Mastering PreparedStatement: Repeat Java Strings Dynamically
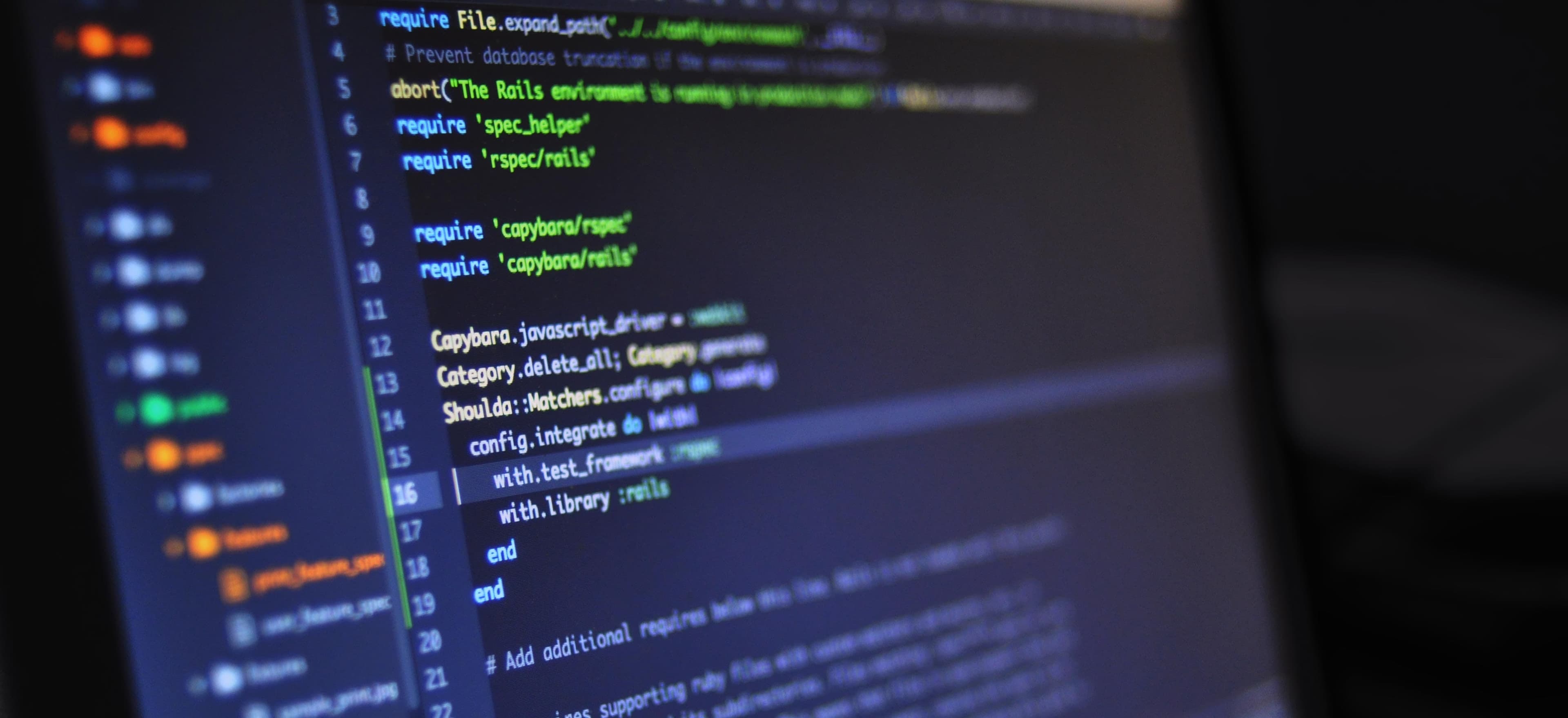
- Published on
Mastering PreparedStatement: Repeat Java Strings Dynamically
When working with databases in Java, one of the most important tools we have at our disposal is the PreparedStatement
. It not only helps to write more organized and maintainable code but also enhances security by preventing SQL injection attacks. In this blog post, we will dive deep into the world of PreparedStatement
, specifically focusing on how to dynamically repeat Java strings in SQL queries.
Table of Contents
- What is PreparedStatement?
- Basic Syntax and Usage
- Dynamically Repeating Strings
- Example Implementation
- Performance Benefits of PreparedStatement
- Conclusion
What is PreparedStatement?
A PreparedStatement
is a feature in Java's JDBC (Java Database Connectivity) that allows you to execute parameterized SQL statements efficiently. Unlike a Statement
, a PreparedStatement
precompiles the SQL query and allows you to set the values of its parameters dynamically.
Key Benefits:
- Performance: The SQL statement is compiled only once.
- Security: Shields against SQL injection attacks.
- Readability: Improves the organization of your code.
For further reading, check out the official Oracle JDBC documentation.
Basic Syntax and Usage
Creating a PreparedStatement
is straightforward. Follow these basic steps:
- Establish a connection to your database.
- Create a
PreparedStatement
using a SQL query with placeholders (?
). - Set parameter values.
- Execute the query.
Here’s a simple example:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class Example {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydb";
String user = "user";
String password = "password";
try (Connection connection = DriverManager.getConnection(url, user, password)) {
String sql = "SELECT * FROM users WHERE username = ?";
try (PreparedStatement preparedStatement = connection.prepareStatement(sql)) {
preparedStatement.setString(1, "john_doe");
// Execute and process the result set
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Explanation:
- We connect to a MySQL database using JDBC.
- A SQL query is defined with a placeholder for the username.
- We fill in the placeholder at runtime with the
setString
method.
Dynamically Repeating Strings
One interesting feature of PreparedStatement
is the ability to dynamically repeat strings. This is especially useful when you need to insert or query lists of values.
In SQL, when you need to insert multiple records or check against multiple values, you might use the IN
clause. However, you can’t directly concatenate strings in SQL; instead, you will generate placeholders dynamically in your Java code.
Example Scenario
Assume you have a list of usernames and you want to construct a query that selects user information for these usernames.
Step-by-Step Process:
- Prepare the usernames in a list.
- Construct the SQL query dynamically by determining the correct number of placeholders based on the list size.
- Set the parameters in the
PreparedStatement
effectively.
Code Snippet:
Below is an example demonstrating this approach.
import java.sql.*;
import java.util.Arrays;
import java.util.List;
public class DynamicRepeatExample {
public static void main(String[] args) {
List<String> usernames = Arrays.asList("john_doe", "jane_smith", "alice_jones");
String url = "jdbc:mysql://localhost:3306/mydb";
String user = "user";
String password = "password";
try (Connection connection = DriverManager.getConnection(url, user, password)) {
String placeholders = String.join(",", "?".repeat(usernames.size()));
String sql = "SELECT * FROM users WHERE username IN (" + placeholders + ")";
try (PreparedStatement preparedStatement = connection.prepareStatement(sql)) {
for (int i = 0; i < usernames.size(); i++) {
preparedStatement.setString(i + 1, usernames.get(i)); // Dynamically set parameters
}
ResultSet resultSet = preparedStatement.executeQuery();
while (resultSet.next()) {
// Process the retrieved data
System.out.println("User ID: " + resultSet.getInt("id") + ", Name: " + resultSet.getString("name"));
}
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Explanation:
-
Dynamic Query Construction: The line
String placeholders = String.join(",", "?".repeat(usernames.size()));
creates a dynamic list of placeholders based on the number of usernames. -
Setting Parameters Dynamically: The loop
for (int i = 0; i < usernames.size(); i++)
iterates over the list of usernames, setting each one in the corresponding placeholder.
Performance Benefits of PreparedStatement
Using PreparedStatement
can significantly enhance performance in several situations, particularly when executing the same query multiple times with different parameters. When a query is prepared, the database optimizes its execution plan, which reduces the overhead for subsequent executions.
Benefits in Performance:
- Reduced Compilation Time: The SQL command is already parsed and compiled.
- Batch Processing: Multiple executions can be handled efficiently without recompiling.
For a more detailed discussion on JDBC performance, you can refer to JDBC Performance Tuning Techniques.
Bringing It All Together
Mastering PreparedStatement
in Java is a valuable skill, especially when it comes to forming dynamic queries effectively and securely. The ability to repeat Java strings dynamically aids in handling complex scenarios, such as inserting multiple records or fetching rows based on multiple conditions.
By following the patterns and examples outlined in this article, you can start implementing dynamic queries in your applications while enjoying the benefits of improved performance and security.
Further Reading
If you would like to explore more about this topic, check out the following resources:
- Java 8 Stream API
- Understanding Java JDBC
Feel free to share your thoughts, questions, or additional examples in the comments below! Happy coding!
Checkout our other articles