Dynamic Logging Techniques Without Static Loggers
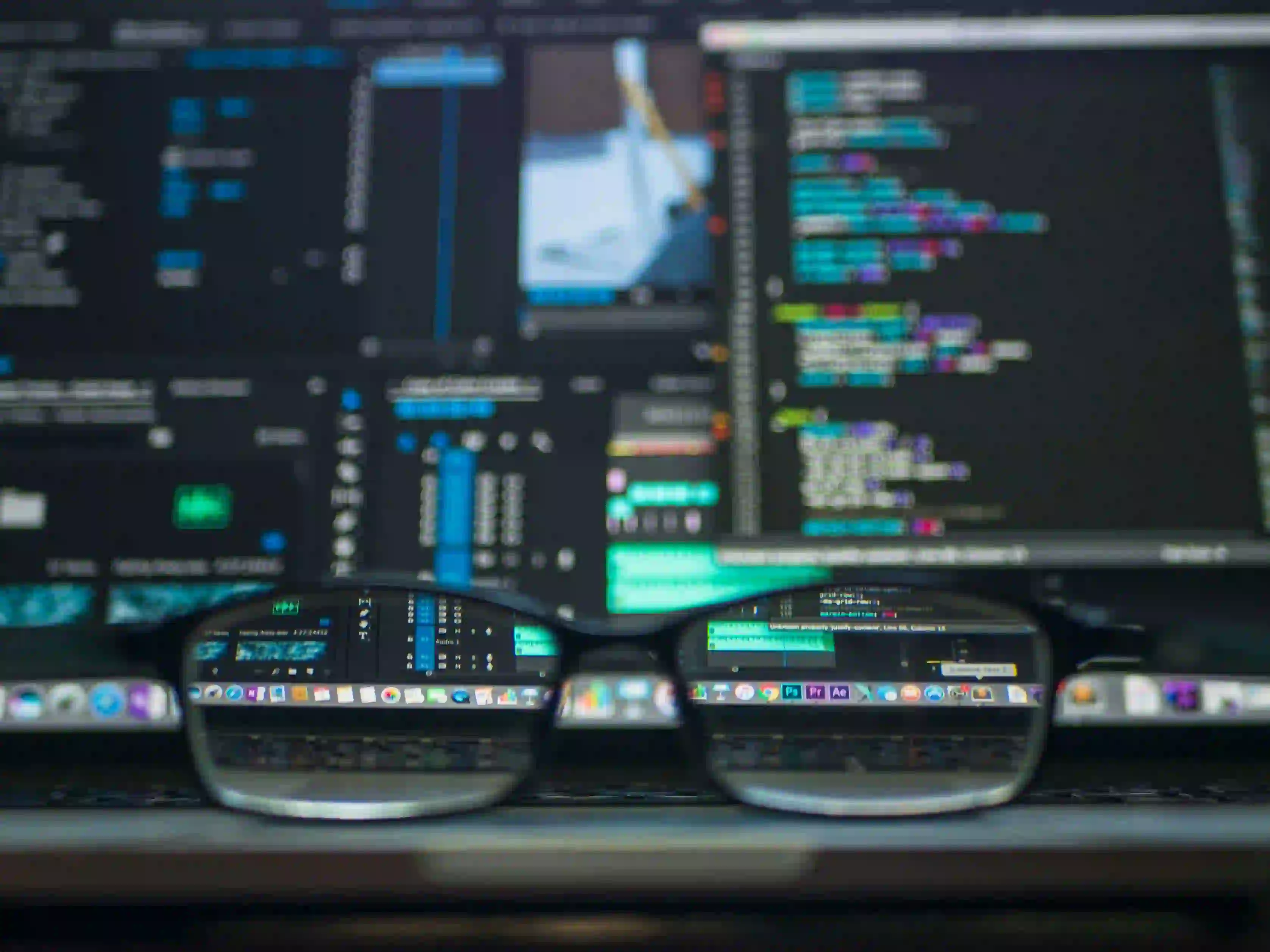
Dynamic Logging Techniques Without Static Loggers in Java
In the world of software development, logging is an essential feature that enhances debug-ability and tracks application behavior. Traditionally, Java developers have relied on static loggers, such as those provided by libraries like Log4j or SLF4J. However, static loggers have their drawbacks, including the inability to change logging behavior at runtime without modifying the code base.
In this blog post, we will explore dynamic logging techniques in Java that allow for more flexibility and control over logging behavior without relying on static loggers.
Why Dynamic Logging?
Dynamic logging offers several advantages, including:
- Runtime Configuration: Modify logging levels and behaviors without restarting the application.
- Enhanced Flexibility: Apply different logging strategies in various environments (development, testing, production).
- Performance Optimization: Reduce the amount of logging performed based on current application requirements.
Key Concepts
Before delving into the code, let's outline some key concepts we will use throughout our examples:
- Logging Level: Various levels (e.g., DEBUG, INFO, WARN, ERROR) categorize the significance of logged messages.
- Logger Factory: A mechanism to create and configure loggers dynamically.
- Configuration Management: Use of external configuration files or services to adjust logging behavior.
Dynamic Logger Implementation
We can achieve dynamic logging through a combination of the following practices:
- Using Java's built-in logging framework (java.util.logging) instead of static loggers.
- Leveraging frameworks like Log4j 2 that support configuration at runtime via XML or JSON.
- Implementing Aspect-Oriented Programming (AOP) using frameworks like Spring, which allows intercepting method calls and logging dynamically.
Example: Using Java's Built-in Logging Framework
Java's built-in logging framework (java.util.logging
) provides a good foundation for dynamic logging. Below is an example where we can adjust logging levels at runtime.
import java.util.logging.Level;
import java.util.logging.Logger;
public class DynamicLoggerExample {
private static final Logger logger = Logger.getLogger(DynamicLoggerExample.class.getName());
public static void main(String[] args) {
// Starting with INFO level
setLogLevel(Level.INFO);
logger.info("This is an INFO level message.");
logger.warning("This is a WARNING level message.");
// Change log level to WARNING at runtime
setLogLevel(Level.WARNING);
logger.info("This INFO message will not be logged.");
logger.warning("This WARNING message will be logged.");
}
public static void setLogLevel(Level newLevel) {
logger.setLevel(newLevel);
System.out.println("Log level changed to: " + newLevel.getName());
}
}
Code Commentary
- The
setLogLevel
method allows us to alter the logger's level dynamically. - We can observe how messages of lesser importance (INFO level) are effectively filtered out when the logging level is raised to WARNING.
Enhancing with Log4j 2
Log4j 2 supports dynamic configuration through XML or JSON files. This means you can change logging configurations without modifying code.
Here is a basic example using Log4j 2 with external configuration:
<!-- log4j2.xml -->
<Configuration status="WARN">
<Appenders>
<Console name="Console" target="SYSTEM_OUT">
<PatternLayout pattern="%d{yyyy-MM-dd HH:mm:ss} [%t] %-5level %logger{36} - %msg%n"/>
</Console>
</Appenders>
<Loggers>
<Root level="info">
<AppenderRef ref="Console"/>
</Root>
</Loggers>
</Configuration>
Java Code Example
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class Log4jDynamicLogger {
private static final Logger logger = LogManager.getLogger(Log4jDynamicLogger.class);
public static void main(String[] args) {
logger.info("Initial info log");
// Dynamically changing logger level
System.setProperty("log4j.configurationFile", "path/to/log4j2.xml");
logger.debug("Debug log after updating configuration");
}
}
Code Commentary
- The
System.setProperty
method alters the logging configuration at runtime by pointing to a new configuration file. - The log level of logs generated can be adjusted independently, offering fine-grained control.
Implementing Aspect-Oriented Programming (AOP)
AOP provides another avenue for dynamic logging. Spring AOP allows method-level logging without changing the actual business logic.
Here’s how you can implement logging using AOP:
- First, add Spring AOP dependencies in your
pom.xml
(for Maven users).
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
- Create the Aspect class:
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.After;
import org.aspectj.lang.annotation.Aspect;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class LoggingAspect {
private static final Logger logger = LoggerFactory.getLogger(LoggingAspect.class);
@After("execution(* com.example.service.*.*(..))")
public void logAfter(JoinPoint joinPoint) {
logger.info("Method executed: " + joinPoint.getSignature().getName());
}
}
- Apply it to your Service class:
import org.springframework.stereotype.Service;
@Service
public class ExampleService {
public void performAction() {
System.out.println("Action performed!");
}
}
Code Commentary
- The
@Aspect
annotation tells Spring to recognize this class as an aspect. - The
@After
advice runs after any method execution in thecom.example.service
package, automatically logging method execution, thus separating concerns and maintaining clean code.
Wrapping Up
Dynamic logging techniques enable developers to implement flexible logging without relying solely on static loggers. Whether it is through Java's built-in logging, Log4j 2, or Aspect-Oriented Programming (AOP), you have the capability to adapt your logging strategy based on runtime needs, ultimately allowing you to optimize performance and debug effectively.
For further reading on advanced logging strategies, check out the official Log4j 2 documentation and explore Spring AOP capabilities.
Implementing these techniques will not only improve your code quality but also enhance the maintainability and adaptability of your Java applications. Happy logging!