Mastering JUnit's Runner Architecture: Common Pitfalls Explained
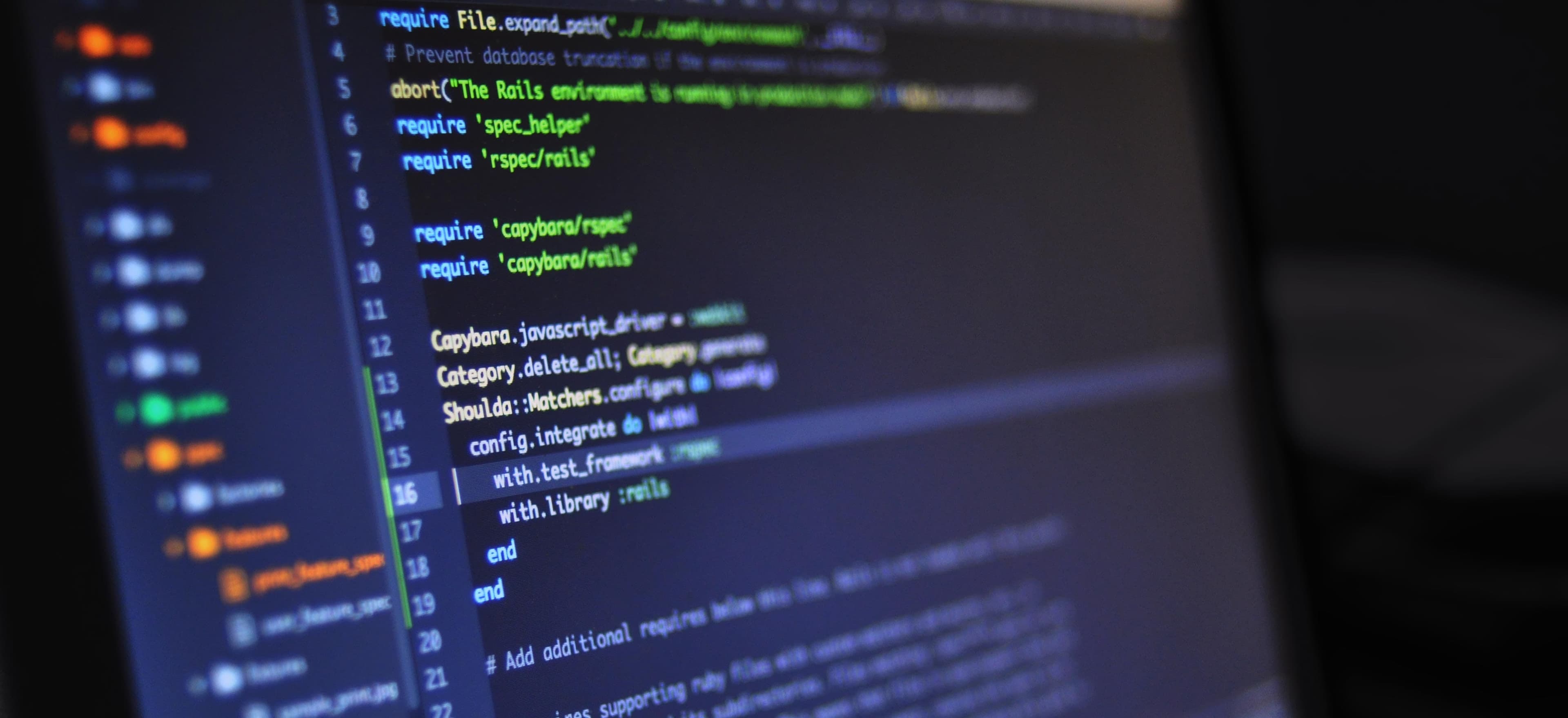
- Published on
Mastering JUnit's Runner Architecture: Common Pitfalls Explained
JUnit is a powerful testing framework designed for the Java programming language. Understanding its inner workings, particularly the Runner architecture, is key to writing better tests. The ability to write effective tests is critical for maintaining code quality and ensuring the reliability of applications. In this article, we will delve deep into the Runner architecture of JUnit, exploring common pitfalls and how to avoid them.
What is a JUnit Runner?
JUnit runners are responsible for executing tests, reporting results, and handling lifecycle management of test methods. They are the bridge between your test classes and the JUnit testing framework. JUnit provides several built-in runners, like BlockJUnit4ClassRunner
for standard tests, and Parameterized
for parameterized tests. However, you can also create custom runners to fit your specific needs.
Understanding the Workflow
When you run a JUnit test, here's what happens behind the scenes:
- Initialization: The JUnit runner initializes the test class and its members.
- Test Execution: The test methods are executed in the order defined by the runner.
- Result Reporting: The outcome of each test (pass, fail, ignore) is reported back to the JUnit framework.
This workflow can be easily visualized in the following code snippet:
@RunWith(MyCustomRunner.class)
public class MyTest {
@Test
public void testSomething() {
// Test implementation
}
}
In the snippet above, MyCustomRunner
is the runner that will handle how the MyTest
class's tests are executed.
Common Pitfalls and Solutions
Understanding potential pitfalls in JUnit's Runner architecture can save you from headaches down the road. Let's discuss some of these common pitfalls along with their solutions.
1. Misusing @RunWith Annotation
The @RunWith
annotation tells JUnit which runner to use for a particular test class. A common mistake is to apply multiple @RunWith
annotations or inadvertently inherit this annotation in child classes.
Solution: Always ensure that the @RunWith
annotation is applied at the class level and that it is only used once per class.
@RunWith(MyCustomRunner.class)
public class MyTest {
// Correct usage
}
@RunWith(MyCustomRunner.class)
@RunWith(AnotherRunner.class) // Incorrect usage
public class MyTest {
}
2. Failing to Override Required Methods in Custom Runners
When creating a custom runner, it is essential to override specific methods like runChild()
and describeChild()
. Neglecting these can lead to unexpected behaviors or failures.
Solution: Make sure to implement all necessary overrides. Here’s an example of a simple custom runner:
public class MyCustomRunner extends BlockJUnit4ClassRunner {
public MyCustomRunner(Class<?> testClass) throws Exception {
super(testClass);
}
@Override
protected void runChild(final FrameworkMethod method, RunNotifier notifier) {
// Custom execution logic can be added here
super.runChild(method, notifier);
}
@Override
protected String describeChild(FrameworkMethod method) {
return "Custom Description: " + method.getName();
}
}
3. Improper Lifecycle Management
JUnit runners have lifecycle methods such as @BeforeClass
, @AfterClass
, @Before
, and @After
. Failing to understand their purpose can lead to resource leaks or improper setup of your tests.
Solution: Use lifecycle annotations appropriately, ensuring that shared resources are initialized and cleaned up correctly.
public class MyTest {
@BeforeClass
public static void setupOnce() {
// Setup that runs once before all tests
}
@AfterClass
public static void tearDownOnce() {
// Cleanup that runs once after all tests
}
@Before
public void setup() {
// Setup for each test method
}
@After
public void tearDown() {
// Cleanup after each test method
}
}
4. Neglecting Parameterized Tests
Parameterized tests can be powerful for testing with multiple sets of data. A common pitfall is either not implementing these correctly or misunderstanding how to use the @Parameters
annotation.
Solution: Ensure that you utilize the @RunWith(Parameterized.class)
correctly, and define the test data appropriately.
@RunWith(Parameterized.class)
public class ParameterizedTest {
private int input;
private int expected;
public ParameterizedTest(int input, int expected) {
this.input = input;
this.expected = expected;
}
@Parameterized.Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][] {
{ 1, 2 },
{ 2, 3 },
{ 3, 4 }
});
}
@Test
public void testIncrement() {
assertEquals(expected, input + 1);
}
}
5. Ignoring Exception Handling
Tests that expect exceptions need to be handled carefully. Usual mistakes include failing to assert the expected exception or neglecting to use @Test(expected = SomeException.class)
properly.
Solution: Ensure that exceptions are expected and handled correctly:
@Test(expected = IllegalArgumentException.class)
public void testExceptionThrowing() {
throw new IllegalArgumentException("Expected exception");
}
Final Thoughts
Mastering JUnit's Runner architecture involves a bit of exploration and understanding of the common pitfalls discussed above. By ensuring that you creatively use annotations, implement necessary methods, manage lifecycle events correctly, utilize parameterized tests, and handle exceptions, you can significantly improve your test reliability and maintainability.
JUnit continues to evolve and adapt to developers' needs, making it crucial to stay updated. For more in-depth information about writing better tests, you might want to delve into the official JUnit documentation and explore additional testing strategies.
By understanding these elements, you will harness the full potential of JUnit, ensuring that your Java applications remain robust and trustworthy as they evolve. Happy testing!