Mastering ClassNotFoundException and NoClassDefFoundError
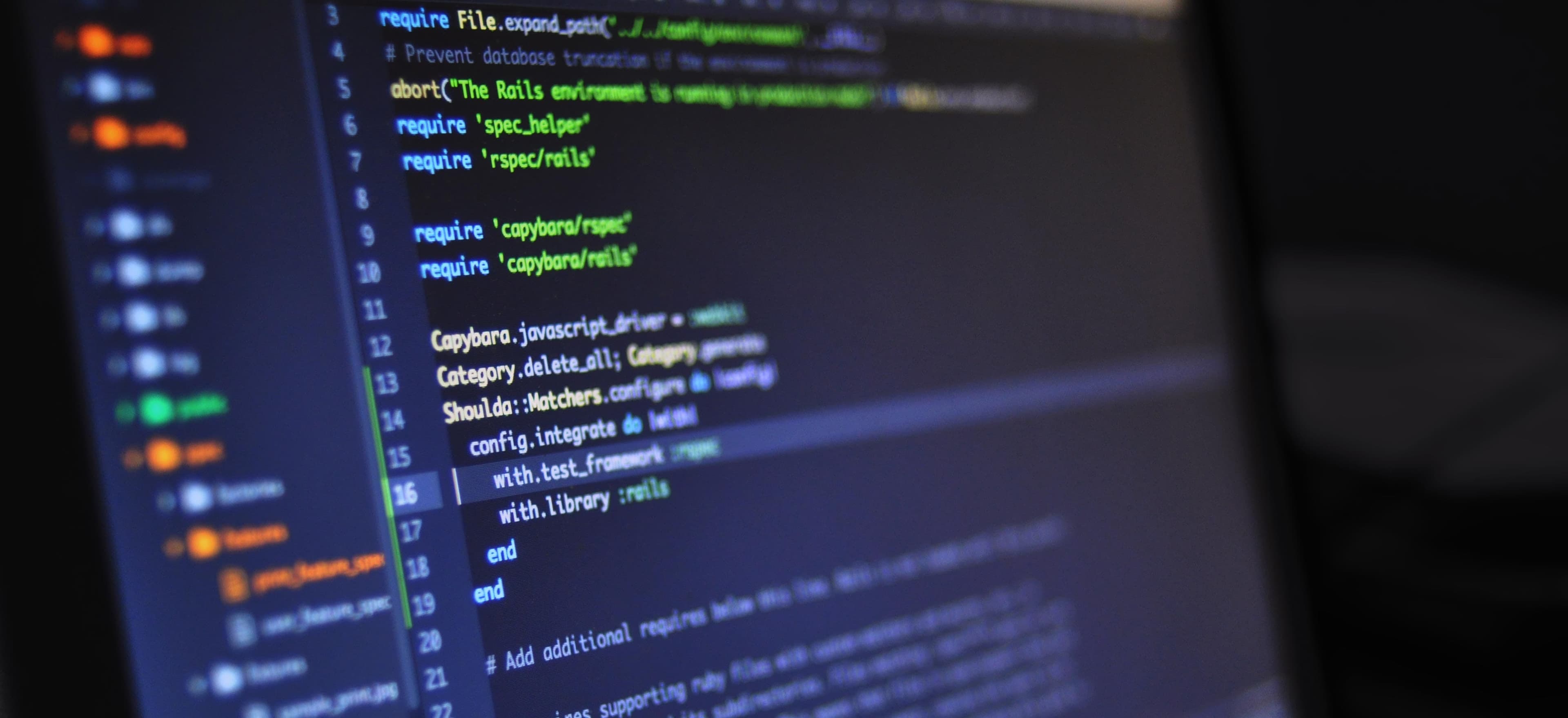
- Published on
Mastering ClassNotFoundException and NoClassDefFoundError in Java
Java is renowned for its portability and versatility, yet developers still encounter challenges when running Java applications. Two of the most common exceptions they face are ClassNotFoundException
and NoClassDefFoundError
. Understanding the nuances of these errors is essential for any Java programmer. In this post, we will delve into these exceptions, how they occur, and strategies for resolving them.
Table of Contents
- Understanding ClassNotFoundException
- Understanding NoClassDefFoundError
- Common Causes
- How to Fix These Errors
- Best Practices
- Conclusion
Understanding ClassNotFoundException
What is ClassNotFoundException?
ClassNotFoundException
is a checked exception in Java, which means it must be either caught or declared in the method signature. This exception is thrown when an application tries to load a class through its string name using methods like Class.forName()
, but the class cannot be found in the classpath.
Example of ClassNotFoundException
public class ClassNotFoundExample {
public static void main(String[] args) {
try {
Class.forName("com.example.NonExistentClass");
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
Why Does This Matter?
In this example, we attempt to load a class that does not exist. The exception is caught, and its stack trace is printed. This is a common pattern in Java applications, especially when dealing with reflection.
Understanding NoClassDefFoundError
What is NoClassDefFoundError?
On the other hand, NoClassDefFoundError
is an error (not an exception) that occurs when the JVM notifies you that it could not find the definition of a class that was already loaded during the compilation but is not available at runtime.
Example of NoClassDefFoundError
public class NoClassDefFoundExample {
static {
// This simulates a scenario where a dependency is missing at runtime
throw new RuntimeException("Simulated error");
}
public static void main(String[] args) {
try {
new ExampleClass(); // Suppose ExampleClass was available during compilation but not at runtime
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why Does This Matter?
In this scenario, even though ExampleClass
was compiled successfully, the JVM throws NoClassDefFoundError
when it attempts to load the class during execution due to a failure in the static block.
Common Causes
ClassNotFoundException Causes
- Incorrect Classpath: The most common reason is that the class is not available in the declared classpath.
- Spelling Errors: Typos in the classname or package name can lead to this exception.
- Dependency Issues: Missing libraries or modules can prevent the loading of classes.
NoClassDefFoundError Causes
- Classpath Issues: Similar to
ClassNotFoundException
, a missing class from the runtime classpath can lead to errors. - Changes in Class Structure: If a class is modified significantly after being compiled, it may not be recognized successfully at runtime.
- Incompatible Dependencies: Using a class that relies on another class that isn’t available can also prompt this error.
How to Fix These Errors
Fixing ClassNotFoundException
-
Check Classpath Settings: Ensure that your classpath includes all necessary directories and JAR files. If you’re using an IDE like Eclipse or IntelliJ IDEA, you can manage your classpath settings easily within the project’s properties.
-
Validate Class Names: Make sure there are no typographical errors in the class name or package name.
Example correction:
Class.forName("com.example.ExampleClass"); // Correct usage
-
Maven/Gradle Dependencies: If you're using Maven or Gradle, ensure that your dependencies are correctly defined and downloaded. You can run
mvn clean install
orgradle build
to troubleshoot issues.
Fixing NoClassDefFoundError
-
Verify Runtime Classpath: Just like for
ClassNotFoundException
, check that all necessary JARs are included in the runtime classpath. -
Recompile Classes: If a class was modified but not recompiled, recompiling can resolve inconsistencies.
-
Check for Compiler Warnings: Always be mindful of any warnings during compilation; they can hint at potential issues with class definitions.
-
Use Dependency Analysis Tools: Tools like Maven Dependency Plugin can help you analyze and resolve dependency issues.
Best Practices
To mitigate the chances of encountering ClassNotFoundException
and NoClassDefFoundError
, consider adopting the following best practices:
- Use Build Tools: Utilize build tools like Maven or Gradle that help manage dependencies effectively.
- Keep Dependencies Up-to-Date: Regularly update your libraries to their latest versions.
- Modularize Your Code: Consider organizing your code into multiple modules. This ensures that classes are loaded as needed and reduces the possibility of conflicts.
- Test in Different Environments: Test applications in development, staging, and production environments to ensure consistency in class availability.
- Implement Exception Handling: Handle these exceptions gracefully in your code to prevent application crashes.
My Closing Thoughts on the Matter
Understanding ClassNotFoundException
and NoClassDefFoundError
can significantly improve your debugging skills as a Java developer. By grasping their implications, causes, and solutions, you can navigate your Java projects with confidence.
The next time you encounter these errors, refer back to this guide to help you troubleshoot effectively. Happy coding!
For further reading on Java exceptions, visit the Java Documentation or explore community discussions on platforms like Stack Overflow.
Checkout our other articles