How to Minimize Production Bugs in CI/CD Pipelines
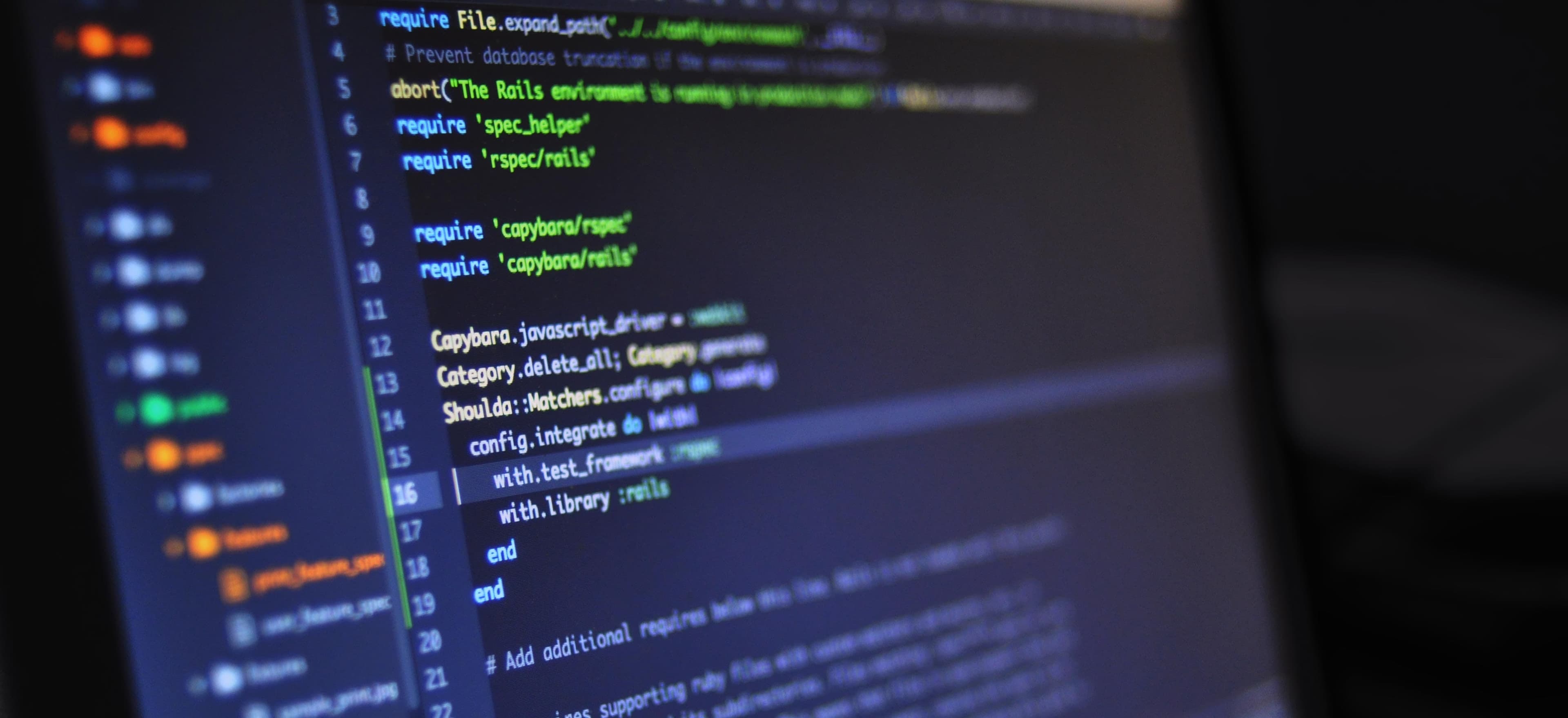
- Published on
How to Minimize Production Bugs in CI/CD Pipelines
Continuous Integration/Continuous Deployment (CI/CD) pipelines play a crucial role in modern software development. They enable teams to deliver software updates quickly and efficiently. However, a poorly configured CI/CD pipeline can lead to production bugs that impact the user experience and can be costly to fix. In this blog post, we’ll explore best practices to minimize production bugs in CI/CD pipelines through a combination of effective automation, thorough testing, and continuous monitoring.
Understanding CI/CD
Before we dive into the solutions for minimizing bugs, let’s briefly understand CI/CD.
-
Continuous Integration (CI) is the practice of merging all code changes into a shared repository several times a day. This enables automated builds and tests to be run on every change, helping to identify errors as soon as they are introduced.
-
Continuous Deployment (CD) extends the automated processes to deployment. Code changes that pass automated tests can automatically be deployed to production environments.
Effective CI/CD pipelines ensure that bugs are caught early in the development cycle.
Common Causes of Bugs in CI/CD
- Inadequate Testing: Relying solely on manual testing can lead to missed defects. Automated unit tests or integration tests can help catch these issues earlier.
- Poor Code Quality: Code that has not been properly reviewed can introduce new bugs. Implementing code reviews can help mitigate this risk.
- Environmental Discrepancies: Differences between staging and production environments can lead to unexpected behavior of the application.
- Changes Under Pressure: Hastily made changes often lead to production bugs. Proper change management practices are essential in mitigating this risk.
Best Practices to Minimize Bugs
1. Implement Comprehensive Testing
Testing is the cornerstone of any CI/CD pipeline. Various testing strategies can be employed at different stages:
-
Unit Testing: Tests individual components in isolation. It helps catch errors early in the development phase. In Java, for instance, you can use JUnit for writing unit tests. Here’s an example:
import static org.junit.jupiter.api.Assertions.assertEquals; import org.junit.jupiter.api.Test; public class CalculatorTest { @Test public void testAdd() { Calculator calculator = new Calculator(); assertEquals(5, calculator.add(2, 3), "2 + 3 should equal 5"); } }
Why: This code tests a simple method of the Calculator class to ensure it functions correctly. Catching such bugs does not only improve quality but saves time.
-
Integration Testing: Tests the interaction between multiple components. Tools such as TestNG can assist with this.
-
End-to-End Testing: Simulates user scenarios to validate the application as a whole. Selenium is a popular framework for this purpose.
2. Automate Quality Checks
Automating code reviews and quality checks can significantly reduce the number of bugs. Tools like SonarQube can help analyze code for vulnerabilities and code smells.
Here’s a simple configuration example for a Maven project:
<plugin>
<groupId>org.sonarsource.scanner.maven</groupId>
<artifactId>sonar-maven-plugin</artifactId>
<version>3.9.0.2155</version>
</plugin>
Why: This snippet integrates SonarQube into your Maven project so you can analyze your code quality as part of the build process.
3. Infrastructure as Code (IaC)
Using IaC ensures that your environments (including production) are set up consistently. Tools like Terraform or Ansible help automate the setup. Consider a Terraform example:
provider "aws" {
region = "us-west-2"
}
resource "aws_instance" "web" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
}
Why: This Terraform code allows your team to provision AWS infrastructure using code. It ensures that configurations are reproducible, reducing the chances of discrepancies between environments.
4. Monitor and Rollback
Implement monitoring solutions to detect issues in production. Tools like Prometheus and Grafana provide immense help. Here is how to set up basic Prometheus monitoring:
job_name: 'java-app'
static_configs:
- targets: ['localhost:8080']
Why: This configuration tells Prometheus to scrape metrics from your Java application. Monitoring allows for quicker detection and response to issues that do make it to production.
Additionally, ensure you have a robust rollback mechanism. If a new deployment causes bugs, you should quickly revert to the previous stable release.
5. Maintain a Culture of Collaboration
Finally, create a culture where developers, testers, and operations teams collaborate closely. Daily stand-ups can help to maintain open communication. Encourage team members to share knowledge and to use pair programming for critical components.
In Conclusion, Here is What Matters
Bug-free production is a challenging goal in CI/CD processes. However, by applying these practices consistently, you can mitigate the risks significantly. Implement automated testing, perform quality checks, use Infrastructure as Code, monitor your applications actively, and foster a collaborative team environment.
Reducing production bugs isn’t about eliminating them entirely; it’s about minimizing risk and maintaining a high-quality user experience. Looking towards the future of software development, embracing these practices will pave the way for smoother and more reliable deployments.
For further reading on CI/CD practices, consider exploring the articles from Atlassian or DevOps.com.
Adopting these strategies will empower your team and help ensure that your deployments are as seamless and free of bugs as possible. Thank you for reading, and happy coding!
Checkout our other articles