Decoding Java Memory Issues: Common Pitfalls Explained
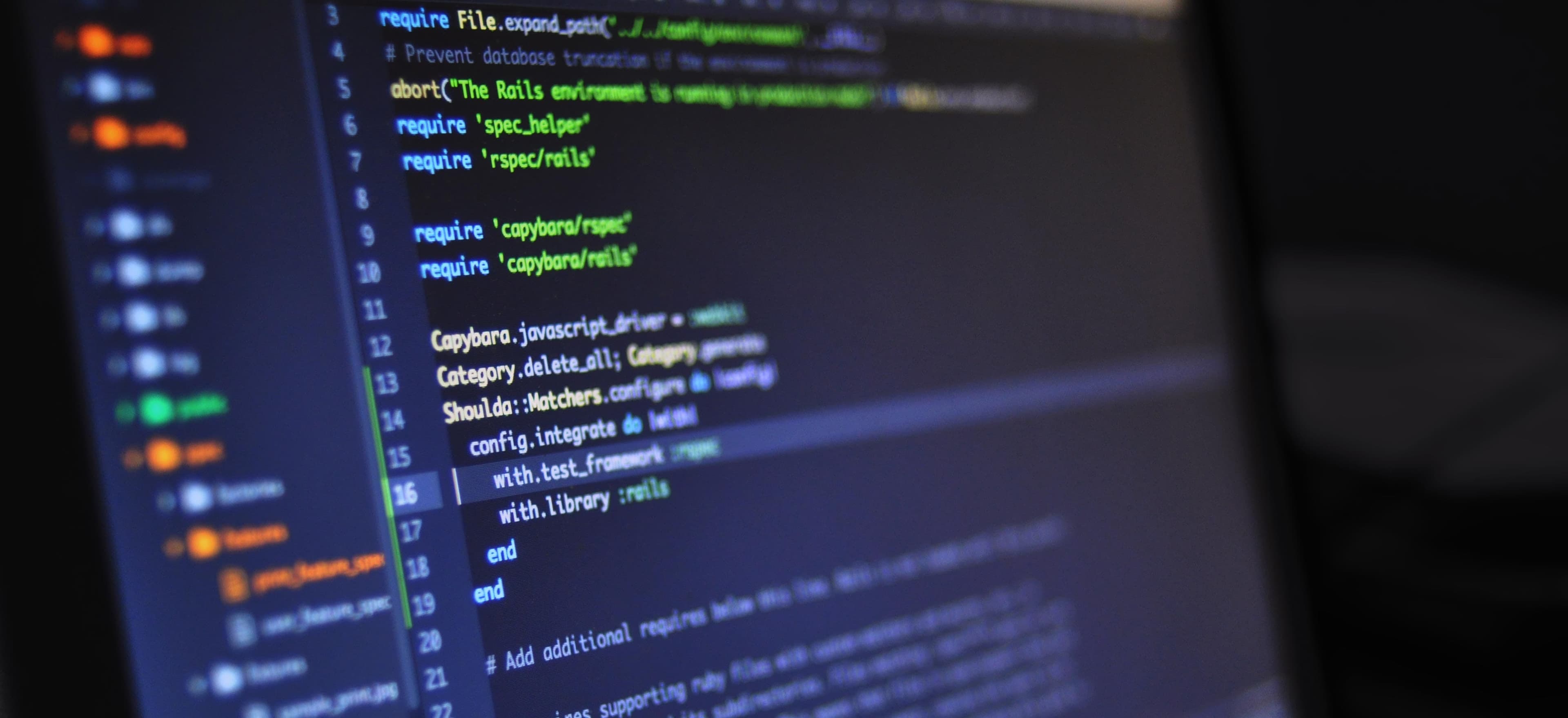
- Published on
Decoding Java Memory Issues: Common Pitfalls Explained
Java is known for its simplicity and robustness, allowing developers to focus on solving problems instead of dealing with low-level memory management. However, as applications grow in complexity, memory issues may surface, leading to performance bottlenecks or crashes. In this blog post, we will dissect common Java memory issues, their causes, and offer solutions to mitigate them.
Understanding Java Memory Management
Before diving into specific memory issues, it’s essential to understand how Java handles memory. The Java Virtual Machine (JVM) uses a system called the heap for dynamic memory allocation and a stack for static memory allocation. The heap is where objects are created, while the stack is where primitives and references to objects are stored.
There are also areas within the heap that we must consider:
- Young Generation: This is where newly created objects reside. They are frequently collected by the garbage collector (GC).
- Old Generation: Objects that survive multiple GCs may be promoted to this area.
- Permanent Generation (Metaspace in Java 8 and above): This is where the JVM stores class definitions and metadata.
The efficient use of these memory areas is crucial for optimal performance.
Common Java Memory Issues
1. OutOfMemoryError
The OutOfMemoryError
occurs when the JVM runs out of memory for allocation. Unlike runtime exceptions, this error is critical and often leads to application failure.
Causes:
- Excessive object creation
- Memory leaks
- Incorrect configuration of heap space
Solution:
Increase the heap size using JVM options. Example:
java -Xms512m -Xmx1024m -jar yourapp.jar
This command sets the initial heap size to 512MB and the maximum to 1024MB. However, this should not always be your go-to solution. It's essential to analyze and optimize your code first.
2. Memory Leaks
Memory leaks occur when an application retains references to objects that are no longer needed. Over time, this can consume substantial memory.
Causes:
- Unintentional references (e.g., static collections that keep growing)
- Unreleased resources (e.g., database connections)
Solution:
Use tools like VisualVM or Eclipse Memory Analyzer to identify memory leaks.
Here is an example of a memory leak caused by a static list:
import java.util.ArrayList;
import java.util.List;
public class MemoryLeakExample {
private static List<String> dataList = new ArrayList<>();
public void addData(String data) {
dataList.add(data); // This keeps adding data indefinitely
}
}
Why This Happens: The static dataList
retains all added strings indefinitely. Consider switching to a non-static list or clearing it periodically.
3. Excessive Garbage Collection
Garbage collection is a double-edged sword. While it's essential for memory management, excessive GC can degrade application performance.
Causes:
- Large number of short-lived objects (common in high-throughput applications)
Solution:
Minimize object creation. Consider object pooling or using more efficient data structures.
For instance, instead of creating a new String object each time, use a StringBuilder
.
public String concatenateStrings(List<String> strings) {
StringBuilder sb = new StringBuilder();
for (String str : strings) {
sb.append(str);
}
return sb.toString();
}
Why This is Better: StringBuilder
minimizes the number of string objects created, reducing GC pressure.
4. Misconfigured JVM Settings
The default settings might not suit your application, especially under heavy load.
Causes:
- Default heap size limitations
- Garbage collector configuration
Solution:
Tweak the JVM settings for your specific use case.
Example of a command to specify a different garbage collector:
java -XX:+UseG1GC -jar yourapp.jar
Why this Matters: The G1 Garbage Collector is designed for applications with large heaps and can improve latency by prioritizing the collection of regions with the most garbage.
5. PermGen Space Issues
For Java versions before Java 8, using a too-small Permanent Generation can lead to java.lang.OutOfMemoryError: PermGen space
. After Java 8, PermGen is replaced by Metaspace, which dynamically resizes, but similar issues can still occur.
Causes:
- Too many loaded classes
- Large class metadata
Solution:
In Java 7 and below, increase PermGen size:
java -XX:MaxPermSize=256m -jar yourapp.jar
For Java 8 and above, monitor class loading and explore class unloading options.
Best Practices for Memory Management
While recognizing and solving memory issues is vital, you also should adopt best practices to prevent these issues in the first place:
1. Perform Regular Profiling
Tools like Java Flight Recorder or JProfiler can help monitor memory consumption and analyze efficiency.
2. Optimize Data Structures
Choose data structures that align with your use case. For example, if you rarely access elements by index, a LinkedList
can save memory compared to an ArrayList
.
3. Release Resources Explicitly
Always close resources like database connections, file streams, etc. Implement a finally
block:
Connection conn = null;
try {
conn = DriverManager.getConnection(url, user, password);
// Use connection
} catch (SQLException e) {
e.printStackTrace();
} finally {
if (conn != null) {
conn.close();
}
}
Why This is Important: It ensures that resources are freed even in cases of exceptions.
4. Write Efficient Code
Look for ways to enhance your code's efficiency. Avoid unnecessary computations, and reuse objects wherever possible.
Wrapping Up
In this blog post, we delved into various Java memory issues, highlighted their causes, and provided solutions to tackle each problem effectively. Memory management is a crucial skill for any Java developer, underlining the importance of profiling and optimizing code. By applying the best practices discussed, you can significantly enhance your application's stability and performance.
For further insights on optimizing Java applications, be sure to check out the Java Performance Tuning guide. By being mindful of these common pitfalls, you can utilize Java’s robust memory management features to their fullest potential. Happy coding!
Checkout our other articles