Balancing Game Difficulty in JavaFX Tower Defense
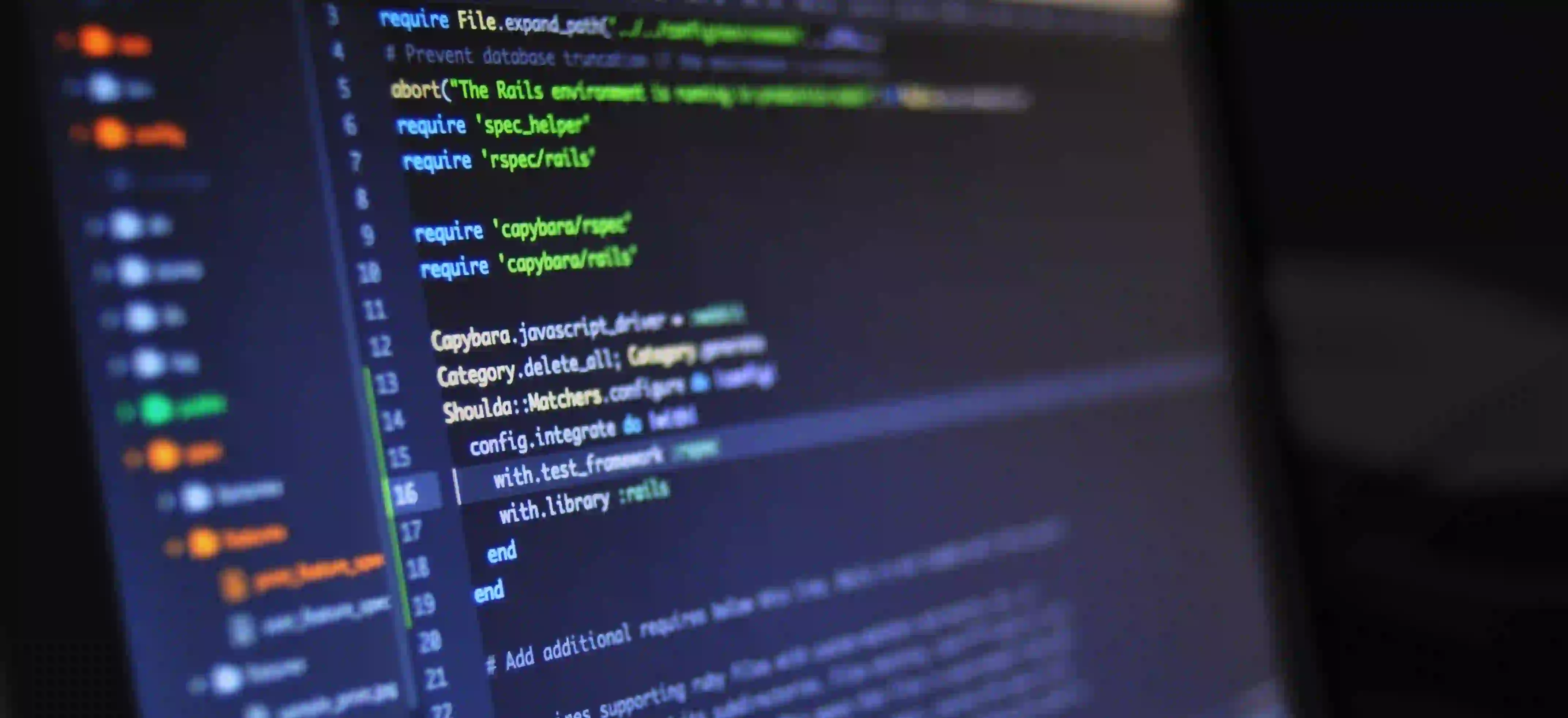
Balancing Game Difficulty in JavaFX Tower Defense
When developing a tower defense game in JavaFX, one of the most crucial aspects is balancing game difficulty. If a game is too easy, players may lose interest; if it's too hard, they may get frustrated. Striking the right balance offers players a satisfying challenge that keeps them engaged. This blog post will delve into various strategies for balancing difficulty, focusing on practical implementation in JavaFX.
Understanding Game Difficulty
Game difficulty can be defined as a measure of how challenging a game is for the player. It involves multiple aspects, such as enemy speed, spawn rate, damage dealt, and resource availability. Depending on the target audience, the ideal difficulty may vary. Consider the following elements when adjusting your game difficulty:
- Player Progression: How players progress through levels.
- Resource Management: Balancing the player's ability to gather and spend resources.
- Enemy Variety: The types of enemies they will face and their behaviors.
Importance of Difficulty Balancing
Balancing difficulty ensures that players remain engaged without feeling overwhelmed. Properly balanced games promote:
- Player Retention: Keeping users engaged over time.
- Challenge: Offering a rewarding experience that feels earned.
- Fairness: Ensuring that difficulty adjustments don’t unjustly penalize players.
Strategies for Difficulty Balancing
There are multiple strategies you can implement to balance difficulty, ranging from algorithmic approaches to intuitive design decisions. Here, we will explore a few effective strategies.
1. Dynamic Difficulty Adjustment (DDA)
Dynamic difficulty adjustment involves automatically altering the game's difficulty based on the player's performance. This can be achieved by evaluating data, such as how many levels a player has completed, their win/loss ratio, and how quickly they clear levels.
Here is a simple JavaFX snippet demonstrating dynamic difficulty adjustment based on player performance:
public class GameDifficulty {
private int playerScore;
private int playerLevel;
private Difficulty difficulty;
public GameDifficulty(int playerScore, int playerLevel) {
this.playerScore = playerScore;
this.playerLevel = playerLevel;
this.difficulty = Difficulty.EASY; // Initial difficulty setting
}
public void updateDifficulty() {
if (playerScore > 1000) {
difficulty = Difficulty.MEDIUM;
}
if (playerScore > 2000 || playerLevel >= 5) {
difficulty = Difficulty.HARD;
}
}
public Difficulty getDifficulty() {
return difficulty;
}
public enum Difficulty {
EASY,
MEDIUM,
HARD
}
}
Why Use DDA?
Dynamic difficulty is crucial to adapt the player experience in real-time. It maintains challenge without making it frustrating.
2. Enemy Progression
Balancing enemy progression ensures that enemies get progressively harder as players advance. This can involve increasing health, speed, or introducing new enemy types.
For instance, you might define a base enemy class and extend it to create more advanced enemies:
public abstract class Enemy {
protected int health;
protected int speed;
public abstract void attack();
public int getHealth() {
return health;
}
public int getSpeed() {
return speed;
}
}
public class BasicEnemy extends Enemy {
public BasicEnemy() {
this.health = 100;
this.speed = 1;
}
@Override
public void attack() {
System.out.println("BasicEnemy attacks!");
}
}
public class EliteEnemy extends Enemy {
public EliteEnemy() {
this.health = 200; // More health than BasicEnemy
this.speed = 2; // Faster
}
@Override
public void attack() {
System.out.println("EliteEnemy attacks with fierce power!");
}
}
Why Utilize Enemy Progression?
Variety keeps the gameplay fresh and challenging. Players enjoy facing new enemies with unique abilities as they advance.
3. Resource Management
In most tower defense games, players can earn resources allowing them to build defenses or upgrade their towers. Resource management provides a gratifying sense of progression.
Here’s a JavaFX example of managing resources that can be adjusted for difficulty:
public class ResourceManager {
private int resources;
public ResourceManager() {
this.resources = 100; // Initial resources
}
public void earnResources(int amount) {
resources += amount;
System.out.println("Earned resources: " + amount);
}
public int getResources() {
return resources;
}
public void spendResources(int amount) {
if (resources >= amount) {
resources -= amount;
System.out.println("Spent resources: " + amount);
} else {
System.out.println("Not enough resources!");
}
}
}
Why Focus on Resource Management?
Managing resources effectively can create a broader strategic layer in your game, providing players with various options on how to approach each challenge.
4. Playtesting and Feedback
No game is perfect on its first iteration. Continuous playtesting helps identify areas where the difficulty might be off-balance.
Collect data from testers:
- How many attempts did they take to beat certain levels?
- Which enemies felt too unfair?
- Were there moments when they felt they had no chance to win?
Tools like Google Forms can help you collect this feedback systematically.
5. Level Design and Mechanics
Finally, consider the level design and how mechanics can contribute to difficulty. Use strategically placed obstacles and varied terrain to shape player strategies. For instance, different enemy paths or choke points can significantly affect how easy or hard a level feels.
Here is an example of creating a basic level structure:
public class GameLevel {
private List<Enemy> enemies;
private int levelNumber;
public GameLevel(int levelNumber) {
this.levelNumber = levelNumber;
this.enemies = new ArrayList<>();
generateEnemies();
}
private void generateEnemies() {
for (int i = 0; i < levelNumber * 2; i++) {
enemies.add(new BasicEnemy()); // Adjust difficulty based on level number
}
if (levelNumber > 3) {
enemies.add(new EliteEnemy()); // Introduce EliteEnemy at higher levels
}
}
public List<Enemy> getEnemies() {
return enemies;
}
}
Why Design Levels Specifically?
Good level design creates natural flow and pacing, making the experience more enjoyable. Player decisions can become more strategic when levels vary in layout and challenges.
Key Takeaways
Balancing game difficulty in JavaFX tower defense games is an art that requires both analytical thinking and creativity. By leveraging methods such as dynamic difficulty adjustment, enemy progression, effective resource management, and thoughtful level design, you can create an engaging experience that keeps players coming back for more.
Balancing is not a one-and-done effort but an ongoing process based on continuous feedback and iterations. Happy coding!
For more on game development, check out GameDev.net or explore other resources available that focus on Java and game mechanics.
This post showcases how multiple facets of game design affect players' experiences, emphasizing adaptability and player feedback. By incorporating these strategies in your development workflow, you can create an enjoyable tower defense game that balances challenge and engagement effectively.