Fixing the Java NoSuchMethodError: Common Causes and Solutions
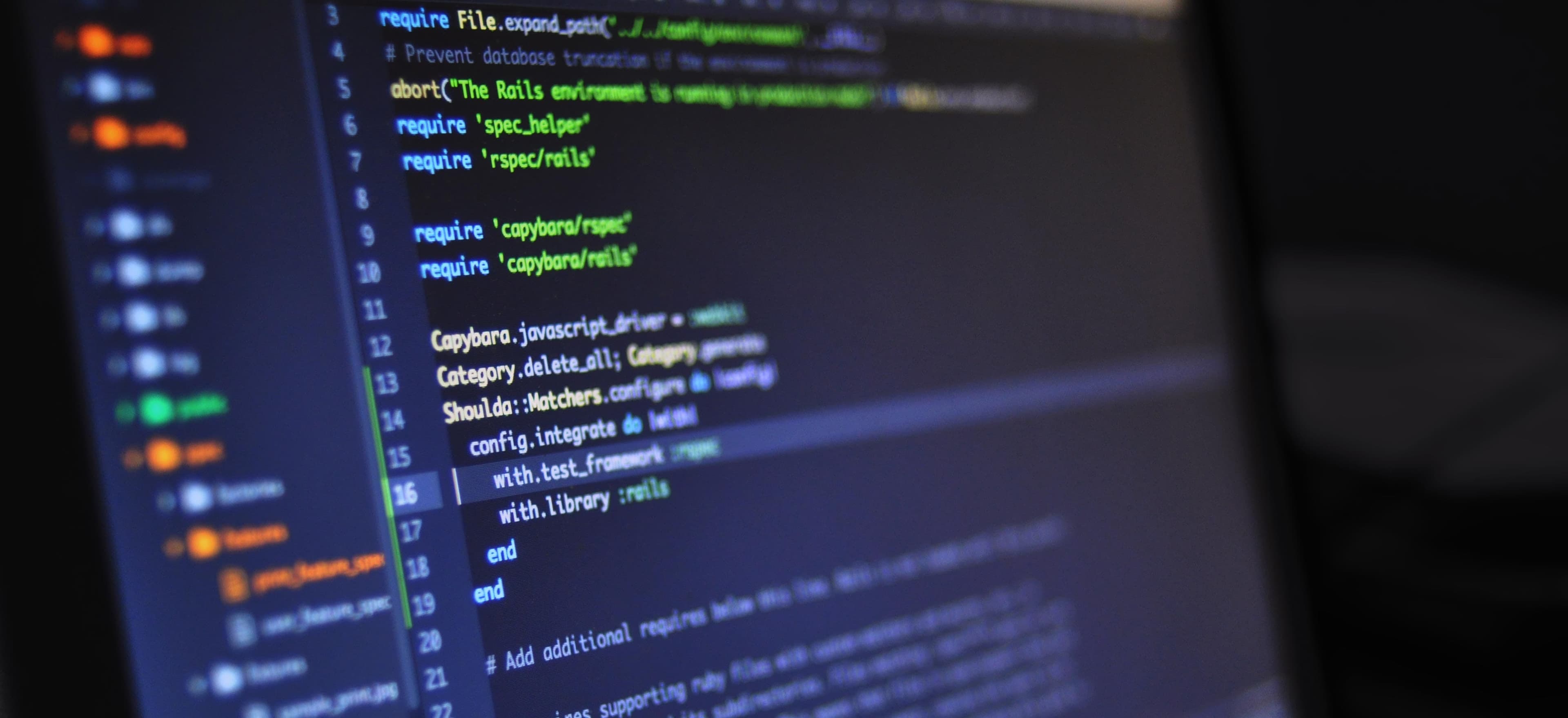
- Published on
Fixing the Java NoSuchMethodError: Common Causes and Solutions
Java is a powerful and versatile programming language, but it is not without its pitfalls. One common issue that developers encounter is the NoSuchMethodError
. In this blog post, we'll delve deep into the root causes of this error and discuss various strategies to resolve it. Along the way, we’ll illustrate our points with code snippets, practical tips, and useful links for further reading.
Understanding NoSuchMethodError
The NoSuchMethodError
is a runtime exception that indicates that the Java Virtual Machine (JVM) is trying to invoke a method that does not exist in a class. This can happen for a variety of reasons, including but not limited to:
- Method signature mismatches
- Classpath issues
- Versioning problems in dependencies
Let’s break down these scenarios to fully understand how to prevent or resolve them.
1. Method Signature Mismatches
One of the primary causes of the NoSuchMethodError
can be tracing back to method signature differences. In Java, method signatures include the method name and its parameter types. If you change either, you can cause significant confusion during execution.
Example Scenario:
Suppose you have a class Calculator
:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
Now, let’s say a different developer modifies this class to change the parameter type:
public class Calculator {
public int add(double a, double b) { // Changed from int to double
return (int) (a + b);
}
}
During runtime, if you attempt to call the original add(int, int)
method that no longer exists, you will encounter a NoSuchMethodError
.
Solution:
- Always verify that method signatures are consistent across all class versions.
- Use IDE refactoring tools to update method calls automatically. This reduces the chances of discrepancies.
2. Classpath Issues
Classpath problems can also lead to the NoSuchMethodError
. Having multiple versions of a class in the classpath can cause the JVM to use the wrong version at runtime.
Example Scenario:
Assume you have two JAR files loaded in your build path, one containing an older version of your class:
- library-v1.jar (contains an old method)
- library-v2.jar (contains your latest code)
If the JVM decides to load library-v1.jar
, you may run into the NoSuchMethodError
.
Solution:
- Check your build configuration and ensure that only the necessary JAR files are included.
- Use build systems like Maven or Gradle that manage dependencies effectively to prevent conflicts.
3. Versioning Problems in Dependencies
With Java projects, it is common to rely on various libraries or frameworks. If you update a library but your code is still expecting an older version with different method signatures, a NoSuchMethodError
may occur.
Example Scenario:
You may be using a library for JSON parsing:
import com.example.json.JSONParser;
public class Main {
public static void main(String[] args) {
JSONParser parser = new JSONParser();
parser.parse("{ \"key\" : \"value\" }");
}
}
If the library is updated and the parse
method is modified or removed, you will see the NoSuchMethodError
.
Solution:
- Regularly check for library updates and consult the library's change logs to understand impact.
- Use semantic versioning principles to manage your dependencies effectively.
Best Practices to Avoid NoSuchMethodError
Use Interface Segregation
Define your methods in interfaces so that multiple implementations can be provided without changing method signatures extensively. This can also help you avoid tight coupling, which could lead to the dreaded NoSuchMethodError
.
public interface CalculatorInterface {
int add(int a, int b);
}
public class BasicCalculator implements CalculatorInterface {
public int add(int a, int b) {
return a + b;
}
}
Keep Your Build Tools Updated
If you’re using Maven or Gradle, keep your build tools and dependencies updated. This will ensure you benefit from the latest features and fixes.
<!-- pom.xml configuration for Maven -->
<dependency>
<groupId>org.example</groupId>
<artifactId>library</artifactId>
<version>2.0.0</version>
</dependency>
Maintain Backward Compatibility
If you’re modifying methods, aim to maintain backward compatibility whenever possible. This tie of approach will reduce breaking changes and NoSuchMethodError
occurrences.
Summary
The NoSuchMethodError
can be a frustrating issue for developers, but understanding its causes allows you to tackle it head-on. Key takeaways include:
- Method signature consistency: Always double-check your method signatures, especially after modification.
- Classpath management: Ensure only one version of a class is accessible to the JVM at runtime.
- Managing dependencies: Keep track of library updates and implement semantic versioning where applicable.
For further insight into Java exception handling, feel free to check out the official Java Documentation on Exceptions.
For more on dependency management, take a look at Maven Dependency Management.
By adhering to the practices discussed in this article, you should be able to significantly minimize the chances of encountering a NoSuchMethodError
in your Java applications. Happy coding!