Mastering API Versioning: Common Pitfalls to Avoid
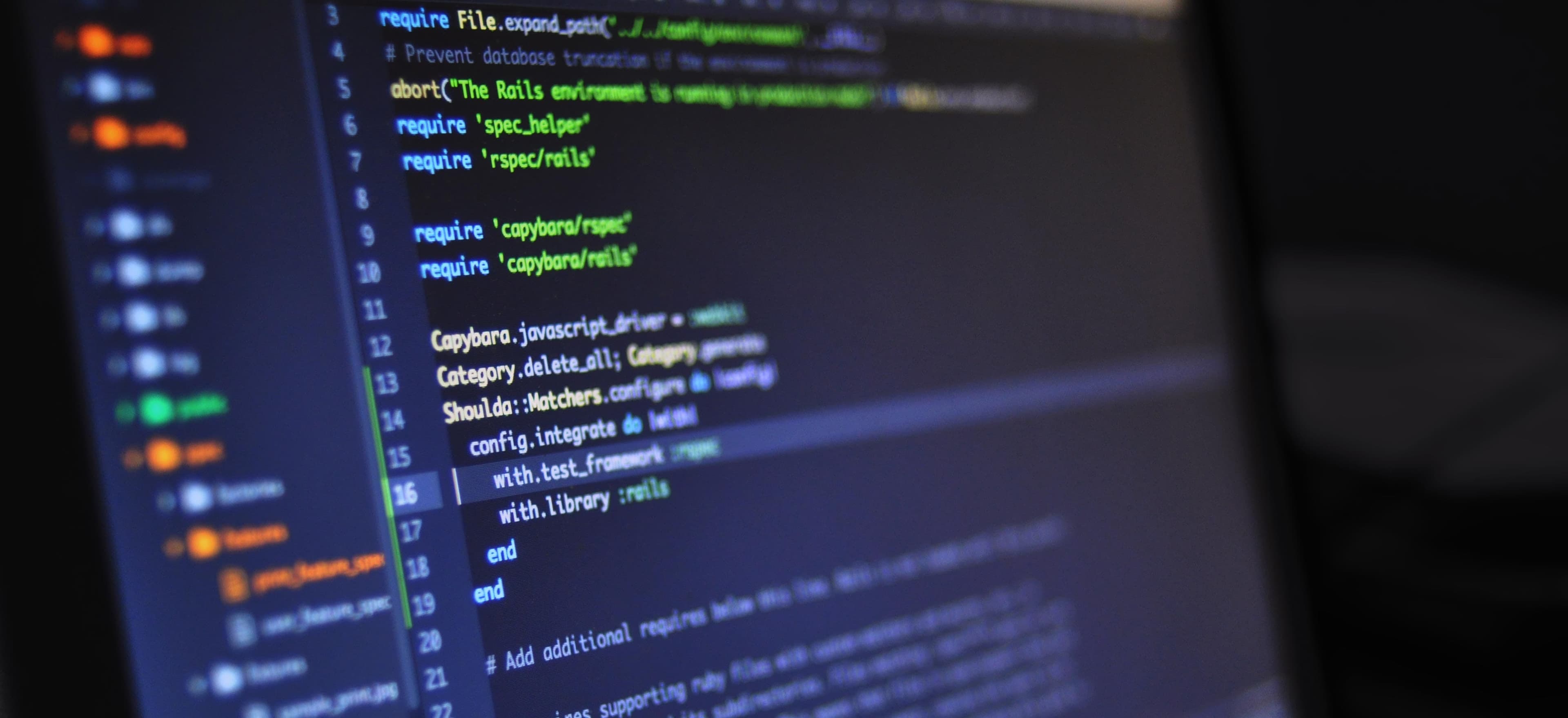
- Published on
Mastering API Versioning: Common Pitfalls to Avoid
Versioning APIs is a fundamental aspect of modern software development. As your application evolves, ensuring backward compatibility while introducing new features can become challenging. In this blog post, we’ll explore the common pitfalls of API versioning and how to avoid them effectively.
Understanding API Versioning
API versioning is the practice of managing changes in your API while maintaining its usability for existing consumers. This ensures that changes don't break existing integrations when new features or modifications are introduced.
Common Pitfalls in API Versioning
Let's delve into some of the common pitfalls that developers encounter when dealing with API versioning.
1. Ignoring the Need for Versioning
Many developers might bypass versioning altogether, believing that their initial API design is sufficient and won't change. However, this can lead to technical debt as the project grows.
Why This is a Pitfall: Ignoring versioning may lead to breaking changes that could disrupt user experience and force integrations to adapt quickly.
Solution: Implement versioning from the start. Even if it seems unnecessary, it lays a foundation for future expansions and adjustments.
2. Choosing the Wrong Versioning Strategy
When it comes to API versioning, there are several strategies available:
- URI Versioning: Including the version in the URL (e.g.,
/api/v1/resource
). - Parameter Versioning: Using a query parameter (e.g.,
/api/resource?version=1
). - Header Versioning: Specifying the version in the request header.
Each has its pros and cons; for example, URI versioning is the most straightforward but can lead to outdated endpoints.
Why This is a Pitfall: If you select a versioning strategy that doesn't fit your API usage patterns or audience, it can create confusion and unnecessary complexity.
Solution: Select a versioning strategy that best suits your development cycle and consumer needs; URI versioning is recommended for most RESTful APIs due to its simplicity.
3. Not Clearly Documenting Version Changes
Version changes should be documented clearly and concisely. When teams don't adequately communicate the alterations between versions, it results in confusion and potential errors when users attempt to adapt to the new versions.
Why This is a Pitfall: Without clear documentation, consumers may struggle to adapt their implementations, leading to frustration and possibly abandonment of your API.
Solution: Adopt a systematic approach to versioning your API documentation. Consider using GitHub Pages or Markdown files to create a changelog that is easy to access and understand.
Example Documentation Approach:
# API Changelog
## Version 1.0
- Initial release
- Endpoints: GET, POST on `/api/v1/resource`
## Version 2.0
- Added pagination to GET endpoint
- Deprecated POST method; recommend using PATCH instead
4. Overusing Versioning
While versioning is crucial, excessive versioning can lead to an overwhelming experience for the users of your API.
Why This is a Pitfall: If every minor change or update results in a new version, it can congest the options your consumers face, making it hard for them to know which version is the best to use.
Solution: Aim to version your API only when necessary – typically for breaking changes or long-lasting features that require significant adjustments.
5. Not Supporting Old Versions
As new versions roll out, it's common to phase out support for older versions. However, this process should be meticulous.
Why This is a Pitfall: Ending support abruptly can disrupt users still relying on the old version, possibly leading to broken integrations.
Solution: Plan deprecation notice strategies. Provide ample time (ideally a grace period of several months) and clear migration paths to newer versions.
Example of a deprecation notice in API documentation:
## Deprecated Version Notice
- Version 1.0 will be deprecated on [insert date].
- Transition to version 2.0 or higher is recommended.
- For help migrating your integration, visit [Migration Guide](link-to-migration-guide).
6. Failing to Consider API Consumers
When designing your API, it is easy to overlook the actual users.
Why This is a Pitfall: If the API design does not meet user requirements, it may result in poor adoption rates, even if the versioning is flawless.
Solution: Engage with your API consumers regularly. Gather feedback continuously and adapt your API based on user experience. User-oriented development usually yields a better-designed API.
7. Neglecting Testing for Each Version
Many developers might test their latest version extensively but forget to verify processes and functionalities of older versions.
Why This is a Pitfall: Inconsistent testing across versions can lead to immeasurable discrepancies, resulting in broken features for older API clients.
Solution: Incorporate automated testing that builds against all supported versions. This ensures that changes do not adversely affect older versions.
@Test
public void testOldVersion() {
ApiClient client = new ApiClient("http://example.com/api/v1/resource");
Response response = client.get();
assertEquals(HttpStatus.OK, response.getStatusCode());
}
Closing Remarks
Versioning your API is not simply a technical requirement; it's a core practice that allows flexibility, sustainability, and user satisfaction. Understanding and avoiding the common pitfalls mentioned above will pave the way for seamless API evolutions and satisfied users.
To further your reading on API versioning and methods to enhance your API design, consider visiting REST API Tutorial for in-depth strategies and best practices.
By following these guidelines, you can design an API that not only delivers features to meet current demands but is also equipped to adapt to future needs. Always remember – successful APIs are those that communicate clearly and operate smoothly across different versions!