Mastering NullPointerExceptions in Java Streams and Lambdas
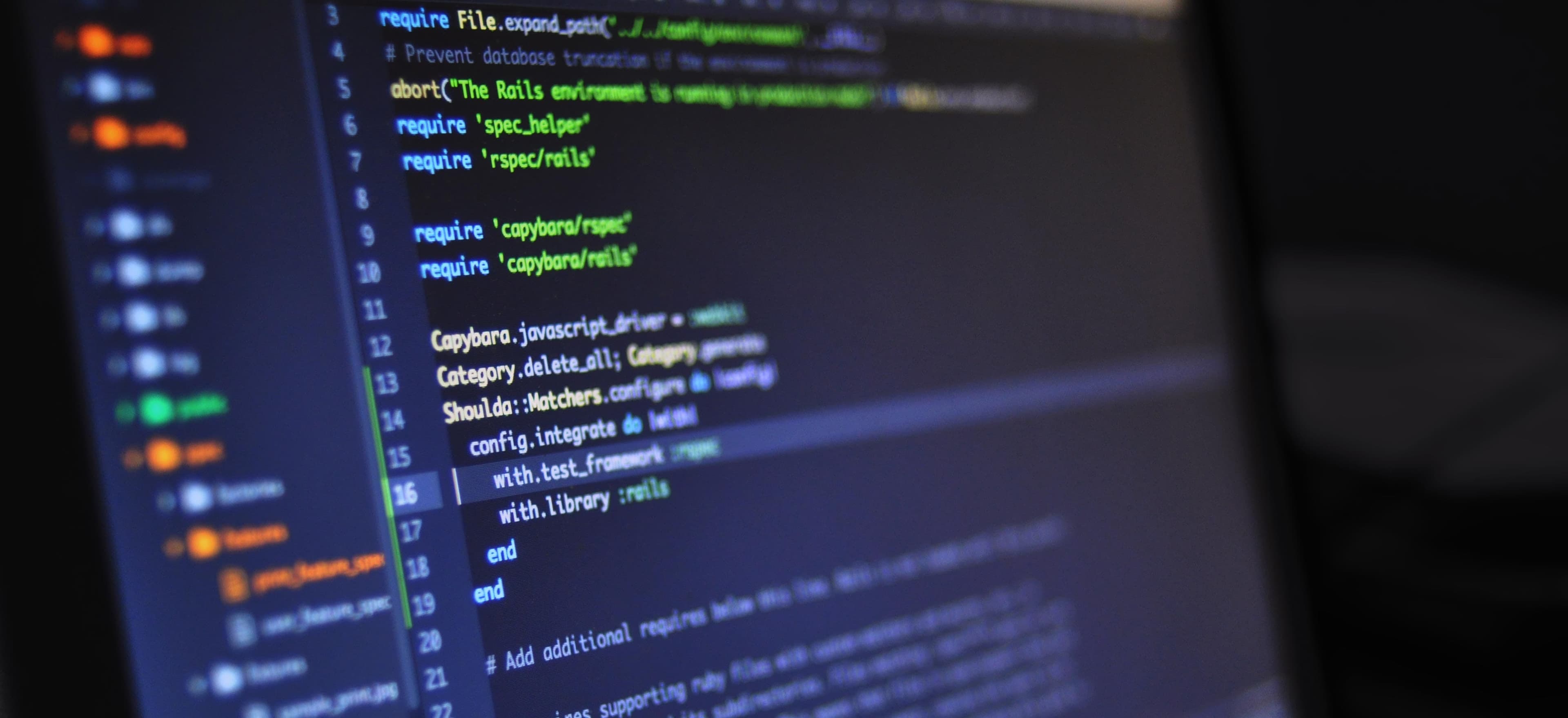
- Published on
Mastering NullPointerExceptions in Java Streams and Lambdas
Java is a powerful programming language used by millions of developers worldwide. Among its many features, the introduction of Streams and Lambda expressions redefined how developers approach data processing. However, with this sophistication comes complexity, particularly when dealing with NullPointerExceptions
. In this blog post, we'll explore how to effectively manage NullPointerExceptions
when working with Java Streams and Lambdas.
Understanding NullPointerExceptions
A NullPointerException
in Java occurs when the JVM attempts to use an object reference that has not been initialized or has been set to null
. This is often seen when methods are called on null
references, leading to runtime errors that can cripple application performance.
Common Scenarios for NullPointerExceptions
The following scenarios may generate NullPointerExceptions
:
- Dereferencing null references.
- Accessing methods or fields of null objects.
- Attempting to use an uninitialized variable.
These scenarios are crucial to understand, especially when processing collections with Streams.
The Power of Streams and Lambdas
Java Streams offer a streamlined approach to processing sequences of elements. Coupled with Lambda expressions, they can elegantly handle operations like filtering, mapping, and reducing collections. However, this convenience can also lead to NullPointerExceptions
if not handled properly.
Sample Stream Operations
Consider the following code snippet, which filters a list of strings to find non-null values:
import java.util.Arrays;
import java.util.List;
public class StreamNullCheckExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", null, "Bob", "Charlie", null);
// Filtering non-null names
List<String> filteredNames = names.stream()
.filter(name -> name != null) // Check for null before operations
.toList();
System.out.println(filteredNames); // Output: [Alice, Bob, Charlie]
}
}
Why the Filter?
The filter
method is essential in the above code to exclude null
values beforehand. If you were to omit this check, subsequently trying to process the names (e.g., printing their lengths) could lead to NullPointerExceptions
.
Strategies to Avoid NullPointerExceptions in Streams
To effectively avoid NullPointerExceptions
, consider implementing the following strategies:
1. Nullable Annotations
Using annotations such as @Nullable
from the Javax or JetBrains libraries can help make it clear where null values are acceptable. This can lead to safer code where developers are more aware of potential null references.
2. Optional Class
Java 8 introduced the Optional
class, which can be used to represent nullable values instead of relying on null references directly.
Here’s an example that utilizes Optional
:
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
public class OptionalExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", null, "Bob");
List<Optional<String>> optionalNames = names.stream()
.map(Optional::ofNullable) // Wrap in Optional to handle null values
.toList();
optionalNames.forEach(optName -> {
// Safely process or handle absence
System.out.println(optName.orElse("No Name Provided"));
});
}
}
Why Use Optionals?
Optional
acts as a container that may or may not hold a value, thereby making it easier to deal with cases where a value might not be present. This leads to cleaner, more robust code.
3. Use of Map with Safety
A common use case with Streams involves transforming elements. Lambda expressions allow for seamlessly mapping values while avoiding null checks inline.
import java.util.Arrays;
import java.util.List;
public class SafeMappingExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", null, "Bob");
// Safely mapping to upper case, if not null
List<String> upperCaseNames = names.stream()
.map(name -> (name != null ? name.toUpperCase() : "Unnamed")) // Provide default value
.toList();
System.out.println(upperCaseNames); // Output: [ALICE, Unnamed, BOB]
}
}
Why Mapping?
Using the safe mapping approach means you always have a result, even if the original value is null. This technique prevents NullPointerExceptions
while providing a default value when necessary.
Testing and Robustness
Testing your code for null scenarios is equally crucial. By using JUnit or any other testing framework, you can ensure that your streams and lambdas function correctly even with null inputs.
Here’s a simple test case:
import org.junit.jupiter.api.Test;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class NullHandlingTest {
@Test
public void testOptionalHandlingWithNulls() {
List<String> names = Arrays.asList("Alice", null, "Bob");
List<Optional<String>> optionalNames = names.stream()
.map(Optional::ofNullable)
.toList();
assertEquals("No Name Provided", optionalNames.get(1).orElse("No Name Provided"));
}
}
The Importance of Testing
Unit testing helps catch potential NullPointerExceptions
during development rather than in production, saving time and improving reliability.
Bringing It All Together
Mastering NullPointerExceptions
when using Java Streams and Lambdas is key to writing robust applications. Using techniques like filtering, leveraging the Optional
class, and implementing safe mapping can greatly reduce the chances of encountering runtime errors. Remember, it's not just about preventing exceptions but also about writing clear, manageable, and safe code.
For further reading on Java Streams and Lambdas, check the official Java documentation and Effective Java by Joshua Bloch.
By mastering these tools and strategies, you can ensure that your Java applications remain resilient in the face of null references. Happy coding!
Checkout our other articles