Common Pitfalls in Testing Repositories with DataJpaTest
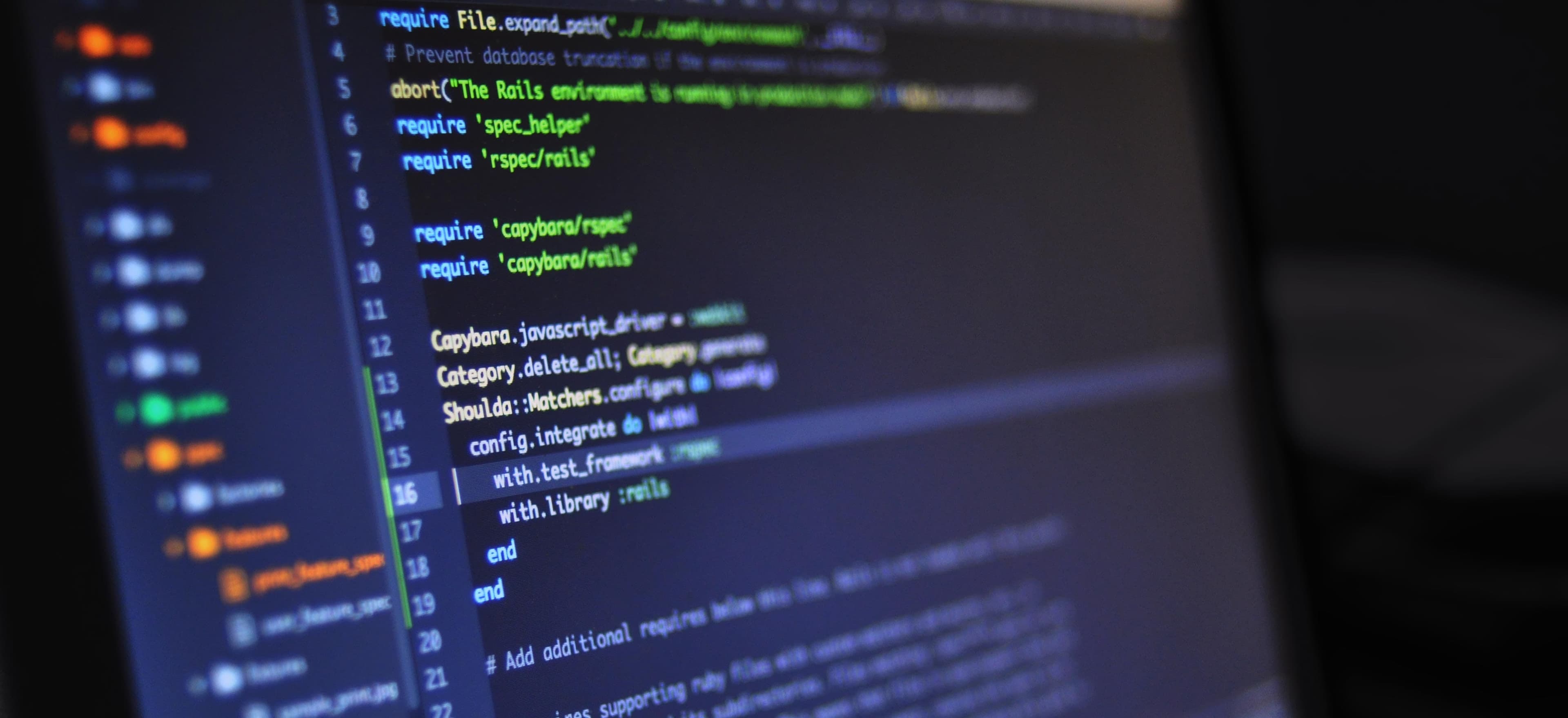
- Published on
Common Pitfalls in Testing Repositories with DataJpaTest
When developing applications in Spring Boot, Data JPA simplifies our data access layer. However, testing these repositories can introduce its share of complexities. This blog post discusses common pitfalls developers encounter while using @DataJpaTest
, along with tips and best practices to avoid these issues. If you're striving for clean, maintainable, and efficient integration tests for your repositories, you're in the right place.
Understanding @DataJpaTest
The @DataJpaTest
annotation is a specialized form of Spring testing that configures an in-memory database and scans for JPA repositories. This greatly simplifies the testing environment for data access layers.
Here is a basic example of how to use @DataJpaTest
:
import org.junit.jupiter.api.Test;
import org.springframework.boot.test.autoconfigure.data.jpa.DataJpaTest;
import org.springframework.beans.factory.annotation.Autowired;
import static org.assertj.core.api.Assertions.assertThat;
@DataJpaTest
public class UserRepositoryTest {
@Autowired
private UserRepository userRepository;
@Test
public void testFindByEmail() {
User user = new User();
user.setEmail("test@example.com");
userRepository.save(user);
User found = userRepository.findByEmail("test@example.com");
assertThat(found).isNotNull();
}
}
This code illustrates a straightforward usage of @DataJpaTest
. We are initializing an in-memory database for testing, saving a user record, and checking if it can be retrieved correctly.
Common Pitfalls
1. Not Resetting the Database State
A frequent issue is the failure to reset the test database state between tests. If a test modifies the database, subsequent tests may fail due to leftover data from previous tests.
Solution:
To ensure isolation, consider using @BeforeEach
to clean up or reset the state:
import org.junit.jupiter.api.BeforeEach;
@BeforeEach
public void setup() {
userRepository.deleteAll();
}
This makes sure each test starts with a clean slate.
2. Ignoring Transactional Rollback
Another pitfall relates to how transactions work in @DataJpaTest
. By default, each test is wrapped in a transaction, and those transactions are rolled back after completion. Forgetting or misconfiguring this behavior can lead to confusion about data persisting between tests.
Solution:
To avoid pitfalls here, make sure you understand the transaction boundaries in your tests. If you want to test commit behavior and have persistent data throughout the test class, you can choose to use @Commit
.
import org.springframework.test.annotation.Commit;
@Commit
public class UserRepositoryIntegrationTest {
// your tests using persistent data
}
3. Not Utilizing @MockBean Properly
When integrating with external services, such as REST APIs, you might want to mock those interactions. @MockBean
comes in handy here, but sometimes it is misapplied.
Solution:
Use @MockBean
wisely and ensure that only the external dependencies you want to control are mocked. For instance, if you have a service class that fetches data from a REST API:
@MockBean
private ExternalApiService externalApiService;
@Test
public void testIntegration() {
when(externalApiService.callApi()).thenReturn(expectedResponse);
}
This allows you to isolate your JPA testing from external influences.
4. Underestimating Spring Profiles
Sometimes developers overlook Spring profiles in their tests, which could lead to unexpected configurations. Misconfigurations could have downstream effects in tests that you might not notice immediately.
Solution:
Use the @ActiveProfiles
annotation to ensure that the proper application context is loaded for your tests.
@ActiveProfiles("test")
@DataJpaTest
public class ProfileSpecificTest {
// test methods here
}
This guarantees that the right properties are used when testing with your repository.
5. Not Testing with Different Database Settings
Relying solely on H2 or any other in-memory database can create a false sense of security. The behavior of the database may not reflect in production, especially with complex queries or specific SQL functions.
Solution:
Whenever possible, consider running tests against a Dockerized version of the same database your production application will use. Tools like Testcontainers help in promoting this practice.
@Testcontainers
@SpringBootTest
public class PostgresRepositoryTest {
// Set up and tests here
}
This methodology can highlight issues that arise due to differences in SQL dialects or database behaviors.
6. Using Assertions Ineffectively
Sometimes developers use assertions that are too lenient, ignoring the need to validate actual data states. This leads to false positives, where tests pass without accurately confirming the database state.
Solution:
Utilize precise assertions that validate actual database states. Instead of merely checking for an object’s existence, confirm its attributes as well.
@Test
public void testFindUser() {
User user = new User();
user.setEmail("test@example.com");
user.setName("Test User");
userRepository.save(user);
User found = userRepository.findByEmail("test@example.com");
assertThat(found.getName()).isEqualTo("Test User");
}
This ensures that your tests are robust and verify real outcomes.
Bringing It All Together
Testing repositories with @DataJpaTest
can streamline your integration testing process if approached thoughtfully. Be mindful of common pitfalls like database state, transaction management, external service mocking, Spring configuration, and assertion accuracy.
As you develop your testing strategies, continue to learn from both successes and failures. Utilize resources like the Spring Testing Documentation to keep abreast of best practices.
By avoiding these pitfalls, you can write more effective, reliable, and maintainable tests for your Spring Data JPA repositories. Happy testing!
Further Reading
With this comprehensive understanding of testing repositories with @DataJpaTest
, you are better equipped to tackle challenges and ensure your application remains bug-free and organized.