Mastering LazyInitializationException in JPA: A Quick Guide
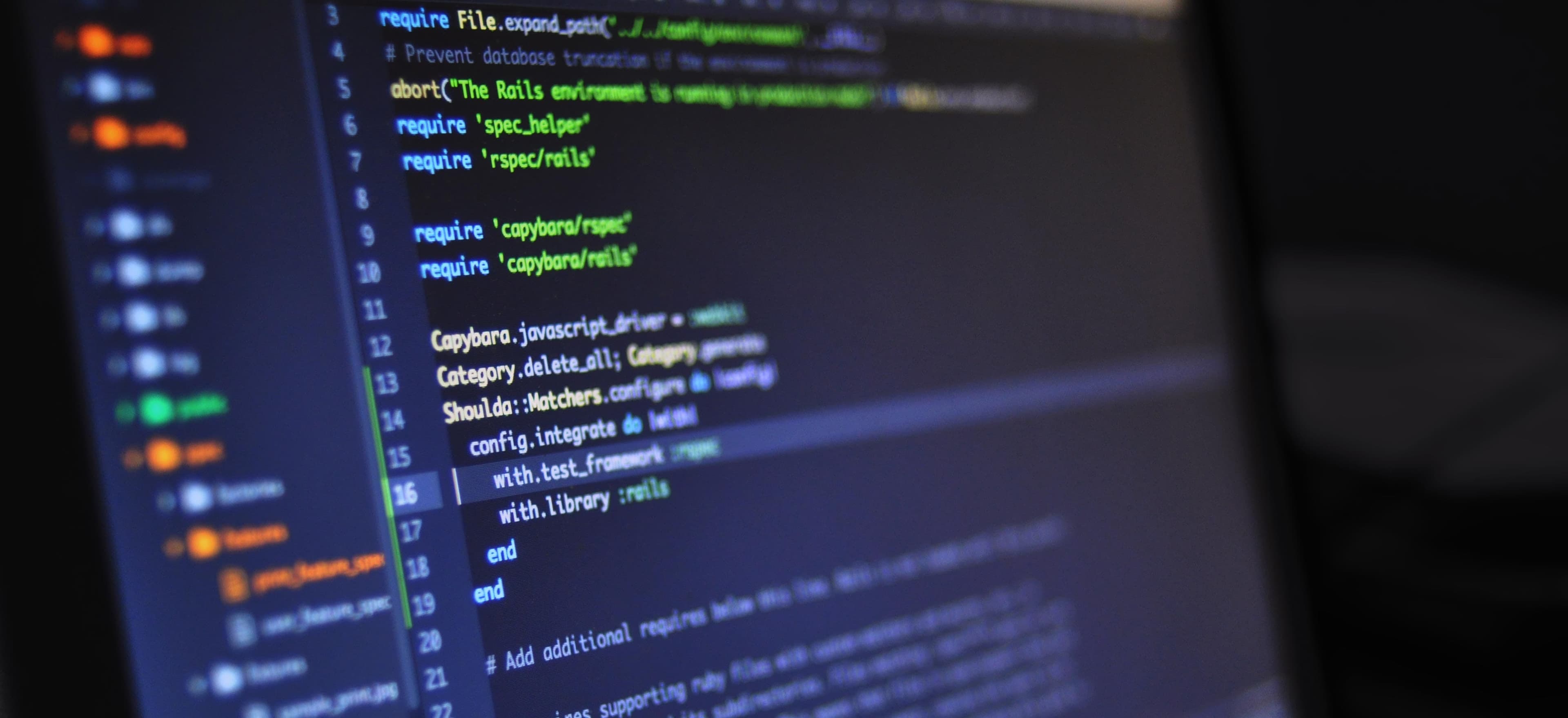
- Published on
Mastering LazyInitializationException in JPA: A Quick Guide
Java Persistence API (JPA) is widely utilized in Java applications to manage relational data. Although it simplifies data operations, developers often encounter challenges, one of the most notorious being the LazyInitializationException
. Understanding and mastering this exception is crucial for every JPA programmer. This guide will explore what LazyInitializationException
is, why it occurs, and how to effectively prevent it.
What is LazyInitializationException?
The LazyInitializationException
occurs when your code attempts to access a lazily fetched association outside the context of an active Hibernate session. When an entity is fetched along with its relationships in a JPA application, those relationships can be marked for "lazy" loading. This means the data for these relationships will not be fetched immediately from the database but will only be retrieved when explicitly accessed.
Example of Lazy Loading
Consider the following example where a Post
entity has a list of Comment
entities.
@Entity
public class Post {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String content;
@OneToMany(mappedBy = "post", fetch = FetchType.LAZY)
private List<Comment> comments;
}
In this snippet, the comments
attribute is set to be loaded lazily. This means that when you fetch a Post
instance from the database, the comments
are not retrieved right away.
Why Does LazyInitializationException Occur?
The exception arises during an attempt to access the lazily loaded properties (like comments
) after the Hibernate session is closed. Consider this scenario:
public Post getPost(Long id) {
Post post = entityManager.find(Post.class, id);
// Session is still open within this context
return post;
}
// Later, outside the transaction context:
Post post = getPost(1L);
System.out.println(post.getComments().size()); // LazyInitializationException
Here, after getPost
has executed, the session associated with the entityManager
is closed. If the code attempts to access post.getComments()
, Hibernate throws a LazyInitializationException
since there is no active session to retrieve that data from the database.
How to Prevent LazyInitializationException
Preventing LazyInitializationException
can be achieved in several ways:
1. Open Session in View Pattern
This is a common design pattern that maintains the Hibernate session open until the view layer has been fully rendered.
public void viewPost(Long id) {
// Transaction begins; session is open
Post post = entityManager.find(Post.class, id);
// All necessary operations occur within this method
// view logic occurs here, session remains open
}
While this pattern provides access to lazy-loaded properties, it may lead to performance issues if not carefully managed.
2. Eager Loading
Modify the fetch type from LAZY
to EAGER
. This way, JPA loads the associated entities at the same time as the parent entity:
@OneToMany(mappedBy = "post", fetch = FetchType.EAGER)
private List<Comment> comments;
Eager loading ensures comments are always loaded, but it can lead to increased data transfer and slower performance if the entity graph is large.
3. Join Fetching
Using JPQL or Criteria API, you can specify that you want to fetch associations using a JOIN
clause. Here's a JPQL example:
String jpql = "SELECT p FROM Post p JOIN FETCH p.comments WHERE p.id = :id";
Post post = entityManager.createQuery(jpql, Post.class)
.setParameter("id", id)
.getSingleResult();
In this example, both the Post
and its comments
are fetched in a single query, preventing the LazyInitializationException
.
4. DTO Projections
You can also create Data Transfer Objects (DTOs) to load only what you need. Here's a simple DTO:
public class PostDTO {
private Long id;
private String content;
private List<String> commentContents;
public PostDTO(Long id, String content, List<String> commentContents) {
this.id = id;
this.content = content;
this.commentContents = commentContents;
}
}
You can fetch the DTO like this:
String jpql = "SELECT new PostDTO(p.id, p.content, c.content) "
+ "FROM Post p LEFT JOIN p.comments c "
+ "WHERE p.id = :id";
PostDTO postDTO = entityManager.createQuery(jpql, PostDTO.class)
.setParameter("id", id)
.getSingleResult();
In this case, data is transferred efficiently and avoids over-fetching.
5. Use a Transaction
Ensure that your entity access is done within an active transaction context. Spring Framework, for example, makes this easy:
@Transactional
public Post fetchPost(Long id) {
Post post = entityManager.find(Post.class, id);
return post; // Hibernate Session is active
}
When leveraging Spring’s @Transactional
, the session remains open throughout the execution of the method.
Lessons Learned
The LazyInitializationException
is a common stumbling block in JPA development, but it can be managed effectively through various strategies. From open session patterns to eager fetching and DTOs, each method has its ideal use cases.
For a deeper understanding of JPA and its workings, consider exploring Hibernate's official documentation or looking at JPA in Spring for practical implementation scenarios. Understanding these concepts will not only make you proficient but also improve the performance and reliability of your Java applications.
By employing these techniques thoughtfully, you can master the challenges posed by LazyInitializationException
and ensure a smoother development experience while working with JPA.