Troubleshooting Dynamic Proxy Issues in Proxied Applications
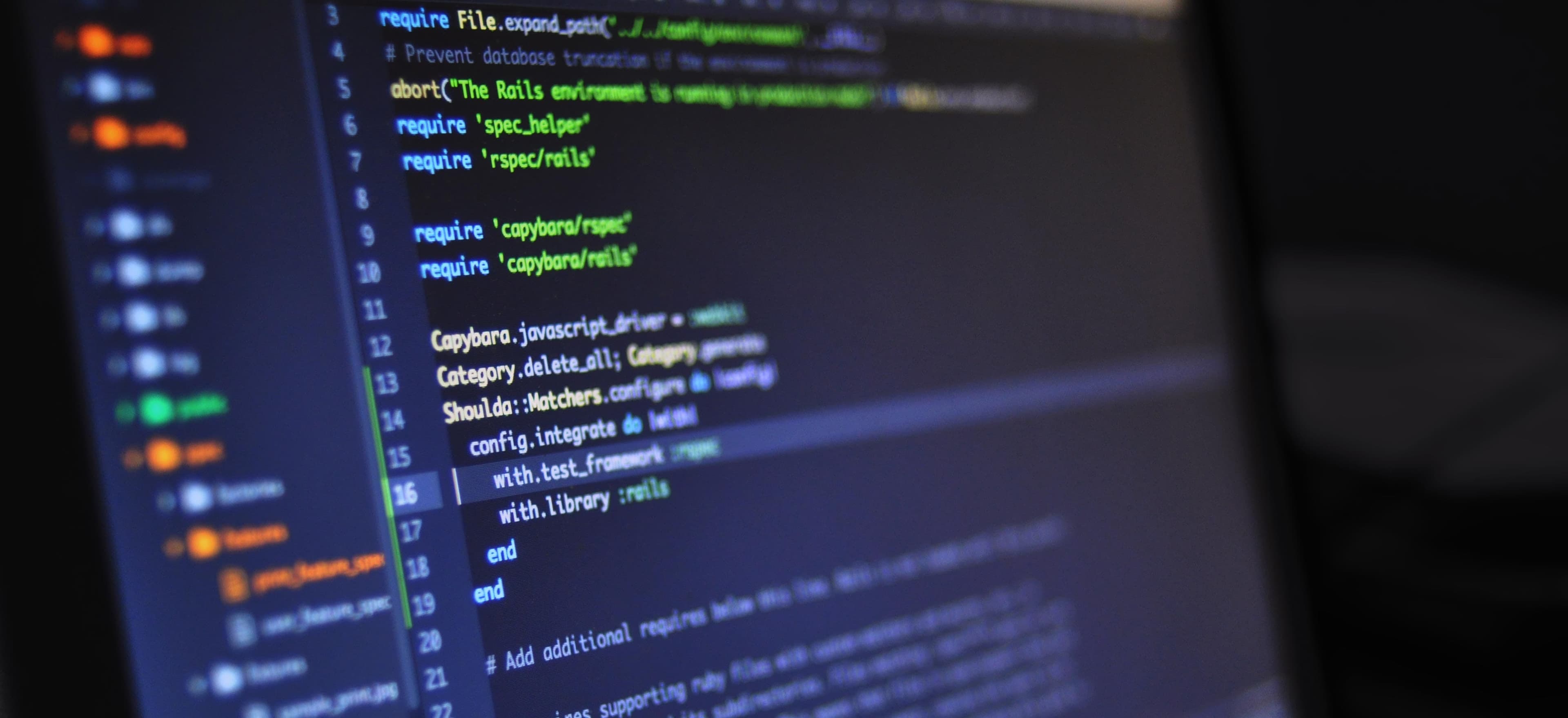
- Published on
Troubleshooting Dynamic Proxy Issues in Proxied Applications
Dynamic proxies are a powerful feature in Java, allowing developers to create proxy instances at runtime. These proxies can wrap original object implementations, offering functionalities such as logging, authentication, and transaction management. However, issues can arise when using dynamic proxies, and troubleshooting these issues requires understanding both the theory and practical application of Java's Proxy functionalities.
Table of Contents
- Understanding Dynamic Proxies
- Common Issues with Dynamic Proxies
- Troubleshooting Steps
- Code Implementation Example
- Best Practices
- Conclusion
Understanding Dynamic Proxies
Java provides a built-in mechanism for creating dynamic proxies through the java.lang.reflect.Proxy
class. A dynamic proxy allows you to create a proxy object that implements one or more interfaces specified at runtime.
How Dynamic Proxies Work
- Interface-Based: The proxy must implement at least one interface.
- Invocation Handler: You must provide an
InvocationHandler
that handles method calls on the proxy instance. The handler defines what happens when methods are invoked on the proxy interface.
For a deeper dive, check out the official documentation here.
Example of Creating a Dynamic Proxy
Here's a basic example of creating a dynamic proxy:
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
interface HelloWorld {
void sayHello();
}
class HelloWorldImpl implements HelloWorld {
public void sayHello() {
System.out.println("Hello, World!");
}
}
class MyInvocationHandler implements InvocationHandler {
private final HelloWorld helloWorld;
public MyInvocationHandler(HelloWorld helloWorld) {
this.helloWorld = helloWorld;
}
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
System.out.println("Before method " + method.getName());
Object result = method.invoke(helloWorld, args);
System.out.println("After method " + method.getName());
return result;
}
}
public class ProxyExample {
public static void main(String[] args) {
HelloWorld helloWorld = new HelloWorldImpl();
InvocationHandler handler = new MyInvocationHandler(helloWorld);
HelloWorld proxyInstance =
(HelloWorld) Proxy.newProxyInstance(
helloWorld.getClass().getClassLoader(),
new Class<?>[]{HelloWorld.class},
handler);
proxyInstance.sayHello();
}
}
In this example, we encapsulate the implementation of the sayHello
method and add pre- and post-method invocations to log when the method is being called. This is a typical use case for proxies, but things can go wrong. Let’s identify those potential issues.
Common Issues with Dynamic Proxies
- ClassCastException: The proxy is not recognized as the instance of the given interface.
- Method Invocation Failing: The invoked method does not exist on the original object.
- Null Pointer Exceptions: Dereferencing a null object can cause issues.
- Performance Overheads: The invocation proxies may cause decreased performance due to additional method calls.
- Security Exceptions: Accessing methods without proper permissions can lead to security exceptions.
Troubleshooting Steps
1. Check Interface Implementation
Ensure the proxy is implemented from an interface. If you mistakenly attempt to cast to a superclass instead of an implemented interface, you will encounter a ClassCastException
.
HelloWorld proxyInstance = (HelloWorld) Proxy.newProxyInstance(
helloWorld.getClass().getClassLoader(),
new Class<?>[]{HelloWorld.class},
handler);
If HelloWorld.class
is not implemented in the casting, this will lead to issues.
2. Validate Method Signatures
Make sure that the method being invoked is present in the interfaces you're working with. For example, invoking a method like proxyInstance.sayGoodbye();
without defining it in the HelloWorld
interface will throw an exception.
3. Check for Null References
Always check for null pointers in your InvocationHandler
implementation to prevent NullPointerException
.
if (helloWorld != null) {
return method.invoke(helloWorld, args);
} else {
throw new NullPointerException("HelloWorld instance is null");
}
4. Monitor Performance
If your application slows down, consider profiling the execution. Adding a proxy layer brings additional overhead. You can consider caching or reducing method call frequency to enhance performance.
5. Handle Security Contexts
Ensure appropriate permissions are granted to your proxy actions. If the proxy is attempting to access private methods or members, it will trigger security exceptions.
Code Implementation Example
Let’s look at an enhanced version of our previous example, this time integrating troubleshooting measures:
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
interface HelloWorld {
void sayHello();
void sayGoodbye();
}
class HelloWorldImpl implements HelloWorld {
public void sayHello() {
System.out.println("Hello, World!");
}
public void sayGoodbye() {
System.out.println("Goodbye, World!");
}
}
class MyInvocationHandler implements InvocationHandler {
private final HelloWorld helloWorld;
public MyInvocationHandler(HelloWorld helloWorld) {
this.helloWorld = helloWorld;
}
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
if (helloWorld == null) {
throw new NullPointerException("HelloWorld instance is null");
}
try {
System.out.println("Before method " + method.getName());
Object result = method.invoke(helloWorld, args);
System.out.println("After method " + method.getName());
return result;
} catch (Exception e) {
System.err.println("Error while invoking method: " + method.getName());
throw e;
}
}
}
public class ProxyExample {
public static void main(String[] args) {
HelloWorld helloWorld = new HelloWorldImpl();
InvocationHandler handler = new MyInvocationHandler(helloWorld);
HelloWorld proxyInstance =
(HelloWorld) Proxy.newProxyInstance(
helloWorld.getClass().getClassLoader(),
new Class<?>[]{HelloWorld.class},
handler);
proxyInstance.sayHello();
proxyInstance.sayGoodbye();
}
}
Key Enhancements
- Null Check: The
helloWorld
instance is checked for nullity to avoidNullPointerExceptions
. - Error Logging: We log errors that occur during invocation, making debugging easier.
Best Practices
- Interface Usage: Use interfaces wisely to ensure the integrity of dynamic proxies.
- Handle Exceptions: Always anticipate possible exceptions and handle them gracefully.
- Keep Proxies Lightweight: Avoid excessive logic in the
InvocationHandler
to ensure the proxy remains efficient. - Document and Test: Clearly document your use of proxies and test extensively, especially with various edge cases.
Bringing It All Together
Dynamic proxies in Java provide flexibility and extendability but are often accompanied by challenges. Understanding how to effectively troubleshoot common issues can significantly enhance the stability and performance of your applications. By recognizing the potential pitfalls and implementing solid patterns, you'll be better equipped to take full advantage of this powerful feature.
For further reading, you might be interested in more about Java Reflection or Java Proxies.
Embrace dynamic proxies and implement them wisely for optimal results in your Java applications!