Understanding Type Erasure: The Challenge of Retaining Generics
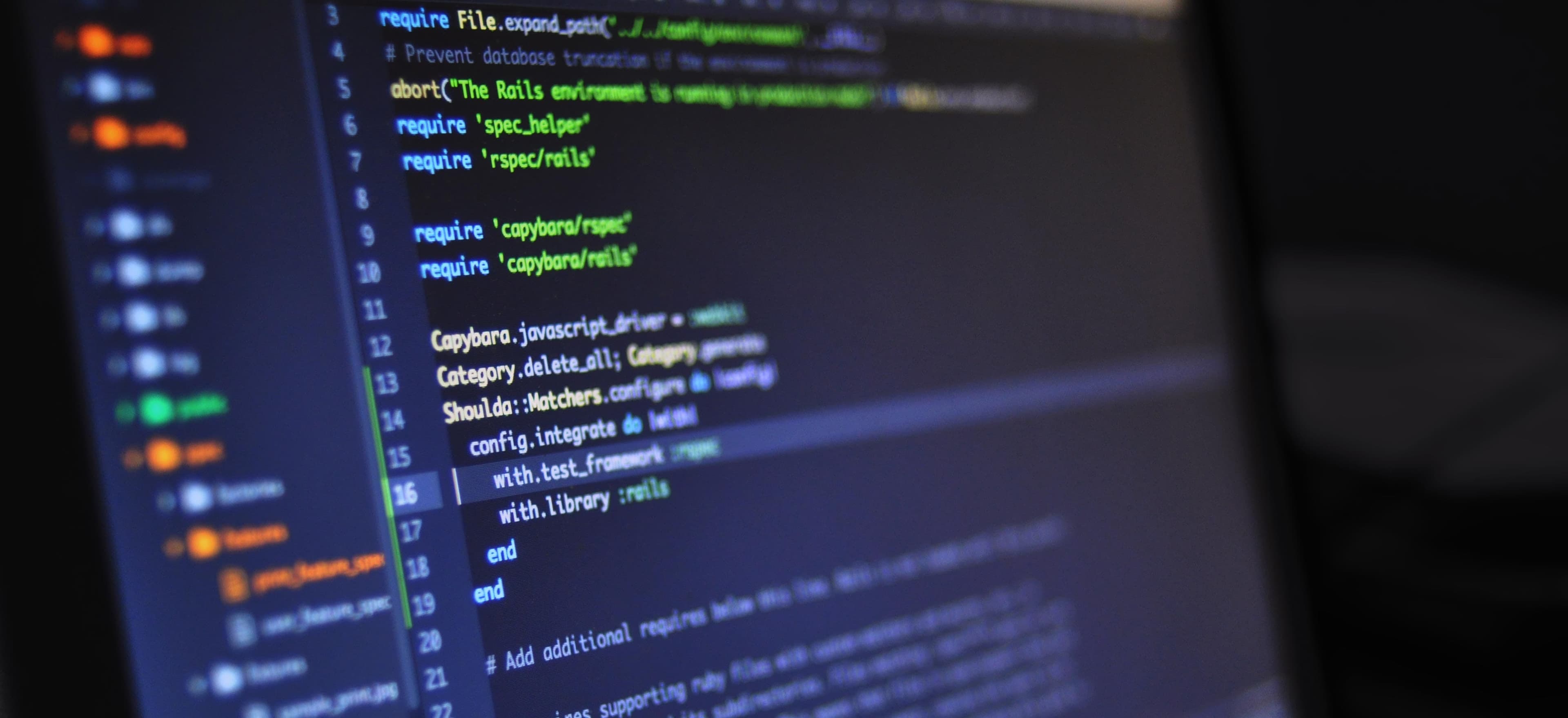
- Published on
Understanding Type Erasure: The Challenge of Retaining Generics in Java
Java is a powerful programming language known for its rich set of features, including object-oriented programming, automatic memory management, and perhaps most notably, generics. Generics allow developers to create classes, interfaces, and methods that operate on specified types, enhancing code reusability and type safety.
However, one of the more complex aspects of Java generics is the concept of type erasure. This blog post aims to shed light on type erasure, its implications, and its overall impact on Java's generics system.
What is Type Erasure?
Type erasure is a process by which the Java compiler removes all information regarding generic types when compiling the code. This means that all the generic types are replaced with their bounds or with Object
if no bounds are specified. Consequently, the generic type information is lost at runtime.
Why Was Type Erasure Implemented?
Type erasure was designed for backward compatibility with older versions of Java (prior to Java 1.5). In those versions, there was no support for generics, and the core principle was to ensure that generics could be added without breaking existing code. The main reasons for employing type erasure are:
- Backward Compatibility: Older codebases could still run without modification.
- Code Simplicity: Maintaining a single compiled version reduces complexity at runtime.
- Polymorphism: It allows Java's data structures and runtime environment to remain compatible.
How Type Erasure Works
Let's explore how type erasure manipulates generics during compilation. Consider the following example:
public class Box<T> {
private T item;
public void setItem(T item) {
this.item = item;
}
public T getItem() {
return item;
}
}
Compiled Form
When the Java compiler processes the code above, it transforms it approximately like this:
public class Box {
private Object item; // T is replaced with Object
public void setItem(Object item) { // T replaced with Object
this.item = item;
}
public Object getItem() { // T replaced with Object
return item;
}
}
As you can see, the specified type T
has been replaced with Object
. This leads to several implications, as we will discuss next.
Implications of Type Erasure
While type erasure provides flexibility and maintains backward compatibility, it also comes with several significant implications:
1. Loss of Type Information
When you use generics, the specific type information does not exist at runtime. This loss can cause runtime exceptions, particularly ClassCastException
. For instance, suppose you have the following code:
Box<String> stringBox = new Box<>();
stringBox.setItem("Hello");
Box<Integer> intBox = new Box<>();
intBox.setItem(123);
String retrievedString = stringBox.getItem(); // No warning
The last line compiles without issue. However, if you try to retrieve a string from an integer box, you'd encounter a runtime error:
String retrievedIntAsString = (String) intBox.getItem(); // ClassCastException
To mitigate such risks, it is beneficial to use bounded type parameters.
2. Cannot Instantiate Generic Types
You cannot create instances of parameterized types due to type erasure. For instance:
public class GenericArray<T> {
private T[] array = new T[10]; // Compilation Error
}
Instead, it's common to create an array of Object
and cast it as needed:
public class GenericArray<T> {
private Object[] array = new Object[10];
public void set(int index, T value) {
array[index] = value; // Safe because we control the type
}
@SuppressWarnings("unchecked")
public T get(int index) {
return (T) array[index]; // Caution: unchecked cast
}
}
3. Type Limitations in Collections
Due to type erasure, certain operations and features of collections may become limited. For example:
List<String> list1 = new ArrayList<>();
List<Integer> list2 = new ArrayList<>();
boolean isSame = list1 instanceof List<String>; // Compilation Error
In this case, even though we know list1
is a List<String>
, there’s no way for the JVM to confirm it at runtime because the type information has been erased.
4. Can't Use Primitive Types
Generics do not work with primitive types directly. You must use their wrapper classes instead. Here’s an example:
Box<int> intBox = new Box<>(); // Compilation Error
Instead, use:
Box<Integer> intBox = new Box<>(); // Correct Usage
Alternatives to Type Erasure
While type erasure served Java well, other languages may utilize different mechanisms for generics. Languages like C# utilize reified generics. In C#, the type information is retained at runtime, which allows for operations that require type information. However, this can lead to increased runtime complexity and potential overhead.
The Bottom Line
Understanding type erasure is crucial for any Java developer working with generics. It explains why certain behaviors occur when using generics and helps in designing robust and type-safe applications. Keep in mind the considerations of type loss, generics instantiation, limitations with collections, and always prefer wrapper classes for primitives.
While Java's type erasure has its downsides, it plays an integral role in the language's evolution, ensuring backward compatibility and stability. By understanding the underlying mechanics, you can write more effective Java code that leverages the strengths of generics while navigating the intricacies of type erasure.
For further reading on generics and type handling in Java, consider reviewing the Java Generics Tutorial.
Happy coding!