Overcoming Data Loss with Apache Ignite Native Persistence
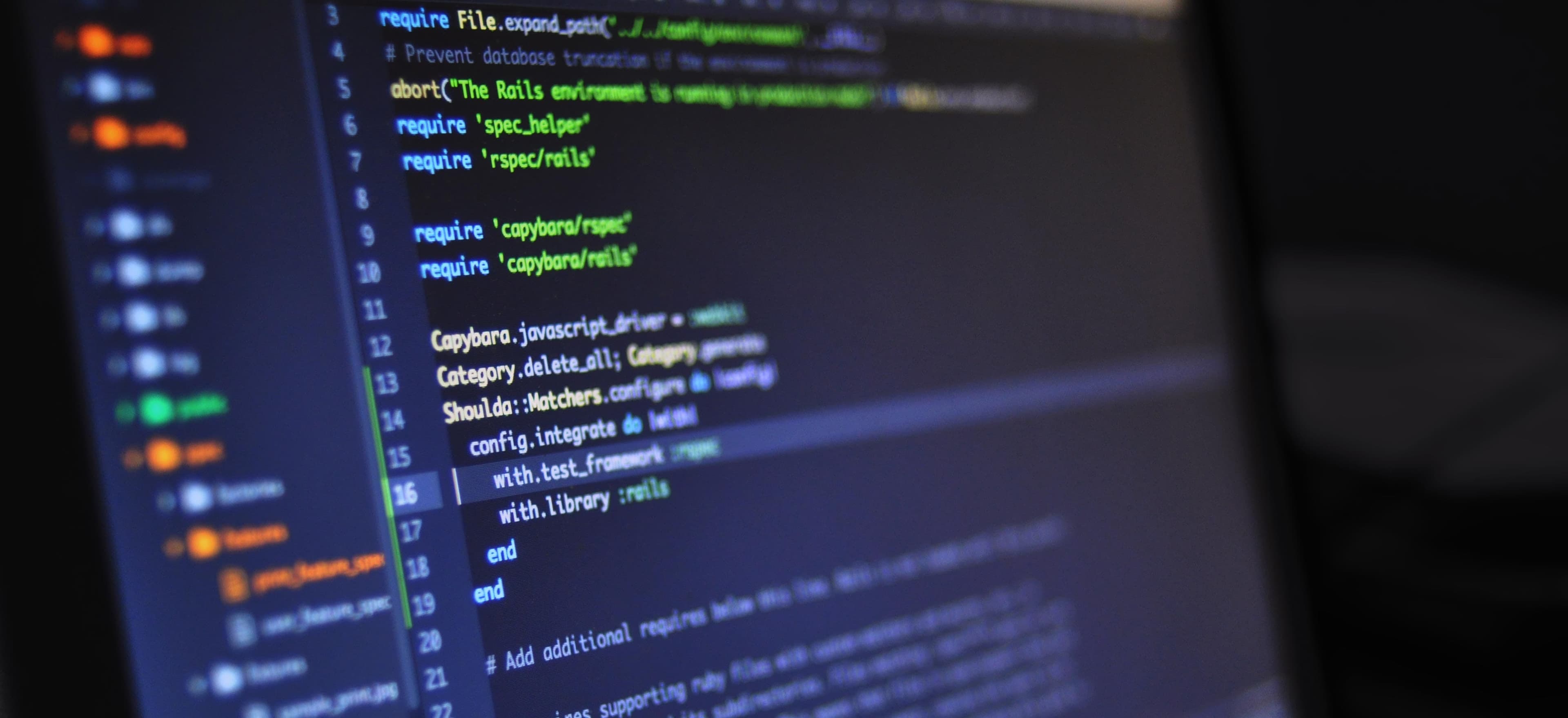
- Published on
Overcoming Data Loss with Apache Ignite Native Persistence
In the dynamic world of data management, data loss is one of the most dire issues that developers and IT teams face. Data can be lost due to system failures, application bugs, or even human errors. Fortunately, powerful solutions exist that can help prevent data loss. One such solution is Apache Ignite, an in-memory computing platform that provides a suite of capabilities, including Native Persistence. This blog post delves into how Apache Ignite can help overcome data loss through its native persistence feature.
What is Apache Ignite?
Apache Ignite is an open-source distributed database, caching, and processing platform designed to handle large-scale applications. It provides in-memory data storage which significantly enhances performance, making it useful for applications requiring high speed and low latency.
Key Features of Apache Ignite
- In-Memory Computing: Achieves faster data access and processing times.
- Distributed Architecture: Provides scalability and reliability.
- SQL Support: Offers robust query capabilities.
- Compute Grid: Supports distributed computing to execute tasks across cluster nodes.
- Native Persistence: Ensures data durability and recovery.
Understanding Apache Ignite Native Persistence
Native Persistence is a groundbreaking feature in Apache Ignite that allows the platform to store the data in a native format on disk, while still providing the advantages of in-memory computing. It is often a requirement for applications that demand high availability and durability of data.
Why Use Native Persistence?
- Data Durability: It protects against data loss from unexpected failures by storing data on disk.
- Fast Recovery: In the event of a failure, Ignite can quickly restore data from disk, allowing for less downtime.
- Memory Efficiency: Native Persistence enables an efficient use of memory, allowing you to scale your cluster without memory constraints.
- Hybrid Storage: You can manage both in-memory and on-disk data seamlessly.
Setting up Apache Ignite with Native Persistence
Let’s dive into practical steps to configure Apache Ignite with Native Persistence enabled.
Step 1: Adding Maven Dependencies
If you are using Maven for your project dependencies, first, include the following in your pom.xml
file:
<dependencies>
<dependency>
<groupId>org.apache.ignite</groupId>
<artifactId>ignite-core</artifactId>
<version>2.13.0</version>
</dependency>
<dependency>
<groupId>org.apache.ignite</groupId>
<artifactId>ignite-spring</artifactId>
<version>2.13.0</version>
</dependency>
</dependencies>
Step 2: Configure Ignite with Native Persistence
Next, you need to configure Ignite to use its native persistence. The configuration can be done via an XML file or programmatically.
Below is an example of how to configure it programmatically:
import org.apache.ignite.Ignite;
import org.apache.ignite.Ignition;
import org.apache.ignite.configuration.IgniteConfiguration;
import org.apache.ignite.configuration.DataStorageConfiguration;
import org.apache.ignite.configuration.CacheConfiguration;
public class IgniteNativePersistence {
public static void main(String[] args) {
// Configure Ignite instance with Native Persistence
IgniteConfiguration cfg = new IgniteConfiguration();
// Enable Native Persistence
DataStorageConfiguration storageCfg = new DataStorageConfiguration();
storageCfg.setPersistenceEnabled(true);
cfg.setDataStorageConfiguration(storageCfg);
// Configure Cache
CacheConfiguration<Integer, String> cacheCfg = new CacheConfiguration<>("CacheName");
cacheCfg.setIndexedTypes(Integer.class, String.class);
cfg.setCacheConfiguration(cacheCfg);
// Start Ignite
try (Ignite ignite = Ignition.start(cfg)) {
System.out.println("Ignite instance started with Native Persistence enabled.");
}
}
}
Explanation of the Code
- DataStorageConfiguration: This configuration allows you to enable native persistence. By calling
setPersistenceEnabled(true)
, data will be stored on disk. - CacheConfiguration: Here we define a cache with an indexed type. This configures how Ignite will cache data in memory.
- Ignite Start: The Ignite instance is started with the configured settings, which includes our persistence settings.
Data Operations with Native Persistence
Once Ignite is set up, you can perform basic data operations that will be persisted to disk. Below is an example of how to put and retrieve data from your cache.
import org.apache.ignite.Ignite;
import org.apache.ignite.Ignition;
import org.apache.ignite.cache.CacheEntryProcessor;
import org.apache.ignite.configuration.CacheConfiguration;
// Assuming Ignite has been started with Native Persistence enabled
public class IgniteDataOperations {
public static void main(String[] args) {
try (Ignite ignite = Ignition.start()) {
// Getting the cache
var cache = ignite.cache("CacheName");
// Storing data
cache.put(1, "Hello, Ignite!");
// Retrieving data
String value = cache.get(1);
System.out.println("Retrieved Value: " + value);
}
}
}
Commentary on Data Operations
- Put Operation: The
put
method stores data in the cache, which will persist it to disk due to our earlier configuration. - Get Operation: The
get
method retrieves the value associated with the specified key, demonstrating the ability to perform standard operations as if the data were only in-memory.
Advantages of Apache Ignite Native Persistence
Using Apache Ignite Native Persistence not only protects against data loss but provides a plethora of advantages:
- High Availability: Applications can recover quickly from crashes.
- Efficient Resource Usage: Leverages disk storage to manage larger datasets beyond memory limits.
- Seamless Queries: Enables full SQL capabilities even on persisted data.
For further reading about Apache Ignite and its capabilities, you can visit their official documentation.
Final Considerations
In a world that increasingly depends on data, ensuring its safety is crucial for any business. Apache Ignite's Native Persistence feature offers an elegant solution for overcoming data loss, safeguarding both in-memory and on-disk data.
By following the setup process outlined in this blog, you can effectively implement Ignite in your application and take full advantage of its powerful features. Whether you're managing large datasets or need high-speed access, Ignite with Native Persistence is worth considering.
Remember, a robust data management strategy is key to preventing data loss, and Apache Ignite is a strong contender in that space.
Now that you're equipped with the foundational knowledge, consider implementing these strategies in your next project!