Common Mistakes in Implementing JDK Design Patterns
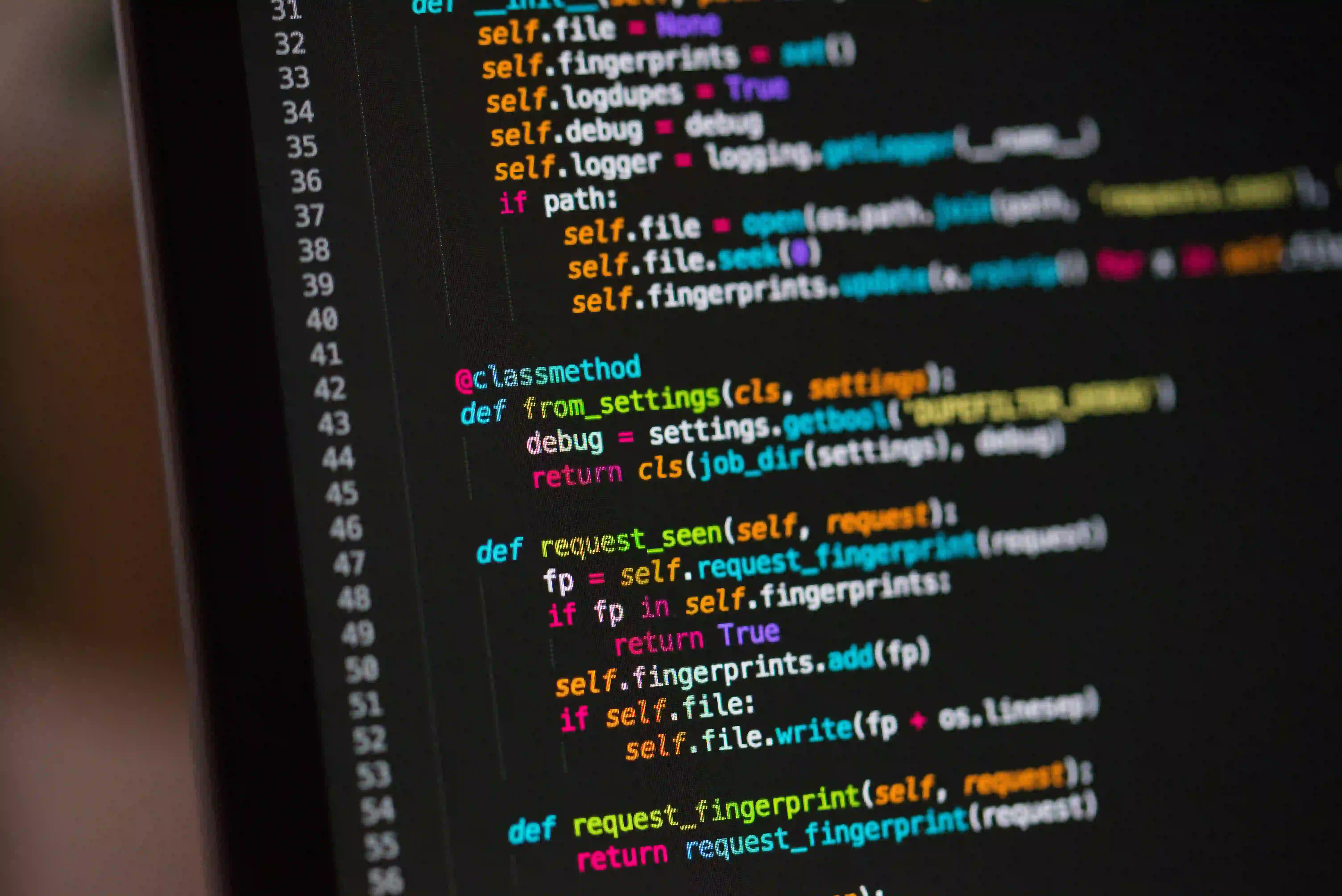
Common Mistakes in Implementing JDK Design Patterns
Design patterns are reusable solutions to common problems that software developers face. They are best practices that efficiently solve problems in a well-defined manner. In the Java Development Kit (JDK), several design patterns are commonly used due to their robust, flexible design. However, even experienced programmers can fall into common pitfalls while implementing these patterns. Understanding these mistakes can enhance your development practices, leading to cleaner, more maintainable code.
Understanding Design Patterns
Design patterns are categorized into three main types:
- Creational Patterns: Deals with object creation mechanisms. Examples: Singleton, Factory, Builder.
- Structural Patterns: Focuses on object composition. Examples: Adapter, Composite, Proxy.
- Behavioral Patterns: Involves communication between objects. Examples: Observer, Strategy, Command.
The Importance of Implementing Patterns Correctly
Implementing design patterns incorrectly can lead to increased complexity, degraded performance, and a steep learning curve for your team. This blog post aims to explore the most common mistakes developers encounter when implementing JDK design patterns, along with tips for executing them effectively.
1. Overusing Design Patterns
One of the most frequent errors is overusing design patterns. Sometimes developers implement a design pattern where a simpler solution would suffice. For instance, using the Strategy Pattern in situations where a simple if-else
statement would do is unnecessary and adds complexity.
Example
public interface PaymentStrategy {
void pay(int amount);
}
public class CreditCardPayment implements PaymentStrategy {
public void pay(int amount) {
// Logic for credit card payment
}
}
public class CashPayment implements PaymentStrategy {
public void pay(int amount) {
// Logic for cash payment
}
}
// Usage
public class ShoppingCart {
private PaymentStrategy paymentStrategy;
public void setPaymentStrategy(PaymentStrategy strategy) {
this.paymentStrategy = strategy;
}
public void checkout(int amount) {
paymentStrategy.pay(amount);
}
}
Why Avoid Overuse?
While the usage of the Strategy Pattern might seem ideal for extensibility, consider if you will need more than two or three payment methods. If not, merely using conditionals could make the code more readable and maintainable.
2. Ignoring the Open/Closed Principle
The Open/Closed Principle suggests that software entities should be open for extension but closed for modification. However, many developers modify existing classes rather than extending them.
Example
For instance, if you have an existing class that defines different shape classes and modifies it every time a new shape is added, you're ignoring this principle.
public class AreaCalculator {
public double calculateArea(Shape shape) {
if (shape instanceof Circle) {
return Math.PI * Math.pow(((Circle) shape).getRadius(), 2);
}
// More shapes...
}
}
How to Fix?
Utilize polymorphism to extend behavior instead of changing the class.
public abstract class Shape {
public abstract double calculateArea();
}
public class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double calculateArea() {
return Math.PI * Math.pow(radius, 2);
}
}
// Usage
public class AreaCalculator {
public double calculateArea(Shape shape) {
return shape.calculateArea();
}
}
3. Poor Naming Conventions
Another mistake is using vague or misleading names when implementing design patterns. Names should clearly express the intention of the code. This is particularly crucial in large teams or projects.
Example
Instead of naming your classes Manager
or Worker
, use more expressive names like OrderManager
or EmployeeWorker
. Clarify the roles of your classes.
4. Inappropriate Use of Singleton
The Singleton pattern ensures a class has only one instance and provides a global point of access to it. However, over-reliance on Singletons can lead to tightly coupled code and global state, making testing difficult.
Example
public class ConfigurationManager {
private static ConfigurationManager instance;
private ConfigurationManager() {
// Load configuration
}
public static ConfigurationManager getInstance() {
if (instance == null) {
instance = new ConfigurationManager();
}
return instance;
}
}
Better Practices
Consider dependency injection frameworks (like Spring) to manage singletons instead of handling them yourself. This approach reduces coupling and improves testability.
5. Not Considering Thread Safety
When using design patterns in a multithreading environment, it is crucial to ensure that your implementation is thread-safe. Failure to do so can result in unpredictable behavior.
Example
Using the Singleton pattern without synchronization is a common mistake.
public class ThreadSafeSingleton {
private static ThreadSafeSingleton instance;
private ThreadSafeSingleton() {
// Constructor
}
public static synchronized ThreadSafeSingleton getInstance() {
if (instance == null) {
instance = new ThreadSafeSingleton();
}
return instance;
}
}
Alternative Approach
Consider using the Bill Pugh Singleton Design, which leverages static inner classes for lazy initialization while remaining thread-safe:
public class BillPughSingleton {
private BillPughSingleton() {}
private static class SingletonHelper {
private static final BillPughSingleton INSTANCE = new BillPughSingleton();
}
public static BillPughSingleton getInstance() {
return SingletonHelper.INSTANCE;
}
}
6. Failing to Understand Context
Implementation of design patterns requires a deep understanding of the context in which they will be used. Blindly following patterns without analyzing specific project requirements can lead to poor results.
Best Practices
- Evaluate Needs: Before implementing a design pattern, analyze whether it genuinely fits the situation.
- Prototyping: Create prototypical models to test the usability of the design pattern before finalizing it in your application.
Closing the Chapter
Implementing design patterns effectively can significantly improve code quality, maintainability, and readability. However, developers need to be wary of common pitfalls such as overuse, poor naming conventions, misunderstanding principles like the Open/Closed principle, improper use of Singleton, thread safety issues, and inadequate contextual analysis.
To learn more about design patterns, you might find resources like Refactoring: Improving the Design of Existing Code by Martin Fowler and Design Patterns: Elements of Reusable Object-Oriented Software by Erich Gamma et al. particularly useful.
For further reference on design patterns, check out:
- Java Design Patterns on Baeldung
- Java Design Patterns on DZone
By being aware of these common mistakes and employing best practices, you can leverage the full power of design patterns in your Java applications.