Java vs Python: The 2018 Performance Showdown
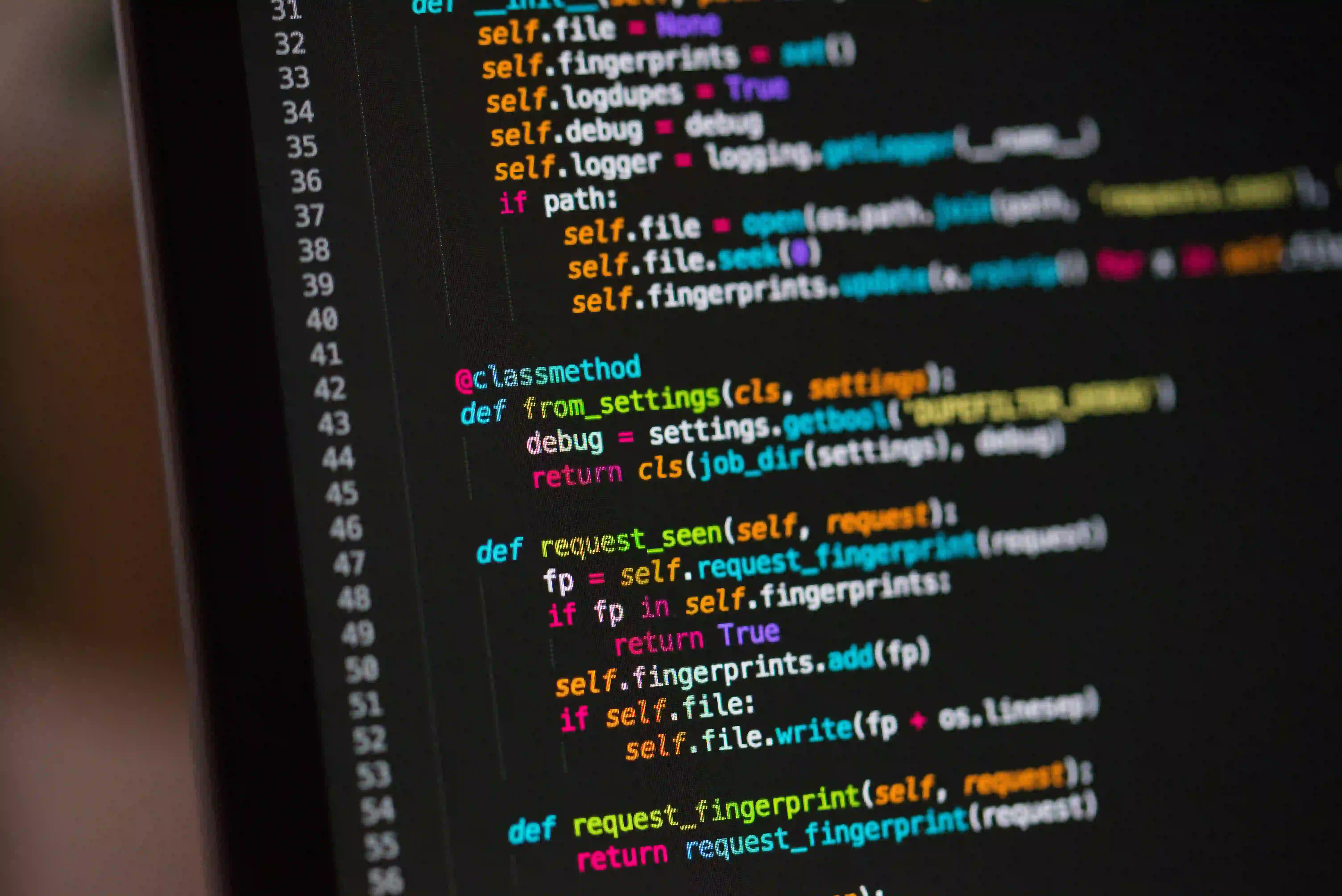
Java vs Python: The 2018 Performance Showdown
In the dynamic landscape of programming languages, Java and Python have secured prominent positions. Developers worldwide wrestle with the age-old question: which language reigns superior? While both languages have their unique strengths, this showdown will specifically focus on performance—an essential factor for developers working on resource-intensive applications.
Overview of Java and Python
What is Java?
Java, a statically-typed, object-oriented programming language, was developed by Sun Microsystems in 1995. It is renowned for its "Write Once, Run Anywhere" capability, thanks to the Java Virtual Machine (JVM). Java emphasizes portability, performance, and security, making it a popular choice for large applications and enterprise-level solutions.
What is Python?
Python, conceived by Guido van Rossum and released in 1991, is an interpreted, dynamically-typed language praised for its simplicity and readability. It supports multiple programming paradigms, including procedural, object-oriented, and functional programming. Python's robust libraries and frameworks make it a go-to for rapid development and data analysis.
Performance in Perspective
Performance can be defined in various ways such as execution speed, memory consumption, and the language's suitability for concurrent processing. In 2018, discussions around Java and Python were heavily influenced by the use cases of both languages.
Speed of Execution
One of the most significant performance differences lies in execution speed. Java generally outperforms Python primarily due to its compilation process.
Java Compilation vs. Python Interpretation
Java code is compiled into bytecode, which is executed by the JVM. This compilation process allows Java to run faster, especially in CPU-intensive tasks. Here's a simple example of Java code showing the performance of a loop:
public class PerformanceTest {
public static void main(String[] args) {
long startTime = System.currentTimeMillis();
for (int i = 0; i < 1_000_000; i++) {
// Simulating some computation
Math.sqrt(i);
}
long endTime = System.currentTimeMillis();
System.out.println("Java Execution Time: " + (endTime - startTime) + " ms");
}
}
In the snippet above, we measure the time taken to compute the square root of a million integers. Java’s compiled nature allows it to execute this task swiftly, making it suitable for performance-sensitive applications.
In contrast, Python is executed line-by-line, which can lead to slower performance in CPU-bound tasks. Here's an equivalent Python snippet:
import time
import math
start_time = time.time()
for i in range(1_000_000):
# Simulating some computation
math.sqrt(i)
end_time = time.time()
print("Python Execution Time: {:.2f} ms".format((end_time - start_time) * 1000))
While the above Python code accomplishes the same task, it likely will take longer to execute than its Java counterpart, primarily due to its interpreted nature.
Memory Consumption
When it comes to memory consumption, Java tends to use more memory due to its object-oriented architecture and garbage collection. However, this trade-off often results in optimized performance.
Python, while more memory-efficient for smaller projects, may face scalability issues in larger systems:
- In Java, new objects consume memory consistently, and the garbage collector cleans up unused objects.
- In Python, the reference counting system may incur memory overhead, particularly in complex applications.
Concurrency and Parallelism
Java shines in multi-threading and concurrent programming. The language has built-in support for multi-threading, with its robust concurrency library making it easier to scale applications effectively. Here's a brief example demonstrating thread creation in Java:
class ThreadExample extends Thread {
public void run() {
System.out.println("Thread " + Thread.currentThread().getName() + " is running.");
}
public static void main(String[] args) {
for (int i = 0; i < 5; i++) {
ThreadExample thread = new ThreadExample();
thread.start();
}
}
}
In this code, we create multiple threads, allowing the application to perform multiple tasks at once, enhancing overall execution time.
On the other hand, Python has the Global Interpreter Lock (GIL), which prevents multiple native threads from executing at once, effectively negating the benefits of multi-threading in certain contexts. Instead, developers often turn to libraries like multiprocessing
for parallel execution, which can introduce additional complexity.
Libraries and Ecosystem
Both languages boast extensive libraries, although their focuses often differ:
- Java excels in enterprise-level applications, game development, and mobile applications via Android.
- Python thrives in scientific computing, machine learning, and web development.
Libraries like Spring for Java and Pandas for Python have transformed their respective ecosystems, offering developers powerful tools for a wide range of applications.
When to Use Java or Python?
Selecting between Java and Python is not merely reflective of performance metrics; it requires a comprehensive understanding of project requirements and team capabilities. Here are various scenarios that may dictate your choice:
Use Java When:
- Performance is a critical factor (e.g., financial applications or gaming).
- Working on large enterprise applications where scalability is needed.
- Creating Android applications.
Use Python When:
- Speed of development is prioritized (e.g., startups).
- Working on data-focused projects involving machine learning or data analysis.
- Collaborating with a team that emphasizes code readability and simplicity.
Closing Remarks
The Java vs. Python performance showdown of 2018 revealed significant contrasts that continue to influence developer decisions today. Java generally excels in execution speed and concurrent processing, while Python champions ease of development and flexibility. Choosing the right language ultimately depends on your project's specific needs, team expertise, and long-term objectives.
For a deeper understanding of how these languages compare, you can check out these useful resources:
- Java Performance Tuning
- Python Performance Tips
Each language has its unique advantages, and understanding these characteristics will empower you to make informed decisions tailored to your development needs. Happy coding!