Overcoming Common Pitfalls in Automation Testing Success
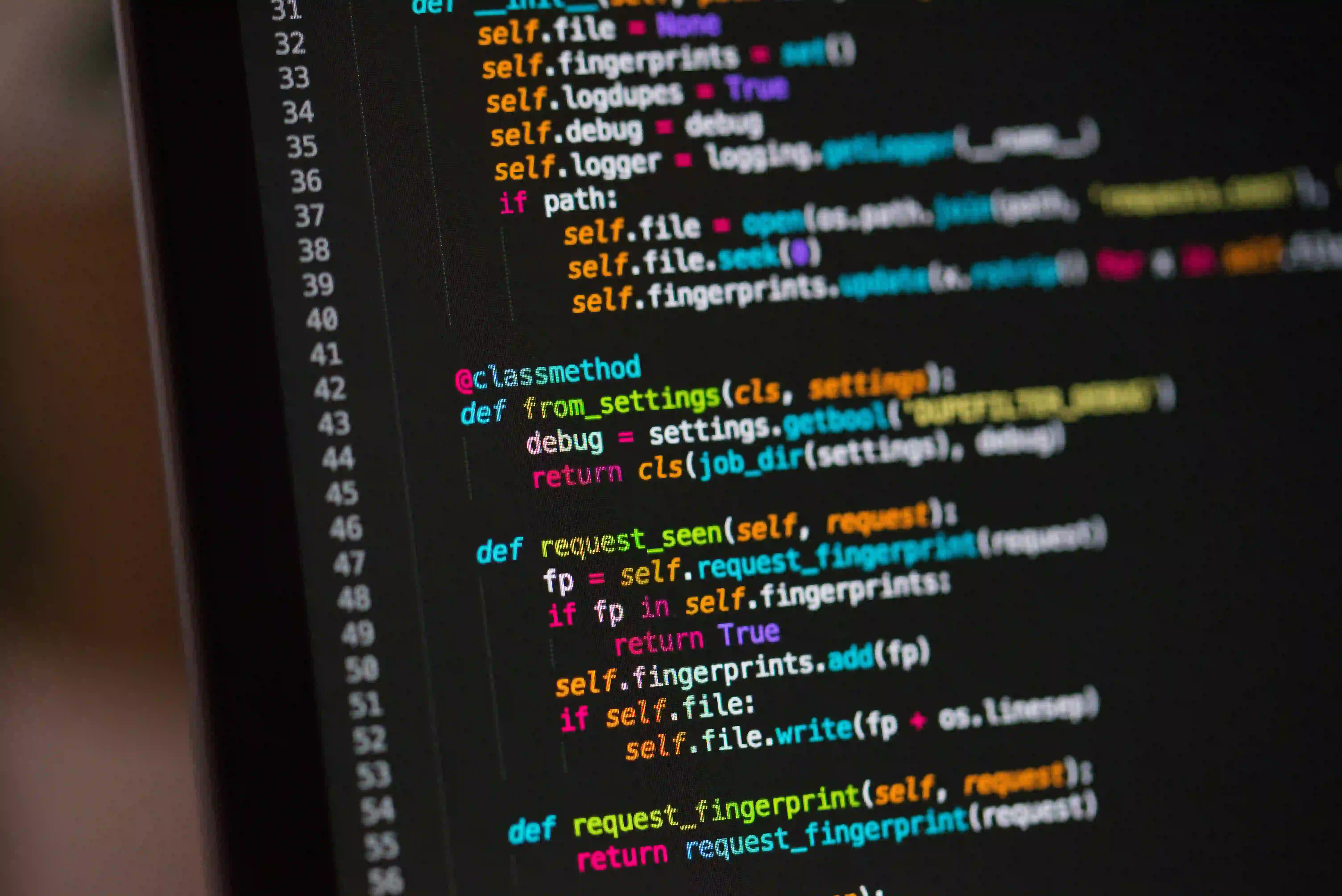
Overcoming Common Pitfalls in Automation Testing Success
Automation testing is an integral part of the software development life cycle. It helps in improving the efficiency, accuracy, and overall quality of software products. However, despite its numerous benefits, many teams encounter challenges during the implementation and execution of automation testing. In this blog post, we'll explore common pitfalls in automation testing and provide actionable strategies to overcome them.
Understanding the Importance of Automation Testing
Before diving into the pitfalls, it’s essential to appreciate the significance of automation testing. Automation testing allows teams to:
- Reduce Testing Time: Automated tests can run significantly faster than manual tests.
- Increase Test Coverage: With automation, you can test more scenarios in less time, covering more aspects of your application.
- Enhance Accuracy: Automated tests eliminate human error, ensuring that tests are performed consistently.
- Enable Continuous Integration: Automated testing is vital for DevOps environments, where code changes are constantly integrated and deployed.
Despite these benefits, teams often fail to fully harness the power of automation testing due to several common pitfalls.
Common Pitfalls in Automation Testing
1. Lack of Clear Objectives
Automation testing must have defined goals. Without clear objectives, teams might end up automating tests that don't contribute to the overall testing strategy.
Tip: Start with identifying what you want to achieve with automation—whether it's reducing testing time, increasing accuracy, or enhancing coverage. For example, if your goal is to reduce testing time, prioritize automating repetitive tasks.
2. Choosing the Wrong Tools
Selecting the appropriate tools for automation is crucial. Many teams dive into automation without researching tools that best fit their needs.
// Example: Using Selenium WebDriver for automating web applications
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class WebDriverExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver.exe");
WebDriver driver = new ChromeDriver();
driver.get("http://www.example.com"); // Navigate to the webpage
driver.quit(); // Close the browser
}
}
In this code snippet, we're using Selenium WebDriver, a popular tool for automation testing of web applications. It allows for dynamic interaction with the web pages and is suitable for testing various browsers.
3. Insufficient Test Case Design
Poorly designed test cases are one of the biggest roadblocks in automation testing. Complex and convoluted test cases can lead to automation failures and unreliable results.
Tip: Design your test cases to be simple and modular. Organize tests by functionality or user scenarios. This modular approach not only allows for easier maintenance but also fosters reusability.
4. Inadequate Maintenance of Automated Test Scripts
Automated tests require regular updates and maintenance. As applications evolve, so too must the corresponding tests. Neglecting to update your tests can lead to failures and unreliable test results.
Tip: Implement a regular schedule for reviewing and updating your test scripts. Allocate dedicated time in your release cycles to refine and enhance the automated tests.
5. Over-Automation
Despite the propensity to automate everything, it's important not to overdo it. Automating every single test can lead to diminishing returns.
Tip: Focus on automating tests that are repetitive, high in volume, and critical for your application. Manual testing can still play a valuable role in exploratory and usability testing.
6. Lack of Skilled Resources
Automation frameworks can be complex, and the lack of skilled testers can hinder the success of your automation efforts. Teams might struggle with scripting languages or may not be familiar with the testing tools in use.
Tip: Invest in training your team on automation frameworks and scripting languages. Consider hiring specialists or incorporating automation training in your onboarding process.
7. Ignoring the Integration with CI/CD Pipelines
Incorporating automation tests into Continuous Integration/Continuous Deployment (CI/CD) workflows can significantly enhance the testing process. However, many teams neglect this essential step.
# Example: A simple CI/CD pipeline configuration using Jenkins
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean install' // Build the application
}
}
stage('Test') {
steps {
sh 'mvn test' // Run the automated tests
}
}
stage('Deploy') {
steps {
sh 'deploy.sh' // Deploy the application
}
}
}
}
In this configuration file, we outline a Jenkins pipeline where tests run immediately after the build stage, ensuring immediate feedback on the validity of the code changes.
8. Not Analyzing Test Results
Automation testing is not simply about running scripts. Analyzing the test results is equally critical to understand the state of your application.
Tip: Establish a process for reviewing test results regularly. Look for patterns in failures to identify if there are issues in the code or if the tests themselves are flawed.
Strategies for Successful Automation Testing
Now that we’ve discussed common pitfalls, let’s outline some strategies to ensure your automation testing is successful.
1. Define a Comprehensive Automation Strategy
Start by creating a clear strategy that encompasses your objectives, tool selection, and resource allocation. Involve the entire team in developing this strategy to gain buy-in and insights.
2. Utilize Version Control
Maintain your test scripts in a version control system like Git. This allows for better collaboration and tracking of changes, especially in teams.
3. Focus on Performance and Load Testing
In addition to functional testing, consider incorporating performance and load testing through automation to ensure your application can handle the expected number of simultaneous users.
4. Encourage Continuous Testing Culture
Promote a culture of continuous testing within your organization. Make sure that automation is seen as a team responsibility and not just a task for a few individuals.
5. Review and Refactor Regularly
Just as we do with production code, commit to regularly reviewing and refactoring your test scripts. Cleaning up the code enhances readability and maintainability.
The Last Word
Automation testing offers numerous advantages, but to truly gain from these benefits, teams must navigate common pitfalls with diligence and strategic planning. By setting clear objectives, using the right tools, designing effective test cases, performing regular maintenance, and integrating tests into CI/CD pipelines, teams can enhance their automated testing efforts and ultimately deliver higher-quality software products.
For more insights on automation testing best practices, consider checking out Automation Testing - A Guide. Stay ahead of challenges and watch your automation efforts flourish!
Reach out in the comments below if you have other experiences or strategies that have worked for you in overcoming obstacles in automation testing!