Understanding Java's Equals Method: Common Pitfalls Explained
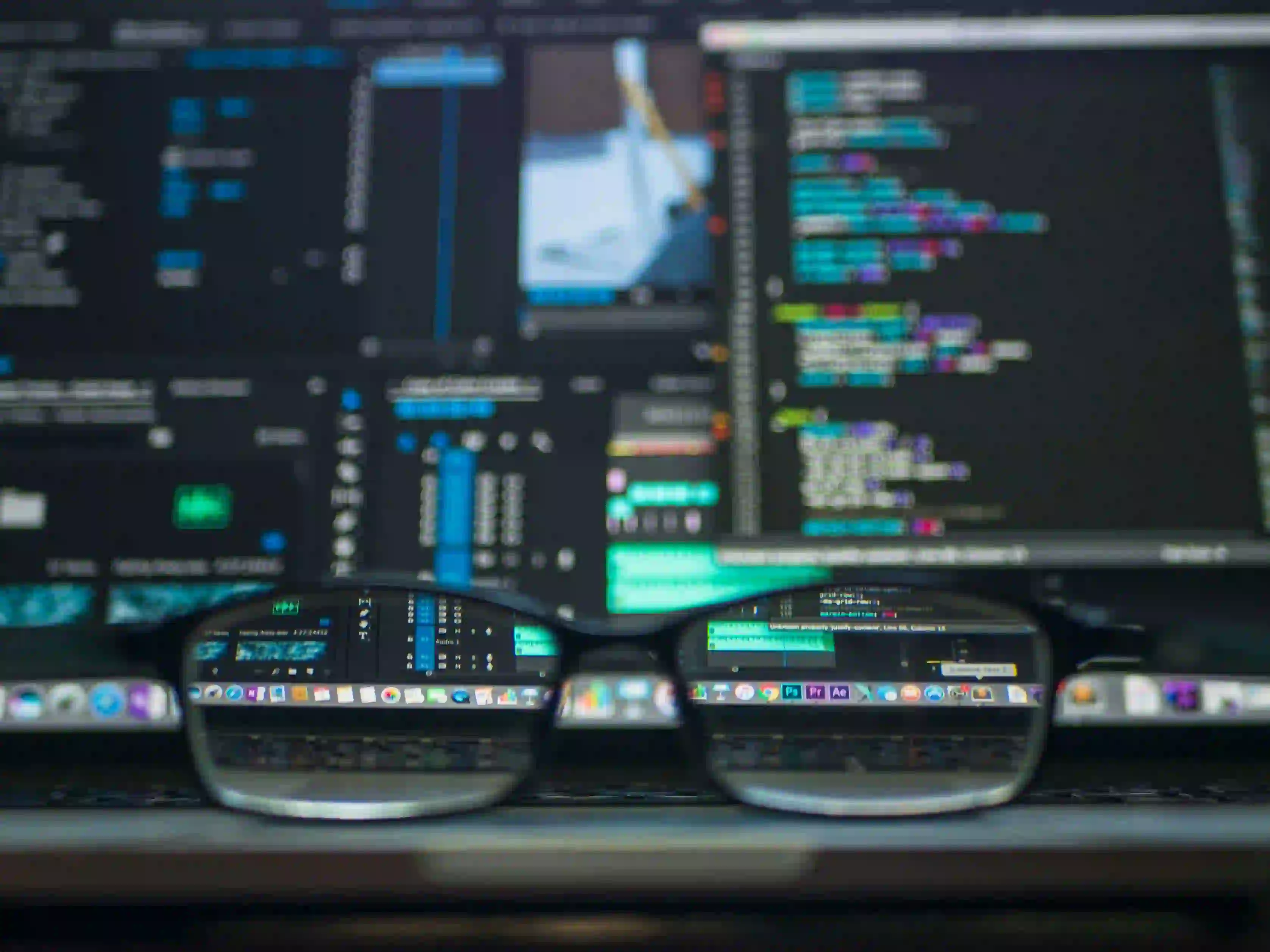
Understanding Java's Equals Method: Common Pitfalls Explained
In the realm of Java programming, the equals()
method is an essential feature that plays a significant role in object comparison. It's vital for ensuring that your objects are compared in a meaningful way. However, misuse or misunderstanding can lead to unpredictable behavior and subtle bugs. In this blog post, we will dive deep into Java's equals()
method, explore common pitfalls, and offer clear solutions.
What is the Equals Method?
The equals()
method is defined in the Object
class, and it determines whether two objects are "equal" in terms of content. By default, Object
's equals()
method checks if both references point to the same object, which is not always what developers intend.
The Default Behavior
Here is the default implementation in the Object
class:
public boolean equals(Object obj) {
return this == obj;
}
The default method uses the ==
operator, which tests for reference equality, not logial equality.
Why Override the Equals Method?
When you create custom objects and intend for them to have logical equality based on their fields, you need to override the equals()
method. For example, if you have a Person
class with name
and age
fields, two Person
objects should be considered equal if their names and ages are the same.
Implementing the Equals Method Properly
To correctly override the equals()
method, follow the best practices:
- Use the
@Override
annotation to indicate that this method overrides a superclass method. - Check if the object is the same instance using
==
. - Check if the object is of the correct type using
instanceof
. - Cast the object to the appropriate type.
- Compare the relevant fields for equality.
Here’s an example for a Person
class:
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true; // Step 1: Check for reference equality
}
if (!(obj instanceof Person)) {
return false; // Step 2: Check type
}
Person other = (Person) obj; // Step 3: Cast to Person
// Step 4: Compare fields
return this.name.equals(other.name) && this.age == other.age;
}
}
Commentary on the Implementation
- Reference Equality: The first
if
statement checks if both references point to the same object, which is a fast path for equality. - Type Check: Using
instanceof
is critical to avoidClassCastException
. - Field Comparison: Finally, we check both relevant fields, ensuring logical equality instead of mere reference comparison.
Common Pitfalls
Now, let's discuss some common pitfalls developers encounter when working with the equals()
method.
1. Failing to Check for Null
One major pitfall is forgetting to handle null
objects:
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null || getClass() != obj.getClass()) { // Null check added
return false;
}
}
By adding a null check, we ensure that our method is safe against null comparisons.
2. Symmetry Violations
The equals()
method must be symmetric. If a.equals(b)
returns true, then b.equals(a)
should also return true. If you're not careful with your implementation, this could lead to unexpected results.
3. Override hashCode() Method
When you override equals()
, it's crucial to also override hashCode()
. This is particularly important for objects used in hash-based collections like HashMap
and HashSet
.
@Override
public int hashCode() {
return Objects.hash(name, age); // Generates hash code based on properties
}
Not doing so could lead to inconsistencies when storing and retrieving objects from collections.
4. Transitivity Violations
Transitivity implies that if a.equals(b)
and b.equals(c)
are both true, then a.equals(c)
should also be true. This can often trip developers up, particularly in complex class hierarchies.
5. Mutable Fields
If your class has mutable fields, the result of equals()
comparisons can change over time. Here’s an example:
public void setAge(int age) {
this.age = age; // Changing a mutable field
}
If the age
changes after an object is inserted into a HashMap
, retrieving that object by key might yield incorrect results. Consider using immutable classes or defensively copying mutable fields.
Final Thoughts
To summarize, Java’s equals()
method is an essential part of any object-oriented programming paradigm. By understanding how to override it correctly, you can make sure your objects are compared meaningfully.
- Always check for reference equality first.
- Use
instanceof
to confirm the type. - Don’t forget to implement
hashCode()
accordingly. - Be aware of the pitfalls involving null checks, symmetry, and mutable fields.
Additional Resources
If you'd like a deeper understanding of Java's object comparison, consider the following resources:
- Java Documentation on equals() and hashCode()
- Effective Java by Joshua Bloch
- Understanding the Java equals() method
By keeping these guidelines and pitfalls in mind, you can ensure your Java applications handle object comparison accurately and effectively. Happy coding!
The Bottom Line
As we conclude our exploration of the equals()
method, remember that correct implementation strategies are key to building robust Java applications. Misguided object comparisons can lead to wasted time debugging bugs that could have been easily avoided. Embrace these best practices, and you will stand better equipped to write clearer and more dependable Java code.