The Hidden Dangers of Using Volatile Variables in Java
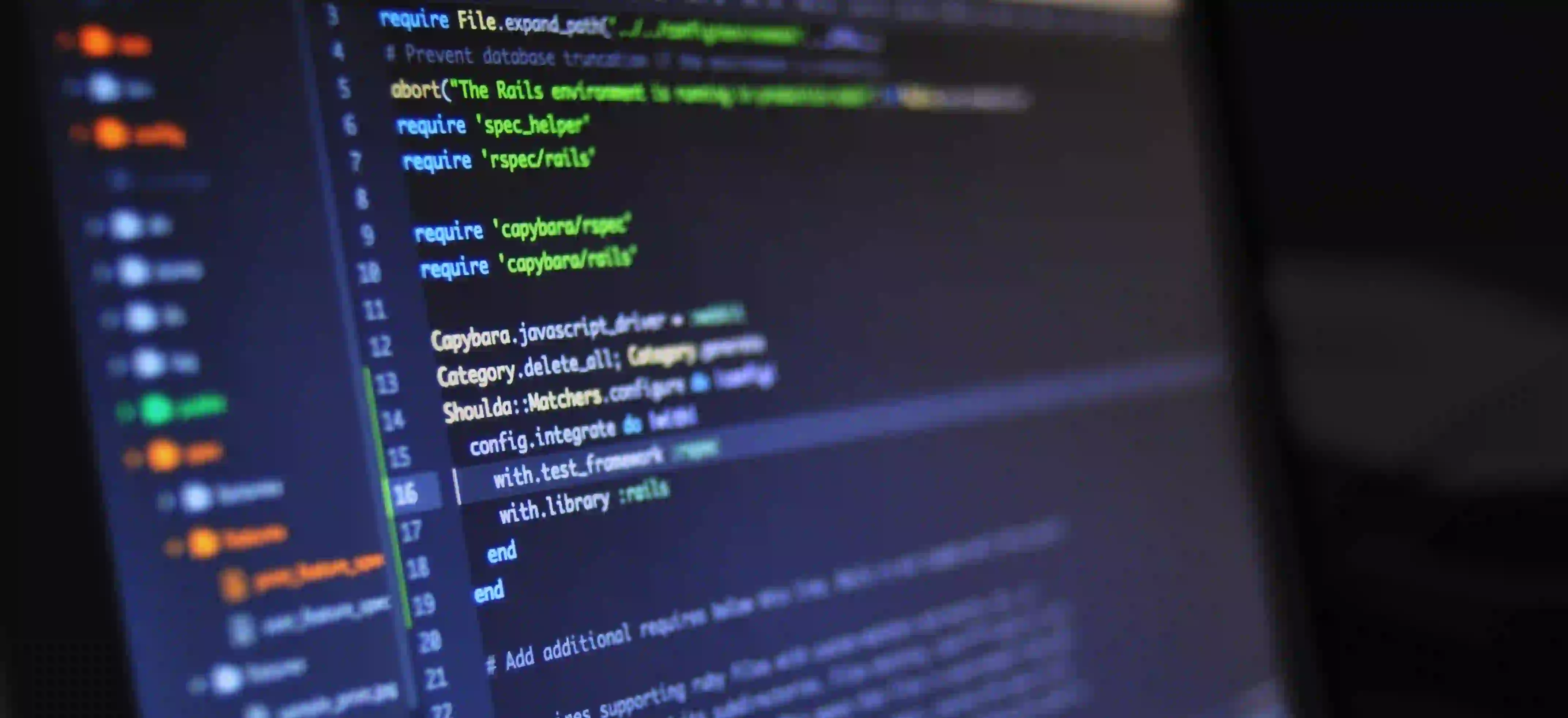
The Hidden Dangers of Using Volatile Variables in Java
Understanding the nuances of threading and synchronization in Java is crucial for building robust applications. Among the mechanisms Java provides for this purpose, the volatile
keyword often stands at the forefront. While it offers certain advantages for multithreaded programming, using volatile variables can lead to unexpected behaviors and subtle bugs. In this blog post, we'll explore the hidden dangers of using volatile
variables in Java, elucidate their behavior, and provide best practices for their use.
What are Volatile Variables?
In Java, the volatile
keyword is used to indicate that a variable's value will be modified by different threads. When a variable is declared as volatile
, it ensures that the most up-to-date version of that variable is always visible to all threads.
Here is a basic definition:
volatile int myVolatileVar;
Why Use Volatile?
Before diving into the dangers, let's understand why one would choose volatile
over other synchronizations, such as locks (synchronized
blocks).
- Visibility Guarantee: Modifications made by one thread to a
volatile
variable are visible to other threads immediately. - Atomicity of Reads/Writes: Reads and writes to
volatile
variables are atomic. - Lightweight: Using
volatile
can reduce the overhead associated with acquiring and releasing locks.
Hidden Dangers of Volatile Variables
However appealing it may seem, volatile
comes with significant drawbacks that programmers should be aware of. Let's discuss some of these dangers in detail.
1. Lack of Atomicity for Compound Actions
While reads and writes to volatile variables are atomic, compound actions—like checking then updating—are not. Consider the following scenario:
class Counter {
private volatile int count = 0;
public void increment() {
count++; // Not atomic
}
public int getCount() {
return count;
}
}
In this example, count++
is not an atomic operation; it consists of reading, incrementing, and writing the value back. This can lead to race conditions where multiple threads may read the same value and perform increments simultaneously, resulting in incorrect total counts.
2. Possible Outdated Data
With volatile
, you might think that reading a variable always returns the latest value. However, this is not the case when dealing with non-volatile variables.
class SharedResource {
private volatile boolean flag = false;
private int data;
public void writer() {
data = 42; // Not volatile
flag = true; // volatile
}
public void reader() {
if (flag) {
System.out.println(data); // Could print 0
}
}
}
In the above example, the reader
might observe the flag
as true
while the data
could still be 0
. This occurs because flag
and data
are not synchronized together leading to what may be termed as a "stale read".
3. No Mutual Exclusion
A significant aspect of multithreading is ensuring that certain code blocks can be accessed by only one thread at a time. Using volatile
does not help in achieving mutual exclusion. In scenarios where critical sections of code need to execute without interference from other threads, volatile
alone won't suffice.
class SafeCounter {
private volatile int count = 0; // Thread-safe for direct reads and writes
public void safeIncrement() {
count++; // Still not thread-safe
}
}
In this case, concurrent increments could still lead to inconsistent results. Utilizing synchronized
methods or locks would be necessary here.
4. Possible Performance Hit
Although volatile
variables can reduce locking overhead, they may incur a performance penalty. This is because the CPU may be forced to read from main memory rather than caches, decreasing access speed. Hence, use volatile
only when necessary, balancing performance with safety.
5. Misleading Semantics
The intent behind using volatile
could be misunderstood, particularly for less experienced developers. The presence of volatile
signals that a variable can be accessed by multiple threads, creating misleading assumptions about the thread-safety of the code.
Best Practices for Using Volatile Variables
Despite the dangers, there are practical cases for using volatile
meaningfully:
- Use for State Flags:
volatile
is useful in flags to control the stopping of threads. For instance, setting a boolean flag to stop a worker thread can be appropriately done using avolatile
variable.
class Worker extends Thread {
private volatile boolean running = true;
public void run() {
while (running) {
// Perform work
}
}
public void stopWorker() {
running = false; // Immediately visible to other threads
}
}
-
Double-checked Locking: If you opt for a singleton pattern, use a combination of volatile and synchronized blocks for effective double-checked locking to prevent multiple threads from instantiating the singleton class.
-
Read/Write Scenarios: When a variable is written by one thread but read by others with no need for atomicity in compound actions,
volatile
can be beneficial. -
Thorough Documentation: Always ensure to document the use of
volatile
clearly in your code. This helps other developers understand the reasons behind its usage and the conditions under which it operates effectively.
Closing the Chapter
While volatile
can be a powerful tool in a Java developer's toolkit, its misapplication can lead to bugs that are challenging to diagnose. Understanding its limitations is crucial for ensuring thread safety and application integrity. Whenever there is a need for complex interactions between threads, consider leveraging more sophisticated concurrency utilities provided in the java.util.concurrent
package, such as ReentrantLock
, CountDownLatch
, and others.
For further reading on synchronization in Java, explore Java Concurrency in Practice or delve into the Java Documentation on Concurrency.
Remember, the right choice depends on your application’s needs. Use volatile
judiciously, and you can avoid some of the hidden dangers that come with multithreading in Java.