Overcoming Spring Boot WebSocket Connection Challenges
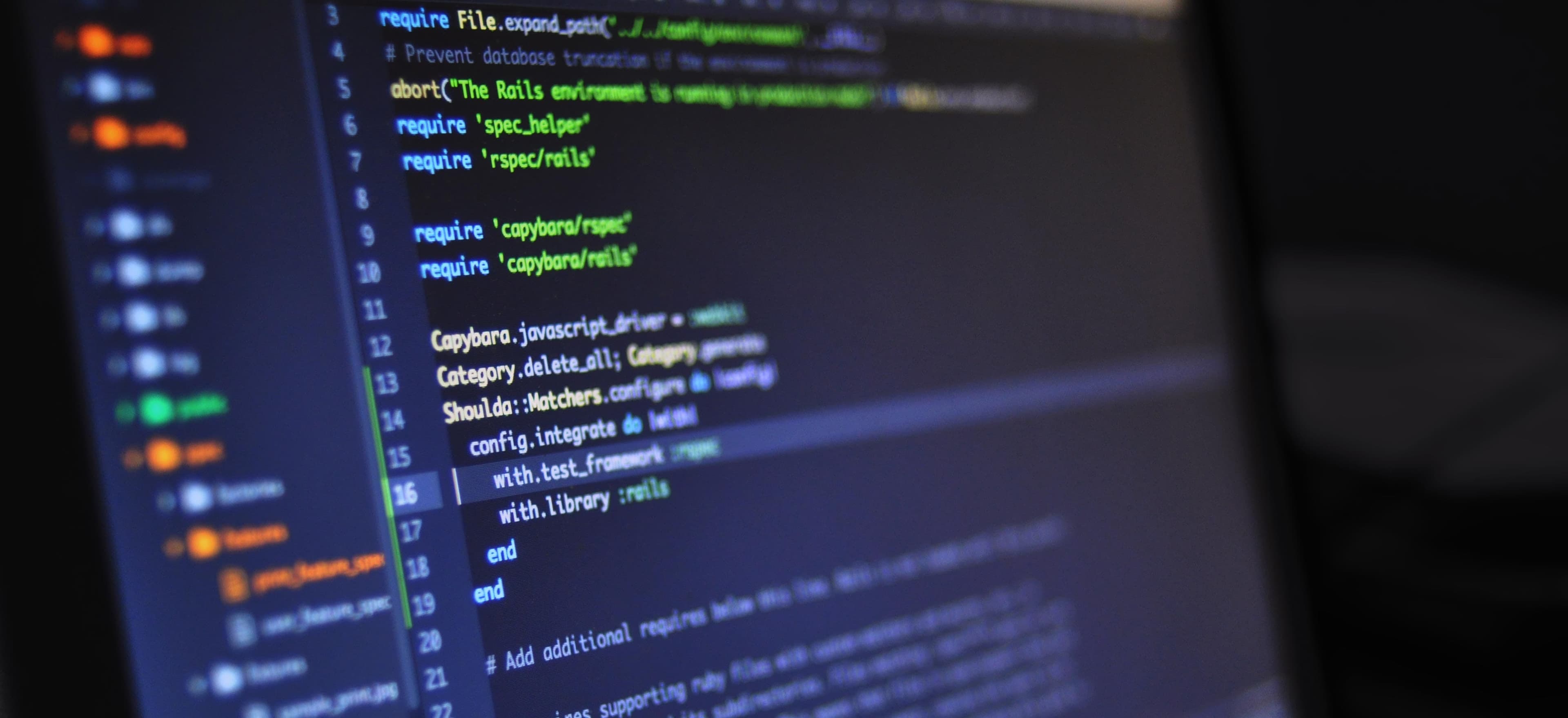
- Published on
Overcoming Spring Boot WebSocket Connection Challenges
WebSockets have become an essential element in modern web application development, especially for real-time data exchange. When working with Spring Boot, creating a WebSocket connection can sometimes present challenges. In this blog post, we will explore common issues developers face when implementing WebSockets in Spring Boot, and we will provide effective strategies for resolving them. We will cover the setup, troubleshooting, and optimization of WebSocket applications.
Understanding WebSockets
Before diving into the challenges, it's crucial to have a basic understanding of what WebSockets are. Unlike traditional HTTP connections, WebSockets provide a persistent connection between client and server, allowing for two-way communication. This means that both the client and server can send messages to one another independently.
For more detailed insights on WebSockets, refer to the MDN Web Docs on WebSockets.
Setting Up a Spring Boot WebSocket Application
To better understand the challenges, let's first set up a basic Spring Boot WebSocket application. This implementation will serve as a foundation for our exploration of connection issues.
Step 1: Project Setup
Create a new Spring Boot project using Spring Initializr with the following dependencies:
- Spring Web
- Spring WebSocket
- Thymeleaf (for the front-end)
After generating the project, you may have a directory structure similar to this:
- src
- main
- java
- com
- example
- websocketdemo
- WebSocketDemoApplication.java
- config
- WebSocketConfig.java
- controller
- ChatController.java
- resources
- templates
- chat.html
Step 2: WebSocket Configuration
Create a configuration class to enable WebSocket support.
package com.example.websocketdemo.config;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.socket.config.annotation.EnableWebSocketMessageBroker;
import org.springframework.web.socket.config.annotation.StompEndpointRegistry;
import org.springframework.web.socket.config.annotation.WebSocketMessageBrokerConfigurer;
@Configuration
@EnableWebSocketMessageBroker
public class WebSocketConfig implements WebSocketMessageBrokerConfigurer {
@Override
public void configureMessageBroker(MessageBrokerRegistry config) {
config.enableSimpleBroker("/topic");
config.setApplicationDestinationPrefixes("/app");
}
@Override
public void registerStompEndpoints(StompEndpointRegistry registry) {
registry.addEndpoint("/chat").withSockJS();
}
}
Why: The WebSocketConfig
class sets up a simple message broker and registers a STOMP (Simple Text Oriented Messaging Protocol) endpoint. The method .withSockJS()
ensures that alternative connection methods are available for browsers that do not support WebSockets.
Step 3: Creating a Chat Controller
Now let’s implement a simple chat controller to handle incoming messages.
package com.example.websocketdemo.controller;
import org.springframework.messaging.handler.annotation.MessageMapping;
import org.springframework.messaging.handler.annotation.SendTo;
import org.springframework.messaging.simp.SimpMessagingTemplate;
import org.springframework.stereotype.Controller;
@Controller
public class ChatController {
private final SimpMessagingTemplate messagingTemplate;
public ChatController(SimpMessagingTemplate messagingTemplate) {
this.messagingTemplate = messagingTemplate;
}
@MessageMapping("/sendMsg")
@SendTo("/topic/messages")
public String sendMessage(String message) {
return message;
}
}
Why: The ChatController
is responsible for routing incoming messages from the client to the appropriate topic. It uses SimpMessagingTemplate
to send messages to the specified destination.
Step 4: Creating the Chat Interface
Finally, create the HTML file to allow users to send and receive messages.
<!DOCTYPE html>
<html>
<head>
<title>WebSocket Chat</title>
<script src="https://cdn.jsdelivr.net/npm/sockjs-client/dist/sockjs.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/stomp.js/2.3.3/stomp.min.js"></script>
</head>
<body>
<div>
<input id="message" type="text" placeholder="Type a message..."/>
<button onclick="sendMessage()">Send</button>
</div>
<div id="chat"></div>
<script>
var stompClient = null;
function connect() {
var socket = new SockJS('/chat');
stompClient = Stomp.over(socket);
stompClient.connect({}, function (frame) {
console.log('Connected: ' + frame);
stompClient.subscribe('/topic/messages', function (message) {
showMessage(JSON.parse(message.body).content);
});
});
}
function sendMessage() {
var messageContent = document.getElementById('message').value;
stompClient.send("/app/sendMsg", {}, JSON.stringify({'content': messageContent}));
}
function showMessage(message) {
var chat = document.getElementById('chat');
chat.innerHTML += "<div>" + message + "</div>";
}
window.onload = connect;
</script>
</body>
</html>
Why: This simple interface creates an input box for the message and a button to send it. It also connects to the WebSocket server and subscribes to the specified topic.
Common WebSocket Connection Challenges
Now that we have a basic WebSocket application, let's discuss some common challenges and how to overcome them.
1. Connection Timeouts
Symptoms: The WebSocket connection fails to establish.
Solution: Ensure that your server is running and accessible. Also, check CORS (Cross-Origin Resource Sharing) configurations. Add the following to your WebSocketConfig
if you plan to use a front-end on a different domain.
@Override
public void registerStompEndpoints(StompEndpointRegistry registry) {
registry.addEndpoint("/chat").setAllowedOrigins("http://localhost:3000").withSockJS();
}
Why: This configuration allows connections from the specified origin. Allowing origins is important for local development or when using different domains for API and frontend.
2. SSL Issues
Symptoms: Errors appearing during HTTPS connection attempts.
Solution: If your application is served over HTTPS, ensure that you are accessing your WebSocket endpoint with the wss://
protocol.
var socket = new SockJS('https://yourdomain.com/chat');
Why: WebSocket connections must utilize secure protocols when the site itself is secure to prevent mixed content issues.
3. Handling Disconnects
Symptoms: Clients unexpectedly being disconnected.
Solution: Implement a reconnection strategy. SOCKJS handles some reconnection attempts automatically, but you might want to extend that functionality.
stompClient.connect({}, function (frame) {
console.log('Connected: ' + frame);
stompClient.subscribe('/topic/messages', function (message) {
showMessage(JSON.parse(message.body).content);
});
}, function (error) {
console.error(error);
setTimeout(function() { connect(); }, 5000);
});
Why: Adding a reconnection strategy helps maintain a seamless user experience during network disruptions.
4. Message Format Issues
Symptoms: Messages do not appear as expected.
Solution: Ensure that your message payloads match the expected format on both the sender and receiver sides. Always validate the structure before sending.
stompClient.send("/app/sendMsg", {}, JSON.stringify({'content': messageContent}));
Why: When the structure does not align, it can lead to message parsing errors. Regular structure checks can prevent these problems.
Closing Remarks
Building and maintaining a WebSocket connection with Spring Boot can be fraught with challenges, from connection timeouts to message handling issues. By understanding the core principles and best practices outlined in this post, you can develop a robust and efficient WebSocket application.
If you want more information on advanced WebSocket configurations and additional options, I recommend checking out the official Spring WebSocket documentation.
Implementing effective error handling and connection management strategies will ensure a smoother experience for your users. Remember, practice makes perfect; experiment with the code and try different configurations to find what works best for your specific use case.
Happy coding!
Checkout our other articles