Resolving ClassLoader Conflicts: A Java Developer's Guide
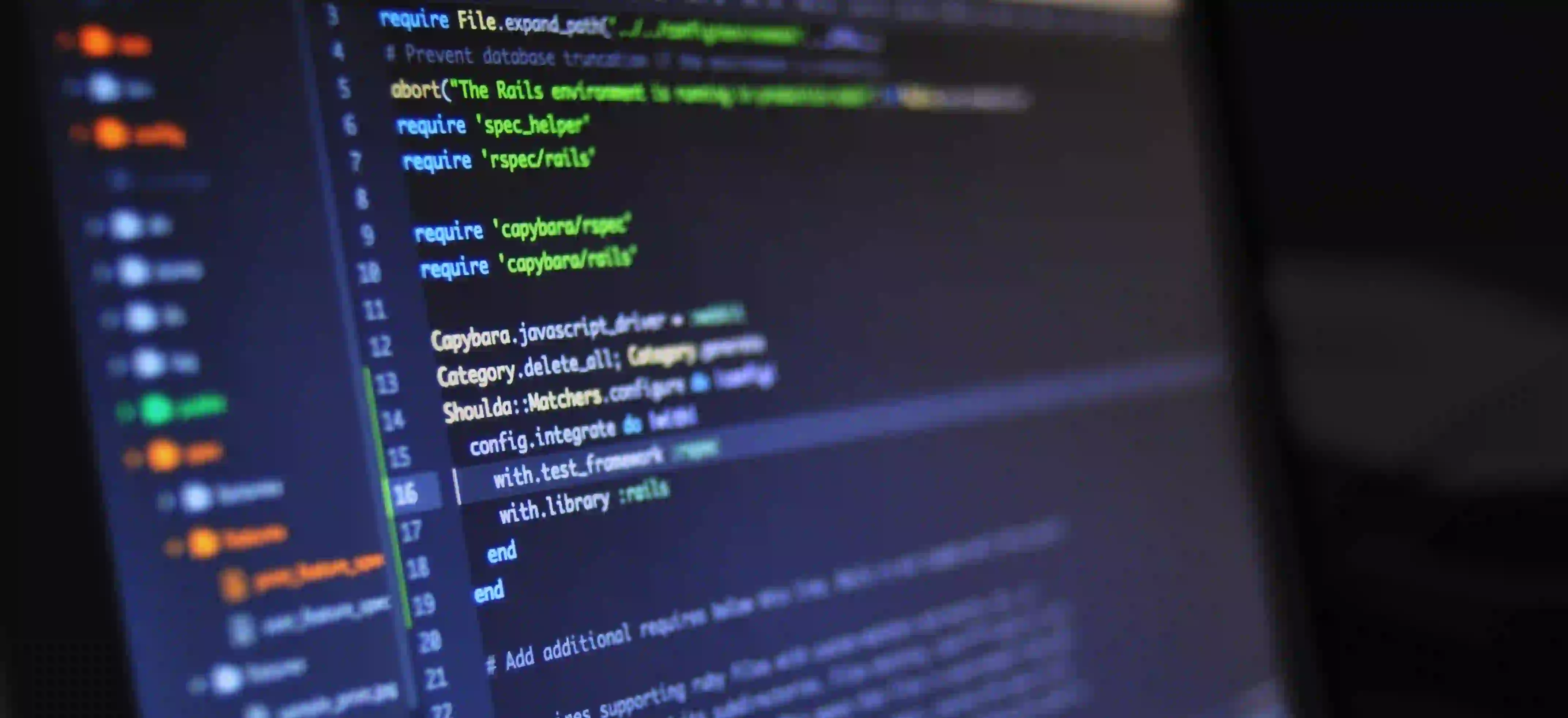
Resolving ClassLoader Conflicts: A Java Developer's Guide
When developing Java applications, you might encounter various challenges. One of the most confusing and troublesome issues is ClassLoader conflicts. This guide aims to demystify ClassLoaders and provide you with effective strategies for resolving conflicts. By the end of this article, you'll have a better understanding of ClassLoader mechanics, common problems, and practical solutions.
Understanding ClassLoaders
ClassLoaders are part of the Java Runtime Environment (JRE) responsible for loading classes into memory. They serve several vital functions, such as:
- Loading classes as needed.
- Enforcing the visibility and access rules of packages.
- Managing the namespace of classes.
Here’s a quick overview of the ClassLoader hierarchy in Java:
- Bootstrap ClassLoader: Loads core Java classes (e.g., from the
rt.jar
). - Extension ClassLoader: Loads classes from the extensions directory (
jre/lib/ext
). - System ClassLoader: Loads application classes from the classpath.
Understanding these layers can help you troubleshoot ClassLoader issues effectively.
Why ClassLoader Conflicts Occur
ClassLoader conflicts typically arise in larger applications, particularly those using multiple libraries or frameworks (think Java EE apps or Spring-based projects). The main reasons for these conflicts include:
- Multiple versions of a library: In complex systems, different libraries may require different versions of the same dependency.
- Classpath issues: Classes might be loaded from different locations, causing inconsistencies.
- Isolated environments: When using frameworks like OSGi, ClassLoader isolation can lead to conflicts if not managed correctly.
Example of ClassLoader Conflict
Consider two versions of commons-lang
in your project's dependencies. Both versions have a method named StringUtils
, but they behave differently. When the class is loaded, the incorrect version could land in your code, causing runtime exceptions.
Code Example of ClassLoader Conflict
Let's look at a simplified example of how ClassLoader behavior can lead to issues.
// Class from version 2.4 of commons-lang
public class StringUtils {
public static String capitalize(String str) {
return str.charAt(0) + str.substring(1);
}
}
// Class from version 3.0 of commons-lang
public class StringUtils {
public static String capitalize(String str) {
return str.toUpperCase(); // a different behavior
}
}
If both versions are on the classpath without proper exclusion, you may encounter unexpected behavior.
Strategies for Resolving ClassLoader Conflicts
1. Use Dependency Management Tools
Modern build tools like Maven or Gradle provide robust dependency management features. They can help resolve conflicts by:
- Performing a dependency tree analysis.
- Excluding specific transitive dependencies.
Example in Maven:
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang</artifactId>
<version>2.6</version>
<exclusions>
<exclusion>
<groupId>commons-logging</groupId>
<artifactId>commons-logging</artifactId>
</exclusion>
</exclusions>
</dependency>
Using the dependency:tree
command can help you identify potential conflicts.
2. ClassLoader Annotations
For Java EE or Spring applications, you can use ClassLoader annotations to manage different versions of libraries. Utilizing the @ClassLoader
annotation ensures that your code uses the specified ClassLoader context for particular classes.
3. Isolate Your Libraries
If you have conflicting libraries, consider using an OSGi framework that allows you to isolate libraries. OSGi's modular approach can help manage different versions of classes more effectively.
4. Custom ClassLoader
In more advanced scenarios, you can create a custom ClassLoader to control how classes are loaded. Below is an example of a simple custom ClassLoader:
public class MyCustomClassLoader extends ClassLoader {
@Override
protected Class<?> findClass(String name) throws ClassNotFoundException {
byte[] b = loadClassData(name);
return defineClass(name, b, 0, b.length);
}
private byte[] loadClassData(String name) {
// Load class data from a specific location to prevent conflicts
// Placeholder for loading logic
}
}
Using a custom ClassLoader is a more advanced solution but can add the flexibility you may need to avoid conflicts.
5. Dependency Convergence
To eliminate conflicts, aim for dependency convergence. This means ensuring that all your libraries use the same version of shared dependencies. This approach can drastically reduce the potential for errors and inconsistencies.
6. Continuous Integration Practices
Implement CI/CD practices that include:
- Regular updates of dependencies.
- Automated tests to catch conflicts before they reach production.
By adhering to these practices, you can catch ClassLoader issues early in the development process.
Common Tools for Diagnosing ClassLoader Conflicts
- jvisualvm: A performance monitoring tool that can help you see which classes are loaded and from where.
- ClassLoader Hierarchy Visualizer: Tools available as plugins or standalone applications that can illustrate the ClassLoader hierarchy and help you visualize conflicts.
- Maven Dependency Plugin: This can analyze your dependencies, simplifying the identification of versions that can lead to conflicts.
Bringing It All Together
ClassLoader conflicts in Java can be a source of frustration for even the most seasoned developers. However, by understanding how ClassLoaders function and applying strategic management techniques, you can navigate these challenges effectively.
Do remember to use dependency management tools, practice sound coding principles, and continuously integrate your applications. With these strategies, you can minimize ClassLoader conflicts and focus on what really matters—building robust applications.
For more information on managing Java dependencies, check out the Maven official documentation.
Navigating Java's world can be complex, but with the right knowledge and tools, you'll be well-equipped to tackle any ClassLoader conflicts that arise in your development journey. Happy coding!