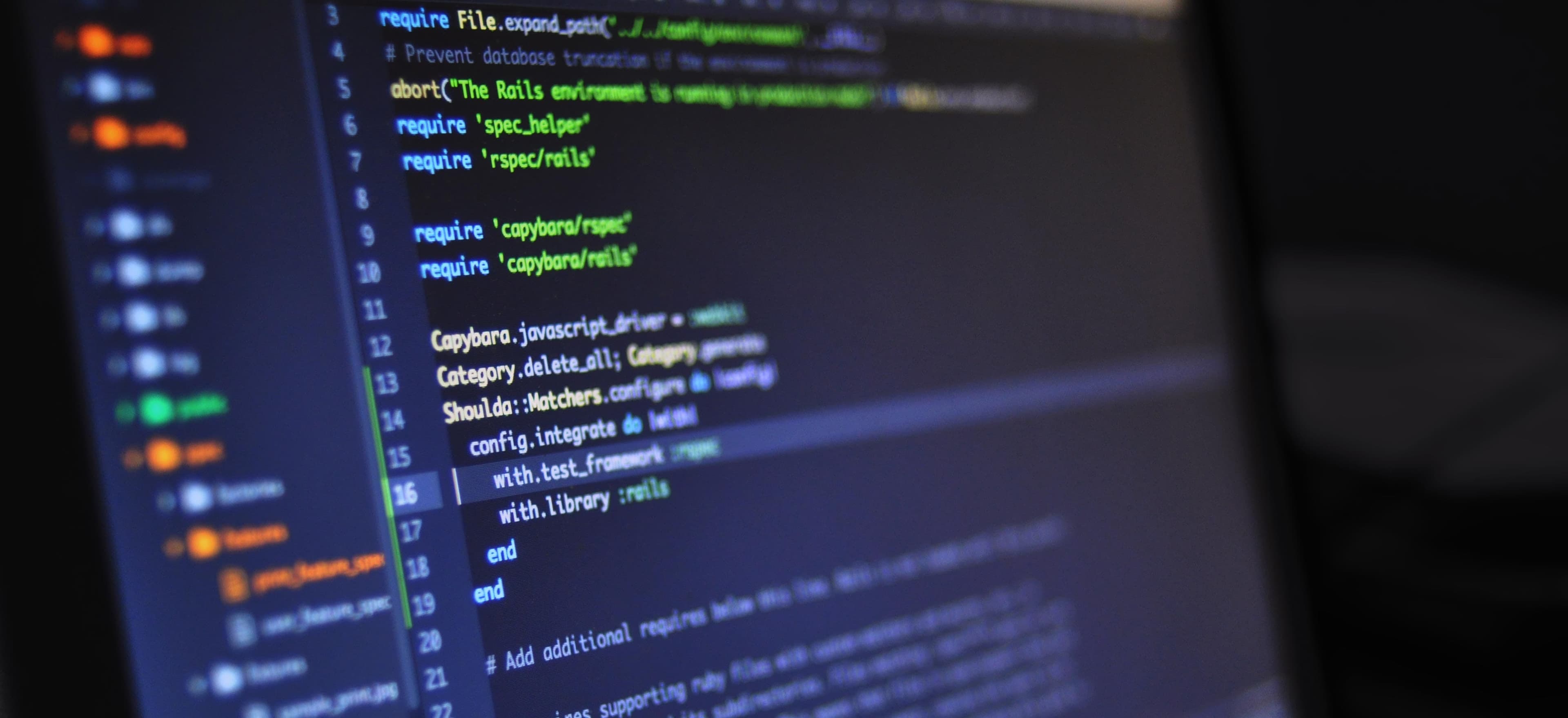
- Published on
Avoiding ClassCastException in Java 8 Streams
Java 8 introduced a powerful feature known as Streams, which allows for functional-style operations on collections of data. While they simplify code significantly, they come with their own set of challenges, one of which is the notorious ClassCastException
. In this blog post, we'll explore how to avoid ClassCastException
when using Java 8 Streams, providing clear explanations and practical examples.
Understanding ClassCastException
ClassCastException
occurs when you attempt to cast an object to a class of which it is not an instance. In the context of Java Streams, this often arises when elements within a stream are of mixed types, leading to unsafe casting. For instance, if you attempt to stream a list of mixed objects and cast them to a specific class without proper checks, you will run into a ClassCastException
.
The Challenge with Mixed Types
Let's consider a simple scenario where we have a list containing elements of different types:
import java.util.Arrays;
import java.util.List;
public class MixedTypesExample {
public static void main(String[] args) {
List<Object> mixedList = Arrays.asList("String", 10, 3.14, new Object());
mixedList.stream().forEach(item -> {
// Attempt to cast item to String
String str = (String) item; // This line can throw ClassCastException
System.out.println(str);
});
}
}
Here, when the stream processes an Integer
or Double
, it will throw a ClassCastException
since they cannot be cast to a String
.
Identifying the Source of ClassCastException
To avoid ClassCastException
, we need to identify which elements in the stream will be cast, and ensure type safety. This can be achieved by using instance checks before casting.
Using the instanceof
Operator
One effective way to prevent ClassCastException
is by utilizing the instanceof
operator to filter elements of the incorrect type before performing any operations.
Here's an improved version of our earlier example:
import java.util.Arrays;
import java.util.List;
public class SafeCastingExample {
public static void main(String[] args) {
List<Object> mixedList = Arrays.asList("Hello", 42, 3.14, "World");
mixedList.stream()
.filter(item -> item instanceof String) // Filter only Strings
.map(item -> (String) item) // Safe to cast to String
.forEach(System.out::println);
}
}
Explanation
- Filtering: The
filter
method is called to retain only the elements that areinstanceof String
. - Mapping: After ensuring that all remaining elements are
String
instances, we safely cast them. - Output: This method ensures that we only process the desired type, gracefully avoiding any
ClassCastException
.
Leveraging Generics in Stream Operations
Using generic types allows us to maintain type safety at compile time. This becomes particularly useful when working with custom objects.
Example with Generics
Consider the following example where we have a list of a custom class:
import java.util.Arrays;
import java.util.List;
class Person {
String name;
Person(String name) {
this.name = name;
}
@Override
public String toString() {
return name;
}
}
public class PersonStreamExample {
public static void main(String[] args) {
List<Person> personList = Arrays.asList(new Person("Alice"), new Person("Bob"));
personList.stream()
.map(person -> person.name) // Implicitly safe
.forEach(System.out::println);
}
}
Key Takeaways
- Generics maintain type safety and eliminate the risk of casting errors.
- The code remains clean and expressive, focusing on the data transformation desired.
Using Optional for Safety
To further prevent the potential for errors when accessing an object that may be absent, leverage Optional
. This approach adds another layer of safety to your stream operations.
Example with Optional
Let's consider an example involving optional names:
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
public class OptionalStreamExample {
public static void main(String[] args) {
List<Optional<String>> names = Arrays.asList(Optional.of("Alice"), Optional.empty(), Optional.of("Bob"));
names.stream()
.flatMap(Optional::stream) // Only get present names
.forEach(System.out::println);
}
}
Explanation
- Using
flatMap
: This method converts eachOptional
to a stream and flattens the result. Only present values are included, while absent values are safely ignored. - Output: This approach avoids any checks or casts. If the
Optional
is empty, it simply does not appear in the output.
Closing the Chapter
Avoiding ClassCastException
in Java 8 Streams doesn't have to be a daunting task. By implementing checks using instanceof
, using generics, and leveraging Optional
, you can write clean, efficient, and safe code.
In summary:
- Always check the type before casting: Use
instanceof
for safety. - Utilize generics: They provide compile-time type safety.
- Incorporate
Optional
: This helps you manage the absence of values gracefully.
Using these strategies will lead to a more robust Java application and a better programming experience. For further reading on Java Streams and functional programming concepts, consider visiting the official Java documentation.
Feel free to share your thoughts or questions in the comments below. Happy coding!
Checkout our other articles