Mastering the Art of Java: List to Array Conversion Simplified
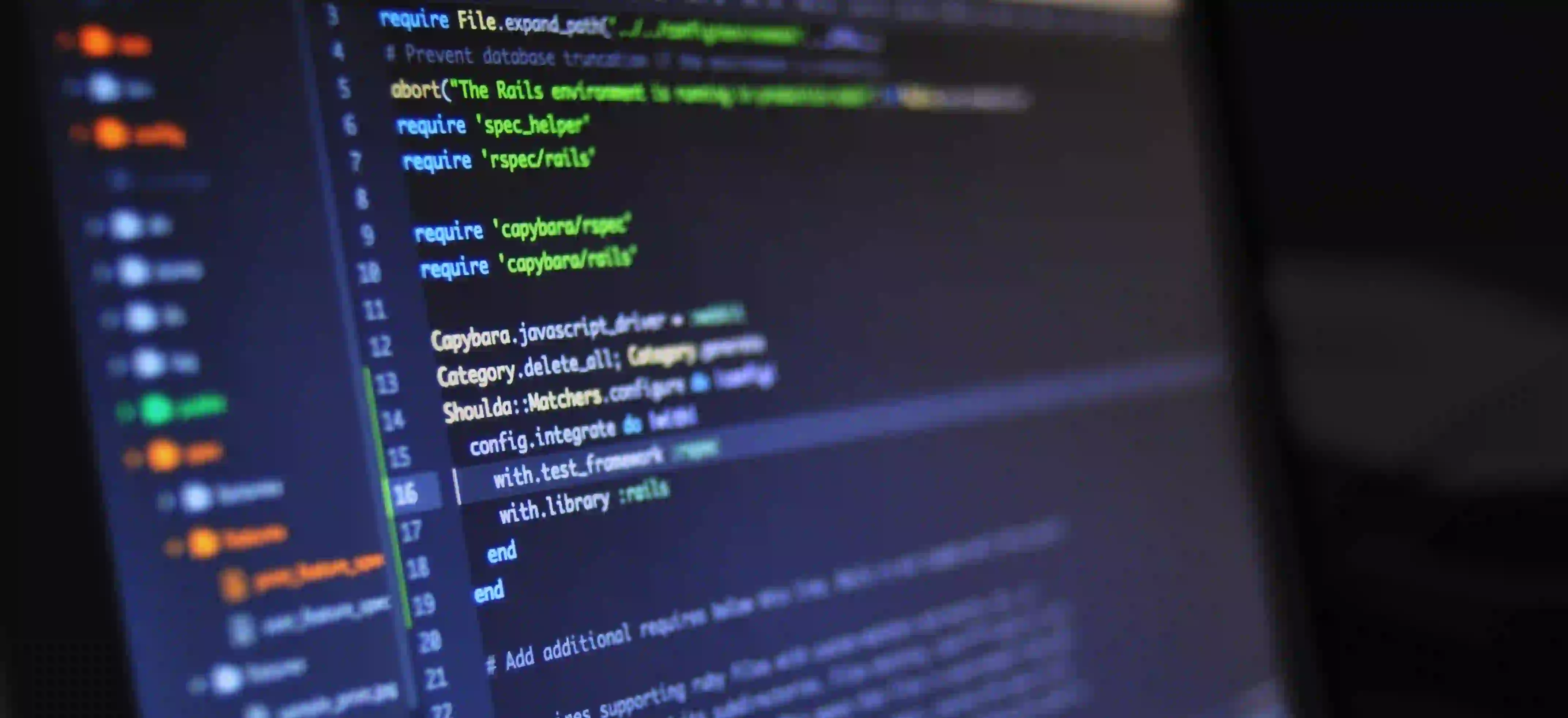
Mastering the Art of Java: List to Array Conversion Simplified
Java is a powerful programming language that excels in various applications, from web development to data processing. One common task that Java developers encounter is converting a List
to an Array
. This blog post will explore the fundamental concepts behind this conversion, demonstrate practical code snippets, and highlight best practices. Join us as we simplify the process of list to array conversion in Java.
Understanding the Basics
In Java, List
is part of the Java Collections Framework, which provides a way to store ordered collections of items. On the other hand, arrays are fixed-size data structures that hold multiple values of the same type. This dichotomy often raises the question: why might you need to convert a List
to an Array
?
When to Convert a List to an Array
- Interoperability: Some APIs or libraries might require arrays for input.
- Performance: For certain performance-sensitive tasks, arrays might provide faster access times.
- Legacy Code: You may be working with legacy systems that heavily utilize arrays.
The Conversion Process
The process of converting a List
to an Array
in Java is straightforward. Java provides built-in methods that simplify this task. The primary method used is toArray()
, which comes in two flavors:
toArray()
- Returns an array containing all the elements in the list.toArray(T[] a)
- Returns an array containing all the elements in the list, with a specific type.
Example Code Snippet 1: Basic Conversion Using toArray()
Here’s a simple illustration of how to convert a List
to an Array
:
import java.util.ArrayList;
import java.util.List;
public class ListToArrayExample {
public static void main(String[] args) {
// Create a List of Strings
List<String> stringList = new ArrayList<>();
stringList.add("Java");
stringList.add("Python");
stringList.add("C++");
// Convert List to Array
String[] stringArray = stringList.toArray(new String[0]);
// Print the Array
for (String language : stringArray) {
System.out.println(language);
}
}
}
Explanation
In the example above:
- We import necessary classes from the
java.util
package. - An
ArrayList
of strings is created, and a few programming languages are added. - The
toArray(new String[0])
method converts theList
to an array. Usingnew String[0]
allows the JVM to know the desired type, facilitating type-safe conversion. - Finally, we print the contents of the new array to the console.
This illustrates a simple yet effective way to convert a List
into an array.
Code Snippet 2: Array of a Specific Type
If you require more control over the type of the resulting array, you can specify it explicitly. Let’s tweak the previous example slightly:
import java.util.Arrays;
import java.util.List;
public class ExplicitTypeArray {
public static void main(String[] args) {
List<Integer> integerList = Arrays.asList(1, 2, 3, 4, 5);
// Convert List to Array
Integer[] integerArray = integerList.toArray(new Integer[0]);
// Print the Array
System.out.println("Integer Array: " + Arrays.toString(integerArray));
}
}
Explanation
In this code:
- We created a
List
of integers usingArrays.asList()
. - The
toArray(new Integer[0])
function is again used to perform the conversion. - The output utilizes
Arrays.toString()
to present the array in a readable format.
Why Use new Type[0]
?
One pertinent question is why you should use new Type[0]
instead of a larger array.
- Dynamic Sizing: When calling
toArray()
, if you provide an array larger than required, it retains the excess elements as the underlying array is not resized. It’s often more efficient to create an empty array. - Performance: The JVM can optimize the memory allocation for smaller arrays due to its reference, minimizing waste.
Example Code Snippet 3: Handling Primitive Types
Java has special rules regarding primitives. If you have a list of primitive types, you cannot directly use toArray()
. Instead, you need to convert them to their respective wrapper types. Here’s how you handle this situation:
import java.util.ArrayList;
import java.util.List;
public class ListToPrimitiveArray {
public static void main(String[] args) {
List<Integer> integerList = new ArrayList<>();
integerList.add(1);
integerList.add(2);
integerList.add(3);
// Convert List<Integer> to int[]
int[] primitiveArray = integerList.stream().mapToInt(Integer::intValue).toArray();
// Print the Primitive Array
for (int i : primitiveArray) {
System.out.println(i);
}
}
}
Explanation
Here’s what happens in the above snippet:
- We use a
List<Integer>
to hold the integer values. - The
stream().mapToInt(Integer::intValue).toArray()
constructs anint[]
from theList
. - This is necessary since
int
is a primitive type, distinguishing it fromInteger
, the wrapper class.
Using Streams
Java’s Stream API provides an elegant and functional way to handle collections. The streams facilitate chaining and functional programming patterns, which can be very efficient.
Performance Considerations
When converting lists to arrays, it’s essential to remember a few key performance tips:
- Avoiding Unnecessary Copies: Always try to minimize overhead by ensuring you’re only copying necessary elements.
- Using Streams Judiciously: While Streams provide clarity, they may introduce some overhead. Analyze performance when processing large datasets.
- Memory Management: If dealing with large collections, profiling the memory usage can help ensure that you are not adversely affecting the application’s performance.
Final Thoughts
Converting a List
to an Array
in Java can initially seem like a daunting task. However, with a solid understanding of the available methods and their appropriate usage, it can be a straightforward process. From utilizing toArray()
to handling specific types with Streams, these techniques are fundamental in mastering Java collections.
For further reading, consider exploring the official Java documentation to deepen your understanding of the Collections Framework. Additionally, check out Oracle's Java Tutorials for more comprehensive guides and insights into Java programming.
Remember, every line of code you write should enhance your understanding of Java. Embrace the art of Java programming, and soon you'll be converting lists to arrays with ease! Happy coding!