Mastering Hibernate's Subselect Annotation: Common Pitfalls
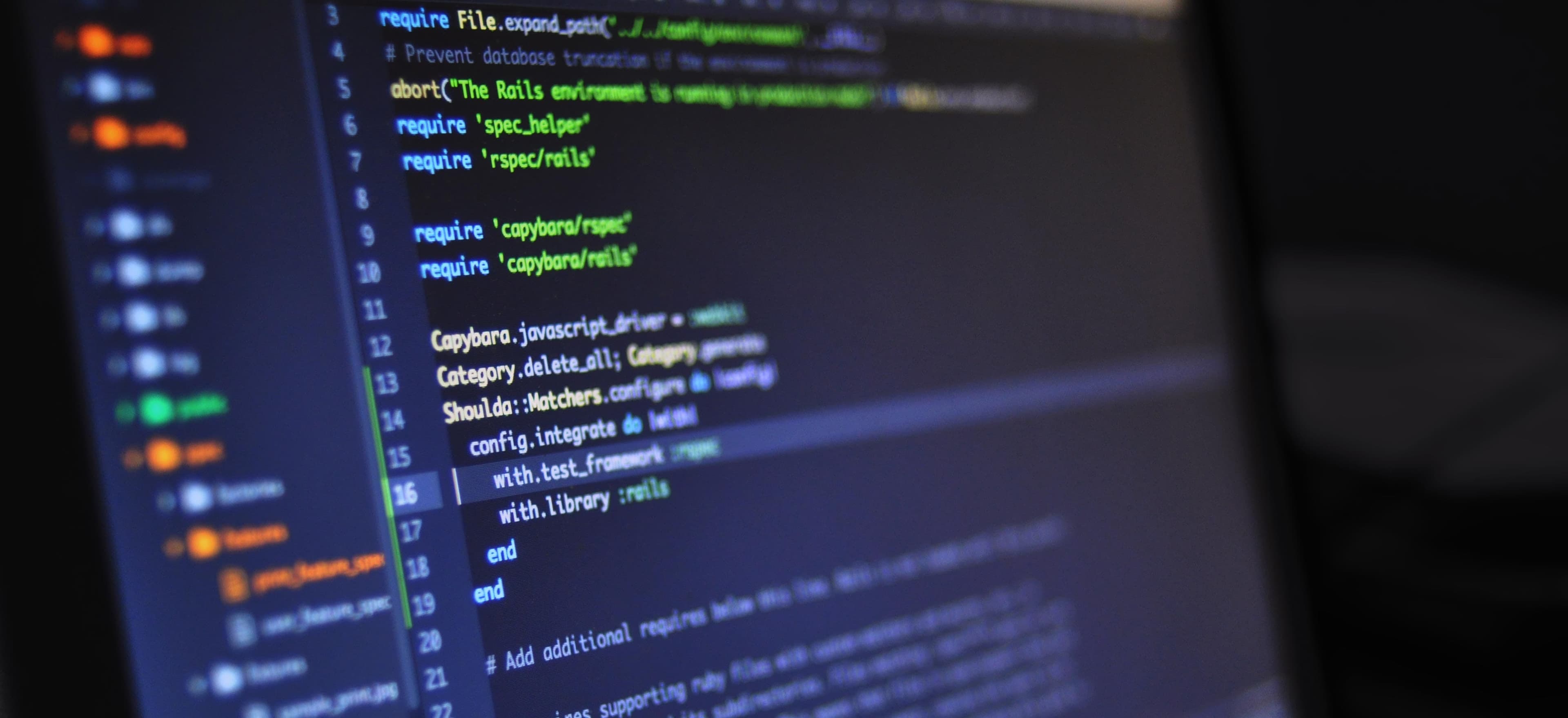
- Published on
Mastering Hibernate's Subselect Annotation: Common Pitfalls
Hibernate is a powerful object-relational mapping (ORM) tool for Java that provides critical functionality for data persistence. Among its many features, the @Subselect
annotation is a critical one that allows developers to define a subselect query directly within an entity mapping. This opens up possibilities for more complex queries and enhances performance, but it can also lead to pitfalls if not correctly handled. In this post, we will explore the @Subselect
annotation in Hibernate, discuss its benefits, and examine common pitfalls that developers encounter.
Understanding the @Subselect Annotation
The @Subselect
annotation is used to create a read-only entity mapping based on the result of a SQL subquery. It essentially allows you to define a view that can be treated as an entity, allowing you to work with projections and subsets of data much more easily.
Here is a basic example of how to use the @Subselect
annotation:
import org.hibernate.annotations.Subselect;
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
@Subselect("SELECT * FROM orders WHERE amount > 100")
public class HighValueOrder {
@Id
private Long id;
private Double amount;
// Getters and setters
}
Why Use the @Subselect Annotation?
- Simplified Queries: The ability to encapsulate complex queries makes your code cleaner and more maintainable.
- Performance Enhancement: Instead of pulling massive datasets into your application, you can filter and aggregate data efficiently at the database level.
- Decoupling: Reduces the coupling between your application and its underlying data structure, allowing changes in the database schema without impacting the codebase directly.
By leveraging the @Subselect
annotation, you can transform how you deal with data fetching in your Java applications.
Common Pitfalls of @Subselect
While using the @Subselect
annotation can be highly beneficial, there are several pitfalls to be aware of. Let’s delve into some of these common mistakes.
Pitfall 1: Performance Issues with Large Datasets
One of the most significant drawbacks of using @Subselect
can be performance-related when dealing with large datasets. Since subselects are executed each time entities are loaded, if not properly indexed or planned, this can lead to slow performance.
Solution: Optimize your subqueries. Use proper indexing on tables involved in the subqueries and regularly analyze query performance using tools like EXPLAIN
in SQL databases.
Pitfall 2: Read-Only Limitations
As noted, entities created with @Subselect
are read-only. This limitation can lead to confusion, particularly for developers expecting these entities to support standard persistence operations (like merges and saves).
Solution: Always design your application logic with an understanding that entities mapped with @Subselect
are not designed for modification. Use transactions in your primary entities for saving data changes.
Pitfall 3: Lack of Proper Documentation and Visibility
Subselect queries can become complex and obscured, making it challenging for other developers (or even future you) to understand the logic later on. This lack of visibility can introduce bugs or misinterpretations of the data.
Solution: Document your subselect queries thoroughly. Provide comments explaining your SQL logic. Additionally, consider creating accompanying diagrams of how different entities relate, so it’s easier to visualize the risk of misinterpretation.
Pitfall 4: Complex Join Conditions
Using complex join conditions in @Subselect
can lead to unexpected results. Did you know that subtle differences in join conditions can change the outcome of your data sets significantly?
@Subselect("SELECT o.id, o.amount, c.name " +
"FROM orders o JOIN customers c ON o.customer_id = c.id " +
"WHERE o.status = 'completed'")
public class CompletedOrders {
// attributes and methods
}
Solution: Always test your subselect queries outside of your application first, directly in your database client tools. Verify the results to ensure correctness.
Pitfall 5: Missing Entity Relationships
When you're using @Subselect
, you may inadvertently overlook defining relationships with other entities. This can lead to LazyInitializationException
or issues fetching associated data.
Solution: Define your relationships clearly within the @Subselect
entities. If you need to fetch related entities, ensure that you handle session and transaction management appropriately.
@Entity
@Subselect("SELECT o.id, o.amount, c.id FROM orders o JOIN customers c ...")
public class OrderWithCustomer {
// attributes
@ManyToOne(fetch = FetchType.LAZY)
private Customer customer;
}
Pitfall 6: Compatibility with Different Database Dialects
Not all databases handle subqueries in the same way. Some optimizations or features may not translate well, causing different behaviors or issues when running your application on different databases.
Solution: Test your @Subselect
queries against all target databases. This ensures expected portability and behavior regardless of where your application is deployed.
Best Practices for Using @Subselect
To get the most out of the @Subselect
annotation while mitigating its pitfalls, consider the following best practices:
- Testing: Always test your subselects in isolation to ensure they return the desired results.
- Optimization: Regularly check and optimize subqueries for performance using the database's built-in tools.
- Documentation: Provide clear documentation and comments to explain complex logic within the subselects.
- Modular Design: Use modular designs to avoid complex joins, breaking down larger queries into simpler, manageable parts wherever possible.
- Stay Updated: Keep abreast of new features in Hibernate and improvements in the ORM ecosystem, as updates may introduce more efficient ways to achieve your desired outcomes.
Key Takeaways
Hibernate’s @Subselect
annotation provides a powerful tool for dealing with complex data queries. However, it is not without its pitfalls. By understanding these challenges and applying best practices, you can effectively use subselects in your Java applications. Always remember that thorough testing and optimization are your best allies in harnessing the full potential of Hibernate.
For more extensive resources on Hibernate, check out the Hibernate ORM documentation and various tutorials available online.
Exploring annotations like @Subselect
not only enhances your Hibernate skills but also provides deeper insights into SQL and database management. Happy coding!
Checkout our other articles