Avoiding Common Pitfalls in Java Proxy Design Pattern
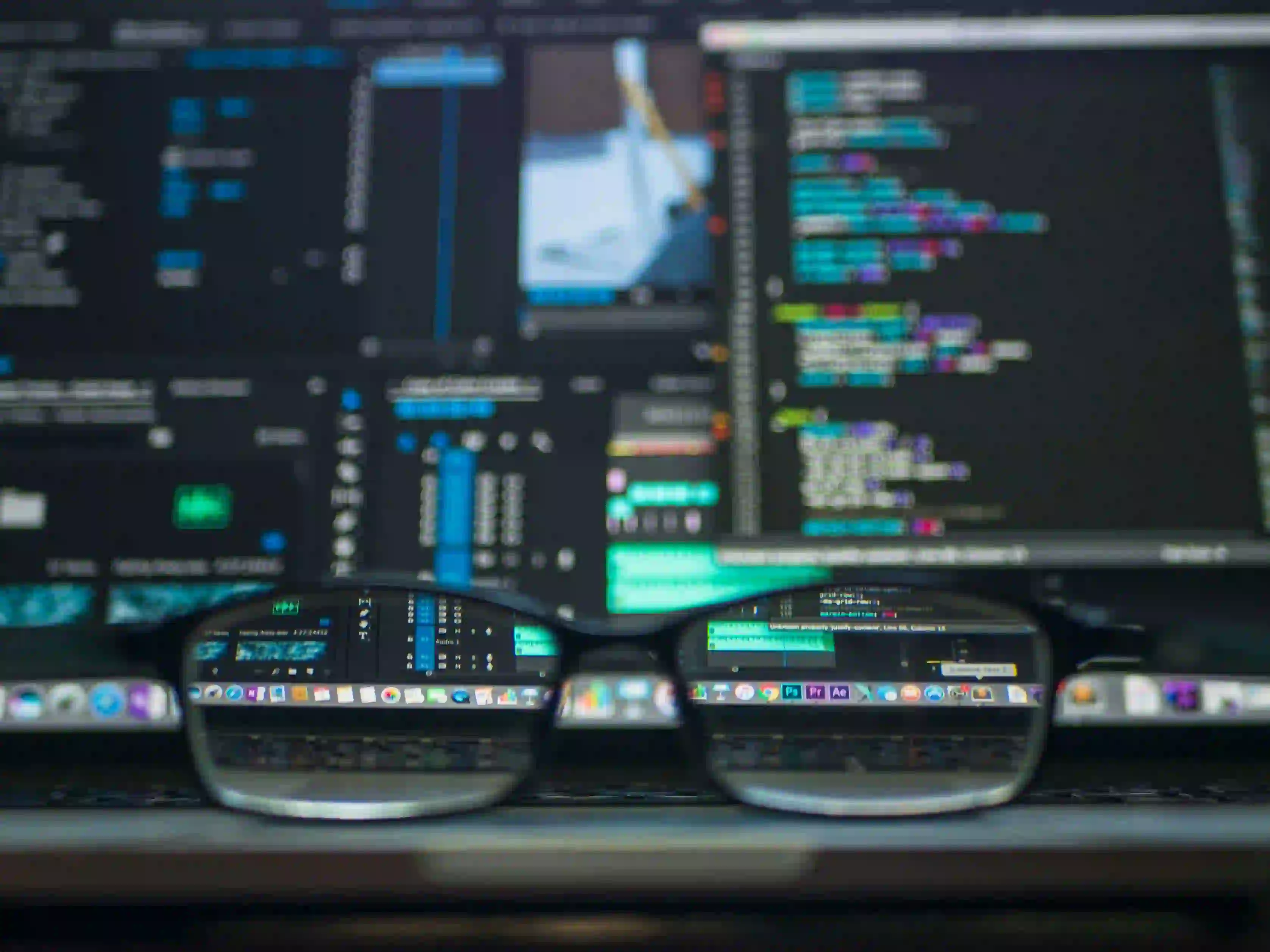
Avoiding Common Pitfalls in Java Proxy Design Pattern
The Proxy Design Pattern serves as a fundamental architectural tool in software design, particularly in Java. It allows objects to serve as proxies or representatives for other objects, enabling various capabilities such as lazy loading, access control, logging, and more. However, it comes with its share of challenges. In this blog post, we will explore some common pitfalls associated with the Proxy Design Pattern in Java and how you can avoid them.
What is the Proxy Design Pattern?
The Proxy Design Pattern provides a surrogate or placeholder for another object to control access to it. The main types of proxies are:
- Virtual Proxy: Delays the creation of the real object until it's actually required.
- Protection Proxy: Controls access to the real object, usually for security reasons.
- Remote Proxy: Represents an object that is in another address space, often used in distributed systems.
How Does It Work?
In Java, the Proxy Design Pattern often relies on interfaces. Let's look at a simple example to illustrate how it works.
// Subject Interface
public interface Image {
void display();
}
// Real Subject
public class RealImage implements Image {
private String filename;
public RealImage(String filename) {
this.filename = filename;
loadImageFromDisk();
}
private void loadImageFromDisk() {
System.out.println("Loading " + filename);
}
public void display() {
System.out.println("Displaying " + filename);
}
}
// Proxy
public class ProxyImage implements Image {
private RealImage realImage;
private String filename;
public ProxyImage(String filename) {
this.filename = filename;
}
public void display() {
if (realImage == null) {
realImage = new RealImage(filename);
}
realImage.display();
}
}
Why Use Proxies?
Using a Proxy reduces memory utilization if the full object is not necessary immediately. It prevents excessive resources from being consumed. Real-world applications, such as image loading, exemplify the performance benefits of using Proxies.
Common Pitfalls of Proxy Design Pattern
1. Excessive Complexity
Problem: Implementing the Proxy Design Pattern can inadvertently lead to over-engineering. Adding unnecessary layers can confuse the program flow and decrease performance.
Solution: Keep your design straightforward. Make sure the added complexity is justified by the benefits. Proxies should enhance the design, not complicate it.
2. No Synchronization
Problem: Proxies in a multi-threaded environment can create issues. If a Proxy manages access to a resource without proper synchronization, it can lead to race conditions.
Solution: Use synchronized methods or blocks where necessary.
public class ProxyImage implements Image {
private RealImage realImage;
private String filename;
public ProxyImage(String filename) {
this.filename = filename;
}
public synchronized void display() {
if (realImage == null) {
realImage = new RealImage(filename);
}
realImage.display();
}
}
3. Breaking the Interface Segregation Principle
Problem: Sometimes, Proxy classes can be built to implement many interfaces resulting in bloated classes that try to accomplish too much.
Solution: Stick to the Interface Segregation Principle (ISP). Create smaller, more focused interfaces specifically for the Proxy to implement. This leads to better separation of concerns and easier maintenance.
4. Not Using Interfaces
Problem: Some developers opt to directly reference the concrete class instead of relying on interfaces. This practice compromises flexibility and reusability.
Solution: Always code to an interface. This practice allows you to easily switch implementations without modifying client code.
Image image = new ProxyImage("test_image.jpg");
image.display();
Using an interface makes it easier to replace the ProxyImage
with another implementation later without any changes to the client code.
5. Unintended Side Effects
Problem: Using a Proxy can lead to unexpected behaviors, especially if the Proxy alters the state or functionality of the real class.
Solution: Be cautious about side effects. Proxies should mirror the operations of the real subject as closely as possible. If state changes are necessary, document them clearly.
Best Practices for Using the Proxy Pattern
-
Encapsulation: Ensure that the Proxy encapsulates all calls to the real subject adequately.
-
Caching: If applicable, implement caching at the Proxy level to avoid repetitive operations and improve performance.
-
Testing: Write unit tests specifically for your Proxies. Ensure they behave as expected under various conditions.
-
Logging: Consider adding logging capabilities to track when the Proxy is engaged, which can provide useful debugging insights.
Real-World Applications of Proxies
To further illustrate the usefulness of the Proxy Design Pattern, consider the following common applications:
- Remote Method Invocation (RMI): In distributed systems, proxies can represent remote objects, allowing local calling methods to interact with them seamlessly.
- Virtual Proxies in GUIs: Applications that have to manage large images or components often use virtual proxies to speed up user interface loading times.
- Security Proxies: In services requiring user authentication, proxies can check user permissions before allowing access to sensitive operations.
For more information on design patterns and their applications, refer to Refactoring Guru and Java Design Patterns.
Bringing It All Together
The Proxy Design Pattern is a powerful tool in Java, but like all patterns, it must be implemented wisely. Avoiding common pitfalls such as excessive complexity, synchronization issues, and breaking the Interface Segregation Principle will lead to cleaner, more maintainable code.
Remember, the ultimate goal of any design pattern is to enhance your system's maintainability, scalability, and performance. Use the Proxy Design Pattern where appropriate, adhering to best practices, and your Java applications will benefit immensely.
Happy coding!