Why Using finalize() in Java Can Lead to Serious Issues
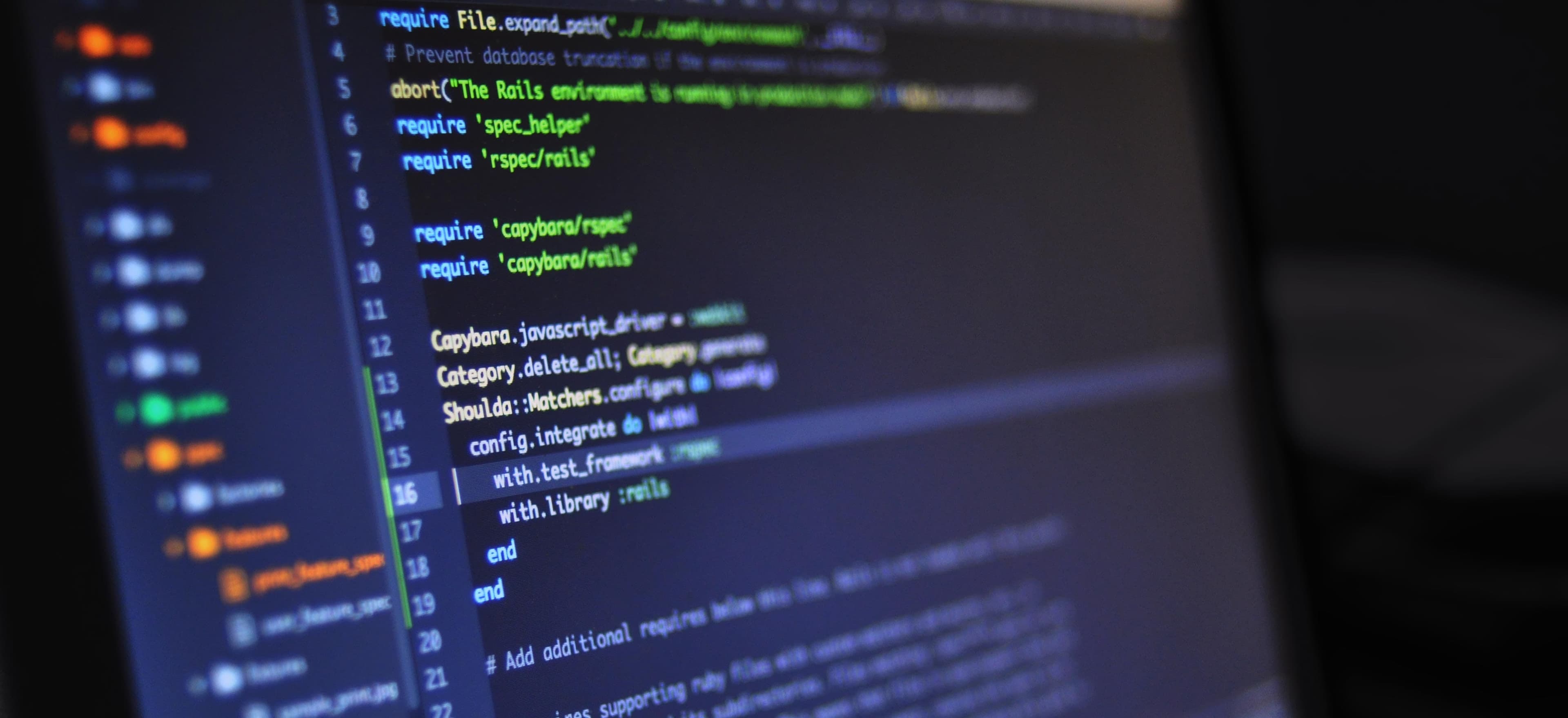
- Published on
Why Using finalize() in Java Can Lead to Serious Issues
In Java, the finalize()
method is a special method that belongs to the Object
class and is invoked by the garbage collector when it determines that there are no more references to an object. For many developers, finalize()
might seem like a convenient way to handle cleanup during garbage collection. However, its use can lead to serious issues, making it a controversial topic among Java developers. In this blog post, we will explore the implications, drawbacks, and better alternatives for resource management in Java.
What is the finalize() Method?
The finalize()
method is defined in the Object
class, which means every Java object inherits it. The typical purpose of overriding this method is to perform cleanup operations before the object is reclaimed by the garbage collector.
@Override
protected void finalize() throws Throwable {
try {
// Clean up resources
System.out.println("Finalizing the object...");
} finally {
super.finalize();
}
}
Why Should You Avoid finalize()?
While finalize()
might seem useful, it is fraught with problems:
-
Unpredictable Timing: The execution of
finalize()
during garbage collection cannot be predicted. It may not run for a long time after the object becomes unreachable, causing resource leaks. -
Performance Penalties: When an object is finalized, it may be promoted to the "finalizer queue," delaying the release of resources and slowing down your application.
-
No Guarantees: There are no guarantees that
finalize()
will be executed. If the application exits abruptly, the garbage collector may not have a chance to invoke thefinalize()
method. -
Potential for Resource Leaks: If your objects hold onto other resources (like file handles, database connections, etc.), relying on
finalize()
increases the risk of resource leaks. These may lead to performance degradation or application failure. -
Incompatibility with Modern Garbage Collectors: Java's modern garbage collectors do not rely on finalization, making it redundant and potentially misleading.
The Case Against finalize()
To illustrate these points better, let’s look at an example where we manage a database connection using finalize()
.
public class DatabaseConnection {
private Connection connection;
public DatabaseConnection() {
this.connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "user", "password");
}
@Override
protected void finalize() throws Throwable {
try {
if (connection != null && !connection.isClosed()) {
System.out.println("Closing database connection in finalize...");
connection.close(); // This may never actually run
}
} finally {
super.finalize();
}
}
}
Problems in this Code
- In the above example, there is no guarantee when or if the
finalize()
method will be called to close the database connection. - If many instances of
DatabaseConnection
objects are created and quickly go out of scope, you may exhaust database resources leading to application crashes.
Better Alternatives to finalize()
Use try-with-resources
Introduced in Java 7, the try-with-resources statement ensures that each resource is closed at the end of the statement. This provides a much safer alternative to finalize()
.
public class DatabaseConnection implements AutoCloseable {
private Connection connection;
public DatabaseConnection() throws SQLException {
this.connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "user", "password");
}
public void executeQuery(String query) throws SQLException {
Statement stmt = connection.createStatement();
ResultSet rs = stmt.executeQuery(query);
// Process the ResultSet...
}
@Override
public void close() throws SQLException {
if (connection != null && !connection.isClosed()) {
System.out.println("Closing database connection...");
connection.close();
}
}
}
// Using try-with-resources
try (DatabaseConnection dbConnection = new DatabaseConnection()) {
dbConnection.executeQuery("SELECT * FROM table");
} catch (SQLException e) {
e.printStackTrace();
}
Advantages of try-with-resources
-
Automatic Resource Management: The framework automatically invokes the
close()
method of resources that implementAutoCloseable
orCloseable
, ensuring timely resource cleanup. -
Easier to Understand and Maintain: It makes the code cleaner and avoids boilerplate code associated with manually closing resources.
-
Predictable Exceptions: Any exceptions thrown while closing are suppressed and can be handled or logged appropriately.
References
If you wish to delve deeper into resource management in Java, consider reading about try-with-resources and the implications on performance and reliability.
Final Thoughts
While the finalize()
method offers a way to perform cleanup, its drawbacks far outweigh its benefits. Relying on it can lead to unpredictable behavior, resource leaks, and performance issues in your Java applications. Instead, adopting modern resource management practices such as try-with-resources will minimize complexity and promote sound coding practices.
By transitioning away from finalize()
, you'll write cleaner, more efficient Java code and embrace the future of the Java ecosystem. Remember, maintaining your code's reliability and performance is the key to success in software development.
Feel free to share your thoughts in the comments below or reach out with your experiences concerning resource management in Java. Happy coding!