Overcoming Java FlatBuffers Performance Issues
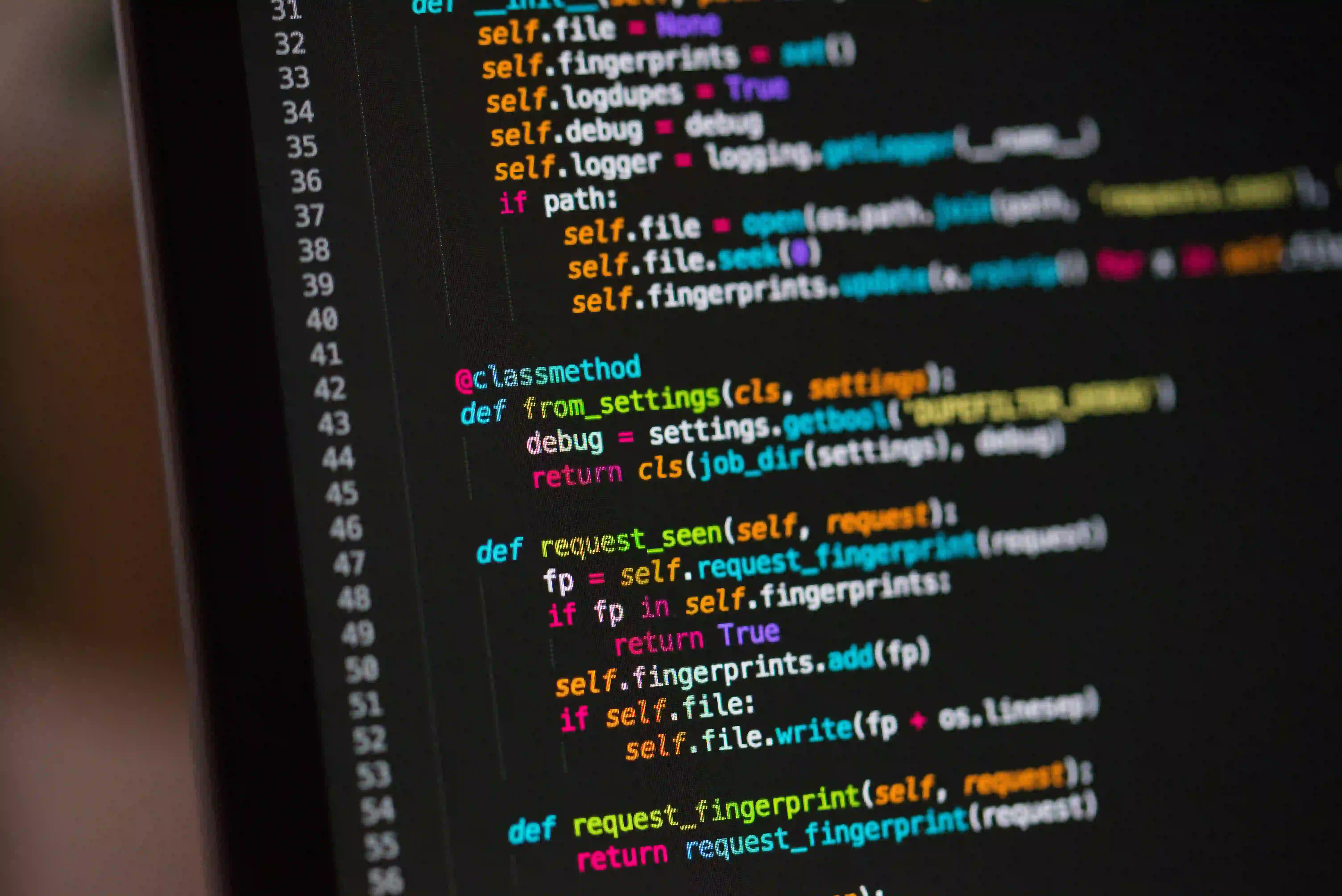
Overcoming Java FlatBuffers Performance Issues
FlatBuffers is a powerful serialization library developed by Google, designed for efficiency and speed. It’s particularly beneficial in scenarios where performance is critical, such as gaming, Android, and network communications. However, developers occasionally encounter performance issues while working with FlatBuffers in Java. This blog post aims to discuss common performance challenges and provide practical solutions to enhance your Java FlatBuffers performance.
What Are FlatBuffers?
FlatBuffers allow for direct access to serialized data without unpacking or unpacking. This approach is ideal for applications that require fast data access. Unlike typical serialization methods, FlatBuffers provide:
- Zero-Copy Access: You can read data directly without allocating extra memory for its representation.
- Efficient Memory Usage: Only the relevant data is serialized, saving on space.
- Cross-Platform Support: Works across different programming languages, making it versatile.
However, like any tool, FlatBuffers may not perform optimally in every situation.
Identifying Performance Issues
Before diving into solutions, it is essential to identify where the performance bottlenecks might be. Common performance issues include:
- High Memory Consumption: When FlatBuffers are incorrectly structured, it can lead to increased memory usage.
- Inefficient Object Creation: Inefficiencies in how objects are being created and filled can affect performance negatively.
- Improper Use of Buffers: Not utilizing buffers adequately for data reads and writes.
Having the awareness of these issues serves as the foundation to effectively overcome performance challenges when using FlatBuffers in Java.
Solution: Structuring Your FlatBuffers Efficiently
When constructing your FlatBuffers schemas, consider the following guidelines:
Choosing the Right Schema Design
FlatBuffers schemas are defined using a specific language. An effective schema can drastically improve performance due to reduced memory footprint and better data organization.
Example Schema:
namespace MonsterGame;
table Monster {
id: int;
name: string;
location: vec3;
}
table vec3 {
x: float;
y: float;
z: float;
}
root_type Monster;
Why This Matters: Well-structured schemas ensure the data is logically organized, which directly impacts serialization and deserialization speed.
Tip: Use Vectors Wisely
Vectors are optimized for performance but can still be a performance bottleneck if used carelessly. Always measure the size of the vectors you create and maintain them as small as necessary.
Example: Filling and Serializing Data
This example demonstrates a simple way to create a FlatBuffer, fill data, and convert it to serialized form efficiently:
import com.google.flatbuffers.FlatBufferBuilder;
public class MonsterCreator {
public static int createMonster(FlatBufferBuilder builder, int id, String name, float x, float y, float z) {
int nameOffset = builder.createString(name);
Monster.startMonster(builder);
Monster.addId(builder, id);
Monster.addName(builder, nameOffset);
Monster.addLocation(builder, createVec3(builder, x, y, z));
return Monster.endMonster(builder);
}
private static int createVec3(FlatBufferBuilder builder, float x, float y, float z) {
Vec3.startVec3(builder);
Vec3.addX(builder, x);
Vec3.addY(builder, y);
Vec3.addZ(builder, z);
return Vec3.endVec3(builder);
}
}
Commentary: In the code snippet above, FlatBufferBuilder
is utilized to create a new monster object efficiently. The method createMonster
demonstrates how to initialize the object while ensuring minimal memory allocations.
Insight: Always finalize your buffers using
builder.finish()
to ensure everything is properly written and accessible.
Efficient Buffer Usage
Managing your buffers effectively helps minimize garbage collection cycles and improve performance. Here’s how:
- Reuse Buffers: Instead of creating new buffers for every serialization, consider reusing existing ones.
- Limit the Size of Buffers: Monitor the size of your buffers. A large buffer can consume more memory than necessary, leading to performance hindrances.
- Use Direct Buffers: Java's NIO package can provide direct access to the memory, improving performance for I/O operations.
Example of Direct Buffer Usage
import java.nio.ByteBuffer;
public class DirectBufferExample {
public static void useDirectBuffer() {
ByteBuffer directBuffer = ByteBuffer.allocateDirect(1024);
// Use direct buffer operations
directBuffer.putInt(42);
directBuffer.flip();
System.out.println("Direct Buffer Data: " + directBuffer.getInt());
}
}
Discussion: Using a Direct ByteBuffer
ensures that you're minimizing the overhead of memory management on the Java heap, thus improving performance.
Profiling Your Application
When performance challenges arise, profiling is your best friend. Tools such as VisualVM or Java Flight Recorder can help you identify data bottlenecks:
- Analyze Object Creation: See how much time and memory is being consumed on object creations.
- Evaluate Garbage Collection: Frequent GC can quickly slow down your application.
For further reading on profiling, consider visiting the Oracle documentation on JDK tools.
Final Considerations
Java FlatBuffers can be highly efficient if structured and used correctly. Through proper schema design, efficient buffer usage, and regular profiling, you can significantly enhance the performance of your application.
Remember, the key is to reduce overhead, optimize memory usage, and ensure your data structures are as lightweight as possible. With diligence, your Java application can withstand any performance scrutiny, leveraging FlatBuffers to their fullest potential.
Happy coding!