Why Java's Extension Mechanism Slows Down Your App Startup
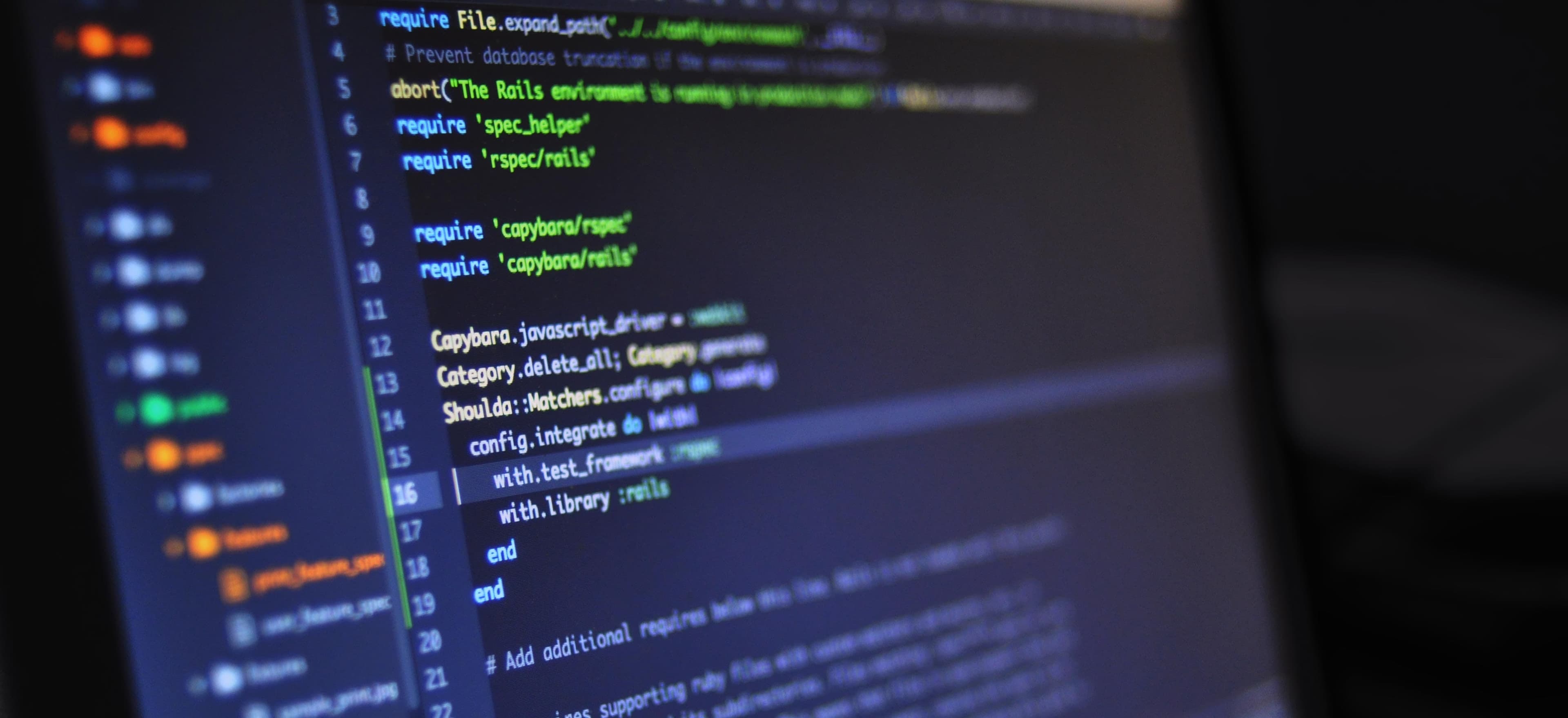
- Published on
Why Java's Extension Mechanism Slows Down Your App Startup
Java is renowned for its robustness, portability, and extensive libraries, making it an ideal choice for many applications. However, one aspect of Java that developers might overlook is how its extension mechanism can adversely affect application startup time. In this blog post, we'll delve into Java's extension mechanism, how it operates, and its impact on your application startup performance.
Understanding Java's Extension Mechanism
Java's extension mechanism allows developers to add new features or libraries to the Java environment without requiring changes to the Java core itself. This is primarily achieved through the use of Java Archive (JAR) files and the lib/ext
directory where these JARs can reside. The Java Virtual Machine (JVM) looks for these JAR files at startup, loading them to enable extended functionalities.
Key Concepts of the Extension Mechanism
-
JAR Files: A JAR file is a compressed package that can contain Java class files, metadata, and resources. They facilitate the distribution of Java applications and libraries.
-
Dynamic Class Loading: The JVM uses dynamic class loading to load classes on demand. However, the JVM also searches through the
lib/ext
folder for extensions at startup. -
Class Loader Hierarchy: Java has a defined class loader hierarchy, which includes the bootstrap class loader, extension class loader, and application class loader. The extension class loader specifically is responsible for loading classes from the extensions directory.
How It Works
Here’s a simplified process of how Java loads extensions:
- Startup Phase: When you start a Java application, the JVM enters a startup phase where it identifies and initializes the necessary classes.
- Extension Loading: During this phase, the JVM scans the
lib/ext
directory (or its equivalent on your platform) to look for any additional JAR files that are marked as extensions. - Class Search: Each JAR file is parsed, and classes contained within are loaded into memory upon their first usage. This can lead to a lengthy startup process, especially if there are many extensions.
The Startup Delay Explained
The extension mechanism introduces several overheads that can slow down application startup:
1. Time-Consuming Directory Scans
Every time a Java application starts, the JVM performs directory scans in the lib/ext
folder. The number of JAR files present directly impacts the duration of this scanning process. Each JAR that the JVM encounters must be analyzed, which takes time.
2. Class Resolution Overhead
Once JAR files are found, their classes need to be resolved. If you have many JARs, this can lead to significant delays due to the repeated process of identifying dependencies and checking for class availability.
3. Increased Memory Footprint
More JAR files mean more classes loaded into memory, even if they are not used immediately. This can lead to an increased memory footprint that could slow down performance.
4. Initialization Costs
When classes are loaded for the first time, the Java Virtual Machine executes static initializers and constructors. If these are resource-intensive, they can add considerable delays.
Strategies to Mitigate the Impact
While the extension mechanism is beneficial for modular programming, it is crucial to avoid pitfalls that lead to sluggish startup times. Here are some strategies to mitigate the impact:
1. Minimize the Number of Extensions
Best Practice: Only include essential JARs in the lib/ext
directory. Reducing the number of extensions minimizes the startup scan time.
2. Use Classpath Instead of Extensions
Instead of placing JARs in the lib/ext
directory, consider adding them to your application's classpath. This avoids the overhead of extension scanning and allows for more controlled class loading.
java -cp /path/to/your/app.jar:/path/to/dependency.jar com.example.Main
3. Lazy Initialization of Classes
Only initialize classes as they are needed rather than upfront. Java’s lazy loading can significantly improve startup time.
public class LazyLoader {
private static SomeDependency instance;
public static SomeDependency getInstance() {
if (instance == null) {
instance = new SomeDependency();
}
return instance;
}
}
4. Profile Your Application
Utilize profiling tools (such as VisualVM or JProfiler) to analyze your application startup times, identifying bottlenecks linked to the extension management.
5. Modularize Your Application
Adopt the Java Platform Module System (JPMS), introduced in Java 9, to create modular applications that can reduce startup overhead by allowing you to specify only the required modules.
The Takeaway
Java's extension mechanism offers flexibility and modularity that can be advantageous in many contexts. However, developers must recognize its potential drawbacks regarding application startup performance. By minimizing the use of the extension mechanism, prefer classpath management, and employing best practices like lazy loading and modularization, your application can enjoy rapid startup times essential for user experience.
For more information about optimizing Java applications, you might find the following resources useful:
- Java Performance Tuning Guide
- Java Class Loading
In conclusion, understanding the mechanics behind Java's performance quirks, such as the extension mechanism, is crucial for developers looking to enhance their application's startup efficiency. Implementing these strategies will lead to faster, smoother Java applications and ultimately a better user experience.
Feel free to drop your thoughts or questions in the comments below!