Troubleshooting AOP Configuration in Spring Applications
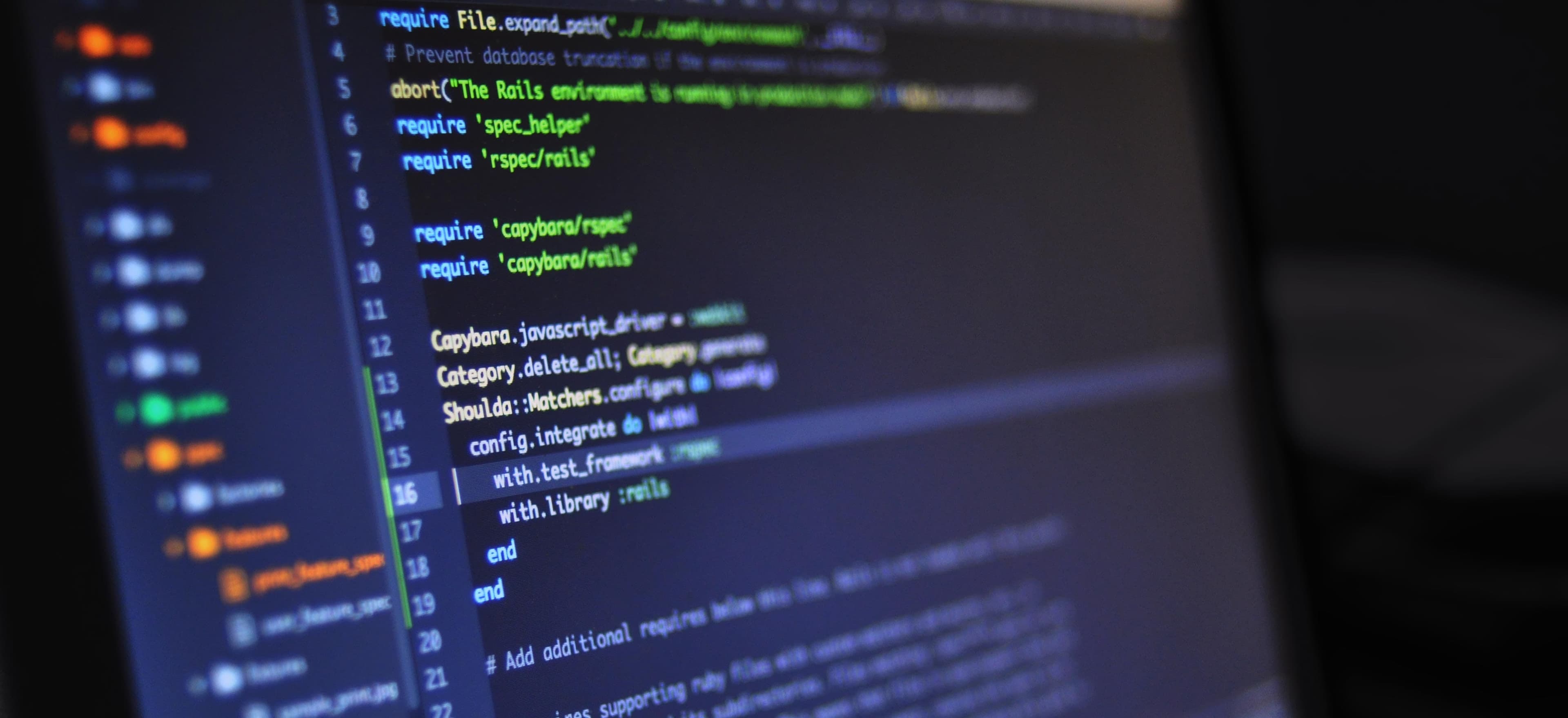
- Published on
Troubleshooting AOP Configuration in Spring Applications
Aspect-Oriented Programming (AOP) is a powerful feature of Spring that allows developers to define cross-cutting concerns separate from business logic. These concerns can include logging, security, or transaction management. However, troubleshooting AOP configuration can sometimes be challenging. This blog post will guide you through common AOP configuration issues, provide practical solutions, and include code snippets for clarity.
Understanding AOP in Spring
AOP enables you to define "aspects" that encapsulate behaviors that affect multiple classes. For example, an aspect can be used to log the execution of methods throughout your application without modifying the underlying business logic.
Key Concepts of AOP
- Aspect: A modularization of a concern that cuts across multiple classes.
- Join Point: A point in the execution of a program, such as method execution.
- Advice: Action taken at a join point. Types include before, after, and around.
- Pointcut: An expression that matches join points.
Common AOP Configuration Issues
- AOP Annotations Not Recognized
- Aspect Not Applied
- Circular Dependencies
- Proxy Issues
1. AOP Annotations Not Recognized
If your AOP annotations are not being recognized, ensure that you've enabled AspectJ support. You can do this by annotating your configuration class with @EnableAspectJAutoProxy
.
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.EnableAspectJAutoProxy;
@Configuration
@EnableAspectJAutoProxy
public class AppConfig {
}
Why? The annotation instructs Spring to look for @Aspect
classes, enabling AOP functionality.
2. Aspect Not Applied
If your aspects are not being applied, there are several factors to consider:
- Component Scanning: Ensure your aspect class is annotated with
@Aspect
and is within the component-scan path.
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void logBefore() {
System.out.println("A method is about to be executed");
}
}
Why? The combination of @Aspect
and @Component
enables automatic detection by Spring's component scanning.
- Pointcut Expression: Ensure your pointcut expression correctly matches the methods you want to advise.
If your pointcut is too restrictive, your advice won't be applied.
3. Circular Dependencies
Circular dependencies can occur if your aspect relies on a bean that also depends on the aspect. Spring cannot create such a loop, resulting in a BeanCurrentlyInCreationException
.
Solution: Refactor to avoid circular dependencies or use @Lazy
on one of the beans to break the cycle.
4. Proxy Issues
Spring AOP operates primarily through proxying. If you're using classes rather than interfaces, you may encounter issues.
Solution: Use Interface-based Proxies
To enforce the best practices, it is recommended to program through interfaces. Here’s an example.
public interface UserService {
void createUser(String name);
}
@Service
public class UserServiceImpl implements UserService {
public void createUser(String name) {
System.out.println("User created: " + name);
}
}
Why? If the service implements an interface, Spring can create a proxy using either JDK dynamic proxies or CGLIB, depending on whether the class only implements interfaces.
Example AOP Configuration
Let’s take a look at a complete example of a Spring AOP setup.
Application Configuration
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.EnableAspectJAutoProxy;
@Configuration
@ComponentScan(basePackages = "com.example")
@EnableAspectJAutoProxy
public class ApplicationConfig {
}
Service Implementation
import org.springframework.stereotype.Service;
@Service
public class UserServiceImpl implements UserService {
public void createUser(String name) {
System.out.println("User created: " + name);
}
}
Logging Aspect
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.aspectj.lang.annotation.After;
import org.aspectj.lang.annotation.Pointcut;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class LoggingAspect {
@Pointcut("execution(* com.example.service.*.*(..))")
public void serviceLayer() {}
@Before("serviceLayer()")
public void logBefore() {
System.out.println("Method is about to be called.");
}
@After("serviceLayer()")
public void logAfter() {
System.out.println("Method has been called.");
}
}
Main Application Class
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class MainApp {
public static void main(String[] args) {
ApplicationContext context = new AnnotationConfigApplicationContext(ApplicationConfig.class);
UserService userService = context.getBean(UserService.class);
userService.createUser("John Doe");
}
}
Testing and Debugging AOP
- Check Logs: Ensure that your logging aspect prints logs as expected. If not, verify the pointcut and aspect configuration.
- Debugging: Use breakpoints to step into your aspect methods.
- Profiling: Use a profiler to understand method calls and identify whether advices are executed.
To Wrap Things Up
Troubleshooting AOP configuration in Spring applications may seem daunting, but by adhering to best practices and understanding common pitfalls, you can effectively navigate these challenges. Always remember to keep aspects concise, check your pointcut expressions diligently, and ensure that your AOP setup fits well within your service design.
For further learning on Spring AOP, consider checking these resources:
- Spring AOP Documentation
- AspectJ Primer
Feel free to ask questions or share your own experiences with AOP in the comments below! Happy coding!