Text to Image: Overcoming Spring AI's Common Pitfalls
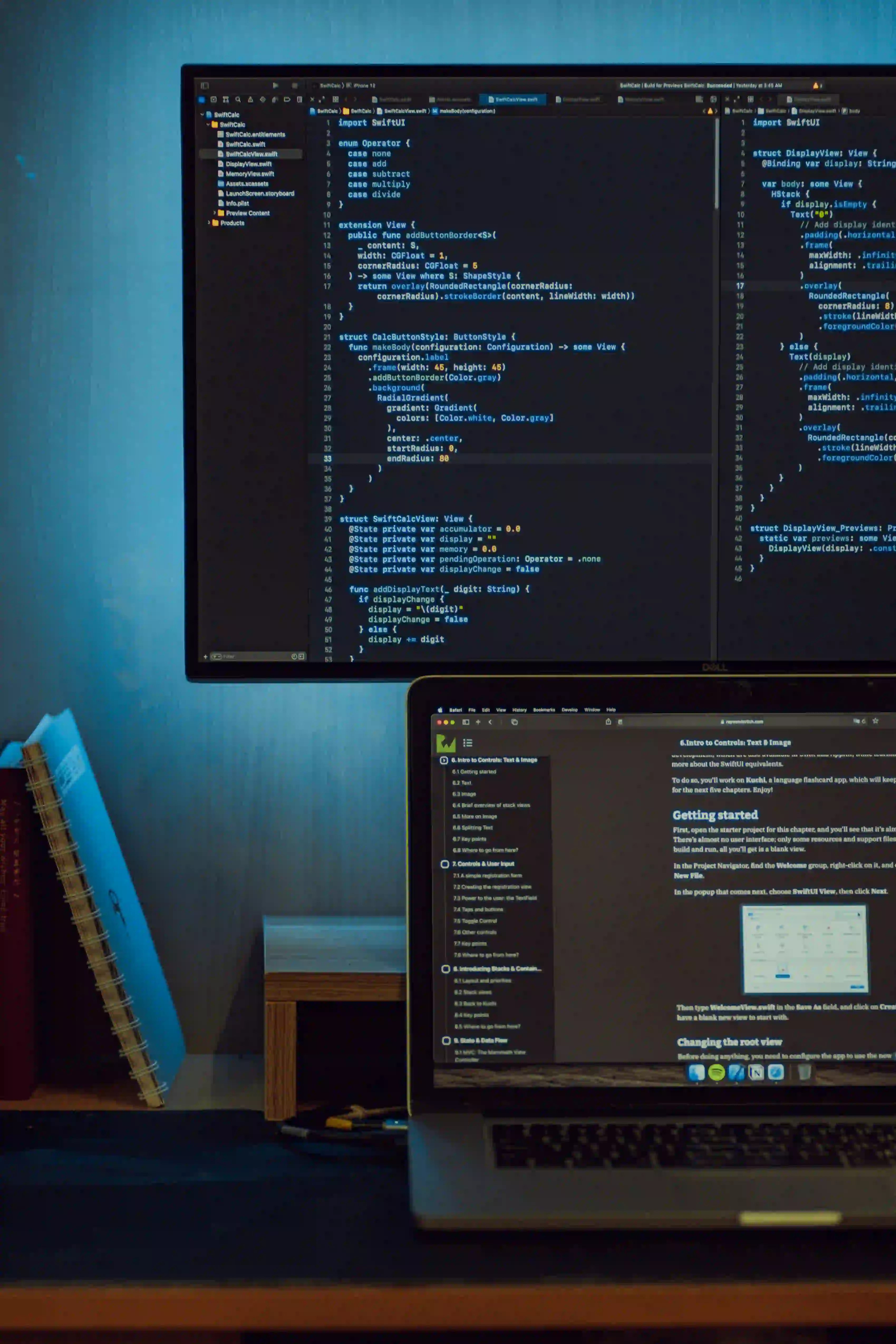
Overcoming Common Pitfalls in Spring AI
Spring AI is a powerful framework that leverages artificial intelligence in Spring Boot applications, enabling developers to enrich their applications with intelligent features. However, like any technology, it comes with its own set of challenges. In this blog post, we will delve into the most common pitfalls encountered when using Spring AI and provide effective strategies to overcome them.
Understanding Spring AI
Before we dive into common pitfalls, let's briefly understand what Spring AI brings to the table. Spring AI integrates machine learning and artificial intelligence capabilities into the Spring ecosystem. This integration allows developers to easily build intelligent applications that utilize pre-trained models, perform data analysis, and more.
Common Pitfalls in Spring AI
1. Improper Dependency Management
One of the first common pitfalls of using Spring AI is related to dependency management. With numerous libraries available in the Spring ecosystem, it can be challenging to manage these dependencies effectively.
Solution
Always use a properly defined pom.xml
or build.gradle
file for Maven or Gradle, respectively. Ensure to include only the necessary dependencies. Here's an example of how you might set up your Maven configuration:
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-core</artifactId>
<version>0.1.0</version>
</dependency>
Using the latest stable version avoids issues with deprecated methods and additional bugs. Regularly check the Spring AI documentation for updates.
2. Model Resource Management
Managing the AI models becomes tricky if not handled properly. Developers often forget to free up resources or make efficient use of CPU/GPU memory.
Solution
Make sure to manage your model resources diligently. Utilize the @PreDestroy
annotation to clean up resources before your bean is destroyed:
@Bean
public Model myModel() {
Model model = new Model.Builder().build();
// Initialize model
return model;
}
@PreDestroy
public void cleanup() {
model.cleanup(); // Free resources
}
By properly managing resources, you can prevent memory leaks and improve application performance.
3. Ignoring Exception Handling
Spring AI applications often interact with various data sources and APIs, raising the chances of runtime exceptions. Ignoring these exceptions can lead to application crashes or unpredictable behaviors.
Solution
Implement proper exception handling mechanisms. Use Spring's @ControllerAdvice
to handle exceptions globally:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleException(Exception e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR)
.body("An error has occurred: " + e.getMessage());
}
}
By implementing a global exception handler, you can ensure that your application gracefully handles errors and provides meaningful feedback.
4. Skipping Data Validation
The input data quality significantly impacts the performance of your AI models. Developers often overlook the importance of validating incoming data.
Solution
Always validate and sanitize inputs using Spring's built-in validation methods. For instance, if you expect a numeric input:
@Valid
@RequestBody
public ResponseEntity<String> predict(@NotNull @Min(0) Integer input) {
// Process your input
}
By validating data, you can ensure that only valid inputs reach your model and manage model effectiveness better.
5. Lack of Performance Monitoring
Failing to monitor the performance of your AI models can lead to inefficiencies and issues down the line. Models may drift or perform poorly over time without any monitoring.
Solution
Integrate performance monitoring tools like Spring Actuator or Micrometer. By doing so, you can monitor various application metrics.
management:
endpoints:
web:
exposure:
include: "*"
Once you have Actuator integrated, you can monitor various metrics, giving you insights into your models' performance over time.
6. Inadequate Documentation and Communication
AI integrations can become complex, and failing to document your code thoroughly leads to misunderstandings among team members, especially in collaborative environments.
Solution
Every class and method should have clear documentation. Use JavaDoc to provide a coherent understanding of your AI components. For example:
/**
* Predicts the outcome of a data input using the trained model.
*
* @param input the data input to analyze
* @return the predicted outcome
*/
public Prediction predictOutcome(InputData input) {
// Implementation here
}
Investing time in good documentation pays off in clearer communication and easier onboarding for new team members.
7. Failing to Understand the Underlying Models
Lastly, developers sometimes work with advanced machine learning functions without fully understanding the underlying models. This ignorance can lead to misuse or suboptimal configurations.
Solution
Invest time in learning about different machine learning algorithms and models. Utilize online platforms like Coursera or edX to broaden your knowledge base. Understanding the models allows you to make better design decisions and volume upscale the capabilities of your application.
Summary
While Spring AI offers powerful functionalities, it is essential to approach it cautiously. Avoiding common pitfalls such as improper resource management, lack of performance monitoring, and inadequate documentation can significantly alter your development experience, resulting in robust and intelligent applications.
Implementing strategies that address these pitfalls will not only enhance the overall performance of your applications but also foster teamwork, ensure data reliability, and maintain the integrity of your AI models.
By adhering to best practices and utilizing the right tools, you can maximize the effectiveness of Spring AI in your projects. For more in-depth learning, you may refer to the official Spring AI documentation.
For further discussions on Spring AI, or if you have additional questions, feel free to leave comments below! Happy coding!