Conquering Code Clutter: Ditching Anonymous Classes
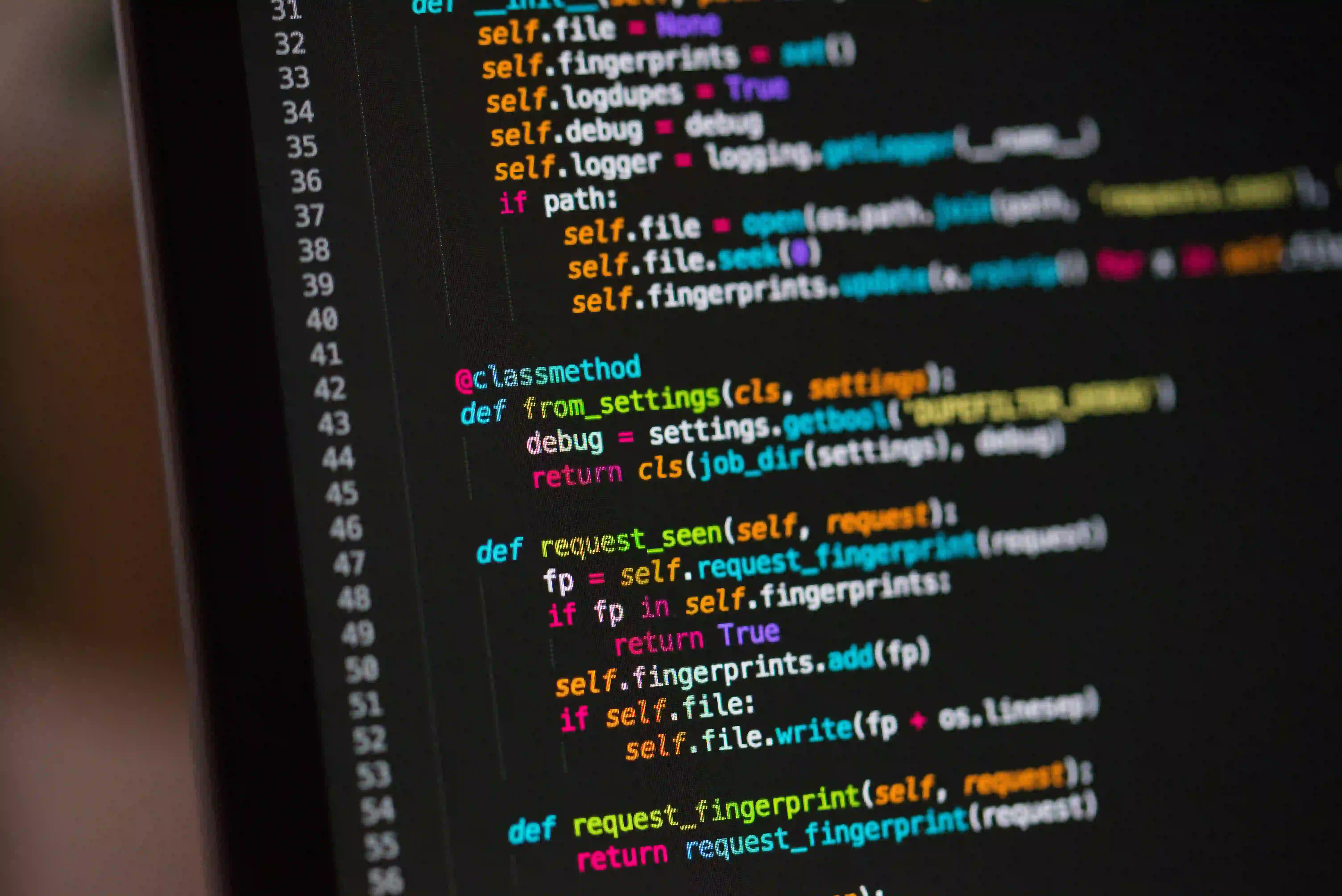
Conquering Code Clutter: Ditching Anonymous Classes in Java
Java is a versatile language that offers several ways to implement functionalities. One of those ways is through anonymous classes. While anonymous classes can be useful for certain tasks, they often introduce complexity and obfuscation. In this post, we'll delve into why you might want to ditch anonymous classes, present cleaner alternatives, and embrace more readable and maintainable code.
What are Anonymous Classes?
In Java, an anonymous class is an inner class without a name. It's often used for instantiating classes that may not require a full-fledged class definition. You typically encounter anonymous classes when implementing interfaces or extending a class in a concise manner.
Here’s a basic example of using an anonymous class:
import javax.swing.*;
public class AnonymousClassDemo {
public static void main(String[] args) {
JButton button = new JButton("Click Me!");
// Anonymous Class Implementation
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
System.out.println("Button Clicked!");
}
});
JFrame frame = new JFrame();
frame.setSize(300, 200);
frame.getContentPane().add(button);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
Why Use Anonymous Classes?
Anonymous classes allow for quick implementations of interfaces and abstract classes right at the point of usage. They are convenient for simple, one-time function implementations, like handling events in GUI applications.
However, using them too frequently can lead to a number of issues:
- Code Readability: They can obscure the logic and flow of your code.
- Maintainability: It can be challenging to update logic that’s buried within an anonymous class. If a class becomes more than just a few lines of code, it should likely be a named class.
- Reusability: Anonymous classes can’t be reused; they are tied directly to where they are defined.
The Case Against Anonymous Classes
1. Unreadable Code
The inline implementation of logic often leads to confusion. Imagine trying to read a method only to find multiple anonymous classes tangled within it. The following code snippet illustrates this problem:
public void performAction() {
new Service().execute(new Callback() {
@Override
public void onSuccess() {
// complex logic here
}
});
}
At first glance, it can be hard to determine the action taken upon success because the code lacks a clear structure.
2. Debugging Difficulties
Anonymous classes can obscure stack traces during exceptions, making debugging a daunting task. When you encounter a runtime error, the stack trace may refer to "X.class" instead of a meaningful name, complicating your debugging efforts.
Cleaner Alternatives to Anonymous Classes
The good news? Java provides several alternatives that can keep your code clean, readable, and maintainable.
1. Named Inner Classes
Instead of using anonymous classes, consider defining named inner classes. They offer the same functionality but with clearer structure.
class ButtonClickListener implements ActionListener {
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("Button Clicked!");
}
}
// Usage
JButton button = new JButton("Click Me!");
button.addActionListener(new ButtonClickListener());
2. Lambda Expressions
Introduced in Java 8, lambda expressions provide a compact way to express instances of single-method interfaces (functional interfaces). They make your code more concise and easier to understand.
Here’s how the previous example looks using a lambda expression:
JButton button = new JButton("Click Me!");
button.addActionListener(e -> System.out.println("Button Clicked!"));
Advantages of Lambda Expressions
- Conciseness: The code is shorter and more direct.
- Readability: Clearer intention of what the block is doing.
- Contextual Scope: Lambdas can access the local variables directly.
Comparing the Approaches
To understand this better, let's summarize the differences between anonymous classes, named inner classes, and lambda expressions:
| Feature | Anonymous Class | Named Inner Class | Lambda Expression | |----------------------|-----------------------|------------------------|-------------------------| | Readability | Low | Medium | High | | Conciseness | Medium | Low | High | | Reusability | No | Yes | No | | Debugging Ease | Difficult | Easier | Easy |
When Should You Use Anonymous Classes?
Despite their drawbacks, there are situations where anonymous classes can be suitable, such as when:
- The logic is genuinely small and straightforward.
- You are tasked with quick prototyping.
However, if your application scales or becomes complex, you should consider a transition to more structured alternatives.
The Closing Argument
While anonymous classes serve a purpose, their overuse can lead to code that is cluttered and difficult to maintain. By utilizing named inner classes and lambda expressions, you can enhance readability and maintainability, ultimately leading to a more robust Java application.
Are you still using anonymous classes in your code? If so, consider refactoring them to improve clarity and reduce complexity. For more in-depth resources on Java programming, check out Oracle's Java Documentation.
Happy coding!